SSH-spring3.2与struts2.0整合小例
2016-09-30 11:41
549 查看
spring与struts整合
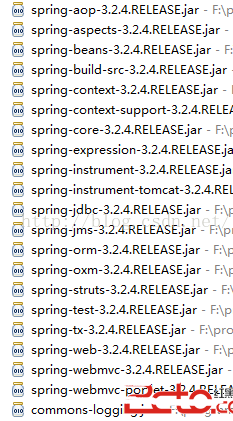
struts2-spring-plugin-2.1.8.jar是struts2、spring整合的关键!
SSH所需包下载地址:
spring包下载:http://repo.springsource.org/libs-release-local/org/springframework/spring/ 点击打开链接
struts包下载:http://struts.apache.org/download.cgi 点击打开链接
hibernate包下载:http://sourceforge.mirrorservice.org/h/project/hi/hibernate/ 点击打开链接
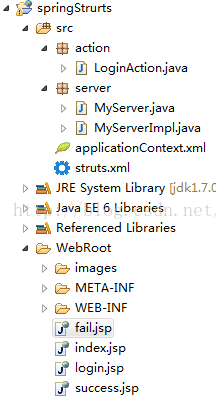
四 程序部分
1.定义一个登录验证接口
1.spring配置文件。在src目录下新建一个applicationContext.xml文件,配置如下:
2.struts配置文件。在src目录下新建struts.xml文件,配置如下
3.修改wel.xml文件
applicaContext.xml的路径是可以变更的,只要在<param-value>那里配上它的路径即可。
4.webRoot目录下新建login.jsp、success.jsp和fail.jsp
(1)login.jsp
一 新建web Project
二 导入spring与struts所需包
struts2-spring-plugin-2.1.8.jar是struts2、spring整合的关键!
SSH所需包下载地址:
spring包下载:http://repo.springsource.org/libs-release-local/org/springframework/spring/ 点击打开链接
struts包下载:http://struts.apache.org/download.cgi 点击打开链接
hibernate包下载:http://sourceforge.mirrorservice.org/h/project/hi/hibernate/ 点击打开链接
三 工程目录
四 程序部分
1.定义一个登录验证接口
package server; public interface MyServer { public abstract boolean LoginValid(String name,String password); }2.实现MyServer接口
package server; public class MyServerImpl implements MyServer{ @Override public boolean LoginValid(String username, String password) { if(username.equals("city") && password.equals("123")){ return true; } return false; } }3.业务代码LoginAction,演示登陆
package action; import com.opensymphony.xwork2.Action; import server.MyServer; public class LoginAction implements Action{ private String username; //与login.jsp页面上保持一致,否则接受不到报空 private String password; private MyServer ms; private String tip; //在jsp页面展示出来 public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public MyServer getMs() { return ms; } public void setMs(MyServer ms) { this.ms = ms; } public String getTip() { return tip; } public void setTip(String tip) { this.tip = tip; } @Override public String execute() throws Exception { if(ms.LoginValid(getUsername(), getPassword())){ setTip("success access!"); return "success"; } return "fail"; } }五 配置文件
1.spring配置文件。在src目录下新建一个applicationContext.xml文件,配置如下:
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd"> <bean name="myServer" class="server.MyServerImpl"></bean> <bean name="loginAction" class="action.LoginAction" scope="prototype"> <property name="ms" ref="myServer"></property> </bean> </beans>依赖注入:MyServerImpl的对象引用myServer被注入给LoginAction类的属性ms,这样ms就可以使用myServer的方法。
2.struts配置文件。在src目录下新建struts.xml文件,配置如下
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <!-- 将Action的创建交给spring来管理 --> <constant name="struts.objectFactory" value="spring" /> <package name="login" extends="struts-default"> <action name="loginPro" class="loginAction"> <result name="success">/success.jsp</result> <result name="fail">/fail.jsp</result> </action> </package> </struts>注意struts.xml文件中action的class属性,不是一个类,而是spring配置中bean的id,属性由spring来注入!
3.修改wel.xml文件
<?xml version="1.0" encoding="UTF-8"?> <web-app version="3.0" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"> <display-name></display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <!--配置applicaContext.xml的路径--> <context-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:applicationContext.xml</param-value> </context-param> <filter> <filter-name>struts2</filter-name> <filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> </web-app>
applicaContext.xml的路径是可以变更的,只要在<param-value>那里配上它的路径即可。
4.webRoot目录下新建login.jsp、success.jsp和fail.jsp
(1)login.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="GB18030"%> <%@ taglib prefix="s" uri="/struts-tags" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>登陆</title> </head> <body> <form action="loginPro" method="post"> <table> <caption>用户登录</caption> <tr> <td>用户名:</td> <td><input type="text" name="username"/></td> </tr> <tr> <td>密码:</td> <td><input type="text" name="password"/></td> </tr> <tr> <td><input value="提交" type="submit"/></td> <td><input value="重置" type="reset"/></td> </tr> </table> </form> </body> </html>(2)success.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="GB18030"%> <%@ taglib prefix="s" uri="/struts-tags" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <b>这是欢迎页面。</b><br> <s:property value="tip" /> </body> </html>(3)fail.sjsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="GB18030"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 操作失败!<br> <img src="images/404.jpg" alt="404" style="position:absolute; left:300px; top:100px; "></img> </body> </html>
相关文章推荐
- Struts 2.0 + Hibernate 3.2 + Spring 2.0 框架整合
- s2sh整合实例 Struts2.0 Spring2.6 Hibernate3.2
- 关于SSH整合, Struts2.3,Hibernate3,Spring3.2
- Struts 2.0 + Spring 2 + Hibernate 3 整合(含源代码下载)
- Struts 2 + Spring 2.0 + Hibernate 3.0整合笔记
- SSH:SSH(Struts1 + Spring + hibernate)整合之配置文件
- Struts2+Hibernate3.2+Spring 2.0整合应用
- Struts 2 + Spring 2.0 + Hibernate 3.0整合笔记
- 使用MyEclipse集成SSH和DWR(一)整合Spring和Struts
- Struts+Spring+Hibernate--SSH整合实例
- 一个简单Spring+Hibernate+Struts2.0+Ajax整合获取客户端IP、URL和请求时间
- Struts Spring Hibernate (SSH) 整合实例
- Struts+Spring+Hibernate/SSH整合开发详细二
- struts1.2+spring2.0+hibernate3.2 整合的小示例
- Spring2.5、Struts2.1、Hibernate3.2、sitemesh、freemarker整合开发常见问题及解决方案
- Struts1.2+Spring2.0+Hibernate3.1整合总结 MyEclipse5.5
- Struts 2 + Spring 2.0 + Hibernate 3.0整合笔记
- 基于SSH(Struts2.0+Spring2.5+Hibernate3)的框架构建(2)
- MyEclipse整合SSH(Struts+Spring+Hibernate)简单登录范例(一)
- Struts+Spring+Hibernate--SSH整合实例