定位框一闪而过 iOS Swift
2016-03-29 19:16
495 查看
需求:获取经纬度。
方案:我自定义了一个类模块CLLocationModule.swift
备注以下代码里
let IS_IOS8 = (UIDevice.currentDevice().systemVersion as NSString).doubleValue >= 8.0
最开始的代码
import UIKit
import CoreLocation
class CLLocationModule: NSObject ,CLLocationManagerDelegate{
var latitude:Double?
var longitude:Double?
var city:NSString?
func GetLatitudeLongitudeAndCity(){
if CLLocationManager.locationServicesEnabled() == false {
print("此设备不能定位")
return
}
var locManager = CLLocationManager()
locManager.delegate = self
locManager.desiredAccuracy = kCLLocationAccuracyBest
locManager.distanceFilter = 1000.0
//设置定位权限仅ios8有意义
if IS_IOS8 {
locManager.requestWhenInUseAuthorization()// 前台定位
locManager.requestAlwaysAuthorization()
}
locManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
for location in locations {
print("纬度:%g",location.coordinate.latitude);
print("经度:%g",location.coordinate.longitude);
latitude = location.coordinate.latitude
longitude = location.coordinate.longitude
self.saveLatitude(latitude!.description)
self.saveLongitude(longitude!.description)
let geocoder: CLGeocoder = CLGeocoder()
geocoder.reverseGeocodeLocation(location) { (placemarks, error) in
if error != nil {
print("reverse geodcode fail: \(error!.localizedDescription)")
return
}
let pm = placemarks! as [CLPlacemark]
if (pm.count > 0){
for placemark :CLPlacemark in placemarks! {
let placemarkDict = placemark.addressDictionary
let placemarkStr = placemarkDict!["State"]
self.city = placemarkStr?.substringToIndex((placemarkStr?.length)!-1)
self.saveCity(self.city!)
}
}else{
print("No Placemarks!")
}
}
}
manager.stopUpdatingLocation()
}
func locationManager(manager:CLLocationManager, didFailWithError error:NSError) {
print("locationManager error");
}
func saveLatitude(latitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(latitude, forKey: "latitude")
defaults.synchronize()
}
func readLatitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let latitude = defaults.objectForKey("latitude") as! NSString
return latitude;
}
func saveLongitude(longitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(longitude, forKey: "longitude")
defaults.synchronize()
}
func readLongitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let longitude = defaults.objectForKey("longitude") as! NSString
return longitude;
}
func saveCity(city: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(city, forKey: "city")
defaults.synchronize()
}
func readCity() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let city = defaults.objectForKey("city") as! NSString
return city;
}
}
外部调用是:
CLLocationModule().GetLatitudeLongitudeAndCity()
结果:定位框一闪而过 (允许 不允许那个弹出框) 导致不走代理方法didUpdateLocations 最后查阅网页 知道
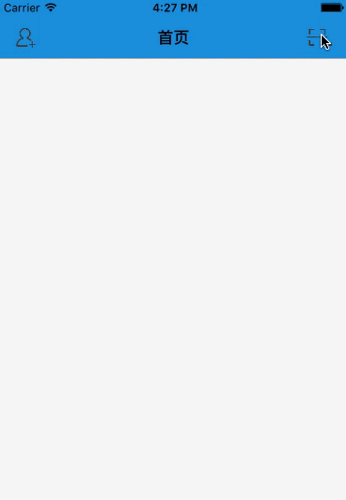
(a)locManager没定义为全局变量
(b)自定义的CLLocationModule.swift这个类 由于ARC的缘故 自动释放了
针对以上(a)问题的解决办法为自定义类CLLocationModule.swift中将var locManager = CLLocationManager() 改为全局变量
var locManager: CLLocationManager!
locManager = CLLocationManager()
针对以上(b)问题的解决方案有两种
(1)外部调用时改为:(改为强引用)
var aCLLocationModule = CLLocationModule()
self.aCLLocationModule.GetLatitudeLongitudeAndCity()
(2)自定义的类CLLocationModule.swift改为单例
//单例
private static let aSharedInstance: CLLocationModule = CLLocationModule()
private override init() {}
class func sharedInstance() -> CLLocationModule {
return aSharedInstance
}
外部调用时改为:
CLLocationModule.sharedInstance().GetLatitudeLongitudeAndCity()
成功的代码附上两份
(1)单例
import UIKit
import CoreLocation
class CLLocationModule: NSObject ,CLLocationManagerDelegate{
//单例
private static let aSharedInstance: CLLocationModule = CLLocationModule()
private override init() {}
class func sharedInstance() -> CLLocationModule {
return aSharedInstance
}
var locManager: CLLocationManager!
var latitude:Double?
var longitude:Double?
var city:NSString?
func GetLatitudeLongitudeAndCity(){
if CLLocationManager.locationServicesEnabled() == false {
print("此设备不能定位")
return
}
locManager = CLLocationManager()
locManager.delegate = self
locManager.desiredAccuracy = kCLLocationAccuracyBest
locManager.distanceFilter = 1000.0
//设置定位权限仅ios8有意义
if IS_IOS8 {
locManager.requestWhenInUseAuthorization()// 前台定位
locManager.requestAlwaysAuthorization()
}
locManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
for location in locations {
print("纬度:%g",location.coordinate.latitude);
print("经度:%g",location.coordinate.longitude);
latitude = location.coordinate.latitude
longitude = location.coordinate.longitude
self.saveLatitude(latitude!.description)
self.saveLongitude(longitude!.description)
let geocoder: CLGeocoder = CLGeocoder()
geocoder.reverseGeocodeLocation(location) { (placemarks, error) in
if error != nil {
print("reverse geodcode fail: \(error!.localizedDescription)")
return
}
let pm = placemarks! as [CLPlacemark]
if (pm.count > 0){
for placemark :CLPlacemark in placemarks! {
let placemarkDict = placemark.addressDictionary
let placemarkStr = placemarkDict!["State"]
self.city = placemarkStr?.substringToIndex((placemarkStr?.length)!-1)
self.saveCity(self.city!)
}
}else{
print("No Placemarks!")
}
}
}
manager.stopUpdatingLocation()
}
func locationManager(manager:CLLocationManager, didFailWithError error:NSError) {
print("locationManager error");
}
func saveLatitude(latitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(latitude, forKey: "latitude")
defaults.synchronize()
}
func readLatitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let latitude = defaults.objectForKey("latitude") as! NSString
return latitude;
}
func saveLongitude(longitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(longitude, forKey: "longitude")
defaults.synchronize()
}
func readLongitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let longitude = defaults.objectForKey("longitude") as! NSString
return longitude;
}
func saveCity(city: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(city, forKey: "city")
defaults.synchronize()
}
func readCity() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let city = defaults.objectForKey("city") as! NSString
return city;
}
}
外部调用:
CLLocationModule.sharedInstance().GetLatitudeLongitudeAndCity()
(2)强引用
import UIKit
import CoreLocation
class CLLocationModule: NSObject ,CLLocationManagerDelegate{
var locManager: CLLocationManager!
var latitude:Double?
var longitude:Double?
var city:NSString?
func GetLatitudeLongitudeAndCity(){
if CLLocationManager.locationServicesEnabled() == false {
print("此设备不能定位")
return
}
locManager = CLLocationManager()
locManager.delegate = self
locManager.desiredAccuracy = kCLLocationAccuracyBest
locManager.distanceFilter = 1000.0
//设置定位权限仅ios8有意义
if IS_IOS8 {
locManager.requestWhenInUseAuthorization()// 前台定位
locManager.requestAlwaysAuthorization()
}
locManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
for location in locations {
print("纬度:%g",location.coordinate.latitude);
print("经度:%g",location.coordinate.longitude);
latitude = location.coordinate.latitude
longitude = location.coordinate.longitude
self.saveLatitude(latitude!.description)
self.saveLongitude(longitude!.description)
let geocoder: CLGeocoder = CLGeocoder()
geocoder.reverseGeocodeLocation(location) { (placemarks, error) in
if error != nil {
print("reverse geodcode fail: \(error!.localizedDescription)")
return
}
let pm = placemarks! as [CLPlacemark]
if (pm.count > 0){
for placemark :CLPlacemark in placemarks! {
let placemarkDict = placemark.addressDictionary
let placemarkStr = placemarkDict!["State"]
self.city = placemarkStr?.substringToIndex((placemarkStr?.length)!-1)
self.saveCity(self.city!)
}
}else{
print("No Placemarks!")
}
}
}
manager.stopUpdatingLocation()
}
func locationManager(manager:CLLocationManager, didFailWithError error:NSError) {
print("locationManager error");
}
func saveLatitude(latitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(latitude, forKey: "latitude")
defaults.synchronize()
}
func readLatitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let latitude = defaults.objectForKey("latitude") as! NSString
return latitude;
}
func saveLongitude(longitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(longitude, forKey: "longitude")
defaults.synchronize()
}
func readLongitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let longitude = defaults.objectForKey("longitude") as! NSString
return longitude;
}
func saveCity(city: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(city, forKey: "city")
defaults.synchronize()
}
func readCity() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let city = defaults.objectForKey("city") as! NSString
return city;
}
}
外部调用:
var aCLLocationModule = CLLocationModule()
self.aCLLocationModule.GetLatitudeLongitudeAndCity()
方案:我自定义了一个类模块CLLocationModule.swift
备注以下代码里
let IS_IOS8 = (UIDevice.currentDevice().systemVersion as NSString).doubleValue >= 8.0
最开始的代码
import UIKit
import CoreLocation
class CLLocationModule: NSObject ,CLLocationManagerDelegate{
var latitude:Double?
var longitude:Double?
var city:NSString?
func GetLatitudeLongitudeAndCity(){
if CLLocationManager.locationServicesEnabled() == false {
print("此设备不能定位")
return
}
var locManager = CLLocationManager()
locManager.delegate = self
locManager.desiredAccuracy = kCLLocationAccuracyBest
locManager.distanceFilter = 1000.0
//设置定位权限仅ios8有意义
if IS_IOS8 {
locManager.requestWhenInUseAuthorization()// 前台定位
locManager.requestAlwaysAuthorization()
}
locManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
for location in locations {
print("纬度:%g",location.coordinate.latitude);
print("经度:%g",location.coordinate.longitude);
latitude = location.coordinate.latitude
longitude = location.coordinate.longitude
self.saveLatitude(latitude!.description)
self.saveLongitude(longitude!.description)
let geocoder: CLGeocoder = CLGeocoder()
geocoder.reverseGeocodeLocation(location) { (placemarks, error) in
if error != nil {
print("reverse geodcode fail: \(error!.localizedDescription)")
return
}
let pm = placemarks! as [CLPlacemark]
if (pm.count > 0){
for placemark :CLPlacemark in placemarks! {
let placemarkDict = placemark.addressDictionary
let placemarkStr = placemarkDict!["State"]
self.city = placemarkStr?.substringToIndex((placemarkStr?.length)!-1)
self.saveCity(self.city!)
}
}else{
print("No Placemarks!")
}
}
}
manager.stopUpdatingLocation()
}
func locationManager(manager:CLLocationManager, didFailWithError error:NSError) {
print("locationManager error");
}
func saveLatitude(latitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(latitude, forKey: "latitude")
defaults.synchronize()
}
func readLatitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let latitude = defaults.objectForKey("latitude") as! NSString
return latitude;
}
func saveLongitude(longitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(longitude, forKey: "longitude")
defaults.synchronize()
}
func readLongitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let longitude = defaults.objectForKey("longitude") as! NSString
return longitude;
}
func saveCity(city: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(city, forKey: "city")
defaults.synchronize()
}
func readCity() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let city = defaults.objectForKey("city") as! NSString
return city;
}
}
外部调用是:
CLLocationModule().GetLatitudeLongitudeAndCity()
结果:定位框一闪而过 (允许 不允许那个弹出框) 导致不走代理方法didUpdateLocations 最后查阅网页 知道
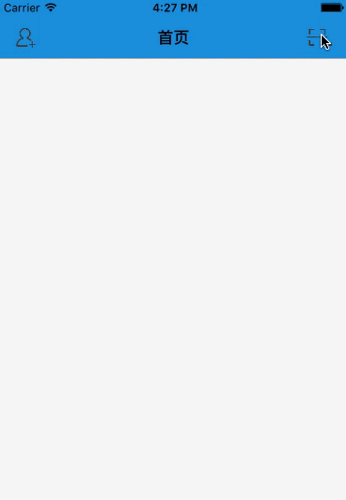
(a)locManager没定义为全局变量
(b)自定义的CLLocationModule.swift这个类 由于ARC的缘故 自动释放了
针对以上(a)问题的解决办法为自定义类CLLocationModule.swift中将var locManager = CLLocationManager() 改为全局变量
var locManager: CLLocationManager!
locManager = CLLocationManager()
针对以上(b)问题的解决方案有两种
(1)外部调用时改为:(改为强引用)
var aCLLocationModule = CLLocationModule()
self.aCLLocationModule.GetLatitudeLongitudeAndCity()
(2)自定义的类CLLocationModule.swift改为单例
//单例
private static let aSharedInstance: CLLocationModule = CLLocationModule()
private override init() {}
class func sharedInstance() -> CLLocationModule {
return aSharedInstance
}
外部调用时改为:
CLLocationModule.sharedInstance().GetLatitudeLongitudeAndCity()
成功的代码附上两份
(1)单例
import UIKit
import CoreLocation
class CLLocationModule: NSObject ,CLLocationManagerDelegate{
//单例
private static let aSharedInstance: CLLocationModule = CLLocationModule()
private override init() {}
class func sharedInstance() -> CLLocationModule {
return aSharedInstance
}
var locManager: CLLocationManager!
var latitude:Double?
var longitude:Double?
var city:NSString?
func GetLatitudeLongitudeAndCity(){
if CLLocationManager.locationServicesEnabled() == false {
print("此设备不能定位")
return
}
locManager = CLLocationManager()
locManager.delegate = self
locManager.desiredAccuracy = kCLLocationAccuracyBest
locManager.distanceFilter = 1000.0
//设置定位权限仅ios8有意义
if IS_IOS8 {
locManager.requestWhenInUseAuthorization()// 前台定位
locManager.requestAlwaysAuthorization()
}
locManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
for location in locations {
print("纬度:%g",location.coordinate.latitude);
print("经度:%g",location.coordinate.longitude);
latitude = location.coordinate.latitude
longitude = location.coordinate.longitude
self.saveLatitude(latitude!.description)
self.saveLongitude(longitude!.description)
let geocoder: CLGeocoder = CLGeocoder()
geocoder.reverseGeocodeLocation(location) { (placemarks, error) in
if error != nil {
print("reverse geodcode fail: \(error!.localizedDescription)")
return
}
let pm = placemarks! as [CLPlacemark]
if (pm.count > 0){
for placemark :CLPlacemark in placemarks! {
let placemarkDict = placemark.addressDictionary
let placemarkStr = placemarkDict!["State"]
self.city = placemarkStr?.substringToIndex((placemarkStr?.length)!-1)
self.saveCity(self.city!)
}
}else{
print("No Placemarks!")
}
}
}
manager.stopUpdatingLocation()
}
func locationManager(manager:CLLocationManager, didFailWithError error:NSError) {
print("locationManager error");
}
func saveLatitude(latitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(latitude, forKey: "latitude")
defaults.synchronize()
}
func readLatitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let latitude = defaults.objectForKey("latitude") as! NSString
return latitude;
}
func saveLongitude(longitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(longitude, forKey: "longitude")
defaults.synchronize()
}
func readLongitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let longitude = defaults.objectForKey("longitude") as! NSString
return longitude;
}
func saveCity(city: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(city, forKey: "city")
defaults.synchronize()
}
func readCity() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let city = defaults.objectForKey("city") as! NSString
return city;
}
}
外部调用:
CLLocationModule.sharedInstance().GetLatitudeLongitudeAndCity()
(2)强引用
import UIKit
import CoreLocation
class CLLocationModule: NSObject ,CLLocationManagerDelegate{
var locManager: CLLocationManager!
var latitude:Double?
var longitude:Double?
var city:NSString?
func GetLatitudeLongitudeAndCity(){
if CLLocationManager.locationServicesEnabled() == false {
print("此设备不能定位")
return
}
locManager = CLLocationManager()
locManager.delegate = self
locManager.desiredAccuracy = kCLLocationAccuracyBest
locManager.distanceFilter = 1000.0
//设置定位权限仅ios8有意义
if IS_IOS8 {
locManager.requestWhenInUseAuthorization()// 前台定位
locManager.requestAlwaysAuthorization()
}
locManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
for location in locations {
print("纬度:%g",location.coordinate.latitude);
print("经度:%g",location.coordinate.longitude);
latitude = location.coordinate.latitude
longitude = location.coordinate.longitude
self.saveLatitude(latitude!.description)
self.saveLongitude(longitude!.description)
let geocoder: CLGeocoder = CLGeocoder()
geocoder.reverseGeocodeLocation(location) { (placemarks, error) in
if error != nil {
print("reverse geodcode fail: \(error!.localizedDescription)")
return
}
let pm = placemarks! as [CLPlacemark]
if (pm.count > 0){
for placemark :CLPlacemark in placemarks! {
let placemarkDict = placemark.addressDictionary
let placemarkStr = placemarkDict!["State"]
self.city = placemarkStr?.substringToIndex((placemarkStr?.length)!-1)
self.saveCity(self.city!)
}
}else{
print("No Placemarks!")
}
}
}
manager.stopUpdatingLocation()
}
func locationManager(manager:CLLocationManager, didFailWithError error:NSError) {
print("locationManager error");
}
func saveLatitude(latitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(latitude, forKey: "latitude")
defaults.synchronize()
}
func readLatitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let latitude = defaults.objectForKey("latitude") as! NSString
return latitude;
}
func saveLongitude(longitude: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(longitude, forKey: "longitude")
defaults.synchronize()
}
func readLongitude() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let longitude = defaults.objectForKey("longitude") as! NSString
return longitude;
}
func saveCity(city: NSString) {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
defaults.setObject(city, forKey: "city")
defaults.synchronize()
}
func readCity() -> NSString {
let defaults: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let city = defaults.objectForKey("city") as! NSString
return city;
}
}
外部调用:
var aCLLocationModule = CLLocationModule()
self.aCLLocationModule.GetLatitudeLongitudeAndCity()
相关文章推荐
- Swift 2.0 异常处理
- Swift中In-Out类型的参数
- Swift中函数返回多值问题
- swift 将闭包声明属性使用
- backBarButtonItem去除title 修改图片 换颜色
- swift中打印一连串的变量,常量
- Swift中的optional
- swift开发笔记25 定时的本地通知
- swift 自定义画渐变色折线图
- swift获取键盘高度
- swift中的willSet与didSet
- swift与OC之间不得不知道的21点
- swift-UITabBarController 设置选中和未选中状态文字图片颜色或使用原图
- Swift--map函数浅析
- Swift - String与NSString的区别,以及各自的使用场景
- Swift - 数字格式化转成字符串(保留两位小数,前面补0等)
- swift 语言的编程范式
- Swift 实例方法&类型方法(九)
- Swift里通知的使用NSNotificationCenter
- Swift解析Json返回值为null的问题