三、插入排序
2016-03-18 19:07
423 查看
1、算法原理
插入排序是将一个数据插入到已经排好序的有序数据中,从而得到一个新的、个数加一的有序数据。此算法适用于少量数据的排序,时间复杂度为O(n^2),空间复杂度为O(1)。插入排序是稳定的排序算法。
2、代码实现
(1)JAVA实现
注:本程序设计生成10个随机数,再对这10个随机数进行从小到大的插入排序
/*
* 排序算法:插入排序
* 作者:xiaoxiao
* 日期:2016-03-17
*/
import java.util.Random;
public class InsertSort {
public
static void main(String[]
args){
int[]
arr = new int[10];//定义可以存10个数的数组
int[]
rs = new int[10];
init(arr);
System.out.print("排序前:");
for(int
i=0; i<arr.length;
i++){
System.out.print(arr[i]+", ");
}
System.out.println();
rs = insertSort(arr);
System.out.print("排序后:");
for(int
i=0; i<rs.length;
i++){
System.out.print(rs[i]+", ");
}
}
public
static void init(int[]
a){
Random r =
new Random();
for(int
i=0; i<a.length;
i++){
a[i] =
r.nextInt(100);
}
}
public
static int[] insertSort(int[]
a){
if(a==null ||
a.length<2){
return
a;
}
int
tmp;
for(int
i=1; i<a.length;
i++){
for(int
j=i;
j>0;
j--){ //对前i-1个数据进行逐一遍历比较,将第i个数插入到恰当位置
if(a[j] <
a[j-1]){
tmp =
a[j];
a[j] =
a[j-1];
a[j-1] =
tmp;
}
else{
break;
}
}
}
return
a;
}
}
运行结果:

(2)PHP实现
注:本程序设计随机生成10~20个1~100的数,再对这些数进行从小到大的插入排序
<!--
Method:插入排序
Author: xiaoxiao
Date: 2016-03-17
-->
<?php
echo "<meta http-equiv='Content-Type' content='text/html; charset=utf-8' />";//如果涉及到PHP网页中文乱码问题,加上该行
function InsertSort($a){
$length = count($a);
for($i=1; $i<$length; $i++){
for ($j=$i; $j>0; $j--) { //对第i+1~$length的数字进行查找,找到最小数字对应的坐标
if ($a[$j]<$a[$j-1]) {
$temp = $a[$j];
$a[$j] = $a[$j-1];
$a[$j-1] = $temp;
}
else{
break;
}
}
}
return $a;
}
$a = array();
$nums = mt_rand(10,20);
for ($i=0; $i < $nums; $i++) {
array_push($a, mt_rand(1,100));
}
echo "待排序的数有".$nums."个!<br />";
echo "排序前: ";
for($i=0; $i<count($a); $i++){
echo $a[$i];
echo ", ";
}
echo "<br />";
echo "排序后: ";
$b = InsertSort($a);
for($i=0; $i<count($b); $i++){
echo $b[$i];
echo ", ";
}
?>
运行结果:
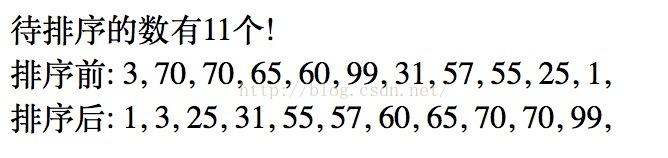
插入排序是将一个数据插入到已经排好序的有序数据中,从而得到一个新的、个数加一的有序数据。此算法适用于少量数据的排序,时间复杂度为O(n^2),空间复杂度为O(1)。插入排序是稳定的排序算法。
2、代码实现
(1)JAVA实现
注:本程序设计生成10个随机数,再对这10个随机数进行从小到大的插入排序
/*
* 排序算法:插入排序
* 作者:xiaoxiao
* 日期:2016-03-17
*/
import java.util.Random;
public class InsertSort {
public
static void main(String[]
args){
int[]
arr = new int[10];//定义可以存10个数的数组
int[]
rs = new int[10];
init(arr);
System.out.print("排序前:");
for(int
i=0; i<arr.length;
i++){
System.out.print(arr[i]+", ");
}
System.out.println();
rs = insertSort(arr);
System.out.print("排序后:");
for(int
i=0; i<rs.length;
i++){
System.out.print(rs[i]+", ");
}
}
public
static void init(int[]
a){
Random r =
new Random();
for(int
i=0; i<a.length;
i++){
a[i] =
r.nextInt(100);
}
}
public
static int[] insertSort(int[]
a){
if(a==null ||
a.length<2){
return
a;
}
int
tmp;
for(int
i=1; i<a.length;
i++){
for(int
j=i;
j>0;
j--){ //对前i-1个数据进行逐一遍历比较,将第i个数插入到恰当位置
if(a[j] <
a[j-1]){
tmp =
a[j];
a[j] =
a[j-1];
a[j-1] =
tmp;
}
else{
break;
}
}
}
return
a;
}
}
运行结果:
(2)PHP实现
注:本程序设计随机生成10~20个1~100的数,再对这些数进行从小到大的插入排序
<!--
Method:插入排序
Author: xiaoxiao
Date: 2016-03-17
-->
<?php
echo "<meta http-equiv='Content-Type' content='text/html; charset=utf-8' />";//如果涉及到PHP网页中文乱码问题,加上该行
function InsertSort($a){
$length = count($a);
for($i=1; $i<$length; $i++){
for ($j=$i; $j>0; $j--) { //对第i+1~$length的数字进行查找,找到最小数字对应的坐标
if ($a[$j]<$a[$j-1]) {
$temp = $a[$j];
$a[$j] = $a[$j-1];
$a[$j-1] = $temp;
}
else{
break;
}
}
}
return $a;
}
$a = array();
$nums = mt_rand(10,20);
for ($i=0; $i < $nums; $i++) {
array_push($a, mt_rand(1,100));
}
echo "待排序的数有".$nums."个!<br />";
echo "排序前: ";
for($i=0; $i<count($a); $i++){
echo $a[$i];
echo ", ";
}
echo "<br />";
echo "排序后: ";
$b = InsertSort($a);
for($i=0; $i<count($b); $i++){
echo $b[$i];
echo ", ";
}
?>
运行结果:
相关文章推荐
- 一个关于if else容易迷惑的问题
- java对世界各个时区(TimeZone)的通用转换处理方法(转载)
- java-注解annotation
- java-模拟tomcat服务器
- java-用HttpURLConnection发送Http请求.
- java-WEB中的监听器Lisener
- Android IPC进程间通讯机制
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- 介绍一款信息管理系统的开源框架---jeecg
- 聚类算法之kmeans算法java版本
- java实现 PageRank算法
- PHP5.2.*防止Hash冲突拒绝服务攻击的Patch
- 深入理解PHP之匿名函数
- PropertyChangeListener简单理解
- JSP/PHP基于Ajax的分页功能实现
- c++11 + SDL2 + ffmpeg +OpenAL + java = Android播放器