python数据结构学习笔记-2016-10-14-03-二维数组
2016-10-15 00:21
645 查看
2.3 二维数组
二维数组(two-dimensional array):将数据按行和列组织,类似于表格和网格。
2.3.1 二维数组ADT
二维数组ADT包含的方法与一维数组的有些类似:
Array2D(nrows, ncols):创建二维数组,行数和列数分别是nrows和ncols,并将所有元素初始化为None;
numRows():返回二维数组的行数;
numCols():返回二维数组的列数;
clear(value):将二维数组的所有元素都设定为value;
getitem(i, j):返回二维数组中行数为i,列数为j的元素,其中(i, j)是以一个二元元组的参数传入;
setitem(i, j, value):将二维数组中行数为i,列数为j的元素,重新设定为value。
应用:
2.3.2 实现二维数组
二维数组的实现,有两种普遍的方式,一种是单纯使用一维数组,另外一种是使用数组的数组,书中采用的是后一种。其具体阐述如下,使用一个一维数组,其内的每个元素都储存了一个引用,指向一个数组,储存引用的数组的长度就是二维数组的行数,而引用所指向的数组的长度就是二维数组的列数。
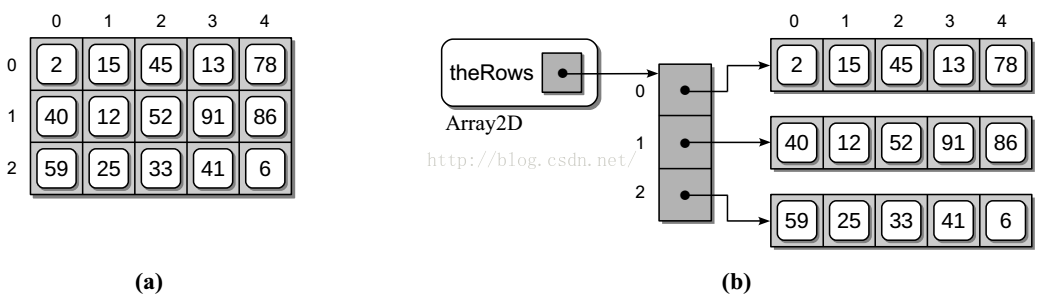
#-*-coding: utf-8-*-
# 二维数组的实现
from myarray import Array
class Array2D(object):
def __init__(self, numRows, numCols):
# 创建一维数组,用于储存对每行数组的引用
self._theRows = Array(numRows)
# 创建一维数组,用于储存二维数组的每一行数据
for i in range(numRows):
self._theRows[i] = Array(numCols)
def numRows(self):
return len(self._theRows)
def numCols(self):
return len(self._theRows[0])
def clear(self, value):
for row in range(self.numRows()):
self._theRows[row].clear(value)
def __getitem__(self, ndxTuple):
assert len(ndxTuple) == 2, "Invalid number of array subscripts." # 保证传入含两个数的元组
row = ndxTuple[0]
col = ndxTuple[1]
assert 0 <= row < self.numRows() and 0 <= col < self.numCols(), "Array subscript out of range."
the1dArray = self._theRows[row] # 不理解为何要使用局部变量the1dArray,而不是直接self._theRows[row][col]?
return the1dArray[col]
def __setitem__(self, ndxTuple, value):
assert len(ndxTuple) == 2, "Invalid number of array subscripts." # 保证传入含两个数的元组
row = ndxTuple[0]
col = ndxTuple[1]
assert 0 <= row < self.numRows() and 0 <= col < self.numCols(), "Array subscript out of range."
the1dArray = self._theRows[row]
the1dArray[col] = value
要注意的是,在这一代码中,访问二维数组的元素是通过一个二元元组来访问的,即通过x[i, j]来访问,而不能通过x[i][j]来访问。
二维数组(two-dimensional array):将数据按行和列组织,类似于表格和网格。
2.3.1 二维数组ADT
二维数组ADT包含的方法与一维数组的有些类似:
Array2D(nrows, ncols):创建二维数组,行数和列数分别是nrows和ncols,并将所有元素初始化为None;
numRows():返回二维数组的行数;
numCols():返回二维数组的列数;
clear(value):将二维数组的所有元素都设定为value;
getitem(i, j):返回二维数组中行数为i,列数为j的元素,其中(i, j)是以一个二元元组的参数传入;
setitem(i, j, value):将二维数组中行数为i,列数为j的元素,重新设定为value。
应用:
#-*-coding: utf-8-*- # 用二维数组储存学生考试成绩,并计算每位学生的平均成绩 from myarray2d import Array2D gradeFile = open(filename, 'r') numExams = int(gradeFile.readline()) numStudents = int(gradeFile.readline()) examGrades = Array2D(numStudents, numExams) i = 0 for student in gradeFile: grades = student.split() for j in range(numExams): examGrades[i, j] = int(grades[j]) i += 1 gradeFile.close() for i in range(numStudents): total = 0 for j in range(numExams): total += examGrades[i, j] examAvg = 1.0 * total / numExams print "{0:0>2}: {1:.6f}".format(i+1, examAvg)
2.3.2 实现二维数组
二维数组的实现,有两种普遍的方式,一种是单纯使用一维数组,另外一种是使用数组的数组,书中采用的是后一种。其具体阐述如下,使用一个一维数组,其内的每个元素都储存了一个引用,指向一个数组,储存引用的数组的长度就是二维数组的行数,而引用所指向的数组的长度就是二维数组的列数。
#-*-coding: utf-8-*-
# 二维数组的实现
from myarray import Array
class Array2D(object):
def __init__(self, numRows, numCols):
# 创建一维数组,用于储存对每行数组的引用
self._theRows = Array(numRows)
# 创建一维数组,用于储存二维数组的每一行数据
for i in range(numRows):
self._theRows[i] = Array(numCols)
def numRows(self):
return len(self._theRows)
def numCols(self):
return len(self._theRows[0])
def clear(self, value):
for row in range(self.numRows()):
self._theRows[row].clear(value)
def __getitem__(self, ndxTuple):
assert len(ndxTuple) == 2, "Invalid number of array subscripts." # 保证传入含两个数的元组
row = ndxTuple[0]
col = ndxTuple[1]
assert 0 <= row < self.numRows() and 0 <= col < self.numCols(), "Array subscript out of range."
the1dArray = self._theRows[row] # 不理解为何要使用局部变量the1dArray,而不是直接self._theRows[row][col]?
return the1dArray[col]
def __setitem__(self, ndxTuple, value):
assert len(ndxTuple) == 2, "Invalid number of array subscripts." # 保证传入含两个数的元组
row = ndxTuple[0]
col = ndxTuple[1]
assert 0 <= row < self.numRows() and 0 <= col < self.numCols(), "Array subscript out of range."
the1dArray = self._theRows[row]
the1dArray[col] = value
要注意的是,在这一代码中,访问二维数组的元素是通过一个二元元组来访问的,即通过x[i, j]来访问,而不能通过x[i][j]来访问。
相关文章推荐
- python数据结构学习笔记-2016-11-07-03-多重链表以及相应的迭代器
- python数据结构学习笔记-2016-10-17-03-多维数组
- python数据结构学习笔记-2016-10-22-03-稀疏矩阵
- python数据结构学习笔记-2016-10-05-03-抽象数据类型(三)
- python数据结构学习笔记-2016-10-28-03-用链表实现多项式ADT
- python数据结构学习笔记-2016-10-14-02-python列表
- Python数据结构--Python学习笔记四
- Python 2.7.11 基本语法和数据结构学习笔记
- python学习笔记(03):函数
- 学习 严蔚敏讲数据结构笔记03
- python 学习笔记(03)
- Python学习笔记03 控制流语句和函数
- 深度学习(DL)与卷积神经网络(CNN)学习笔记随笔-03-基于Python的LeNet之LR
- Python学习笔记 03 Python对象
- 深度学习(DL)与卷积神经网络(CNN)学习笔记随笔-03-基于Python的LeNet之LR
- python数据结构学习笔记-2-算法
- 【学习笔记----数据结构03--线性链表】
- Python学习笔记——基本数据结构
- 学习python笔记——数据结构
- Python 学习笔记 3 数据结构(3.1简要介绍)