Swagger-UI配置使用
2016-09-12 00:00
309 查看
摘要: Swagger-UI配置使用
Swagger-ui是一个用于提供restful接口api测试的工具,结合Springcloud引入后,可以部分代替像postman等
测试rest接口的工具。而且具有实时性,与其他系统对接时具有极大的方便性。
1、引入依赖
pom.xml
2、注入核心加载bean,根据注解在项目启动后会自动加载
SwaggerConfig.java
3、controller注解暴露
CustomerController.java
4、查看restful Api接口在线演示
地址为http://IP地址端口号/swagger-ui.html
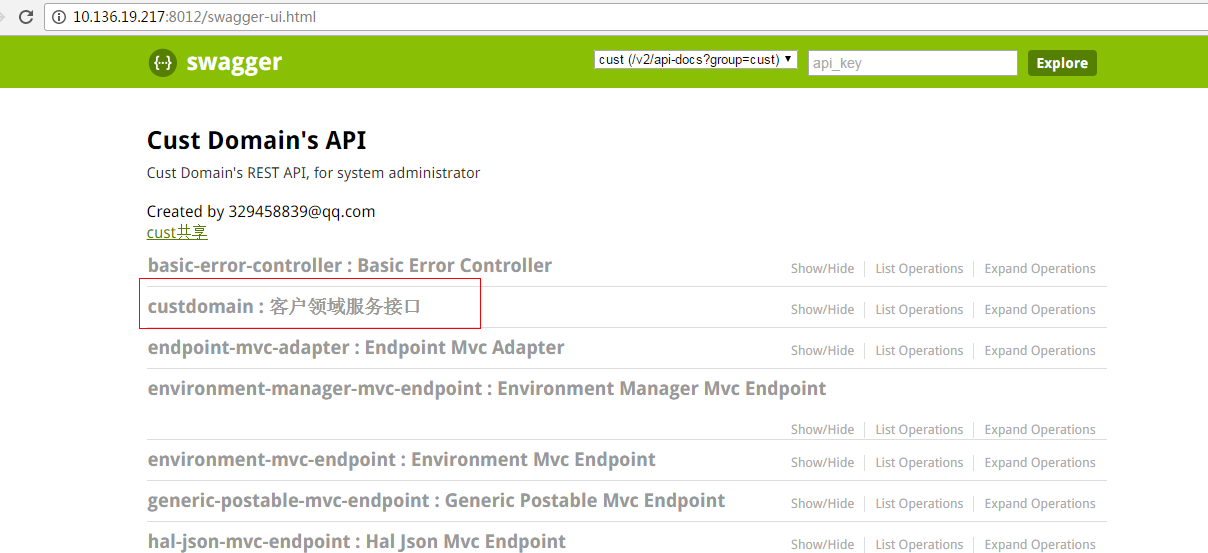
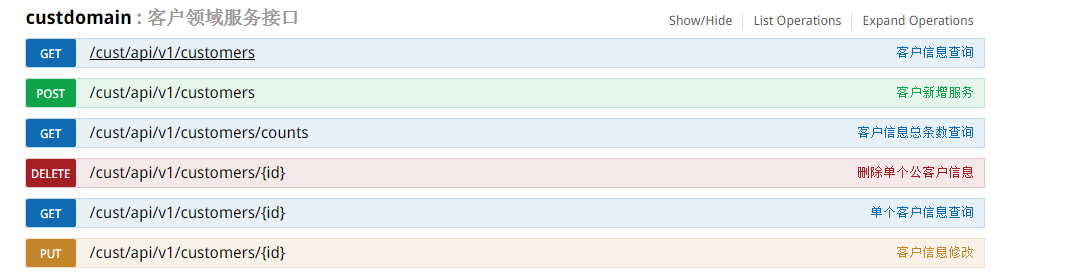
Swagger-ui是一个用于提供restful接口api测试的工具,结合Springcloud引入后,可以部分代替像postman等
测试rest接口的工具。而且具有实时性,与其他系统对接时具有极大的方便性。
1、引入依赖
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.bincai.cloud</groupId> <artifactId>cloud-cust-domain</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>cloud-cust-domain</name> <url>http://maven.apache.org</url> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.3.5.RELEASE</version> </parent> <properties> <start-class>com.taikang.boot.UserApplication</start-class> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> </properties> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Brixton.SR3</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <!-- eureka依赖 --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> </dependency> <!-- 配置 --> <dependency> <groupId>org.springframework.cloud</groupId> 7fe0 <artifactId>spring-cloud-starter-config</artifactId> </dependency> <!-- hystrix依赖 --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-hystrix</artifactId> </dependency> <!-- Swagger --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.2.2</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.2.2</version> </dependency> <!-- ribbon依赖 --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-ribbon</artifactId> </dependency> <!-- sleuth依赖 --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-sleuth</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-stream-rabbit</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-sleuth-stream</artifactId> </dependency> <!-- mybatis依赖 --> <dependency> <groupId>com.zaxxer</groupId> <artifactId>HikariCP</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.1.1</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <!-- json start --> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.8</version> </dependency> <!-- json end --> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project> |
SwaggerConfig.java
package com.bincai.cloud.cust.domain.common; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.web.context.request.async.DeferredResult; import springfox.documentation.service.ApiInfo; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket; import springfox.documentation.swagger2.annotations.EnableSwagger2; @Configuration @EnableSwagger2 public class SwaggerConfig { /** * 可以定义多个组,比如本类中定义把test和demo区分开了 * (访问页面就可以看到效果了) * */ @Bean public Docket demoApi() { return new Docket(DocumentationType.SWAGGER_2) .groupName("cust") .genericModelSubstitutes(DeferredResult.class) .useDefaultResponseMessages(false) .forCodeGeneration(false) .pathMapping("/") .select() // .paths("/demo/.*"))//过滤的接口 .build() .apiInfo(demoApiInfo()); } private ApiInfo demoApiInfo() { ApiInfo apiInfo = new ApiInfo("Cust Domain's API",//大标题 "Cust Domain's REST API, for system administrator",//小标题 "1.0",//版本 "NO terms of service", "329458839@qq.com",//作者 "cust共享",//链接显示文字 "https://github.com/zhbxzc/cust"//网站链接 ); return apiInfo; } } |
CustomerController.java
package com.bincai.cloud.cust.domain.controller; import java.io.UnsupportedEncodingException; import java.util.List; import java.util.UUID; import javax.annotation.Resource; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.JSONArray; import com.alibaba.fastjson.JSONObject; import com.bincai.cloud.cust.domain.model.Customer; import com.bincai.cloud.cust.domain.service.CustService; import com.netflix.hystrix.contrib.javanica.annotation.HystrixCommand; import io.swagger.annotations.Api; import io.swagger.annotations.ApiImplicitParam; import io.swagger.annotations.ApiImplicitParams; import io.swagger.annotations.ApiOperation; @RestController @RequestMapping("/cust") @Api(value="custdomain",description="客户领域服务接口") public class CustomerController { @Resource private CustService custService; @ApiOperation(value="客户新增服务",notes="客户新增服务接口") @ApiImplicitParam(name="customer",value="客户数据对象JSON",required=true,paramType="body") @RequestMapping(value="/api/v1/customers",method=RequestMethod.POST) @HystrixCommand(fallbackMethod = "hystrixjsonQuery") public String register(@RequestBody String customerInfo){ Customer customer=JSON.parseObject(customerInfo, Customer.class); customer.setId(UUID.randomUUID().toString().replace("-", "")); String result=custService.register(customer); return result; } @ApiOperation(value="客户信息修改",notes="客户信息变更接口") @ApiImplicitParam(name="customerInfo",value="客户信息JSON",required=true,paramType="body") @RequestMapping(value="/api/v1/customers/{id}",method=RequestMethod.PUT) @HystrixCommand(fallbackMethod = "hystrixjsonQuery") public String alter(@RequestBody String customerInfo){ Customer customer=JSON.parseObject(customerInfo, Customer.class); String result=custService.alter(customer); return result; } @ApiOperation(value="客户信息查询",notes="客户信息查询接口") @ApiImplicitParams({ @ApiImplicitParam(name="name",value="客户姓名",required=false,paramType="query",dataType="String") }) @RequestMapping(value = "/api/v1/customers", method = RequestMethod.GET, produces = "application/json;charset=UTF-8") @HystrixCommand(fallbackMethod = "searchhystrixjsonQuery") public String search(Customer customer) { if(customer.getPageIndex()!=null&&customer.getPageSize()!=null){ customer.offset(); } List<Customer> list = custService.search(customer); String Array =JSONArray.toJSONString(list); return Array; } @ApiOperation(value="单个客户信息查询",notes="单个客户信息查询接口") @RequestMapping(value="/api/v1/customers/{id}",method=RequestMethod.GET) @HystrixCommand(fallbackMethod = "hystrixidQuery") public String getById(@PathVariable("id") String id){ Customer cust = custService.getById(id); String result = JSONObject.toJSONString(cust); return result; } @ApiOperation(value="客户信息总条数查询",notes="客户信息总条数查询接口") @ApiImplicitParams({ @ApiImplicitParam(name="name",value="客户名称",required=false,paramType="query",dataType="String") }) @RequestMapping(value = "/api/v1/customers/counts", method = RequestMethod.GET, produces = "application/json;charset=UTF-8") @HystrixCommand(fallbackMethod = "searchhystrixjsonQuery") public String searchCount(Customer customer) { if(customer.getPageIndex()!=null&&customer.getPageSize()!=null){ customer.offset(); } int count = custService.searchCount(customer); return String.valueOf(count); } @ApiOperation(value="删除单个公客户信息",notes="删除单个公客户信息接口") @RequestMapping(value="/api/v1/customers/{id}",method=RequestMethod.DELETE) @HystrixCommand(fallbackMethod = "hystrixidQuery") public String delete(@PathVariable("id") String id) throws UnsupportedEncodingException{ String result= custService.delete(id); return result; } public String hystrixQuery() { return "{\"result\":\"error\"}"; } public String hystrixidQuery(String id) { return "{\"result\":\"error\"}"; } public String hystrixjsonQuery(String customerInfo) { return "{\"result\":\"error\"}"; } public String searchhystrixjsonQuery(Customer customer) { return "{\"result\":\"error\"}"; } } |
地址为http://IP地址端口号/swagger-ui.html
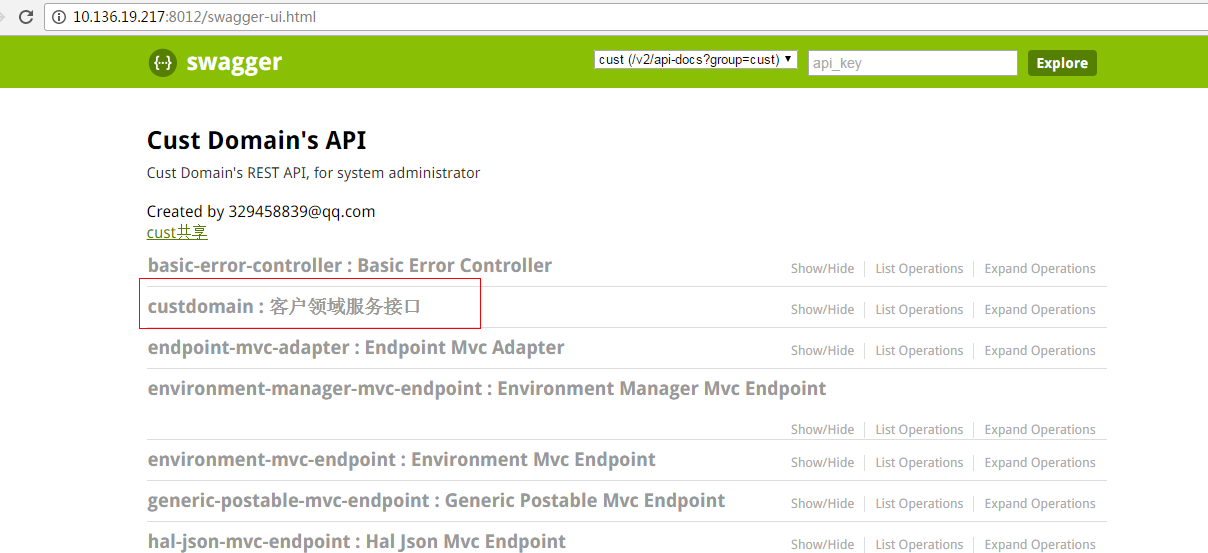
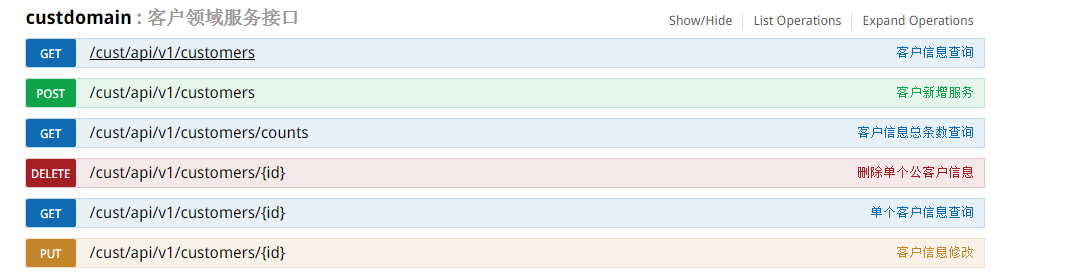
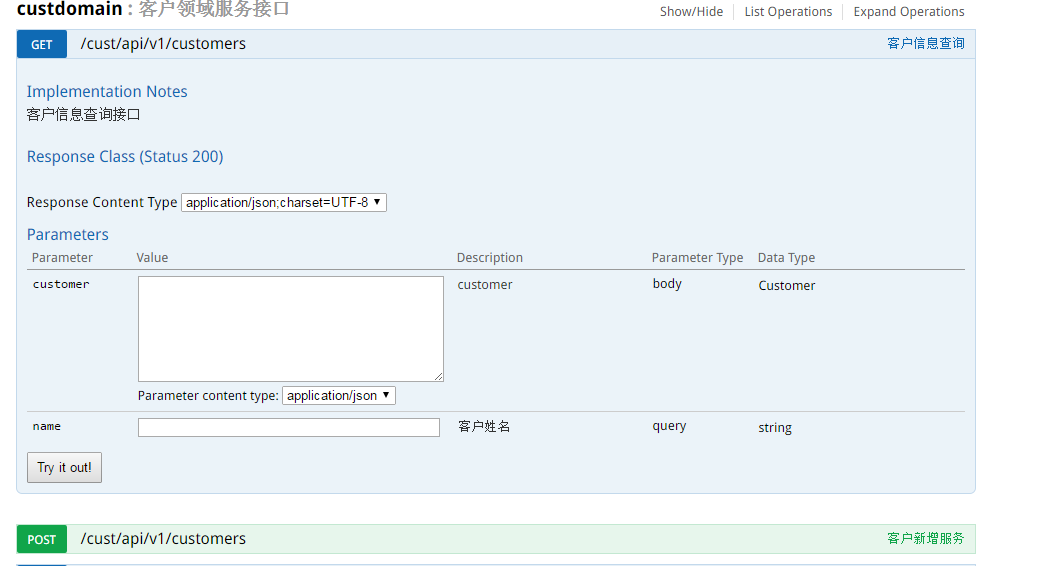
相关文章推荐
- Swagger-UI的配置与使用
- android游戏开发框架libgdx的使用(十一)—Skin和UI配置文件的使用
- 使用WeifenLuo.WinFormsUI.Docking界面布局中的保存配置
- Spring boot 中使用swagger-ui实现 restful-api
- swagger-UI在springMVC结构中的使用
- Swagger UI教程 API 文档神器 搭配Node使用
- android游戏开发框架libgdx的使用(十一)—Skin和UI配置文件的使用
- swagger-ui使用问题记录
- 使用Swagger,Swagger-UI生成REST API接口文档
- Asp.net Core WebApi 使用Swagger做帮助文档,并且自定义Swagger的UI
- 安卓app生成和使用xpath工具—lazy-uiautomatorviewer的配置和使用
- CloudFoundry Admin-UI 配置使用
- 在Web API中使用Swagger-UI开源组件(一个深坑的解决)
- Swagger UI教程 API 文档神器 搭配Node使用
- Swagger UI教程 API 文档神器 搭配Node使用
- Android游戏引擎libgdx使用教程11:如何使用Skin和UI配置文件
- swagger-UI使用之app服务器(JavaApplication)
- Apache shiro+springmvc+springdata+jpa+swagger(零配置文件使用)
- 解决spring boot中swagger-ui.html访问404以及配置全局header
- ng中使用ui-route配置demo