Python学习day2作业总结
2016-10-25 20:00
381 查看
为了以后更好更快速的复习,此博客记录我对作业的总结。对于基础作业,我认为最重要的是过程,至于实现是不是完美,代码是不是完美,虽然重要,但是作业过程中,用到的知识点是值得总结和整理的。
一.购物车:
1. 商品信息- 数量、单价、名称2. 用户信息- 帐号、密码、余额
3. 用户可充值
4. 购物历史信息
5. 允许用户多次购买,每次可购买多件
6. 余额不足时进行提醒
7. 用户退出时 ,输出当次购物信息
8. 用户下次登陆时可查看购物历史
9. 商品列表分级显示
思路:
1.此作业是day1作业的结合体;2.用户登录是否首次,分为新用户和旧用户;
3.旧用户查看是否有购物历史;
4.购买时可输入购买数量;
5.余额大于等于购买商品花费则购买成功,购买成功记录购买信息;
6.余额小于购买商品花费则提示充值,要求输入充值金额;
6.购物车自动整理购物商品与花费;
7.可随时查看购物车;
基本流程图:
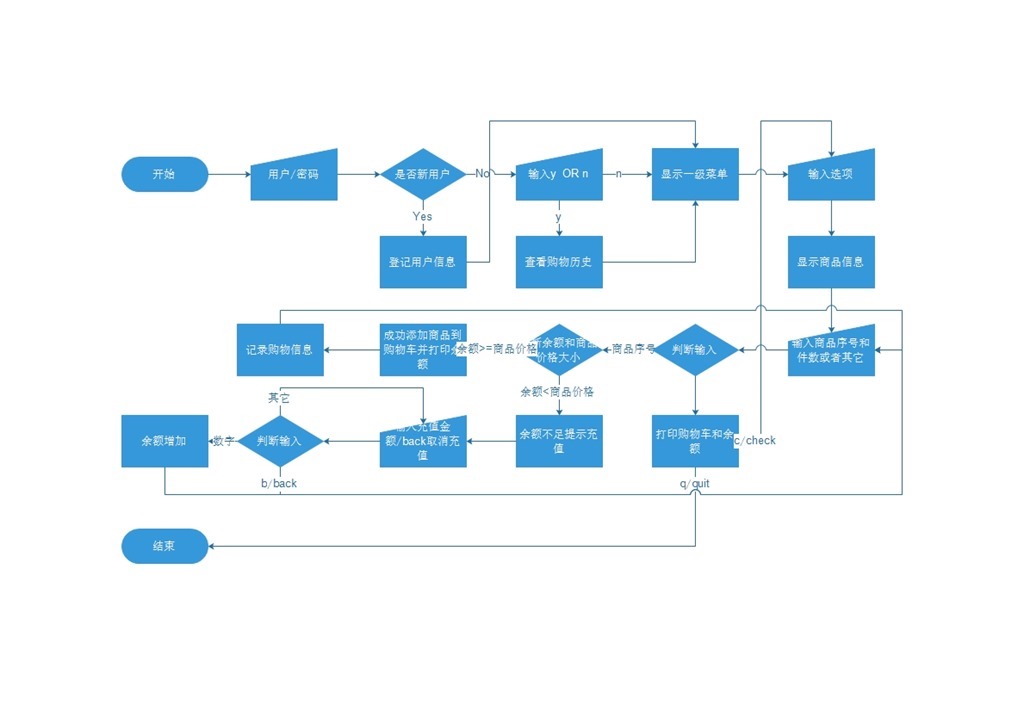
代码:
cat shopping_cart.py
#!/usr/bin/env python # _*_coding:utf-8_*_ ''' * Created on 2016/10/18 22:01. * @author: Chinge_Yang. ''' import os import getpass import datetime menu = { "Mobile phone": [ ("Iphone7", 6188), ("Iphone7 plus", 7888), ("Xiaomi5", 2888) ], "Car": [ ("Audi Q5", 490000), ("Ferrari 488", 4888888) ], "Drink": [ ("Milk", 59), ("Coffee", 30), ("Tea", 311) ] } user_file = "user.txt" shopping_log = "shopping.log" money = 0 #初始金额 all_cost = 0 shopping_cart = {} exit_user_flag = False exit_flag = False exsit_flag = False if not os.path.exists(user_file): f = open(user_file,'w') f.close() if not os.path.exists(shopping_log): f = open(shopping_log,'w') f.close() def show_shopping_cart (): #显示购物车,更新用户信息,exit前使用 #显示购物车信息 print("You purchased products as below".center(50,"*")) print("%-20s %-15s %-10s %-20s" %("Goods","Price","Number","Cost")) for key in shopping_cart: p_name = key[0] p_price = int(key[1]) p_number = int(shopping_cart[key]) print("%-20s %-15s %-10s \033[32;1m%-20s\033[0m" %(p_name,p_price,p_number,p_price*p_number)) print("End".center(50,"*")) print("%-20s %-15s %-10s \033[32;1m%-20s\033[0m" %("You total cost:","","",all_cost)) print("Your balance is [\033[32;1m%s\033[0m]" % money) if new_user is True: # 将新用户信息写入到用户文件中 file = open(user_file, "a") file.write("%s %s %s\n" % (user_name, user_passwd, money)) # 用户、密码、金钱存入用户文件 file.close() else: if old_money != money: #充值或买了东西 # 将旧用户信息更新到用户文件中 old_user_info = "%s %s %s" % (user_name, user_passwd, old_money) new_user_info = "%s %s %s" % (user_name, user_passwd, money) with open(user_file) as file: info = file.read() new_info = info.replace(old_user_info,new_user_info) with open(user_file,"w") as file: file.write(new_info) while exit_user_flag is not True: #输入用户密码 user_name = input("Please input your name:").strip() #user_passwd = input("Please input your password:").strip() user_passwd = getpass.getpass("\033[1;33mPlease input your password:\033[0m") if user_name == '' or user_passwd == '': continue elif ' ' in user_name: print("\033[31;1mNot allowed to contain spaces!\033[0m") continue #查看是否存在于用户数据库 user_check = open(user_file) for l in user_check: l = l.split() user = l[0] passwd = l[1] if user_name == user: #老用户 new_user = False #标记为老用户 money = int(l[2]) #记录用户余额 old_money = money #旧余额 if not passwd == user_passwd: print ("\033[1;31mYour password is error!\033[0m".center(50,"*")) else: print ("\033[1;31mWelcome to go shopping!\033[0m".center(50,"-")) exit_user_flag = True break else: new_user = True #标记为新用户 exit_user_flag = True user_check.close() #关闭 if not new_user: #旧用户 #读取购物历史,判断是否有此用户记录 file = open(shopping_log) for line in file: line = line.split() line_user = line[0] if line_user == user_name: #存在记录 exsit_flag = True break #跳出检测 file.close() if exsit_flag is True: #内容不为空 #只有输入y或者yes才读取显示购物历史,否则不显示 print("Input \033[1;33m[y|yes]\033[0m to view your purchase history,\033[1;33m[others]\033[0m means not.") see_history = input("Please input:").strip() if see_history == "y" or see_history == "yes": # 显示用户购物历史 # output = os.system("grep %s %s" %(user_name,shopping_log)) # print(output.read()) print("User %s shopping history:" % user_name) print("%-20s %-15s %10s %20s" %("Username","datetime","Number","Goods")) file = open(shopping_log) for line in file: line = line.split("\t") line_user = line[0] if line_user == user_name: #存在记录 print("%-10s %-15s %10s %20s" % (line[0],line[1],line[2],line[3].strip())) file.close() else: print("You are not to view your purchase history!") print("-".center(50,"-")) one_layer_list = [] #一级菜单 while True: while True: #打印各类菜单 print("Species list".center(50,"-")) for index, item in enumerate(menu): print("\033[32;1m%d\033[0m --> %s" % (index, item)) one_layer_list.append(item) print("End".center(50,"-")) print("[q|b] to quit") once_choice = input("Input your choice:").strip() if once_choice.isdigit(): #输入数字 once_choice = int(once_choice) if 0 <= once_choice < len(menu): #输入正确数字 print("---->Enter \033[32;1m%s\033[0m" %(one_layer_list[once_choice])) two_layer_list = menu[one_layer_list[once_choice]] exit_flag = False #重新进入二级商品菜单 break #跳出循环,往下走 else: print("\033[31;1mNumber out of range, please enter again!\033[0m") else: if once_choice == "b" or once_choice == "back" or once_choice == "q" or once_choice == "quit": show_shopping_cart() exit("Bye,thanks!".center(50,"#")) print("\033[31;1mPlease enter the Numbers!\033[0m") while exit_flag is not True: #显示二级商品菜单 print("Product list".center(50,'-')) for item in enumerate(two_layer_list): index = item[0] p_name = item[1][0] p_price = item[1][1] print("%s.%-20s %-20s" %(index,p_name,p_price)) print("End".center(50,'-')) print("[q|quit] to quit;[b|back] to back") user_choice = input("Please choice the product:").strip() if user_choice.isdigit(): #输入数字 user_choice = int(user_choice) if 0 <= user_choice < len(two_layer_list): product_number = input("Please input the number of product:").strip() #输入个数 if product_number.isdigit(): product_number = int(product_number) else: continue #重新选择商品和个数 p_item = two_layer_list[user_choice] p_name = p_item[0] #商品名 p_price = int(p_item[1]) #商品价格 new_added = {} if p_price*product_number <= money: #能付款,表示购买成功 new_added = {p_item:product_number} #整理购物车个数显示总数 for k, v in new_added.items(): if k in shopping_cart.keys(): shopping_cart[k] += v else: shopping_cart[k] = v money -= p_price * product_number all_cost += p_price * product_number print("Added [\033[32;1m%d\033[0m] [\033[32;1m%s\033[0m] into shopping cart," "your balance is [\033[32;1m%s\033[0m]" % (product_number,p_name,money)) with open(shopping_log,"a") as file: log = "%s\t\"%s\"\t%d\t\"%s\"\n" %(user_name,datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S'),product_number,p_name) shopping_history = file.write(log) else: #钱不够时,提示充值 print("Your balance is [\033[31;1m%s\033[0m],cannot afford this.." % money) while True: user_charge = input("Do you want to charge more money?[\033[32;1my|n|b]\033[0m").strip() if user_charge == "y" or user_charge == "yes": charge_number = input("Please input your top-up amount:").strip() if charge_number.isdigit(): charge_number = int(charge_number) money += charge_number #充值成功 print("Your balance is [\033[32;1m%s\033[0m]" % money) else: print("Your input is not number!") continue break elif user_charge == "n" or user_charge == "no" or user_charge == "b" or user_charge == "back": break #放弃充值 else: if user_choice == "q" or user_choice == "quit": show_shopping_cart() exit("Bye,thanks!".center(50,"#")) elif user_choice == "c" or user_choice == "check": print("You purchased products as below".center(50,"*")) print("%-20s %-15s %-10s %-20s" %("Goods","Price","Number","Cost")) for key in shopping_cart: p_name = key[0] p_price = int(key[1]) p_number = int(shopping_cart[key]) print("%-20s %-15s %-10s \033[32;1m%-20s\033[0m" %(p_name,p_price,p_number,p_price*p_number)) print("End".center(50,"*")) print("%-20s %-15s %-10s \033[32;1m%-20s\033[0m" %("You total cost:","","",all_cost)) print("Your balance is [\033[32;1m%s\033[0m]" % money) elif user_choice == "b" or user_choice == "back": exit_flag = True else: print("Your input is error!")
总结:
1.用到的基础知识点不少,不仅复习了day1知识,更灵活运用了列表、字典、元组等;2.购物车还可以再完美,比如增加商品列表固定排序(如果使用json,我不知道怎么用),增加余额充值接口(使用信用卡)等;
3.对于复杂一些的需求,层次要分明,条理清晰,才能使自己逻辑思维鲜明;
相关文章推荐
- Python学习day2作业总结
- Python学习day1作业总结
- Python学习day1作业总结
- python学习总结四(python数字)
- python学习总结二(python基础)
- [Python学习]总结一下Cygwin安装与进阶学习列表
- 总结一下最近 Python 学习心得
- Python基本语法学习总结
- python学习总结之数据结构
- Python MySQLdb 学习总结
- python学习总结之外部传参
- Python MySQLdb 学习总结
- 学习Python中,总结一些语法相关注意点
- Python学习笔记总结(二):函数和模块
- Python学习笔记总结(三):类
- python学习总结三(python对象)
- Python学习总结(四)——Pythonic
- Python学习总结(三)——编码规范
- Python学习总结二
- python学习总结五(python序列)