【算法学习】POJ3070——利用分治法来计算Fibonacci数列的值
2016-07-24 19:16
465 查看
DescriptionIn the Fibonacci integer sequence, F0 = 0, F1 = 1, and Fn = Fn − 1 + Fn − 2 for n ≥ 2. For example, the first ten terms of the Fibonacci sequence are:0, 1, 1, 2, 3, 5, 8, 13, 21, 34, …
An alternative formula for the Fibonacci sequence is

.
Given an integer n, your goal is to compute the last 4 digits of Fn.
InputThe input test file will contain multiple test cases. Each test case consists of a single line containing n (where 0 ≤ n ≤ 1,000,000,000). The end-of-file is denoted by a single line containing the number −1.
OutputFor each test case, print the last four digits of Fn. If the last four digits of Fn are all zeros, print ‘0’; otherwise, omit any leading zeros (i.e., print Fn mod 10000).
Sample Input0
9
999999999
1000000000
-1Sample Output0
34
626
6875HintAs a reminder, matrix multiplication is associative, and the product of two 2 × 2 matrices is given by

.Also, note that raising any 2 × 2 matrix to the 0th power gives the identity matrix:
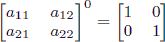
.
我的解决办法是利用了类似于求x^n的分治法来加快矩阵运算,使得计算Fibonacci数列第n项的时间复杂度变成了O(logn),比普通算法的O(n)更快
这题有一个比较坑的点就是每一步矩阵运算都要进行一次mod1000代码如下:#include <iostream>
using namespace std;
struct matrix {
long x00;
long x01;
long x10;
long x11;
};
matrix matrix_mul ( matrix a, matrix b ) {
matrix c = {0,0,0,0};
c.x00 = (a.x00*b.x00 + a.x01*b.x10)%10000;
c.x01 = (a.x00*b.x01 + a.x01*b.x11)%10000;
c.x10 = (a.x10*b.x00 + a.x11*b.x10)%10000;
c.x11 = (a.x10*b.x01 + a.x11*b.x11)%10000;
return c;
}
matrix matrix_pow ( matrix a, long n ) {
if ( n == 1 ) {
return a;
}
else if ( n%2 == 0 ) {
matrix temp = matrix_pow(a,n/2);
return matrix_mul(temp,temp);
}
else {
matrix temp = matrix_pow(a,(n-1)/2);
matrix temp1 = matrix_mul(temp,temp);
return matrix_mul(temp1,a);
}
}
long fibonacci_value ( long n ) {
if( n == 0 ) {
return 0;
}
else {
matrix root = {1,1,1,0};
root = matrix_pow(root,n);
return root.x01 % 10000;
}
}
int main()
{
long input[100] = {0};
int num = 0;
long temp;
while (true) {
cin >> temp;
if( temp != -1 ) {
input[num++] = temp;
}
else {
break;
}
}
for( int i = 0; i < num; ++i ) {
cout << fibonacci_value(input[i]) << endl;
}
return 0;
}
An alternative formula for the Fibonacci sequence is

.
Given an integer n, your goal is to compute the last 4 digits of Fn.
InputThe input test file will contain multiple test cases. Each test case consists of a single line containing n (where 0 ≤ n ≤ 1,000,000,000). The end-of-file is denoted by a single line containing the number −1.
OutputFor each test case, print the last four digits of Fn. If the last four digits of Fn are all zeros, print ‘0’; otherwise, omit any leading zeros (i.e., print Fn mod 10000).
Sample Input0
9
999999999
1000000000
-1Sample Output0
34
626
6875HintAs a reminder, matrix multiplication is associative, and the product of two 2 × 2 matrices is given by

.Also, note that raising any 2 × 2 matrix to the 0th power gives the identity matrix:
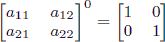
.
我的解决办法是利用了类似于求x^n的分治法来加快矩阵运算,使得计算Fibonacci数列第n项的时间复杂度变成了O(logn),比普通算法的O(n)更快
这题有一个比较坑的点就是每一步矩阵运算都要进行一次mod1000代码如下:#include <iostream>
using namespace std;
struct matrix {
long x00;
long x01;
long x10;
long x11;
};
matrix matrix_mul ( matrix a, matrix b ) {
matrix c = {0,0,0,0};
c.x00 = (a.x00*b.x00 + a.x01*b.x10)%10000;
c.x01 = (a.x00*b.x01 + a.x01*b.x11)%10000;
c.x10 = (a.x10*b.x00 + a.x11*b.x10)%10000;
c.x11 = (a.x10*b.x01 + a.x11*b.x11)%10000;
return c;
}
matrix matrix_pow ( matrix a, long n ) {
if ( n == 1 ) {
return a;
}
else if ( n%2 == 0 ) {
matrix temp = matrix_pow(a,n/2);
return matrix_mul(temp,temp);
}
else {
matrix temp = matrix_pow(a,(n-1)/2);
matrix temp1 = matrix_mul(temp,temp);
return matrix_mul(temp1,a);
}
}
long fibonacci_value ( long n ) {
if( n == 0 ) {
return 0;
}
else {
matrix root = {1,1,1,0};
root = matrix_pow(root,n);
return root.x01 % 10000;
}
}
int main()
{
long input[100] = {0};
int num = 0;
long temp;
while (true) {
cin >> temp;
if( temp != -1 ) {
input[num++] = temp;
}
else {
break;
}
}
for( int i = 0; i < num; ++i ) {
cout << fibonacci_value(input[i]) << endl;
}
return 0;
}
相关文章推荐
- 书评:《算法之美( Algorithms to Live By )》
- 动易2006序列号破解算法公布
- C#递归算法之分而治之策略
- Ruby实现的矩阵连乘算法
- C#插入法排序算法实例分析
- C#算法之大牛生小牛的问题高效解决方法
- C#算法函数:获取一个字符串中的最大长度的数字
- 超大数据量存储常用数据库分表分库算法总结
- C#数据结构与算法揭秘二
- C#冒泡法排序算法实例分析
- 算法练习之从String.indexOf的模拟实现开始
- C#算法之关于大牛生小牛的问题
- C#实现的算24点游戏算法实例分析
- 经典排序算法之冒泡排序(Bubble sort)代码
- c语言实现的带通配符匹配算法
- 浅析STL中的常用算法
- C语言求Fibonacci斐波那契数列通项问题的解法总结
- 算法之排列算法与组合算法详解
- C++实现一维向量旋转算法
- Ruby实现的合并排序算法