光照示例
2016-06-20 00:00
465 查看
技巧如下:
在设置可变顶点格式时加入法线和漫反色,如下所示:
//
The 3D vertex format and descriptor
typedef
struct
{
float
x, y, z;
//
3D coordinates
float
nx, ny, nz;
//
normals
D3DCOLOR diffuse;
//
color
} VERTEX;
#define
VERTEX_FVF (D3DFVF_XYZ | D3DFVF_NORMAL | D3DFVF_DIFFUSE)
开启光照和Z缓存:
//
enable d3d lighting
g_d3d_device
->
SetRenderState(D3DRS_LIGHTING, TRUE);
//
enable z-buffer
g_d3d_device
->
SetRenderState(D3DRS_ZENABLE, D3DZB_TRUE);
设置光源属性并打开光源:
D3DLIGHT9 light;
//
set light data, color, position, range.
ZeroMemory(
&
light,
sizeof
(light));
light.Type
=
D3DLIGHT_POINT;
light.Diffuse.r
=
light.Ambient.r
=
0.5
;
light.Diffuse.g
=
light.Ambient.g
=
0.5
;
light.Diffuse.b
=
light.Ambient.b
=
0.0
;
light.Diffuse.a
=
light.Ambient.a
=
1.0
;
light.Range
=
1000.0
;
light.Attenuation0
=
0.5
;
light.Position.x
=
300.0
;
light.Position.y
=
0.0
;
light.Position.z
=
-
600.0
;
//
set and enable the light
g_d3d_device
->
SetLight(
0
,
&
light);
g_d3d_device
->
LightEnable(
0
, TRUE);
完整源码如下:
/*
**************************************************************************************
PURPOSE:
Light Demo
Required libraries:
WINMM.LIB, D3D9.LIB, D3DX9.LIB.
**************************************************************************************
*/
#include
<
windows.h
>
#include
<
stdio.h
>
#include
"
d3d9.h
"
#include
"
d3dx9.h
"
#pragma comment(lib,
"
winmm.lib
"
)
#pragma comment(lib,
"
d3d9.lib
"
)
#pragma comment(lib,
"
d3dx9.lib
"
)
#pragma warning(disable :
4305
)
#define
WINDOW_WIDTH 400
#define
WINDOW_HEIGHT 400
#define
Safe_Release(p) if((p)) (p)->Release();
//
window handles, class and caption text.
HWND g_hwnd;
HINSTANCE g_inst;
static
char
g_class_name[]
=
"
LightClass
"
;
static
char
g_caption[]
=
"
Light Demo
"
;
//
the Direct3D and device object
IDirect3D9
*
g_d3d
=
NULL;
IDirect3DDevice9
*
g_d3d_device
=
NULL;
//
The 3D vertex format and descriptor
typedef
struct
{
float
x, y, z;
//
3D coordinates
float
nx, ny, nz;
//
normals
D3DCOLOR diffuse;
//
color
} VERTEX;
#define
VERTEX_FVF (D3DFVF_XYZ | D3DFVF_NORMAL | D3DFVF_DIFFUSE)
IDirect3DVertexBuffer9
*
g_vertex_buffer
=
NULL;
//
--------------------------------------------------------------------------------
//
Window procedure.
//
--------------------------------------------------------------------------------
long
WINAPI Window_Proc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch
(msg)
{
case
WM_DESTROY:
PostQuitMessage(
0
);
return
0
;
}
return
(
long
) DefWindowProc(hwnd, msg, wParam, lParam);
}
//
--------------------------------------------------------------------------------
//
Initialize d3d, d3d device, vertex buffer, texutre; set render state for d3d;
//
set perspective matrix and view matrix.
//
--------------------------------------------------------------------------------
BOOL Do_Init()
{
D3DPRESENT_PARAMETERS present_param;
D3DDISPLAYMODE display_mode;
D3DXMATRIX mat_proj, mat_view;
D3DLIGHT9 light;
BYTE
*
vertex_ptr;
//
initialize vertex data
VERTEX verts[]
=
{
{
-
100.0f
,
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
-
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
-
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
100.0f
,
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
100.0f
,
-
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
-
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) }
};
//
do a windowed mode initialization of Direct3D
if
((g_d3d
=
Direct3DCreate9(D3D_SDK_VERSION))
==
NULL)
return
FALSE;
//
retrieves the current display mode of the adapter
if
(FAILED(g_d3d
->
GetAdapterDisplayMode(D3DADAPTER_DEFAULT,
&
display_mode)))
return
FALSE;
ZeroMemory(
&
present_param,
sizeof
(present_param));
//
initialize d3d presentation parameter
present_param.Windowed
=
TRUE;
present_param.SwapEffect
=
D3DSWAPEFFECT_DISCARD;
present_param.BackBufferFormat
=
display_mode.Format;
present_param.EnableAutoDepthStencil
=
TRUE;
present_param.AutoDepthStencilFormat
=
D3DFMT_D16;
//
creates a device to represent the display adapter
if
(FAILED(g_d3d
->
CreateDevice(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, g_hwnd,
D3DCREATE_SOFTWARE_VERTEXPROCESSING,
&
present_param,
&
g_d3d_device)))
return
FALSE;
//
set render state
//
enable d3d lighting
g_d3d_device
->
SetRenderState(D3DRS_LIGHTING, TRUE);
//
enable z-buffer
g_d3d_device
->
SetRenderState(D3DRS_ZENABLE, D3DZB_TRUE);
//
create and set the projection matrix
//
builds a left-handed perspective projection matrix based on a field of view
D3DXMatrixPerspectiveFovLH(
&
mat_proj, D3DX_PI
/
4.0
,
1.33333
,
1.0
,
1000.0
);
//
sets a single device transformation-related state
g_d3d_device
->
SetTransform(D3DTS_PROJECTION,
&
mat_proj);
//
create and set the view matrix
D3DXMatrixLookAtLH(
&
mat_view,
&
D3DXVECTOR3(
0.0
,
0.0
,
-
500.0
),
&
D3DXVECTOR3(
0.0f
,
0.0f
,
0.0f
),
&
D3DXVECTOR3(
0.0f
,
1.0f
,
0.0f
));
g_d3d_device
->
SetTransform(D3DTS_VIEW,
&
mat_view);
//
create the vertex buffer and set data
g_d3d_device
->
CreateVertexBuffer(
sizeof
(VERTEX)
*
16
,
0
, VERTEX_FVF, D3DPOOL_DEFAULT,
&
g_vertex_buffer, NULL);
//
locks a range of vertex data and obtains a pointer to the vertex buffer memory
g_vertex_buffer
->
Lock(
0
,
0
, (
void
**
)
&
vertex_ptr,
0
);
memcpy(vertex_ptr, verts,
sizeof
(verts));
//
unlocks vertex data
g_vertex_buffer
->
Unlock();
//
set light data, color, position, range.
ZeroMemory(
&
light,
sizeof
(light));
light.Type
=
D3DLIGHT_POINT;
light.Diffuse.r
=
light.Ambient.r
=
0.5
;
light.Diffuse.g
=
light.Ambient.g
=
0.5
;
light.Diffuse.b
=
light.Ambient.b
=
0.0
;
light.Diffuse.a
=
light.Ambient.a
=
1.0
;
light.Range
=
1000.0
;
light.Attenuation0
=
0.5
;
light.Position.x
=
300.0
;
light.Position.y
=
0.0
;
light.Position.z
=
-
600.0
;
//
set and enable the light
g_d3d_device
->
SetLight(
0
,
&
light);
g_d3d_device
->
LightEnable(
0
, TRUE);
return
TRUE;
}
//
--------------------------------------------------------------------------------
//
Release all d3d resource.
//
--------------------------------------------------------------------------------
BOOL Do_Shutdown()
{
Safe_Release(g_vertex_buffer);
Safe_Release(g_d3d_device);
Safe_Release(g_d3d);
return
TRUE;
}
//
--------------------------------------------------------------------------------
//
Render a frame.
//
--------------------------------------------------------------------------------
BOOL Do_Frame()
{
D3DXMATRIX mat_world;
//
clear device back buffer
g_d3d_device
->
Clear(
0
, NULL, D3DCLEAR_TARGET
|
D3DCLEAR_ZBUFFER, D3DCOLOR_RGBA(
0
,
0
,
0
,
255
),
1.0f
,
0
);
//
Begin scene
if
(SUCCEEDED(g_d3d_device
->
BeginScene()))
{
//
create and set the world transformation matrix
//
rotate object along y-axis
D3DXMatrixRotationY(
&
mat_world, (
float
) (timeGetTime()
/
1000.0
));
g_d3d_device
->
SetTransform(D3DTS_WORLD,
&
mat_world);
//
set the vertex stream, shader.
//
binds a vertex buffer to a device data stream
g_d3d_device
->
SetStreamSource(
0
, g_vertex_buffer,
0
,
sizeof
(VERTEX));
//
set the current vertex stream declation
g_d3d_device
->
SetFVF(VERTEX_FVF);
//
draw the vertex buffer
for
(
short
i
=
0
; i
<
4
; i
++
)
g_d3d_device
->
DrawPrimitive(D3DPT_TRIANGLESTRIP, i
*
4
,
2
);
//
end the scene
g_d3d_device
->
EndScene();
}
//
present the contents of the next buffer in the sequence of back buffers owned by the device
g_d3d_device
->
Present(NULL, NULL, NULL, NULL);
return
TRUE;
}
//
--------------------------------------------------------------------------------
//
Main function, routine entry.
//
--------------------------------------------------------------------------------
int
WINAPI WinMain(HINSTANCE inst, HINSTANCE, LPSTR cmd_line,
int
cmd_show)
{
WNDCLASSEX win_class;
MSG msg;
g_inst
=
inst;
//
create window class and register it
win_class.cbSize
=
sizeof
(win_class);
win_class.style
=
CS_CLASSDC;
win_class.lpfnWndProc
=
Window_Proc;
win_class.cbClsExtra
=
0
;
win_class.cbWndExtra
=
0
;
win_class.hInstance
=
inst;
win_class.hIcon
=
LoadIcon(NULL, IDI_APPLICATION);
win_class.hCursor
=
LoadCursor(NULL, IDC_ARROW);
win_class.hbrBackground
=
NULL;
win_class.lpszMenuName
=
NULL;
win_class.lpszClassName
=
g_class_name;
win_class.hIconSm
=
LoadIcon(NULL, IDI_APPLICATION);
if
(
!
RegisterClassEx(
&
win_class))
return
FALSE;
//
create the main window
g_hwnd
=
CreateWindow(g_class_name, g_caption, WS_CAPTION
|
WS_SYSMENU,
0
,
0
,
WINDOW_WIDTH, WINDOW_HEIGHT, NULL, NULL, inst, NULL);
if
(g_hwnd
==
NULL)
return
FALSE;
ShowWindow(g_hwnd, SW_NORMAL);
UpdateWindow(g_hwnd);
//
initialize game
if
(Do_Init()
==
FALSE)
return
FALSE;
//
start message pump, waiting for signal to quit.
ZeroMemory(
&
msg,
sizeof
(MSG));
while
(msg.message
!=
WM_QUIT)
{
if
(PeekMessage(
&
msg, NULL,
0
,
0
, PM_REMOVE))
{
TranslateMessage(
&
msg);
DispatchMessage(
&
msg);
}
//
draw a frame
if
(Do_Frame()
==
FALSE)
break
;
}
//
run shutdown function
Do_Shutdown();
UnregisterClass(g_class_name, inst);
return
(
int
) msg.wParam;
}
效果图:
在设置可变顶点格式时加入法线和漫反色,如下所示:
//
The 3D vertex format and descriptor
typedef
struct
{
float
x, y, z;
//
3D coordinates
float
nx, ny, nz;
//
normals
D3DCOLOR diffuse;
//
color
} VERTEX;
#define
VERTEX_FVF (D3DFVF_XYZ | D3DFVF_NORMAL | D3DFVF_DIFFUSE)
开启光照和Z缓存:
//
enable d3d lighting
g_d3d_device
->
SetRenderState(D3DRS_LIGHTING, TRUE);
//
enable z-buffer
g_d3d_device
->
SetRenderState(D3DRS_ZENABLE, D3DZB_TRUE);
设置光源属性并打开光源:
D3DLIGHT9 light;
//
set light data, color, position, range.
ZeroMemory(
&
light,
sizeof
(light));
light.Type
=
D3DLIGHT_POINT;
light.Diffuse.r
=
light.Ambient.r
=
0.5
;
light.Diffuse.g
=
light.Ambient.g
=
0.5
;
light.Diffuse.b
=
light.Ambient.b
=
0.0
;
light.Diffuse.a
=
light.Ambient.a
=
1.0
;
light.Range
=
1000.0
;
light.Attenuation0
=
0.5
;
light.Position.x
=
300.0
;
light.Position.y
=
0.0
;
light.Position.z
=
-
600.0
;
//
set and enable the light
g_d3d_device
->
SetLight(
0
,
&
light);
g_d3d_device
->
LightEnable(
0
, TRUE);
完整源码如下:
/*
**************************************************************************************
PURPOSE:
Light Demo
Required libraries:
WINMM.LIB, D3D9.LIB, D3DX9.LIB.
**************************************************************************************
*/
#include
<
windows.h
>
#include
<
stdio.h
>
#include
"
d3d9.h
"
#include
"
d3dx9.h
"
#pragma comment(lib,
"
winmm.lib
"
)
#pragma comment(lib,
"
d3d9.lib
"
)
#pragma comment(lib,
"
d3dx9.lib
"
)
#pragma warning(disable :
4305
)
#define
WINDOW_WIDTH 400
#define
WINDOW_HEIGHT 400
#define
Safe_Release(p) if((p)) (p)->Release();
//
window handles, class and caption text.
HWND g_hwnd;
HINSTANCE g_inst;
static
char
g_class_name[]
=
"
LightClass
"
;
static
char
g_caption[]
=
"
Light Demo
"
;
//
the Direct3D and device object
IDirect3D9
*
g_d3d
=
NULL;
IDirect3DDevice9
*
g_d3d_device
=
NULL;
//
The 3D vertex format and descriptor
typedef
struct
{
float
x, y, z;
//
3D coordinates
float
nx, ny, nz;
//
normals
D3DCOLOR diffuse;
//
color
} VERTEX;
#define
VERTEX_FVF (D3DFVF_XYZ | D3DFVF_NORMAL | D3DFVF_DIFFUSE)
IDirect3DVertexBuffer9
*
g_vertex_buffer
=
NULL;
//
--------------------------------------------------------------------------------
//
Window procedure.
//
--------------------------------------------------------------------------------
long
WINAPI Window_Proc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch
(msg)
{
case
WM_DESTROY:
PostQuitMessage(
0
);
return
0
;
}
return
(
long
) DefWindowProc(hwnd, msg, wParam, lParam);
}
//
--------------------------------------------------------------------------------
//
Initialize d3d, d3d device, vertex buffer, texutre; set render state for d3d;
//
set perspective matrix and view matrix.
//
--------------------------------------------------------------------------------
BOOL Do_Init()
{
D3DPRESENT_PARAMETERS present_param;
D3DDISPLAYMODE display_mode;
D3DXMATRIX mat_proj, mat_view;
D3DLIGHT9 light;
BYTE
*
vertex_ptr;
//
initialize vertex data
VERTEX verts[]
=
{
{
-
100.0f
,
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
-
100.0f
,
0.0f
,
0.0f
,
-
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
-
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
-
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
100.0f
,
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
100.0f
,
-
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
100.0f
,
0.0f
,
0.0f
,
1.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
100.0f
,
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
100.0f
,
-
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) },
{
-
100.0f
,
-
100.0f
,
-
100.0f
,
-
1.0f
,
0.0f
,
0.0f
, D3DCOLOR_RGBA(
255
,
255
,
255
,
255
) }
};
//
do a windowed mode initialization of Direct3D
if
((g_d3d
=
Direct3DCreate9(D3D_SDK_VERSION))
==
NULL)
return
FALSE;
//
retrieves the current display mode of the adapter
if
(FAILED(g_d3d
->
GetAdapterDisplayMode(D3DADAPTER_DEFAULT,
&
display_mode)))
return
FALSE;
ZeroMemory(
&
present_param,
sizeof
(present_param));
//
initialize d3d presentation parameter
present_param.Windowed
=
TRUE;
present_param.SwapEffect
=
D3DSWAPEFFECT_DISCARD;
present_param.BackBufferFormat
=
display_mode.Format;
present_param.EnableAutoDepthStencil
=
TRUE;
present_param.AutoDepthStencilFormat
=
D3DFMT_D16;
//
creates a device to represent the display adapter
if
(FAILED(g_d3d
->
CreateDevice(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, g_hwnd,
D3DCREATE_SOFTWARE_VERTEXPROCESSING,
&
present_param,
&
g_d3d_device)))
return
FALSE;
//
set render state
//
enable d3d lighting
g_d3d_device
->
SetRenderState(D3DRS_LIGHTING, TRUE);
//
enable z-buffer
g_d3d_device
->
SetRenderState(D3DRS_ZENABLE, D3DZB_TRUE);
//
create and set the projection matrix
//
builds a left-handed perspective projection matrix based on a field of view
D3DXMatrixPerspectiveFovLH(
&
mat_proj, D3DX_PI
/
4.0
,
1.33333
,
1.0
,
1000.0
);
//
sets a single device transformation-related state
g_d3d_device
->
SetTransform(D3DTS_PROJECTION,
&
mat_proj);
//
create and set the view matrix
D3DXMatrixLookAtLH(
&
mat_view,
&
D3DXVECTOR3(
0.0
,
0.0
,
-
500.0
),
&
D3DXVECTOR3(
0.0f
,
0.0f
,
0.0f
),
&
D3DXVECTOR3(
0.0f
,
1.0f
,
0.0f
));
g_d3d_device
->
SetTransform(D3DTS_VIEW,
&
mat_view);
//
create the vertex buffer and set data
g_d3d_device
->
CreateVertexBuffer(
sizeof
(VERTEX)
*
16
,
0
, VERTEX_FVF, D3DPOOL_DEFAULT,
&
g_vertex_buffer, NULL);
//
locks a range of vertex data and obtains a pointer to the vertex buffer memory
g_vertex_buffer
->
Lock(
0
,
0
, (
void
**
)
&
vertex_ptr,
0
);
memcpy(vertex_ptr, verts,
sizeof
(verts));
//
unlocks vertex data
g_vertex_buffer
->
Unlock();
//
set light data, color, position, range.
ZeroMemory(
&
light,
sizeof
(light));
light.Type
=
D3DLIGHT_POINT;
light.Diffuse.r
=
light.Ambient.r
=
0.5
;
light.Diffuse.g
=
light.Ambient.g
=
0.5
;
light.Diffuse.b
=
light.Ambient.b
=
0.0
;
light.Diffuse.a
=
light.Ambient.a
=
1.0
;
light.Range
=
1000.0
;
light.Attenuation0
=
0.5
;
light.Position.x
=
300.0
;
light.Position.y
=
0.0
;
light.Position.z
=
-
600.0
;
//
set and enable the light
g_d3d_device
->
SetLight(
0
,
&
light);
g_d3d_device
->
LightEnable(
0
, TRUE);
return
TRUE;
}
//
--------------------------------------------------------------------------------
//
Release all d3d resource.
//
--------------------------------------------------------------------------------
BOOL Do_Shutdown()
{
Safe_Release(g_vertex_buffer);
Safe_Release(g_d3d_device);
Safe_Release(g_d3d);
return
TRUE;
}
//
--------------------------------------------------------------------------------
//
Render a frame.
//
--------------------------------------------------------------------------------
BOOL Do_Frame()
{
D3DXMATRIX mat_world;
//
clear device back buffer
g_d3d_device
->
Clear(
0
, NULL, D3DCLEAR_TARGET
|
D3DCLEAR_ZBUFFER, D3DCOLOR_RGBA(
0
,
0
,
0
,
255
),
1.0f
,
0
);
//
Begin scene
if
(SUCCEEDED(g_d3d_device
->
BeginScene()))
{
//
create and set the world transformation matrix
//
rotate object along y-axis
D3DXMatrixRotationY(
&
mat_world, (
float
) (timeGetTime()
/
1000.0
));
g_d3d_device
->
SetTransform(D3DTS_WORLD,
&
mat_world);
//
set the vertex stream, shader.
//
binds a vertex buffer to a device data stream
g_d3d_device
->
SetStreamSource(
0
, g_vertex_buffer,
0
,
sizeof
(VERTEX));
//
set the current vertex stream declation
g_d3d_device
->
SetFVF(VERTEX_FVF);
//
draw the vertex buffer
for
(
short
i
=
0
; i
<
4
; i
++
)
g_d3d_device
->
DrawPrimitive(D3DPT_TRIANGLESTRIP, i
*
4
,
2
);
//
end the scene
g_d3d_device
->
EndScene();
}
//
present the contents of the next buffer in the sequence of back buffers owned by the device
g_d3d_device
->
Present(NULL, NULL, NULL, NULL);
return
TRUE;
}
//
--------------------------------------------------------------------------------
//
Main function, routine entry.
//
--------------------------------------------------------------------------------
int
WINAPI WinMain(HINSTANCE inst, HINSTANCE, LPSTR cmd_line,
int
cmd_show)
{
WNDCLASSEX win_class;
MSG msg;
g_inst
=
inst;
//
create window class and register it
win_class.cbSize
=
sizeof
(win_class);
win_class.style
=
CS_CLASSDC;
win_class.lpfnWndProc
=
Window_Proc;
win_class.cbClsExtra
=
0
;
win_class.cbWndExtra
=
0
;
win_class.hInstance
=
inst;
win_class.hIcon
=
LoadIcon(NULL, IDI_APPLICATION);
win_class.hCursor
=
LoadCursor(NULL, IDC_ARROW);
win_class.hbrBackground
=
NULL;
win_class.lpszMenuName
=
NULL;
win_class.lpszClassName
=
g_class_name;
win_class.hIconSm
=
LoadIcon(NULL, IDI_APPLICATION);
if
(
!
RegisterClassEx(
&
win_class))
return
FALSE;
//
create the main window
g_hwnd
=
CreateWindow(g_class_name, g_caption, WS_CAPTION
|
WS_SYSMENU,
0
,
0
,
WINDOW_WIDTH, WINDOW_HEIGHT, NULL, NULL, inst, NULL);
if
(g_hwnd
==
NULL)
return
FALSE;
ShowWindow(g_hwnd, SW_NORMAL);
UpdateWindow(g_hwnd);
//
initialize game
if
(Do_Init()
==
FALSE)
return
FALSE;
//
start message pump, waiting for signal to quit.
ZeroMemory(
&
msg,
sizeof
(MSG));
while
(msg.message
!=
WM_QUIT)
{
if
(PeekMessage(
&
msg, NULL,
0
,
0
, PM_REMOVE))
{
TranslateMessage(
&
msg);
DispatchMessage(
&
msg);
}
//
draw a frame
if
(Do_Frame()
==
FALSE)
break
;
}
//
run shutdown function
Do_Shutdown();
UnregisterClass(g_class_name, inst);
return
(
int
) msg.wParam;
}
效果图:
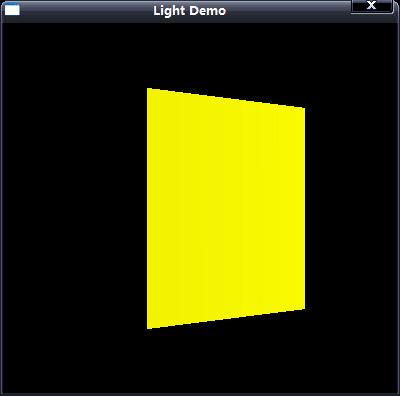
相关文章推荐
- DXUT框架剖析(4)
- 结构体对齐的具体含义(#pragma pack)
- DXUT框架剖析(2)
- 网友怒批“Linux难敌视窗新七大理由”之我见
- 写给所有的IT民工们
- 光照示例
- 此诗甚妙
- 双节棍(C语言版)
- 结构体对齐的具体含义(#pragma pack)
- 我的CS脚本autoexec.cfg
- DXUT框架剖析(2)
- Visual Studio 2005中C++的变化
- 重新启用ClustrMaps记数
- 写给所有的IT民工们
- 此诗甚妙
- 双节棍(C语言版)
- 我的CS脚本autoexec.cfg
- Visual Studio 2005中C++的变化
- Direct3D中的绘制(2)
- 重新启用ClustrMaps记数