【POJ 1691】 Painting A Board(dfs)
2016-03-25 20:10
447 查看
[align=center]【POJ 1691】 Painting A Board(dfs)[/align]
Description
The CE digital company has built an Automatic Painting Machine (APM) to paint a flat board fully covered by adjacent non-overlapping rectangles of different sizes each with a predefined color.
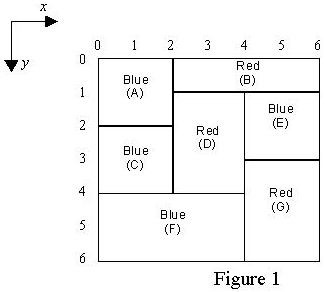
To color the board, the APM has access to a set of brushes. Each brush has a distinct color C. The APM picks one brush with color C and paints all possible rectangles having predefined color C with the following restrictions:
To avoid leaking the paints and mixing colors, a rectangle can only be painted if all rectangles immediately above it have already been painted. For example rectangle labeled F in Figure 1 is painted only after rectangles C and D are painted. Note that each
rectangle must be painted at once, i.e. partial painting of one rectangle is not allowed.
You are to write a program for APM to paint a given board so that the number of brush pick-ups is minimum. Notice that if one brush is picked up more than once, all pick-ups are counted.
Input
The first line of the input file contains an integer M which is the number of test cases to solve (1 <= M <= 10). For each test case, the first line contains an integer N, the number of rectangles, followed by N lines describing
the rectangles. Each rectangle R is specified by 5 integers in one line: the y and x coordinates of the upper left corner of R, the y and x coordinates of the lower right corner of R, followed by the color-code of R.
Note that:
Color-code is an integer in the range of 1 .. 20.
Upper left corner of the board coordinates is always (0,0).
Coordinates are in the range of 0 .. 99.
N is in the range of 1..15.
Output
One line for each test case showing the minimum number of brush pick-ups.
Sample Input
Sample Output
Source
Tehran 1999
题目大意:有一块画布,被分割成了n块矩形,每块矩形有自己的颜色,可能有某些部分颜色相同。
现在要对画布涂色,对应位置要涂成对应的颜色。
对要进行涂色的矩形有如下要求:在它上方的矩形必须已经被涂完。
每当选择一种颜色时,可以对所有要涂这种颜色且可以涂色的区域涂色。当切换另一种眼色时,上一种颜色会被清洗掉。
问最少需要多少色料(每次涂同种颜色时用掉一份色料
由于颜色最多只有20种。
可以把所有矩形区域存下,然后dfs搜索所有涂色顺序。
并且要记得对矩形排个序,以免先访问下面的矩形,可能此时无法对其涂色,但访问到其上面的矩形时,如果能涂色,该矩形也就能涂色了。
所以要提前从上往下按坐标排个序。
对于涂色,没有必要整个矩形区域涂色,只需要涂它的下界。因为只有这个才是有用的标记(下方的矩形需要依靠上方矩形的下界来判断是否可以被涂色
代码如下:
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 3582 | Accepted: 1781 |
The CE digital company has built an Automatic Painting Machine (APM) to paint a flat board fully covered by adjacent non-overlapping rectangles of different sizes each with a predefined color.
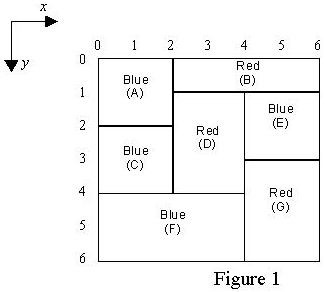
To color the board, the APM has access to a set of brushes. Each brush has a distinct color C. The APM picks one brush with color C and paints all possible rectangles having predefined color C with the following restrictions:
To avoid leaking the paints and mixing colors, a rectangle can only be painted if all rectangles immediately above it have already been painted. For example rectangle labeled F in Figure 1 is painted only after rectangles C and D are painted. Note that each
rectangle must be painted at once, i.e. partial painting of one rectangle is not allowed.
You are to write a program for APM to paint a given board so that the number of brush pick-ups is minimum. Notice that if one brush is picked up more than once, all pick-ups are counted.
Input
The first line of the input file contains an integer M which is the number of test cases to solve (1 <= M <= 10). For each test case, the first line contains an integer N, the number of rectangles, followed by N lines describing
the rectangles. Each rectangle R is specified by 5 integers in one line: the y and x coordinates of the upper left corner of R, the y and x coordinates of the lower right corner of R, followed by the color-code of R.
Note that:
Color-code is an integer in the range of 1 .. 20.
Upper left corner of the board coordinates is always (0,0).
Coordinates are in the range of 0 .. 99.
N is in the range of 1..15.
Output
One line for each test case showing the minimum number of brush pick-ups.
Sample Input
1 7 0 0 2 2 1 0 2 1 6 2 2 0 4 2 1 1 2 4 4 2 1 4 3 6 1 4 0 6 4 1 3 4 6 6 2
Sample Output
3
Source
Tehran 1999
题目大意:有一块画布,被分割成了n块矩形,每块矩形有自己的颜色,可能有某些部分颜色相同。
现在要对画布涂色,对应位置要涂成对应的颜色。
对要进行涂色的矩形有如下要求:在它上方的矩形必须已经被涂完。
每当选择一种颜色时,可以对所有要涂这种颜色且可以涂色的区域涂色。当切换另一种眼色时,上一种颜色会被清洗掉。
问最少需要多少色料(每次涂同种颜色时用掉一份色料
由于颜色最多只有20种。
可以把所有矩形区域存下,然后dfs搜索所有涂色顺序。
并且要记得对矩形排个序,以免先访问下面的矩形,可能此时无法对其涂色,但访问到其上面的矩形时,如果能涂色,该矩形也就能涂色了。
所以要提前从上往下按坐标排个序。
对于涂色,没有必要整个矩形区域涂色,只需要涂它的下界。因为只有这个才是有用的标记(下方的矩形需要依靠上方矩形的下界来判断是否可以被涂色
代码如下:
#include <iostream> #include <cmath> #include <vector> #include <cstdlib> #include <cstdio> #include <cstring> #include <queue> #include <stack> #include <list> #include <algorithm> #include <map> #include <set> #define LL long long #define Pr pair<int,int> #define fread() freopen("in.in","r",stdin) #define fwrite() freopen("out.out","w",stdout) using namespace std; const int INF = 0x3f3f3f3f; const int msz = 10000; const int mod = 1e9+7; const double eps = 1e-8; struct Range { int x1,y1,x2,y2,c; bool f; bool operator < (const struct Range r)const { return x1 == r.x1? y1 < r.y1: x1 < r.x1; } }; Range rg[33]; bool mp[111][111]; int n,mn; void col(int pos) { for(int i = rg[pos].y1; i <= rg[pos].y2; ++i) mp[rg[pos].x2][i] = 1; } bool can(int pos) { if(rg[pos].x1 == 0) return true; for(int i = rg[pos].y1; i <= rg[pos].y2; ++i) if(!mp[rg[pos].x1][i]) return false; return true; } bool cal(int c,int *tmp,int &tp) { tp = 0; for(int i = 0; i < n; ++i) { if(rg[i].f || rg[i].c != c) continue; if(can(i)) { rg[i].f = 1; tmp[tp++] = i; col(i); } } return tp != 0; } void reset(int *tmp,int tp) { for(int i = 0; i < tp; ++i) { rg[tmp[i]].f = 0; for(int j = rg[tmp[i]].y1; j <= rg[tmp[i]].y2; ++j) mp[rg[tmp[i]].x2][j] = 0; } } void dfs(int ok,int us) { if(ok == n) { mn = min(mn,us); return; } int tmp[33]; int tp; for(int i = 1; i <= 20; ++i) { if(!cal(i,tmp,tp)) continue; //printf("%d->%d %d %d\n",ok,ok+tp,us,i); dfs(ok+tp,us+1); reset(tmp,tp); } } int main() { //fread(); //fwrite(); int t; scanf("%d",&t); while(t--) { scanf("%d",&n); for(int i = 0; i < n; ++i) { scanf("%d%d%d%d%d",&rg[i].x1,&rg[i].y1,&rg[i].x2,&rg[i].y2,&rg[i].c); rg[i].f = 0; } sort(rg,rg+n); mn = INF; memset(mp,0,sizeof(mp)); dfs(0,0); printf("%d\n",mn); } return 0; }
相关文章推荐
- [LeetCode]Palindrome Pairs
- PAT (Advanced Level) Practise 1090 Highest Price in Supply Chain (25)
- 主线程MainThread与渲染线程RenderThread
- http://www.cnblogs.com/hnrainll/archive/2011/12/29/2305582.html
- 【POJ 3411】 Paid Roads(搜索/DP)
- dcmtk进行movescu提示:Failed: IdentifierDoesNotMatchSOPClass
- 22. 使用 awk / grep / head / tail 命令进行文本 / 日志分析 (/home/D/acc.log)
- 配置rewrite
- The Responder Chain(响应链)
- leetcode-11 Container With Most Water
- EXPLAIN
- MacBook Air密码忘了,苹果电脑密码忘了怎么办
- Base Class Doesn't Contain Parameterless Constructor?
- PAT (Advanced Level) Practise 1086 Tree Traversals Again (25)
- Step by Step - Exchange 2013 Email Message Size Restriction Detail
- activity_main.xml解析
- ResourceManager High Availability
- [LeetCode] Paint House 粉刷房子
- c 程序必须要从main函数开始执行么?
- HDU 1104 Remainder(BFS 同余定理)