26、使用SimpleAdapter创建ListView和自定义Toast布局显示
2016-05-11 10:57
661 查看
在activity_main.xml文件中(显示的整个Listview)
在listview_item_layout.xml文件中(显示的是listview的每一项的布局)
在toast_layout.xml文件中(显示的是Toast的自定义的布局文件)
在MainActivity.class文件中
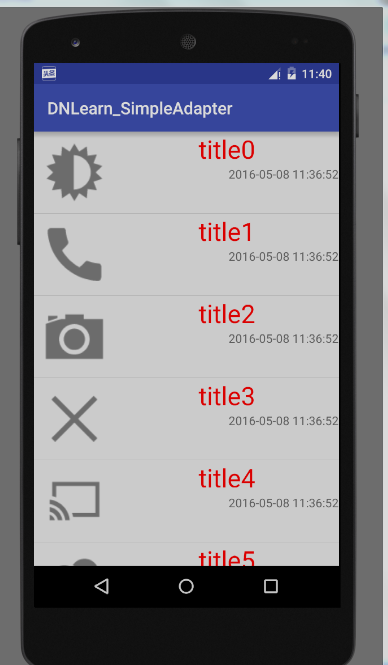
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.example.yuxue.dnlearn_simpleadapter.MainActivity"> <ListView android:id="@+id/listview" android:layout_width="match_parent" android:layout_height="match_parent"></ListView> </LinearLayout>
在listview_item_layout.xml文件中(显示的是listview的每一项的布局)
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:id="@+id/imgview" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@mipmap/ic_launcher" android:layout_alignParentLeft="true" android:layout_alignParentTop="true"/> <TextView android:id="@+id/tvtitle" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:text="title" android:textSize="30dp" android:textColor="#f00" android:gravity="center" android:layout_toRightOf="@id/imgview"/> <TextView android:id="@+id/tvtime" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="2016-5-8" android:layout_alignParentRight="true" android:layout_below="@id/tvtitle"/> </RelativeLayout>
在toast_layout.xml文件中(显示的是Toast的自定义的布局文件)
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#00ff00" android:id="@+id/toastlayout"> <ImageView android:id="@+id/toast_imgview" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@mipmap/ic_launcher" android:layout_alignParentLeft="true" android:layout_alignParentTop="true"/> <TextView android:id="@+id/toast_tvtitle" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:text="title" android:textSize="30dp" android:textColor="#f00" android:gravity="center" android:layout_toRightOf="@id/toast_imgview"/> <TextView android:id="@+id/toast_tvtime" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="2016-5-8" android:layout_alignParentRight="true" android:layout_below="@id/toast_tvtitle"/> </RelativeLayout>
在MainActivity.class文件中
package com.example.yuxue.dnlearn_simpleadapter; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.Gravity; import android.view.View; import android.widget.AdapterView; import android.widget.ImageView; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.TextView; import android.widget.Toast; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Date; import java.util.HashMap; import java.util.List; import java.util.Map; public class MainActivity extends AppCompatActivity implements AdapterView.OnItemClickListener { ListView listView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initViews(); } private void initViews() { // 1、控件 listView = (ListView) findViewById(R.id.listview); //2、数据 List<Map<String, Object>> date = getDate(); // 3、子布局 (listview 的每一项的布局) int itemlayout = R.layout.listview_item_layout; // 4、适配器 SimpleAdapter simpleAdapter = new SimpleAdapter(this,//上下文对象 date, itemlayout,// item view 对应的资源ID new String[]{"imgKey", "titleKey", "timeKey"},// Map的Key new int[]{R.id.imgview, R.id.tvtitle, R.id.tvtime}); // map的数据要显示在Item view的对应位置 //5、关联适配器 listView.setAdapter(simpleAdapter); listView.setOnItemClickListener(this); } public List<Map<String, Object>> getDate() { // 指定时间的格式 SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss"); // 对应整个数据的集合 List<Map<String, Object>> lists = new ArrayList<Map<String, Object>>(); int[] imgid = new int[]{R.drawable.ic01, R.drawable.ic02, R.drawable.ic03, R.drawable.ic04, R.drawable.ic05, R.drawable.ic06, R.drawable.ic07, R.drawable.ic08, R.drawable.ic09}; for (int i = 0; i < imgid.length; i++) { // 一个Map对应ListView中的一个Item Map<String, Object> map = new HashMap<String, Object>(); map.put("imgKey", imgid[i]); map.put("titleKey", "title" + i); map.put("timeKey", sdf.format(new Date()));// 获取当前时间(长整型),进行格式转换 lists.add(map); } return lists; } @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { // 根据位置找到对应的数据并取出 Map<String, Object> map = (Map<String, Object>) parent.getItemAtPosition(position); Integer img = (Integer) map.get("imgKey"); String string_title = (String) map.get("titleKey"); String string_time = (String) map.get("timeKey"); Toast toast = new Toast(MainActivity.this); //Toast显示的是自定义的布局文件的布局 /* 法一: LayoutInflater inflater = getLayoutInflater(); View v=inflater.inflate(R.layout.toast_layout, (ViewGroup) findViewById(R.id.toastlayout));*/ // 映射对应的布局中间的参数就是要映射的布局,这样才可以找到那个布局中的id等 View v=View.inflate(MainActivity.this,R.layout.toast_layout,null); ImageView imgview = (ImageView)v.findViewById(R.id.toast_imgview); TextView tv_title = (TextView) v.findViewById(R.id.toast_tvtitle); TextView tv_time = (TextView) v.findViewById(R.id.toast_tvtime); //改变布局内的内容 imgview.setImageResource(img); tv_title.setText(string_title); tv_time.setText(string_time); // 设置显示界面 toast.setView(v); // 设置显示时间 toast.setDuration(Toast.LENGTH_SHORT); // 设置显示位置 X给正数显示偏右,Y给正数显示偏下 toast.setGravity(Gravity.CENTER, 0, 60); toast.show(); } }
相关文章推荐
- Nginx安装
- android中的反射
- Android基础知识
- 一致性hash算法(consistenthashing)
- C++作业5
- IOS数据存储之归档/解档
- Docker 使用简单介绍
- Eclipse快捷键
- IAR STM8工程中断的使用
- Android Dialog 隐藏键盘问题
- 【TOF-3D】学习笔记(1)-上层界面的功能设计
- 文章标题
- Centos配置VLAN+BRIDGE实现虚拟机KVM划分VLAN
- Mysql 创建查询视图
- Git查看、删除、重命名远程分支和tag
- margin:0 auto 与 text-align:center 的区别
- 【好程序员特训营】- 路新艺-Java面向对象之继承和重写
- Git push到多个远程库
- 系统分区
- 微信支付开发-从零开始-Part4