Python Tkinter实现的简单计算器
2016-12-11 11:25
531 查看
最近初次接触Python,就用计算器来练练手吧。
这个计算器的ui,我自己都不忍心看。
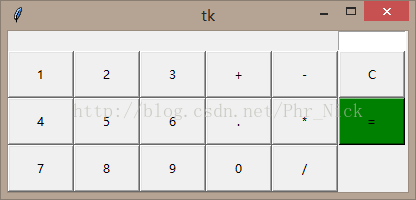
对于代码的一些优化:
①由于用到很多的按钮,我们可以用for循环来简化代码,参考代码如下:
②可以用lambda表达式,来简化代码:这样可以少写一个污染函数,是代码更加简洁
//对于上述代码的一些疑问:
//如果将grid直接写在后面,点击之后就会报错:
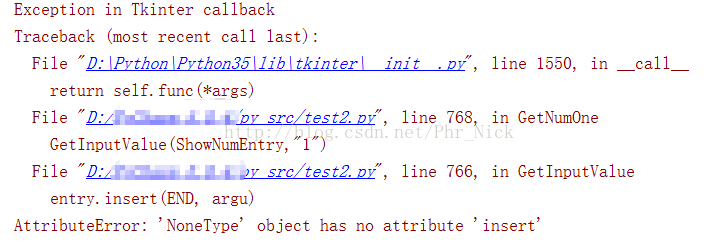
from tkinter import * def GetInputValue(ShowNumEntry, Value):#用来显示值 ShowNumEntry.insert(END,Value) def GetNumOne():#按钮1并显示 GetInputValue(ShowNumEntry,"1") def GetNumTwo():#按钮2并显示 GetInputValue(ShowNumEntry,"2") def GetNumThree():#按钮3并显示 GetInputValue(ShowNumEntry,"3") def GetNumFour():#按钮4并显示 GetInputValue(ShowNumEntry,"4") def GetNumFive():#按钮5并显示 GetInputValue(ShowNumEntry,"5") def GetNumSix():#按钮6并显示 GetInputValue(ShowNumEntry,"6") def GetNumSeven():#按钮7并显示 GetInputValue(ShowNumEntry,"7") def GetNumEight():#按钮8并显示 GetInputValue(ShowNumEntry,"8") def GetNumNine():#按钮9并显示 GetInputValue(ShowNumEntry,"9") def GetNumZero():#按钮0并显示 GetInputValue(ShowNumEntry,"0") def GetDot():#按钮.并显示 GetInputValue(ShowNumEntry,".") def GetPlus():#按钮+并显示 GetInputValue(ShowNumEntry,"+") def GetMinus():#按钮-并显示 GetInputValue(ShowNumEntry,"-") def GetClean():#清除 ShowNumEntry.delete(0,END) def GetMultiply():#按钮*并显示 GetInputValue(ShowNumEntry,"*") def GetDivision():#按钮/并显示 GetInputValue(ShowNumEntry,"/") def GetResult():#计算结果并显示 result = eval(ShowNumEntry.get()) GetClean() ShowNumEntry.insert(0,str(result)) root = Tk() root.wm_minsize(400,160)#设置窗口大小 ShowNumEntry = Entry(root, width=8, justify="right", font=12 ) ShowNumEntry.grid(row=0, column=5) NumOneBtn = Button(root,text = "1", width = 8,height = 2,command = GetNumOne) NumOneBtn.grid(row=1, column=0) NumTwoBtn = Button(root,text = "2", width = 8,height = 2,command = GetNumTwo) NumTwoBtn.grid(row=1, column=1) NumThreeBtn = Button(root,text = "3", width = 8,height = 2,command = GetNumThree) NumThreeBtn.grid(row=1, column=2) PlusBtn = Button(root,text = "+", width = 8,height = 2,command = GetPlus) PlusBtn.grid(row=1, column=3) MinusBtn = Button(root,text = "-", width = 8,height = 2,command = GetMinus) MinusBtn.grid(row=1, column=4) CleanBtn = Button(root,text = "C", width = 8,height = 2,command = GetClean) CleanBtn.grid(row=1, column=5) NumFourBtn = Button(root,text = "4", width = 8,height = 2,command = GetNumFour) NumFourBtn.grid(row=2, column=0) NumFiveBtn = Button(root,text = "5", width = 8,height = 2,command = GetNumFive) NumFiveBtn.grid(row=2, column=1) NumSixBtn = Button(root,text = "6", width = 8,height = 2,command = GetNumSix) NumSixBtn.grid(row=2, column=2) DotBtn = Button(root,text = ".", width = 8,height = 2,command =GetDot) DotBtn.grid(row=2, column=3) MultiplyBtn = Button(root,text = "*", width = 8,height = 2,command = GetMultiply) MultiplyBtn.grid(row=2, column=4) ResultBtn = Button(root,text = "=", width = 8, height = 2,background = "green",command=GetResult) ResultBtn.grid(row=2, column=5) NumSevenBtn = Button(root,text = "7",width = 8, height = 2, command = GetNumSeven) NumSevenBtn.grid(row=3, column=0) NumEightBtn = Button(root,text = "8", width = 8, height = 2, command = GetNumEight) NumEightBtn.grid(row=3, column=1) NumNineBtn = Button(root,text = "9", width = 8, height = 2, command = GetNumNine) NumNineBtn.grid(row=3, column=2) NumZeroBtn = Button(root,text = "0", width = 8, height = 2, command = GetNumZero) NumZeroBtn.grid(row=3, column=3) DivisionBtn = Button(root,text = "/", width = 8, height = 2, command = GetDivision) DivisionBtn.grid(row=3, column=4) root.mainloop()
这个计算器的ui,我自己都不忍心看。
对于代码的一些优化:
①由于用到很多的按钮,我们可以用for循环来简化代码,参考代码如下:
from tkinter import * #创建横条型框架 def frame(root, side): w = Frame(root) w.pack(side = side, expand = YES, fill = BOTH) return w #创建按钮 def button(root, side, text, command = None): w = Button(root, text = text, command = command) w.pack(side = side, expand = YES, fill = BOTH) return w root =Tk() for key in ('123', '456', '789', '-0.'): KeyF =frame(root,TOP) for char in key: button(KeyF, LEFT, char) root.mainloop()
②可以用lambda表达式,来简化代码:这样可以少写一个污染函数,是代码更加简洁
NumNineBtn = Button(root,text = "9", width = 8, height = 2, command = lambda : GetInputValue(ShowNumEntry,"9"))
NumNineBtn .grid(row=3, column=2)
//对于上述代码的一些疑问:
//如果将grid直接写在后面,点击之后就会报错:
def GetInputValue(entry, argu): entry.insert(END, argu) def GetNumOne(): GetInputValue(ShowNumEntry,"1") from tkinter import * root = Tk() ShowNumEntry = Entry(root, justify="right", font=12).grid(row=0, column=0) button = Button(root,text="测试",command=GetNumOne).grid(row=1, column=0) root.mainloop()
相关文章推荐
- python tkinter 实现简单计算器2
- python使用tkinter实现简单计算器
- python tkinter实现简单计算器
- 利用Tkinter(python3.6)实现一个简单计算器
- python的tkinter布局之简单的聊天窗口实现方法
- 基于Tkinter利用python实现颜色空间转换程序
- python3使用tkinter实现ui界面简单实例
- Python Tkinter实现的WIN7电脑端共享WIFI热点(一)
- Python用Tkinter的Frame实现眼睛护士的倒计时黑色屏幕
- Python基于Tkinter实现的记事本实例
- python的tkinter布局之简单的聊天窗口实现方法
- 用Python的Tkinter实现时钟
- python开发之tkinter实现图形随鼠标移动的方法
- python基于Tkinter库实现简单文本编辑器实例
- python:利用tkinter实现的计算器源代码
- 在Tkinter实现一个秒表(参照python cookbook)
- python3使用tkinter实现ui界面简单实例
- python通过Tkinter库实现的一个简单的文本编辑器代码
- Python Tkinter实现的linux命令帮助手册
- python tkinter用Treeview实现ListView效果