iOS 获取健康里的信息(步数和公里数)
2016-10-11 21:15
239 查看
//第一步
打开项目--点击capabilities 将HealthKit 打开 如下图
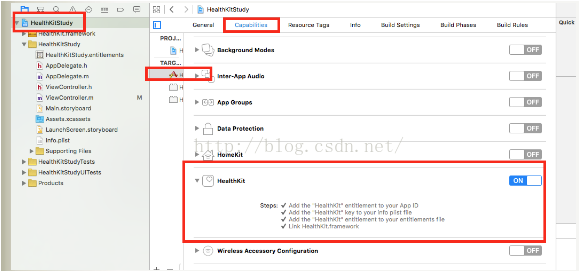
//第二步
1.首先新建一个类 -- 继承NSObject
在.h中
#define HKVersion [[[UIDevice currentDevice] systemVersion] doubleValue]
#define CustomHealthErrorDomain @"com.sdqt.healthError"
@interface HealthKitManage : NSObject
@property (nonatomic, strong) HKHealthStore *healthStore;
+(id)shareInstance;
- (void)authorizeHealthKit:(void(^)(BOOL success, NSError *error))compltion;
- (void)getDistance:(void(^)(double value, NSError *error))completion;
- (void)getStepCount:(void(^)(double value, NSError *error))completion;
2.在.m中
+(id)shareInstance
{
static id manager ;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
manager = [[[self class] alloc] init];
});
return manager;
}
- (void)authorizeHealthKit:(void(^)(BOOL success, NSError *error))compltion
{
if(HKVersion >= 8.0)
{
if (![HKHealthStore isHealthDataAvailable]) {
NSError *error = [NSError errorWithDomain: @"com.raywenderlich.tutorials.healthkit" code: 2 userInfo: [NSDictionary dictionaryWithObject:@"HealthKit is not available in th is Device" forKey:NSLocalizedDescriptionKey]];
if (compltion != nil) {
compltion(false, error);
}
return;
}
if ([HKHealthStore isHealthDataAvailable]) {
if(self.healthStore == nil)
self.healthStore = [[HKHealthStore alloc] init];
NSSet *writeDataTypes = [self dataTypesToWrite];
NSSet *readDataTypes = [self dataTypesRead];
[self.healthStore requestAuthorizationToShareTypes:writeDataTypes readTypes:readDataTypes completion:^(BOOL success, NSError *error) {
if (compltion != nil) {
compltion (success, error);
}
}];
}
else {
NSDictionary *userInfo = [NSDictionary dictionaryWithObject:@"iOS 系统低于8.0" forKey:NSLocalizedDescriptionKey];
NSError *aError = [NSError errorWithDomain:CustomHealthErrorDomain code:0 userInfo:userInfo];
compltion(0,aError);
}
}
- (NSSet *)dataTypesToWrite
{
HKQuantityType *heightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierHeight];
HKQuantityType *weightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyMass];
HKQuantityType *temperatureType = [HKQuantityType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyTemperature];
HKQuantityType *activeEnergyType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierActiveEnergyBurned];
return [NSSet setWithObjects:heightType, temperatureType, weightType,activeEnergyType,nil];
}
- (NSSet *)dataTypesRead
{
HKQuantityType *heightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierHeight];
HKQuantityType *weightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyMass];
HKQuantityType *temperatureType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyTemperature];
HKCharacteristicType *birthdayType = [HKObjectType characteristicTypeForIdentifier:HKCharacteristicTypeIdentifierDateOfBirth];
HKCharacteristicType *sexType = [HKObjectType characteristicTypeForIdentifier:HKCharacteristicTypeIdentifierBiologicalSex];
HKQuantityType *stepCountType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierStepCount];
HKQuantityType *distance = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierDistanceWalkingRunning];
HKQuantityType *activeEnergyType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierActiveEnergyBurned];
return [NSSet setWithObjects:heightType, temperatureType,birthdayType,sexType,weightType,stepCountType, distance, activeEnergyType,nil];
}
- (void)getStepCount:(void(^)(double value, NSError *error))completion
{
HKQuantityType *stepType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierStepCount];
NSSortDescriptor *timeSortDescriptor = [[NSSortDescriptor alloc] initWithKey:HKSampleSortIdentifierEndDate ascending:NO];
HKSampleQuery *query = [[HKSampleQuery alloc] initWithSampleType:stepType predicate:[HealthKitManage predicateForSamplesToday] limit:HKObjectQueryNoLimit sortDescriptors:@[timeSortDescriptor] resultsHandler:^(HKSampleQuery *query, NSArray *results, NSError *error) {
if(error)
{
completion(0,error);
}
else
{
NSInteger totleSteps = 0;
for(HKQuantitySample *quantitySample in results)
{
HKQuantity *quantity = quantitySample.quantity;
HKUnit *heightUnit = [HKUnit countUnit];
double usersHeight = [quantity doubleValueForUnit:heightUnit];
totleSteps += usersHeight;
}
completion(totleSteps,error);
}
}];
[self.healthStore executeQuery:query];
}
+ (NSPredicate *)predicateForSamplesToday {
NSCalendar *calendar = [NSCalendar currentCalendar];
NSDate *now = [NSDate date];
NSDateComponents *components = [calendar components:NSCalendarUnitYear|NSCalendarUnitMonth|NSCalendarUnitDay fromDate:now];
[components setHour:0];
[components setMinute:0];
[components setSecond: 0];
NSDate *startDate = [calendar dateFromComponents:components];
NSDate *endDate = [calendar dateByAddingUnit:NSCalendarUnitDay value:1 toDate:startDate options:0];
NSPredicate *predicate = [HKQuery predicateForSamplesWithStartDate:startDate endDate:endDate options:HKQueryOptionNone];
return predicate;
}
//获取公里数
- (void)getDistance:(void(^)(double value, NSError *error))completion
{
HKQuantityType *distanceType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierDistanceWalkingRunning];
NSSortDescriptor *timeSortDescriptor = [[NSSortDescriptor alloc] initWithKey:HKSampleSortIdentifierEndDate ascending:NO];
HKSampleQuery *query = [[HKSampleQuery alloc] initWithSampleType:distanceType predicate:[HealthKitManage predicateForSamplesToday] limit:HKObjectQueryNoLimit sortDescriptors:@[timeSortDescriptor] resultsHandler:^(HKSampleQuery * _Nonnull query, NSArray<</span>__kindof HKSample *> * _Nullable results, NSError * _Nullable error) {
if(error)
{
completion(0,error);
}
else
{
double totleSteps = 0;
for(HKQuantitySample *quantitySample in results)
{
HKQuantity *quantity = quantitySample.quantity;
HKUnit *distanceUnit = [HKUnit meterUnitWithMetricPrefix:HKMetricPrefixKilo];
double usersHeight = [quantity doubleValueForUnit:distanceUnit];
totleSteps += usersHeight;
}
completion(totleSteps,error);
}
}];
4000
[self.healthStore executeQuery:query];
}
//3.调用单例 -- 在你需要的地方调用两个方法
1.获取每日步数的方法
- (void)onClickBtn1
{
HealthKitManage *manage = [HealthKitManage shareInstance];
[manage authorizeHealthKit:^(BOOL success, NSError *error) {
if (success) {
[manage getStepCount:^(double value, NSError *error) {
dispatch_async(dispatch_get_main_queue(), ^{
//在此处可以获取步数 ---- value
nslog(@"%f",value);
});
}];
}
else {
NSLog(@"fail");
}
}];
}
2.获取公里数的方法
- (void)onClickBtn2
{
HealthKitManage *manage = [HealthKitManage shareInstance];
[manage authorizeHealthKit:^(BOOL success, NSError *error) {
if (success) {
[manage getDistance:^(double value, NSError *error) {
dispatch_async(dispatch_get_main_queue(), ^{
获得公里数 : value
});
}];
}
else {
NSLog(@"fail");
}
}];
}
//4.在你需要调用的viewcontroll里面的viewDidload里面调用方法,然后在block里面去获取数据
[self onClickBtn1];
[self onClickBtn2];
打开项目--点击capabilities 将HealthKit 打开 如下图
//第二步
1.首先新建一个类 -- 继承NSObject
在.h中
#define HKVersion [[[UIDevice currentDevice] systemVersion] doubleValue]
#define CustomHealthErrorDomain @"com.sdqt.healthError"
@interface HealthKitManage : NSObject
@property (nonatomic, strong) HKHealthStore *healthStore;
+(id)shareInstance;
- (void)authorizeHealthKit:(void(^)(BOOL success, NSError *error))compltion;
- (void)getDistance:(void(^)(double value, NSError *error))completion;
- (void)getStepCount:(void(^)(double value, NSError *error))completion;
2.在.m中
+(id)shareInstance
{
static id manager ;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
manager = [[[self class] alloc] init];
});
return manager;
}
- (void)authorizeHealthKit:(void(^)(BOOL success, NSError *error))compltion
{
if(HKVersion >= 8.0)
{
if (![HKHealthStore isHealthDataAvailable]) {
NSError *error = [NSError errorWithDomain: @"com.raywenderlich.tutorials.healthkit" code: 2 userInfo: [NSDictionary dictionaryWithObject:@"HealthKit is not available in th is Device" forKey:NSLocalizedDescriptionKey]];
if (compltion != nil) {
compltion(false, error);
}
return;
}
if ([HKHealthStore isHealthDataAvailable]) {
if(self.healthStore == nil)
self.healthStore = [[HKHealthStore alloc] init];
NSSet *writeDataTypes = [self dataTypesToWrite];
NSSet *readDataTypes = [self dataTypesRead];
[self.healthStore requestAuthorizationToShareTypes:writeDataTypes readTypes:readDataTypes completion:^(BOOL success, NSError *error) {
if (compltion != nil) {
compltion (success, error);
}
}];
}
else {
NSDictionary *userInfo = [NSDictionary dictionaryWithObject:@"iOS 系统低于8.0" forKey:NSLocalizedDescriptionKey];
NSError *aError = [NSError errorWithDomain:CustomHealthErrorDomain code:0 userInfo:userInfo];
compltion(0,aError);
}
}
- (NSSet *)dataTypesToWrite
{
HKQuantityType *heightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierHeight];
HKQuantityType *weightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyMass];
HKQuantityType *temperatureType = [HKQuantityType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyTemperature];
HKQuantityType *activeEnergyType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierActiveEnergyBurned];
return [NSSet setWithObjects:heightType, temperatureType, weightType,activeEnergyType,nil];
}
- (NSSet *)dataTypesRead
{
HKQuantityType *heightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierHeight];
HKQuantityType *weightType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyMass];
HKQuantityType *temperatureType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierBodyTemperature];
HKCharacteristicType *birthdayType = [HKObjectType characteristicTypeForIdentifier:HKCharacteristicTypeIdentifierDateOfBirth];
HKCharacteristicType *sexType = [HKObjectType characteristicTypeForIdentifier:HKCharacteristicTypeIdentifierBiologicalSex];
HKQuantityType *stepCountType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierStepCount];
HKQuantityType *distance = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierDistanceWalkingRunning];
HKQuantityType *activeEnergyType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierActiveEnergyBurned];
return [NSSet setWithObjects:heightType, temperatureType,birthdayType,sexType,weightType,stepCountType, distance, activeEnergyType,nil];
}
- (void)getStepCount:(void(^)(double value, NSError *error))completion
{
HKQuantityType *stepType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierStepCount];
NSSortDescriptor *timeSortDescriptor = [[NSSortDescriptor alloc] initWithKey:HKSampleSortIdentifierEndDate ascending:NO];
HKSampleQuery *query = [[HKSampleQuery alloc] initWithSampleType:stepType predicate:[HealthKitManage predicateForSamplesToday] limit:HKObjectQueryNoLimit sortDescriptors:@[timeSortDescriptor] resultsHandler:^(HKSampleQuery *query, NSArray *results, NSError *error) {
if(error)
{
completion(0,error);
}
else
{
NSInteger totleSteps = 0;
for(HKQuantitySample *quantitySample in results)
{
HKQuantity *quantity = quantitySample.quantity;
HKUnit *heightUnit = [HKUnit countUnit];
double usersHeight = [quantity doubleValueForUnit:heightUnit];
totleSteps += usersHeight;
}
completion(totleSteps,error);
}
}];
[self.healthStore executeQuery:query];
}
+ (NSPredicate *)predicateForSamplesToday {
NSCalendar *calendar = [NSCalendar currentCalendar];
NSDate *now = [NSDate date];
NSDateComponents *components = [calendar components:NSCalendarUnitYear|NSCalendarUnitMonth|NSCalendarUnitDay fromDate:now];
[components setHour:0];
[components setMinute:0];
[components setSecond: 0];
NSDate *startDate = [calendar dateFromComponents:components];
NSDate *endDate = [calendar dateByAddingUnit:NSCalendarUnitDay value:1 toDate:startDate options:0];
NSPredicate *predicate = [HKQuery predicateForSamplesWithStartDate:startDate endDate:endDate options:HKQueryOptionNone];
return predicate;
}
//获取公里数
- (void)getDistance:(void(^)(double value, NSError *error))completion
{
HKQuantityType *distanceType = [HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierDistanceWalkingRunning];
NSSortDescriptor *timeSortDescriptor = [[NSSortDescriptor alloc] initWithKey:HKSampleSortIdentifierEndDate ascending:NO];
HKSampleQuery *query = [[HKSampleQuery alloc] initWithSampleType:distanceType predicate:[HealthKitManage predicateForSamplesToday] limit:HKObjectQueryNoLimit sortDescriptors:@[timeSortDescriptor] resultsHandler:^(HKSampleQuery * _Nonnull query, NSArray<</span>__kindof HKSample *> * _Nullable results, NSError * _Nullable error) {
if(error)
{
completion(0,error);
}
else
{
double totleSteps = 0;
for(HKQuantitySample *quantitySample in results)
{
HKQuantity *quantity = quantitySample.quantity;
HKUnit *distanceUnit = [HKUnit meterUnitWithMetricPrefix:HKMetricPrefixKilo];
double usersHeight = [quantity doubleValueForUnit:distanceUnit];
totleSteps += usersHeight;
}
completion(totleSteps,error);
}
}];
4000
[self.healthStore executeQuery:query];
}
//3.调用单例 -- 在你需要的地方调用两个方法
1.获取每日步数的方法
- (void)onClickBtn1
{
HealthKitManage *manage = [HealthKitManage shareInstance];
[manage authorizeHealthKit:^(BOOL success, NSError *error) {
if (success) {
[manage getStepCount:^(double value, NSError *error) {
dispatch_async(dispatch_get_main_queue(), ^{
//在此处可以获取步数 ---- value
nslog(@"%f",value);
});
}];
}
else {
NSLog(@"fail");
}
}];
}
2.获取公里数的方法
- (void)onClickBtn2
{
HealthKitManage *manage = [HealthKitManage shareInstance];
[manage authorizeHealthKit:^(BOOL success, NSError *error) {
if (success) {
[manage getDistance:^(double value, NSError *error) {
dispatch_async(dispatch_get_main_queue(), ^{
获得公里数 : value
});
}];
}
else {
NSLog(@"fail");
}
}];
}
//4.在你需要调用的viewcontroll里面的viewDidload里面调用方法,然后在block里面去获取数据
[self onClickBtn1];
[self onClickBtn2];
相关文章推荐
- iOS 获取步数等健康信息
- iOS利用HealthKit框架从健康app中获取步数信息
- iOS利用HealthKit框架从健康app中获取步数信息
- iOS利用HealthKit框架从健康app中获取步数信息
- iOS利用HealthKit框架从健康app中获取步数信息
- iOS利用HealthKit框架从健康app中获取步数信息
- iOS利用HealthKit获取健康里的步数和睡眠时间
- iOS利用HealthKit框架从健康app中获取步数信息
- iOS开发——获取UIWebView中视频的长度与播放进度等信息
- iOS App获取编译时间信息
- IOS成长之路-获取设备的信息
- 获取当前ios系统的版本信息
- ios获取设备信息方法
- 获取iOS系统的相关信息
- 尽可能多的获取ios设备的信息
- 获取ios 系统 硬件信息
- ios 获取运营商的相关信息
- 获取ios应用的app相关信息
- 在IOS开发中根据(id)sender获取UIButton的信息
- ios开发之获取手机通讯录中所有联系人的信息二