Android:SpannableString使用详解
2016-04-12 13:57
344 查看
在Android开发过程中,我们有时会需要TextView 显示各种格式的文本,包括字体颜色,大小,下划线,表情符号等等。对于这种需求,我们应该如何实现呢?答案是:SpannableString。
我们可以通过SpannableString来实现各种格式的文本。它的使用非常简单,这里不多介绍了。直接看代码会更加的直观。
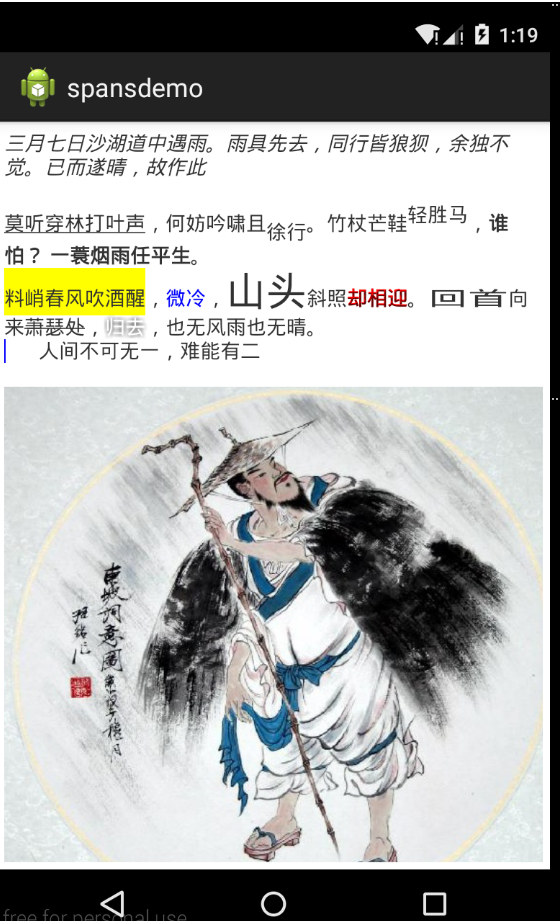
MainActivity.java
【译】Spans,一个强大的概念(上篇文章的中文译文)
我们可以通过SpannableString来实现各种格式的文本。它的使用非常简单,这里不多介绍了。直接看代码会更加的直观。
效果
我们先来看一下运行的效果。代码如下
activity_main.xml<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.nxiangbo.spansdemo.MainActivity" > <TextView android:id="@+id/tv_content" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="5dp" android:text="@string/content"/> </RelativeLayout>
MainActivity.java
public class MainActivity extends Activity { private static final String TAG = "MainActivity"; private TextView mText; private SpannableString mSpannableString; private String mContent; private static final String itallic = "三月七日沙湖道中遇雨。雨具先去,同行皆狼狈,余独不觉。已而遂晴,故作此 "; private static final String bold = "谁怕? 一蓑烟雨任平生"; private static final String underline = "莫听穿林打叶声"; private static final String subscript = "徐行"; private static final String superscript = "轻胜马"; private static final String backgroundCorlor = "料峭春风吹酒醒"; private static final String foregroundColor = "微冷"; private static final String relativeSize = "山头"; private static final String maskFilter = "却相迎"; private static final String scaleX = "回首"; private static final String strikeThrough = "萧瑟处"; private static final String blurMaskFilter = "归去"; private static final String image = "苏轼"; private static final String quote = "人间不可无一,难能有二"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mText = (TextView) findViewById(R.id.tv_content); mContent = getResources().getString(R.string.content); mSpannableString = new SpannableString(mContent); Log.e(TAG, mContent.length()+"mContent.length"); setItallic(itallic); setBold(bold); setUnderLine(underline); setSubscript(subscript); setSuperscript(superscript); setBackgroundColor(backgroundCorlor); setForegroundColor(foregroundColor); setRelativeSize(relativeSize); setMaskFilter(maskFilter); setScaleX(scaleX); setStrikeThrough(strikeThrough); setBlurMaskFilter(blurMaskFilter); setImage(image); setQuote(quote); mText.setText(mSpannableString); } //将"苏轼"替换成一张图片 @SuppressLint("NewApi") private void setImage(String text) { Object span = null; span = new ImageSpan(this,R.drawable.demo); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //将字体 4000 设置为模糊效果 private void setBlurMaskFilter(String text) { Object span = null; span = new MaskFilterSpan(new BlurMaskFilter(5, BlurMaskFilter.Blur.OUTER)); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置删除线 private void setStrikeThrough(String text) { Object span = null; span = new StrikethroughSpan(); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置字体在水平方向上放大两倍 private void setScaleX(String text) { Object span = null; span = new ScaleXSpan(2.0f); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置字体为浮雕形式的 private void setMaskFilter(String text) { Object span = null; span = new MaskFilterSpan(new EmbossMaskFilter(new float[] { 1, 5, 1 }, 0.4f, 6, 3.5f)); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; ForegroundColorSpan fg = new ForegroundColorSpan(Color.RED); StyleSpan style = new StyleSpan(android.graphics.Typeface.BOLD); mSpannableString.setSpan(fg, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); mSpannableString.setSpan(style, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置字体大小的原来大两倍 private void setRelativeSize(String text) { Object span = null; span = new RelativeSizeSpan(2.0f); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置前景颜色 private void setForegroundColor(String text) { Object span = null; span = new ForegroundColorSpan(Color.BLUE); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置背景颜色 private void setBackgroundColor(String text) { Object span = null; span = new BackgroundColorSpan(Color.YELLOW); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置黑体 private void setBold(String text){ Object span = null; span = new StyleSpan(android.graphics.Typeface.BOLD); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置斜体 private void setItallic(String text){ Object span = null; span = new StyleSpan(android.graphics.Typeface.ITALIC); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置引用 private void setQuote(String text){ Object span = null; span = new CustomQuoteSpan(Color.BLUE); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置下划线 private void setUnderLine(String text){ Object span = new UnderlineSpan(); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置下标 private void setSubscript(String text){ Object span = new SubscriptSpan(); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } //设置上标 private void setSuperscript(String text){ Object span = new SuperscriptSpan(); WordPosition position = getWordPosition(text); int textStart = position.start; int textEnd = position.end; mSpannableString.setSpan(span, textStart, textEnd, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } private WordPosition getWordPosition(String text) { int start = mContent.indexOf(text); int end = start + text.length(); return new WordPosition(start, end); } private static final class WordPosition { int start; int end; private WordPosition(int start, int end) { this.start = start; this.end = end; } @Override public String toString() { return "WordPosition{" + "start=" + start + ", end=" + end + '}'; } } }
源码下载地址
参考文章
Spans, a Powerful Concept.【译】Spans,一个强大的概念(上篇文章的中文译文)
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories