Unity3D自学笔记——Photon服务器的后台架构实现(四)
2016-10-28 15:42
555 查看
ALI.ARPG.Domain
实现ICommand, ICommandHandler, IValidateHandler的一系列类
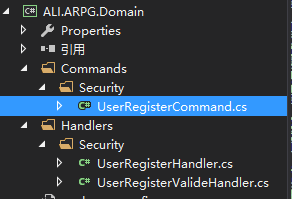
ALI.ARPG.Server
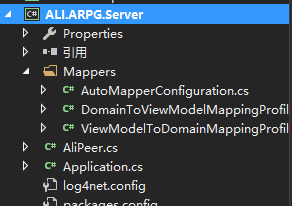
AutoMapper
配置,这里用的是AutoMapper5.0,稍微和前面的版本有些区别
Entity -> Command
Command -> Entity
Application
Photon Server 的Host 类
AliPeer
Photon Server 的Peer类,和客户端进行交互的类
OK 到此,服务端基本框架已完成,还可以加入AOP进行日志处理
实现ICommand, ICommandHandler, IValidateHandler的一系列类
public class UserRegisterCommand : ICommand { public string Name; public string Password; }
public class UserRegisterHandler : ICommandHandler<UserRegisterCommand> { private readonly AccountRepository accountRepository; private UnitOfWork unitOfWork; public UserRegisterHandler() { unitOfWork = new UnitOfWork(); accountRepository = new AccountRepository(unitOfWork.Session); } public ICommandResult Excute(UserRegisterCommand command) { //这里用了AutoMapper,做了 Entity <-->ICommand之间的映射,AutoMapper的配置在Server层 AccountEntity account = Mapper.Map<AccountEntity>(command); accountRepository.Add(account); //通过unitOfWork将数据处理打包执行 unitOfWork.Commit(); return new CommandResult(true); } }
public IEnumerable<ValidationResult> Validate(UserRegisterCommand command) { //TODO 用户注册验证逻辑 //e.g.: //if (string.IsNullOrEmpty(command.Name)) //{ // yield return new ValidationResult("Name", "必须输入用户名"); //} return null; }
ALI.ARPG.Server
AutoMapper
配置,这里用的是AutoMapper5.0,稍微和前面的版本有些区别
public class AutoMapperConfiguration { public static void 4000 Configure() { Mapper.Initialize(x => { x.AddProfile(new ViewModelToDomainMappingProfile()); x.AddProfile(new DomainToViewModelMappingProfile()); }); } }
Entity -> Command
public class DomainToViewModelMappingProfile : Profile { public DomainToViewModelMappingProfile() { this.CreateMap<UserRegisterCommand, AccountEntity>(); } }
Command -> Entity
public class ViewModelToDomainMappingProfile : Profile { public ViewModelToDomainMappingProfile() { this.CreateMap<AccountEntity, UserRegisterCommand>(); } }
Application
Photon Server 的Host 类
public class Application : ApplicationBase { private static readonly ILogger log = ExitGames.Logging.LogManager.GetCurrentClassLogger(); protected override PeerBase CreatePeer(InitRequest initRequest) { return new AliPeer(initRequest.Protocol, initRequest.PhotonPeer); } protected override void Setup() { try { //AutoMapper AutoMapperConfiguration.Configure(); //Log ExitGames.Logging.LogManager.SetLoggerFactory(Log4NetLoggerFactory.Instance); GlobalContext.Properties["Photon:ApplicationLogPath"] = Path.Combine(this.ApplicationRootPath, "log"); GlobalContext.Properties["LogFileName"] = "ALI" + this.ApplicationName; XmlConfigurator.ConfigureAndWatch(new FileInfo(Path.Combine(this.BinaryPath, "log4net.config"))); log.Debug("服务器启动成功"); } catch(Exception ex) { log.Debug(ex.Message); } } protected override void TearDown() { log.Debug("服务器关闭"); } }
AliPeer
Photon Server 的Peer类,和客户端进行交互的类
public class AliPeer : PeerBase { private static readonly ILogger log = ExitGames.Logging.LogManager.GetCurrentClassLogger(); private ICommandBus commandBus; public AliPeer(IRpcProtocol protocol, IPhotonPeer peer) : base(protocol, peer) { commandBus = new CommandBus(); } protected override void OnDisconnect(DisconnectReason reasonCode, string reasonDetail) { } protected override void OnOperationRequest(OperationRequest operationRequest, SendParameters sendParameters) { if (AliPeer.log.IsDebugEnabled) { AliPeer.log.DebugFormat("OnOperationRequest. Code={0}", operationRequest.OperationCode); } //根据OpreationCode 去调度具体的方法 switch (operationRequest.OperationCode) { case (byte)OperationCode.Register: HandleRegisterOperation(operationRequest, sendParameters); break; case (byte)OperationCode.Login: HandleLoginrOperation(operationRequest, sendParameters); break; default: break; } } protected virtual void HandleRegisterOperation(OperationRequest operationRequest, SendParameters sendParameters) { //获取客户端的消息,将Json转化为实体,用到LitJson.dll object json; operationRequest.Parameters.TryGetValue(operationRequest.OperationCode, out json); AccountEntity account = JsonMapper.ToObject<AccountEntity>(json.ToString()); //AutoMapper, Entity -> Command var command = Mapper.Map<AccountEntity, UserRegisterCommand>(account); //通过CommandBus反射调用IValidateHandler, 执行消息验证 IEnumerable<ValidationResult> errors = commandBus.Validate(command); OperationResponse response = new OperationResponse(); if(errors != null && errors.ToList().Count > 0) { response.ReturnCode = (short)ReturnCode.Error; } else { //通过CommandBus反射调用ICommandHandler, 执行Submit var result = commandBus.Submit(command); if (result.Success) { response.ReturnCode = (short)ReturnCode.Sucess; } else { response.ReturnCode = (short)ReturnCode.Faild; } } //返回客户端处理结果 SendOperationResponse(response, sendParameters); } protected virtual void HandleLoginrOperation(OperationRequest operationRequest, SendParameters sendParameters) { } }
OK 到此,服务端基本框架已完成,还可以加入AOP进行日志处理
相关文章推荐
- Unity3D自学笔记——Photon服务器的后台架构实现(三)
- Unity3D自学笔记——Photon服务器的后台架构实现(五)
- Unity3D自学笔记——Photon服务器的后台架构实现(一)
- Unity3D自学笔记——架构应用(二)选择英雄界面
- Unity3D自学笔记——架构应用(六)角色创建
- Unity3D自学笔记——UGUI背包系统(二)UI设计实现及数据绑定
- Unity3D自学笔记——UGUI前台架构
- Unity3D自学笔记——架构应用(八)人物移动与角色相机的跟随
- Unity3D自学笔记——架构应用(四)JsonToEntity帮助类更新
- 实现智能硬件与移动app后台的服务器架构选择
- Unity3D自学笔记——架构应用(一)用户登录
- Unity3D自学笔记——架构应用(七)客户端识别角色是否已经创建
- Unity3D自学笔记——UGUI背包系统(五)沿用Attribute设计实现物品等级
- Unity3D自学笔记——架构应用(五)Loading场景及持久化场景
- Unity3D自学笔记——架构应用(三)JsonToEntity帮助类
- Unity3D自学笔记——实现2D物体漂浮在制定3D物体上方
- 【Linux笔记】在后台执行scp,实现服务器间无密码文件拷贝。
- Unity3D自学笔记——架构应用(十)角色属性
- 一步一步实现企业网络架构之八:利用IIS建立和维护一个WEB服务器 推荐
- 负载均衡--大型在线系统实现的关键(下篇)(服务器集群架构的设计与选择)