c写Python扩展模块
2016-06-21 17:02
651 查看
c写Python扩展模块
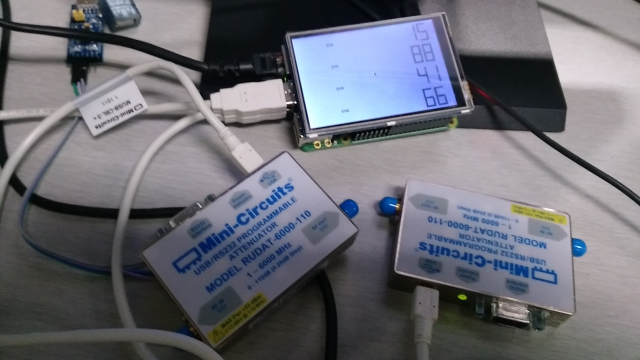
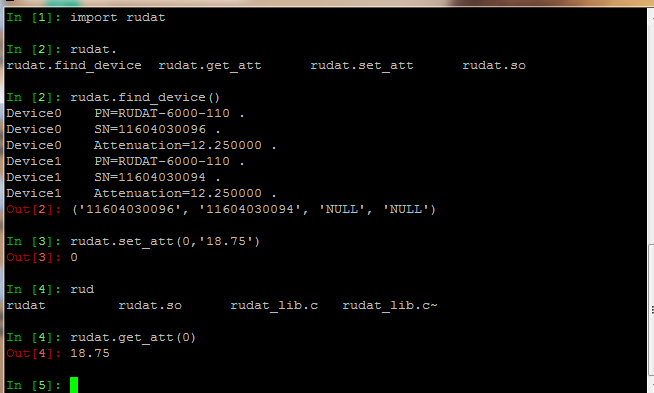
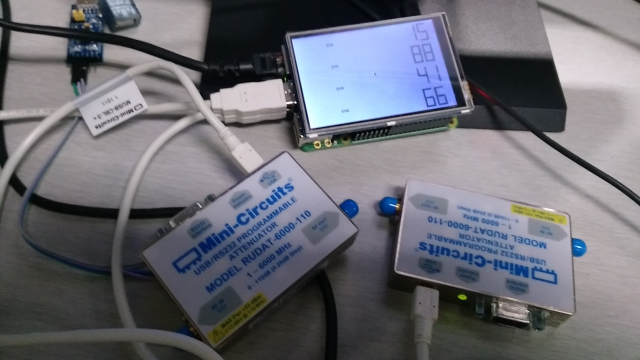
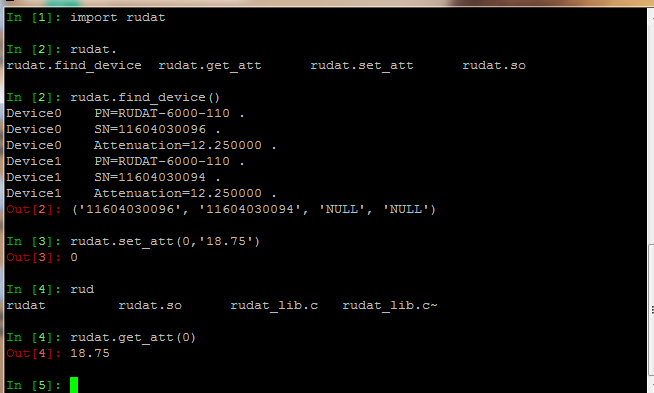
///////////////////////////////////////////////////////////////////////////////// // // //宁建 2016年6月21日16:27:10 // gcc rudat_lib.c lib*/*.c -w -I/usr/include/python2.7 -Ilibhid -Ilibusb -fpic -shared -o rudat.so /////////////////////////////////////////////////////////////////////////////// #include <hid.h> #include <stdio.h> #include <string.h> #include <usb.h> #include <Python.h> #define VENDOR_ID 0x20ce #define PRODUCT_ID 0x0023 #define PATHLEN 2 #define SEND_PACKET_LEN 64 HIDInterface *hid[4] = {NULL,NULL,NULL,NULL}; struct usb_device *usb_dev[4] = {NULL,NULL,NULL,NULL}; char PNreceive[4][SEND_PACKET_LEN]; char SNreceive[4][SEND_PACKET_LEN]; int FindDeviceNumber = 0; const int PATH_IN[PATHLEN] = { 0x00010005, 0x00010033 }; char PACKET[SEND_PACKET_LEN]; hid_return ret; bool match_serial_number(struct usb_dev_handle* usbdev, void* custom, unsigned int len) { return usb_device(usbdev) == custom; } static struct usb_device *device_init(void) { FindDeviceNumber = 0; struct usb_bus *usb_bus; struct usb_device *dev; usb_init(); usb_find_busses(); usb_find_devices(); for (usb_bus = usb_busses; usb_bus; usb_bus = usb_bus->next) { for (dev = usb_bus->devices; dev; dev = dev->next) { if ((dev->descriptor.idVendor == VENDOR_ID) && (dev->descriptor.idProduct == PRODUCT_ID)) { usb_dev[FindDeviceNumber++] = dev; } } } return usb_dev[0]; } void Get_PN (char* PNstr,HIDInterface *hid) { int i; char PACKETreceive[SEND_PACKET_LEN]; PACKET[0]=40; // PN code ret = hid_interrupt_write(hid, 0x01, PACKET, SEND_PACKET_LEN,500); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_write failed with return code %d\n", ret); } ret = hid_interrupt_read(hid, 0x01, PACKETreceive, SEND_PACKET_LEN,500); if (ret == HID_RET_SUCCESS) { strncpy(PNstr,PACKETreceive,SEND_PACKET_LEN); for (i=0;PNstr[i+1]!='\0';i++) { PNstr[i]=PNstr[i+1]; } PNstr[i]='\0'; } if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_read failed with return code %d\n", ret); } } void Get_SN (char* SNstr,HIDInterface *hid) { int i; char PACKETreceive[SEND_PACKET_LEN]; PACKET[0]=41; // SN Code ret = hid_interrupt_write(hid, 0x01, PACKET, SEND_PACKET_LEN,500); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_write failed with return code %d\n", ret); } ret = hid_interrupt_read(hid, 0x01, PACKETreceive, SEND_PACKET_LEN,500); if (ret == HID_RET_SUCCESS) { strncpy(SNstr,PACKETreceive,SEND_PACKET_LEN); for (i=0;SNstr[i+1]!='\0';i++) { SNstr[i]=SNstr[i+1]; } SNstr[i]='\0'; } if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_read failed with return code %d\n", ret); } } int Set_Attenuation (unsigned char *AttValue,HIDInterface *hid) // { if (hid == 0) return -1; int i; char PACKETreceive[SEND_PACKET_LEN]; PACKET[0]=19; // for Models: RUDAT-6000-90", RUDAT-6000-60, RUDAT-6000-30 RCDAT-6000-90", RCDAT-6000-60, RCDAT-6000-30 Set Attenuation code is 19. PACKET[1]= (int)atoi(AttValue); float t1=(float)(atof(AttValue)); PACKET[2]= (int) ((t1-PACKET[1])*4); ret = hid_interrupt_write(hid, 0x01, PACKET, SEND_PACKET_LEN,500); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_write failed with return code %d\n", ret); } ret = hid_interrupt_read(hid, 0x01, PACKETreceive, SEND_PACKET_LEN,500); // Read packet Packetreceive[0]=1 if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_read failed with return code %d\n", ret); return -1; } return 0; } float Get_Attenuation (HIDInterface *hid) // { if (hid == 0) return -1; int i; char PACKETreceive[SEND_PACKET_LEN]; PACKET[0]=18; ret = hid_interrupt_write(hid, 0x01, PACKET, SEND_PACKET_LEN,500); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_write failed with return code %d\n", ret); } ret = hid_interrupt_read(hid, 0x01, PACKETreceive, SEND_PACKET_LEN,500); // Read packet Packetreceive[0]=1 if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_interrupt_read failed with return code %d\n", ret); return -1; }else{ return PACKETreceive[1] + PACKETreceive[2]/4.0 ; } } void rudat_init(){ device_init(); int i= 0; struct usb_dev_handle *usb_handle; char buffer[80], kdname[80]; for(i=0; i<FindDeviceNumber;i++){ if (usb_dev[i] != NULL) { usb_handle = usb_open(usb_dev[i]); int drstatus = usb_get_driver_np(usb_handle, 0, kdname, sizeof(kdname)); if (kdname != NULL && strlen(kdname) > 0) { usb_detach_kernel_driver_np(usb_handle, 0); } } usb_reset(usb_handle); usb_close(usb_handle); HIDInterfaceMatcher matcher = { VENDOR_ID, PRODUCT_ID, match_serial_number, usb_dev[i], 0 }; if (i==0){ ret = hid_init(); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_init failed with return code %d\n", ret); break; } } hid[i] = hid_new_HIDInterface(); if (hid[i] == 0) { fprintf(stderr, "hid_new_HIDInterface() failed, out of memory?\n"); break; } ret = hid_force_open(hid[i], 0, &matcher, 3); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_force_open failed with return code %d\n", ret); break; } int StrLen1; Get_PN(PNreceive[i],hid[i]); fprintf(stderr,"Device%d PN=%s .\n",i,PNreceive[i]); Get_SN(SNreceive[i],hid[i]); fprintf(stderr,"Device%d SN=%s .\n",i,SNreceive[i]); Set_Attenuation("12.25",hid[i]); // set attenuation fprintf(stderr,"Device%d Attenuation=%f .\n",i, Get_Attenuation(hid[i])); } } void rudat_close() { int i = 0; for(i=0; i<4;i++) { if(hid[i]!= NULL) { ret = hid_close(hid[i]); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_close failed with return code %d\n", ret); break; } hid_delete_HIDInterface(&hid[i]); ret = hid_cleanup(); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_cleanup failed with return code %d\n", ret); break; } } } } void main_test(){ device_init(); int i= 0; struct usb_dev_handle *usb_handle; char buffer[80], kdname[80]; for(i=0; i<FindDeviceNumber;i++){ if (usb_dev[i] != NULL) { usb_handle = usb_open(usb_dev[i]); int drstatus = usb_get_driver_np(usb_handle, 0, kdname, sizeof(kdname)); if (kdname != NULL && strlen(kdname) > 0) { usb_detach_kernel_driver_np(usb_handle, 0); } } usb_reset(usb_handle); usb_close(usb_handle); HIDInterfaceMatcher matcher = { VENDOR_ID, PRODUCT_ID, match_serial_number, usb_dev[i], 0 }; ret = hid_init(); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_init failed with return code %d\n", ret); break; } hid[i] = hid_new_HIDInterface(); if (hid[i] == 0) { fprintf(stderr, "hid_new_HIDInterface() failed, out of memory?\n"); break; } ret = hid_force_open(hid[i], 0, &matcher, 3); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_force_open failed with return code %d\n", ret); break; } int StrLen1; Get_PN(PNreceive[i],hid[i]); fprintf(stderr,"Device%d PN=%s .\n",i,PNreceive[i]); Get_SN(SNreceive[i],hid[i]); fprintf(stderr,"Device%d SN=%s .\n",i,SNreceive[i]); // Set_Attenuation("12.25",hid[i]); // set attenuation fprintf(stderr,"Device%d Attenuation=%f .\n",i, Get_Attenuation(hid[i])); ret = hid_close(hid[i]); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_close failed with return code %d\n", ret); break; } hid_delete_HIDInterface(&hid[i]); ret = hid_cleanup(); if (ret != HID_RET_SUCCESS) { fprintf(stderr, "hid_cleanup failed with return code %d\n", ret); break; } } return 0; } PyObject* find_device(PyObject* self, PyObject* args) { rudat_close(); rudat_init(); PyObject* pTuple = PyTuple_New(4); assert(PyTuple_Check(pTuple)); assert(PyTuple_Size(pTuple) ==4); int i = 0; for(i =0 ; i< 4 ;i++){ if(hid[i] != NULL){ PyTuple_SetItem(pTuple, i, Py_BuildValue("s", SNreceive[i])); }else{ PyTuple_SetItem(pTuple, i, Py_BuildValue("s", "NULL")); } } return pTuple; } PyObject* set_att(PyObject* self, PyObject* args) { int result=-1; int index; char * value; if (PyArg_ParseTuple(args, "is", &index,&value)) result = Set_Attenuation(value,hid[index]); return Py_BuildValue("i", result); } PyObject* get_att(PyObject* self, PyObject* args) { float result=0; int index; char * value; if (PyArg_ParseTuple(args, "i", &index)) result = Get_Attenuation(hid[index]); return Py_BuildValue("f", result); } static PyMethodDef rudatMethods[] = { {"find_device", find_device, METH_VARARGS, "find_device"}, {"set_att", set_att, METH_VARARGS, "set_att"}, {"get_att", get_att, METH_VARARGS, "get_att"}, {NULL, NULL} }; void initrudat() { PyObject* m; m = Py_InitModule("rudat", rudatMethods); }
相关文章推荐
- 深入解析Python中的__builtins__内建对象
- 树莓派开机运行Python脚本的方法
- 浅谈Python中函数的参数传递
- Python的locals()函数
- python3.X中简单错误处理,和Python2区别
- python 中判断变量是否定义方法
- WGS84坐标系转火星坐标系
- 对比Python中__getattr__和 __getattribute__获取属性的用法
- python: os模块
- Micropython解释器大致执行流程
- 常见python正则用法的简单实例
- 【p2】·python中嵌套列表list元素输出·模块封装·发布上传(pigeon详细说)
- Python小白带小白初涉多进程
- python 循环遍历笔记
- python中if __name__ == '__main__': 的解析
- 小议Python中自定义函数的可变参数的使用及注意点
- python从数据库中获取utf8格式的中文数据输出时变成问号或乱码
- Pycharm选择pyenv安装的Python版本
- decode()和encode()
- 初心大陆-----python宝典 第五章之列表