C# 学习Action与Func看Virtual。
2016-11-07 22:41
447 查看
通过代码简要描述Action、Func和Virtual。同时还可以了解到匿名委托和Lambda表达式的使用方法。
一、简要描述Action、Func和Virtual
1.Action与Func都是委托的一种,区别在于一个没有返回参数,一个有返回参数。
2.Virtual:关键字用于修饰方法、属性、索引器或事件声明,并且允许在派生类中重写这些对象。例如,此方法可被任何继承它的类重写。
★★★需要注意的是:virtual 修饰符不能与 static、abstract,
private 或 override 修饰符一起使用。
3.在MSDN中有详细的解释这里就不做过多的解释。下面我们就来看看代码!
二、Action :表示无参,无返回值的委托
Action<int,string> :表示有传入参数int,string无返回值的委托
Action<int,string,bool> :表示有传入参数int,string,bool无返回值的委托
Action<int,int,int,int> :表示有传入4个int型参数,无返回值的委托
Action至少0个参数,至多16个参数,无返回值。
下面代码演示的是 Action 无参例子:
1. ParentClass
2.ChildrenClass
3.main
4.运行结果
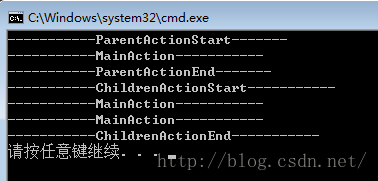
三、Func :是有返回值的泛型委托
Func<string> :表示无参,返回值为string的委托
Func<int,bool> 表示传入参数为int返回值为bool的委托
Func<T1,T2,,T3,int> 表示传入参数为T1,T2,,T3(泛型)返回值为int的委托
Func<object,string,int> 表示传入参数为object, string 返回值为int的委托
Func至少0个参数,至多16个参数,根据返回值泛型返回。必须有返回值,不可void
下面的代码分别演示Func<string>和Func<int,bool>
首先是:《Func<string> 》表示无参,返回值为string类型的委托。
1.parentClass
2.ChildrenClass
3.Main
4.运行结果
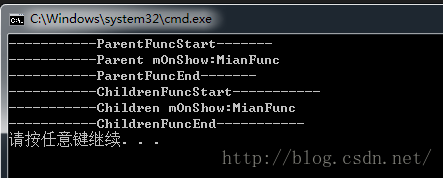
其次是:《Func<int,bool>》表示传入参数为int类型,返回值为bool类型的委托
1.ParentClass
2.ChildrenClass
3.main
4.运行结果
一、简要描述Action、Func和Virtual
1.Action与Func都是委托的一种,区别在于一个没有返回参数,一个有返回参数。
2.Virtual:关键字用于修饰方法、属性、索引器或事件声明,并且允许在派生类中重写这些对象。例如,此方法可被任何继承它的类重写。
★★★需要注意的是:virtual 修饰符不能与 static、abstract,
private 或 override 修饰符一起使用。
3.在MSDN中有详细的解释这里就不做过多的解释。下面我们就来看看代码!
二、Action :表示无参,无返回值的委托
Action<int,string> :表示有传入参数int,string无返回值的委托
Action<int,string,bool> :表示有传入参数int,string,bool无返回值的委托
Action<int,int,int,int> :表示有传入4个int型参数,无返回值的委托
Action至少0个参数,至多16个参数,无返回值。
下面代码演示的是 Action 无参例子:
1. ParentClass
class ParentClass { public virtual void ParentFunction(string mString, Action mOnShow) { Console.WriteLine("-----------ParentActionStart-------"); //?.Invoke是简写<简化委托调用>,等价于mOnShow != null 就调用mOnShow(); mOnShow?.Invoke(); Console.WriteLine("-----------ParentActionEnd-------"); } }
2.ChildrenClass
class ChildrenClass : ParentClass { public override void ParentFunction(string mString, Action mOnShow) { base.ParentFunction(mString, mOnShow); Console.WriteLine("-----------ChildrenActionStart-----------"); //判断字符串是否为空 if (!string.IsNullOrEmpty(mString)) { //?.Invoke是简写,等价于mOnShow != null 就调用mOnShow(); mOnShow?.Invoke(); if (mOnShow != null) //等价于上面的步骤 { mOnShow(); } //分开用两个if是为了介绍简化委托调用 if (mOnShow == null) { //使用Lambda表达式进行赋值 mOnShow = ()=>{ Console.WriteLine("-----------Children Assginment"); }; } Console.WriteLine("-----------ChildrenActionEnd-----------"); } } }
3.main
class Program { static void Main(string[] args) { ChildrenClass _children = new ChildrenClass(); string _str = "-----------Main-------------"; //使用匿名委托进行赋值 _children.ParentFunction(_str, delegate { Console.WriteLine("-----------MainAction-----------"); }); } }
4.运行结果
三、Func :是有返回值的泛型委托
Func<string> :表示无参,返回值为string的委托
Func<int,bool> 表示传入参数为int返回值为bool的委托
Func<T1,T2,,T3,int> 表示传入参数为T1,T2,,T3(泛型)返回值为int的委托
Func<object,string,int> 表示传入参数为object, string 返回值为int的委托
Func至少0个参数,至多16个参数,根据返回值泛型返回。必须有返回值,不可void
下面的代码分别演示Func<string>和Func<int,bool>
首先是:《Func<string> 》表示无参,返回值为string类型的委托。
1.parentClass
class ParentClass { public virtual void ParentFunction(string mString , Func<string> mOnShow) { Console.WriteLine("-----------ParentFuncStart-------"); //?.Invoke是简写<简化委托调用>,等价于mOnShow != null 就调用mOnShow(); string _str = mOnShow?.Invoke(); Console.WriteLine("-----------Parent mOnShow:" + _str); Console.WriteLine("-----------ParentFuncEnd-------"); } }
2.ChildrenClass
class ChildrenClass : ParentClass { public override void ParentFunction(string mString, Func<string> mOnShow) { base.ParentFunction(mString, mOnShow); Console.WriteLine("-----------ChildrenFuncStart-----------"); //判断字符串是否为空 if (!string.IsNullOrEmpty(mString)) { //?.Invoke是简写,等价于mOnShow != null 就调用mOnShow(); string _str = mOnShow?.Invoke(); if(mOnShow != null) //等价于上面的步骤 { _str = mOnShow(); } Console.WriteLine("-----------Children mOnShow:" + _str); //分开用两个if是为了介绍简化委托调用 if (mOnShow == null) { //使用Lambda表达式进行赋值 mOnShow = ()=>{ return "-----------ReadChildrenFunc-----------"; }; string _mStr = mOnShow(); Console.WriteLine("-----------ReadChildrenFunc mOnShow:" + _str); } Console.WriteLine("-----------ChildrenFuncEnd-----------"); } } }
3.Main
class Program { static void Main(string[] b2bb args) { ChildrenClass _children = new ChildrenClass(); string _str = "--------Main-------------"; //使用匿名委托进行赋值 _children.ParentFunction(_str, delegate { return "MianFunc"; }); } }
4.运行结果
其次是:《Func<int,bool>》表示传入参数为int类型,返回值为bool类型的委托
1.ParentClass
class ParentClass { public virtual void ParentFunction(int mSum , Func<int,bool> mOnShow) { Console.WriteLine("-----------ParentFuncStart-------"); if(mOnShow != null) Console.WriteLine("-----------ParentFuncBool-------"); Console.WriteLine("-----------ParentFuncEnd-------"); } }
2.ChildrenClass
class ChildrenClass : ParentClass { public override void ParentFunction(int mSum, Func<int,bool> mOnShow) { base.ParentFunction(mSum, mOnShow); Console.WriteLine("-----------ChildrenActionStart-----------"); //可以使用?.Invoke(mSum)简写,,但要注意:如果mOnShow为空的话就会运行时出错。 //if ((bool)mOnShow?.Invoke(mSum)) //{ //Console.WriteLine("-----------ChildrenFuncBool-----------"); //} if (mOnShow != null) { mOnShow(mSum); Console.WriteLine("-----------ChildrenFuncBool-----------"); } //分开用两个if是为了介绍简化委托调用 if (mOnShow == null) { //使用Lambda表达式进行赋值 mOnShow = (sum) =>{return mSum == 10; }; if (!mOnShow(mSum)) { Console.WriteLine("-----------ChildrenFuncBool---mSum != 10--------"); } } Console.WriteLine("-----------ChildrenActionEnd-----------"); } }
3.main
class Program { static void Main(string[] args) { ChildrenClass _children = new ChildrenClass(); string _str = "-----------Main-------------"; int _sum = 5; //使用匿名委托进行赋值 _children.ParentFunction(_sum, null ); } }
4.运行结果
相关文章推荐
- C# 四种委托 Delegate Action Func Predicate 的学习
- 让 C# 委托来帮你简化代码,学习使用 Func、Action 委托
- [C#基础]Func和Action学习
- Unity学习 - C#委托的介绍(delegate、Action、Func、predicate)
- Unity学习 - C#委托的介绍(delegate、Action、Func、predicate)
- C# 学习笔记_委托(二)Action与Func
- C# 参考:令人惊喜的泛型委托 Predicate/Func/Action
- 推荐C#学习之virtual
- C#的delegate/event/Action/Func/Predicate关键字
- C#.NET学习笔记---C#中方法覆盖时override和new的比较,abstract和virtual的比较(含C#多态的实现)
- c#委托Action和Func
- C#基础:委托之Action<T>和Func<T>的用法
- C#基础:委托之Action<T>和Func<T>的用法
- 为LINQ服务的C#新特性总结篇---扩展方法,匿名委托,lambda表达式,Action委托,Func委托,Linq中的order by,top和sum函数
- C#中的三种委托方式:Func委托,Action委托,Predicate委托
- 【KK学习笔记】c# 虚方法 virtual 、 重写方法 override 、new 的使用与理解
- C#基础-Func,Action
- C#的泛型委托Predicate/Func/Action
- c#中Action<T>和Func<T>委托
- C# 参考:令人惊喜的泛型委托 Predicate/Func/Action