将二叉搜索树转换成双向链表
2016-06-30 20:19
337 查看
题意描述:输入一棵二叉搜索树,将该二叉搜索树转换成一个排序的双向链表。要求不能创建任何新的结点,只能调整树中结点指针的指向。如下图所示:
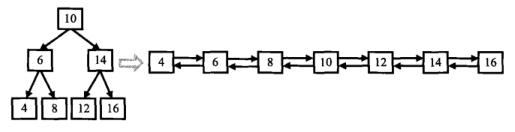
二叉树的定义如下:
而二叉树转换成双向链表时,可以将pLeft指针表示指向上一结点的指针,将pRight指针表示指向下一结点的指针。具体实现如下:
二叉树的定义如下:
struct BinaryTreeNode { int val; BinaryTreeNode* pLeft; BinaryTreeNode* pRight; };解题思路:二叉搜索树的中序遍历是有序的,而对于树的遍历一般都采用递归处理。这里同样,先将二叉搜索树分成左右子树,分别将左右子树排成双向链表,再连接左右子树与根结点,连接成整个双向链表
而二叉树转换成双向链表时,可以将pLeft指针表示指向上一结点的指针,将pRight指针表示指向下一结点的指针。具体实现如下:
BinaryTreeNode* ConvertToList(BinaryTreeNode* root) { BinaryTreeNode* pNode = NULL; ConvertToList(root, &pNode); //经过上一步得到的双向链表,pNode每次指向链表最后一个结点,所以这里要找到头结点返回 BinaryTreeNode* head = pNode; while (head != NULL && head->pLeft != NULL) head = head->pLeft; return head; } void ConvertToList(BinaryTreeNode* root, BinaryTreeNode**pNode) { if (pNode == NULL) return; BinaryTreeNode* tmp = root; if (tmp->pLeft != NULL) ConvertToList(tmp->pLeft, pNode); tmp->pLeft = *pNode; if (*pNode != NULL) (*pNode)->pRight = tmp; *pNode = tmp; if (tmp->pRight != NULL) ConvertToList(tmp->pRight, pNode); }
//中序遍历二叉搜索树 void ShowTree(BinaryTreeNode* root) { if (root->pLeft != NULL) ShowTree(root->pLeft); cout << root->val << " "; if (root->pRight != NULL) ShowTree(root->pRight); } //双向遍历双向链表 void ShowList(BinaryTreeNode* head) { BinaryTreeNode* pNode = head; cout << "正向遍历双向链表:"; while (pNode->pRight != NULL) { cout << pNode->val << " "; pNode = pNode->pRight; } cout << pNode->val << endl; cout << "反向遍历双向链表:"; while (pNode->pLeft != NULL) { cout << pNode->val << " "; pNode = pNode->pLeft; } cout << pNode->val << endl; }
int main() { BinaryTreeNode* root = new BinaryTreeNode(); BinaryTreeNode* node1 = new BinaryTreeNode(); BinaryTreeNode* node2 = new BinaryTreeNode(); BinaryTreeNode* node3 = new BinaryTreeNode(); BinaryTreeNode* node4 = new BinaryTreeNode(); BinaryTreeNode* node5 = new BinaryTreeNode(); BinaryTreeNode* node6 = new BinaryTreeNode(); root->val = 10; node1->val = 6; node2->val = 14; node3->val = 4; node4->val = 8; node5->val = 12; node6->val = 16; root->pLeft = node1; root->pRight = node2; node1->pLeft = node3; node1->pRight = node4; node2->pLeft = node5; node2->pRight = node6; cout << "初始二叉搜索树的中序遍历:"; ShowTree(root); cout << endl; BinaryTreeNode* head = ConvertToList(root); ShowList(head); return 0; }运行结果如下:
相关文章推荐
- hdu_3549_Flow Problem(最大流)
- 开发restful风格的webservice
- nrf24L01接收端只触发一次中断解决办法
- hdu_3549_Flow Problem(最大流)
- 全文搜索原理简单解析
- JAVA多线程和并发基础面试问答
- 百度谷歌离线地图解决方案(离线地图下载)
- CXF实现webservice 解决list<map>类型转换
- 【Android开发经验】android:windowSoftInputMode属性详解
- SceneKit:简单的3D游戏场景搭建
- SceneKit:简单的3D游戏场景搭建
- SceneKit:简单的3D游戏场景搭建
- Linux快速入门02-文件系统管理
- ffmpeg-20160629-git-bin.7z
- 图形绘制-线段绘制相关
- LoadRunner “add measurements”(添加度量)菜单问题
- 2016 ACM赛后总结
- Servlet基本接口与类
- cmake学习
- HDU-2817,同余定理+快速幂取模,水过~