Fragment+ViewPager使用示例
2016-03-10 08:54
471 查看
很多app都采用了底部(或者顶部)Tab,中间ViewPager,点击底部Tab可以切换到对应页,左右滑动页面也会自动改变Tab点亮的设计。不用说,用ViewPager实现。
ViewPager可以看成一个容器,里面放了View或者Fragment,支持左右切换容器里的内容。使用Fragment+ViewPager的优势就是,可以在每个Fragment里独立的编写逻辑,而不用全部挤在MainActivity中。
先来看一个简单的效果图。
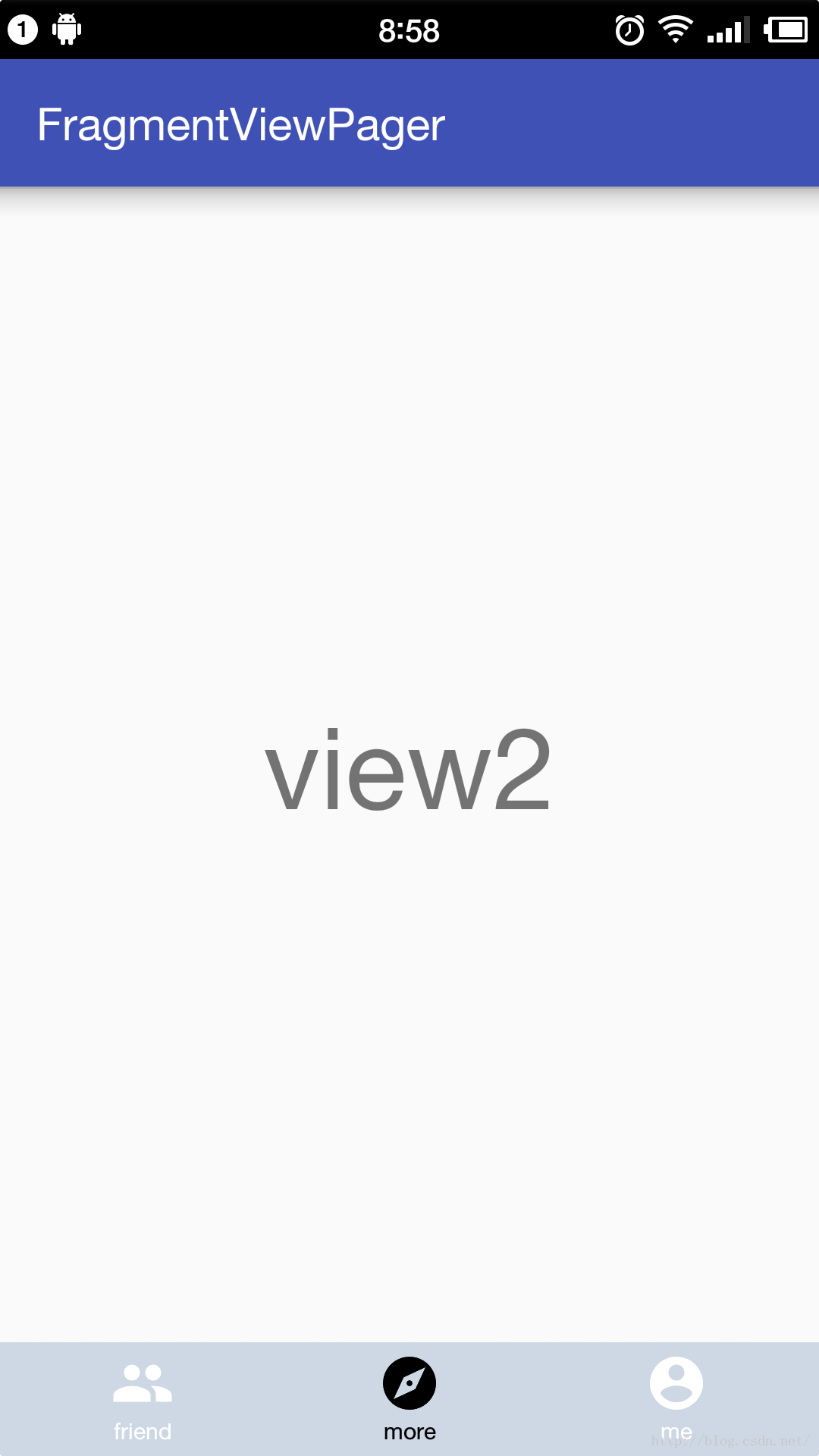
首先,底部的Tag是由三个ImageView+TextView的LinearLa构成的,默认颜色都为白色
主布局文件中间整块ViewPager(这里用了v4下的包),底部把刚才的Tab给include进来。
这里如果直接把ViewPager高度设置为match_parent会把底部Tab挤出屏幕外,所以巧妙地用了weight属性,使它占满剩余的所有空间。
然后就是MainActivity了,逻辑很清楚,用适配器将要显示的Fragment数组加载到ViewPager里,set两个监听器实现滑动和点击都能改变Tab点亮的效果。
完成。
ViewPager可以看成一个容器,里面放了View或者Fragment,支持左右切换容器里的内容。使用Fragment+ViewPager的优势就是,可以在每个Fragment里独立的编写逻辑,而不用全部挤在MainActivity中。
先来看一个简单的效果图。
首先,底部的Tag是由三个ImageView+TextView的LinearLa构成的,默认颜色都为白色
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="50dp" android:background="#CDD8E4" android:padding="4dp"> <LinearLayout android:id="@+id/friend" android:layout_width="0dp" android:layout_height="match_parent" android:layout_weight="1" android:orientation="vertical"> <ImageView android:id="@+id/image_friend" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="2" android:src="@drawable/friend_white" /> <TextView android:id="@+id/text_friend" android:layout_width="match_parent" android:layout_height="0dp" android:gravity="center" android:layout_gravity="center" android:layout_weight="1" android:text="friend" android:textSize="10sp" android:textColor="#ffffff" /> </LinearLayout> <LinearLayout android:id="@+id/more" android:layout_width="0dp" android:layout_height="match_parent" android:layout_weight="1" android:orientation="vertical"> <ImageView android:id="@+id/image_more" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="2" android:src="@drawable/more_white" /> <TextView android:id="@+id/text_more" android:layout_width="match_parent" android:layout_height="0dp" android:gravity="center" android:layout_gravity="center" android:layout_weight="1" android:text="more" android:textSize="10sp" android:textColor="#ffffff" /> </LinearLayout> <LinearLayout android:id="@+id/me" android:layout_width="0dp" android:layout_height="match_parent" android:layout_weight="1" android:orientation="vertical"> <ImageView android:id="@+id/image_me" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="2" android:src="@drawable/me_white" /> <TextView android:id="@+id/text_me" android:layout_width="match_parent" android:layout_height="0dp" android:gravity="center" android:layout_gravity="center" android:layout_weight="1" android:text="me" android:textSize="10sp" android:textColor="#ffffff" /> </LinearLayout> </LinearLayout>
主布局文件中间整块ViewPager(这里用了v4下的包),底部把刚才的Tab给include进来。
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <android.support.v4.view.ViewPager android:id="@+id/id_viewPager" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1"> </android.support.v4.view.ViewPager> <include layout="@layout/bottom_tab" /> </LinearLayout>
这里如果直接把ViewPager高度设置为match_parent会把底部Tab挤出屏幕外,所以巧妙地用了weight属性,使它占满剩余的所有空间。
然后就是MainActivity了,逻辑很清楚,用适配器将要显示的Fragment数组加载到ViewPager里,set两个监听器实现滑动和点击都能改变Tab点亮的效果。
public class MainActivity extends AppCompatActivity implements View.OnClickListener, ViewPager.OnPageChangeListener { private List<Fragment> fragments = new ArrayList<>(); private ViewPager viewPager; private ImageView imageFriend, imageMore, imageMe; private TextView textFriend, textMore, textMe; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); init(); } private void init() { viewPager = (ViewPager) findViewById(R.id.id_viewPager); fragments.add(new Fragment1()); fragments.add(new Fragment2()); fragments.add(new Fragment3()); /*new一个自定义的继承自FragmentStatePagerAdapter的适配器*/ MyAdapter myAdapter = new MyAdapter(getSupportFragmentManager(), fragments); viewPager.setAdapter(myAdapter); viewPager.addOnPageChangeListener(this); /*底下的Tab只用来监听点击事件,所以也不显示的创建了,直接用for循环添加监听器*/ int[] tabIds = new int[]{R.id.friend,R.id.more,R.id.me}; for (int tabId : tabIds) { findViewById(tabId).setOnClickListener(this); } /*Tab里具体的图片和文字都需要动态改变,在这里创建出来*/ imageFriend = (ImageView) findViewById(R.id.image_friend); imageMore = (ImageView) findViewById(R.id.image_more); imageMe = (ImageView) findViewById(R.id.image_me); textFriend = (TextView) findViewById(R.id.text_friend); textMore = (TextView) findViewById(R.id.text_more); textMe = (TextView) findViewById(R.id.text_me); /*一开始的时候就会有一个当前显示页,将对应的Tab设置点亮*/ setTab(viewPager.getCurrentItem()); } private void setTab(int currentItem) { switch (currentItem) { case 0: imageFriend.setImageResource(R.drawable.friend_black); textFriend.setTextColor(Color.BLACK); break; case 1: imageMore.setImageResource(R.drawable.more_black); textMore.setTextColor(Color.BLACK); break; case 2: imageMe.setImageResource(R.drawable.me_black); textMe.setTextColor(Color.BLACK); break; } } @Override public void onClick(View v) { /*无论点击谁,先将他们全部熄灭,再根据具体点击的Tab改变ViewPage页和点亮Tab*/ initTab(); switch (v.getId()) { case R.id.friend: viewPager.setCurrentItem(0); imageFriend.setImageResource(R.drawable.friend_black); textFriend.setTextColor(Color.BLACK); break; case R.id.more: viewPager.setCurrentItem(1); imageMore.setImageResource(R.drawable.more_black); textMore.setTextColor(Color.BLACK); break; case R.id.me: viewPager.setCurrentItem(3); imageMe.setImageResource(R.drawable.me_black); textMe.setTextColor(Color.BLACK); break; } } private void initTab() { imageFriend.setImageResource(R.drawable.friend_white); imageMore.setImageResource(R.drawable.more_white); imageMe.setImageResource(R.drawable.me_white); textFriend.setTextColor(Color.WHITE); textMore.setTextColor(Color.WHITE); textMe.setTextColor(Color.WHITE); } @Override public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) { } @Override public void onPageSelected(int position) { /*滑动改变ViewPage页后也相应设置Tab变化*/ initTab(); setTab(position); } @Override public void onPageScrollStateChanged(int state) { } }
public class MyAdapter extends FragmentStatePagerAdapter{ List<Fragment>fragments; public MyAdapter(FragmentManager fm,List<Fragment>fragments) { super(fm); this.fragments = fragments; } @Override public Fragment getItem(int position) { return fragments.get(position); } @Override public int getCount() { return fragments.size(); } /*当包含的Fragment较多时可以用instantiateItem()和destroyItem进行管理*/ }
完成。
相关文章推荐
- 使用ViewPager实现高仿launcher左右拖动效果
- 灵活使用Android中ActionBar和ViewPager切换页面
- Android中viewPager使用指南
- Android 使用 ViewPager循环广告位的实现
- Android viewpager中动态添加view并实现伪无限循环的方法
- 实现轮转广告带底部指示的自定义ViewPager控件
- 自定义RadioButton和ViewPager实现TabHost带滑动的页卡效果
- Android基于ViewPager Fragment实现选项卡
- 使用ViewPager实现android软件使用向导功能实现步骤
- Android自定义ViewPager实例
- Android编程ViewPager回弹效果实例分析
- Android 使用ViewPager自动滚动循环轮播效果
- Android ViewPager相册横向移动的实现方法
- Android 使用viewpager实现无限循环(定时+手动)
- Android ViewPager实现无限循环效果
- Android编程实现ListView头部ViewPager广告轮询图效果
- Android 利用ViewPager实现图片可以左右循环滑动效果附代码下载
- Android编程开发ScrollView中ViewPager无法正常滑动问题解决方法
- 使用ViewPager+Fragment实现顶部导航栏
- viewpager的layout_width="wrap_content"无效问题