Android消息机制不完全解析(上) .
2016-03-04 19:26
519 查看
转自:http://blog.csdn.net/a220315410/article/details/9857225?utm_source=tuicool&utm_medium=referral
Handler和Message是Android开发者常用的两个API,我一直对于它的内部实现比较好奇,所以用空闲的时间,阅读了一下他们的源码。
相关的Java Class:
android.os.Message
android.os.MessageQueue
android.os.Looper
android.os.Handler
相关的C++ Class:
android.NativeMessageQueue
android.Looper
android.LooperCallback
android.SimpleLooperCallback
android.Message
android.MessageHandler
首先,来看看这些类之间的关系:
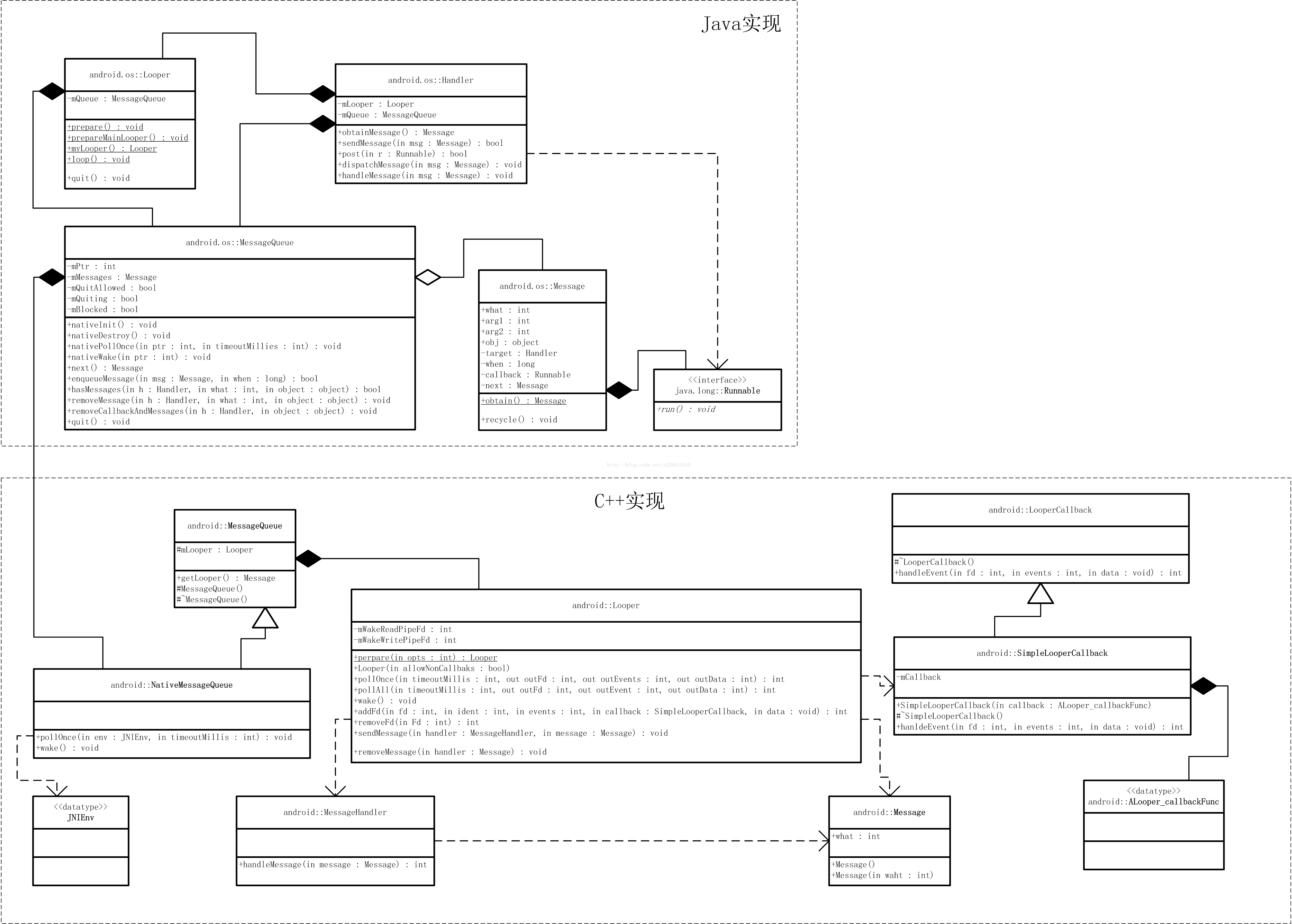
首先,让我们从相对简单的java实现开始看起:
[java]
view plain
copy
print?
public int what;//用以表示消息类别
public int arg1;//消息数据
public int arg2;//消息数据
public Object obj;//消息数据
[java]
view plain
copy
print?
/*package*/ long when;//消息应该被处理的时间
/*package*/ Bundle data;//消息数据
/*package*/ Handler target;//处理这个消息的handler
/*package*/ Runnable callback;//回调函数
// sometimes we store linked lists of these things
/*package*/ Message next;//形成链表,保存Message实例
值得一提的是,Android提供了一个简单,但是有用的消息池,对于Message这种使用频繁的类型,可以有效的减少内存申请和释放的次数,提高性能。
[java]
view plain
copy
print?
private static final Object sPoolSync = new Object();
private static Message sPool;
private static int sPoolSize = 0;
private static final int MAX_POOL_SIZE = 50;
[java]
view plain
copy
print?
/**
* Return a new Message instance from the global pool. Allows us to
* avoid allocating new objects in many cases.
*/
public static Message obtain() {
synchronized (sPoolSync) {
if (sPool != null) {//消息池不为空,则从消息池中获取实例
Message m = sPool;
sPool = m.next;
m.next = null;
sPoolSize--;
return m;
}
}
return new Message();
}
/**
* Return a Message instance to the global pool. You MUST NOT touch
* the Message after calling this function -- it has effectively been
* freed.
*/
public void recycle() {
clearForRecycle();
synchronized (sPoolSync) {
if (sPoolSize < MAX_POOL_SIZE) {//消息池大小未满,则放入消息池
next = sPool;
sPool = this;
sPoolSize++;
}
}
}
/*package*/ void clearForRecycle() {
flags = 0;
what = 0;
arg1 = 0;
arg2 = 0;
obj = null;
replyTo = null;
when = 0;
target = null;
callback = null;
data = null;
}
小结:
Message的核心在于它的数据域,Handler根据这些内容来识别和处理消息
应该使用Message.obtain(或者Handler.obtainMessage)函数获取message实例
[java]
view plain
copy
print?
public interface Callback {
public boolean handleMessage(Message msg);
}
[java]
view plain
copy
print?
public Handler() {
this(null, false);
}
[java]
view plain
copy
print?
public Handler(Callback callback, boolean async) {
if (FIND_POTENTIAL_LEAKS) {
final Class<? extends Handler> klass = getClass();
if ((klass.isAnonymousClass() || klass.isMemberClass() || klass.isLocalClass()) &&
(klass.getModifiers() & Modifier.STATIC) == 0) {
Log.w(TAG, "The following Handler class should be static or leaks might occur: " +
klass.getCanonicalName());
}
}
mLooper = Looper.myLooper();
if (mLooper == null) {
throw new RuntimeException(
"Can't create handler inside thread that has not called Looper.prepare()");
}
mQueue = mLooper.mQueue;
mCallback = callback; //使用Callback可以拦截Handler处理消息,之后会在dispatchMessage函数中,大展身手
mAsynchronous = async;//设置handler的消息为异步消息,暂时先无视这个变量
}
Handler的构造函数最主要的就是初始化成员变量:mLooper和mQueue。 这边需要注意的一个问题是:Looper.myLooper()不能返回null,否则抛出RuntimeExeception。稍后详解Looper.myLooper();函数在何种情况下会抛出异常。
Handler.obtainMessage系列的函数都会调用Message类中对应的静态方法,从消息池中获取一个可用的消息实例。典型实现如下:
[java]
view plain
copy
print?
public final Message obtainMessage()
{
return Message.obtain(this);
}
Handler.post系列和send系列函数最终都会调用enqueueMessage函数,把message入列,不同之处在于post系列函数会以Runable参数构建一个Message实例。
[java]
view plain
copy
print?
private static Message getPostMessage(Runnable r) {
Message m = Message.obtain();
m.callback = r;//一会我们会看到callback非空的message和callback为空的mesage在处理时的差异
return m;
}
public final boolean post(Runnable r)
{
return sendMessageDelayed(getPostMessage(r), 0);
}
public final boolean sendMessage(Message msg)
{
return sendMessageDelayed(msg, 0);
}
public final boolean sendMessageDelayed(Message msg, long delayMillis)
{
if (delayMillis < 0) {
delayMillis = 0;
}
return sendMessageAtTime(msg, SystemClock.uptimeMillis() + delayMillis);
}
public boolean sendMessageAtTime(Message msg, long uptimeMillis) {
MessageQueue queue = mQueue;
if (queue == null) {
RuntimeException e = new RuntimeException(
this + " sendMessageAtTime() called with no mQueue");
Log.w("Looper", e.getMessage(), e);
return false;
}
return enqueueMessage(queue, msg, uptimeMillis);
}
//最终都会调用这个函数,把message入列
private boolean enqueueMessage(MessageQueue queue, Message msg, long uptimeMillis) {
msg.target = this;
if (mAsynchronous) {
msg.setAsynchronous(true);//Handler的<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">mAsynchronous属性,决定了msg是否为asynchronous,稍后在MessageQueue.next函数中,可以看到</SPAN><SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">asynchronous对于消息处理的影响</SPAN><SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">
AN> }
return queue.enqueueMessage(msg, uptimeMillis);
}
接下来主角登场,Handler.dispatchMessage:
[java]
view plain
copy
print?
private static void handleCallback(Message message) {
message.callback.run();
}
/**
* Subclasses must implement this to receive messages.
*/
public void handleMessage(Message msg) {
}
/**
* Handle system messages here.
*/
public void dispatchMessage(Message msg) {
if (msg.callback != null) {//message的callback不为null,则执行
handleCallback(msg);
} else {
if (mCallback != null) {//如果Hanlder的mCallback成员不为null,则调用
if (mCallback.handleMessage(msg)) {//如果handleMessage返回值为true,则拦截消息
return;
}
}
handleMessage(msg);//处理消息
}
}
注释应该比较清楚,不多说。 小结:
Handler类最为核心的函数是enqueueMessage和dispatcherMessage,前者把待处理的消息放入MessageQueue,而Looper调用后者来处理从MessageQueue获取的消息。
callback不为null(通过post系列函数添加到消息队列中)的message无法被拦截,而callback为null的函数可以被Handler的mCallback拦截
[java]
view plain
copy
print?
private Looper(boolean quitAllowed) {
mQueue = new MessageQueue(quitAllowed);//每个Looper有一个MessageQueue
mRun = true;
mThread = Thread.currentThread();
}
[java]
view plain
copy
print?
** Initialize the current thread as a looper.
* This gives you a chance to create handlers that then reference
* this looper, before actually starting the loop. Be sure to call
* {@link #loop()} after calling this method, and end it by calling
* {@link #quit()}.
*/
public static void prepare() {
prepare(true);//后台线程的looper都允许退出
}
private static void prepare(boolean quitAllowed) {
if (sThreadLocal.get() != null) {
throw new RuntimeException("Only one Looper may be created per thread");//每个线程只能有一个Looper
}
sThreadLocal.set(new Looper(quitAllowed));//把实例保存到TLS(Thread Local Save),仅有每个线程访问自己的Looper
}
/**
* Initialize the current thread as a looper, marking it as an
* application's main looper. The main looper for your application
* is created by the Android environment, so you should never need
* to call this function yourself. See also: {@link #prepare()}
*/
public static void prepareMainLooper() {
prepare(false);//主线程的lopper不可以退出
synchronized (Looper.class) {
if (sMainLooper != null) {
throw new IllegalStateException("The main Looper has already been prepared.");
}
sMainLooper = myLooper();
}
}
好了,Looper的实例是构造出来,但是如何获取构造出来的实例呢?
[java]
view plain
copy
print?
/** Returns the application's main looper, which lives in the main thread of the application.
*/
public static Looper getMainLooper() {
synchronized (Looper.class) {
return sMainLooper;
}
}
/**
* Return the Looper object associated with the current thread. Returns
* null if the calling thread is not associated with a Looper.
*/
public static Looper myLooper() {
return sThreadLocal.get();
}
然后,重头戏来了:
[java]
view plain
copy
print?
/**
* Quits the looper.
*
* Causes the {@link #loop} method to terminate as soon as possible.
*/
public void quit() {
mQueue.quit();
}
/**
* Run the message queue in this thread. Be sure to call
* {@link #quit()} to end the loop.
*/
public static void loop() {
final Looper me = myLooper();
if (me == null) {//调用looper之前,需要先调用perpare,否则您懂的...
throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread.");
}
final MessageQueue queue = me.mQueue;
// Make sure the identity of this thread is that of the local process,
// and keep track of what that identity token actually is.
Binder.clearCallingIdentity();//不太明白这个函数,但不是重点可以无视
final long ident = Binder.clearCallingIdentity();
for (;;) {
Message msg = queue.next(); // might block 获取一个下一个消息,如果当前没有要处理的消息,则block,之后我们会看到这个API的实现
if (msg == null) {//调用了MessgeQueu的quit函数后,MessageQueue.next会返回null
// No message indicates that the message queue is quitting.
return;
}
// This must be in a local variable, in case a UI event sets the logger
Printer logging = me.mLogging;
if (logging != null) {//借助logging我们可以打印Looper中处理的消息
logging.println(">>>>> Dispatching to " + msg.target + " " +
msg.callback + ": " + msg.what);
}
msg.target.dispatchMessage(msg);//调用handler处理消息
if (logging != null) {
logging.println("<<<<< Finished to " + msg.target + " " + msg.callback);
}
// Make sure that during the course of dispatching the
// identity of the thread wasn't corrupted.
final long newIdent = Binder.clearCallingIdentity();//选择性无视
if (ident != newIdent) {
Log.wtf(TAG, "Thread identity changed from 0x"
+ Long.toHexString(ident) + " to 0x"
+ Long.toHexString(newIdent) + " while dispatching to "
+ msg.target.getClass().getName() + " "
+ msg.callback + " what=" + msg.what);
}
msg.recycle();//回收消息到消息池
}
}
从MessageQueue中获取一个消息,当前没有消息需要处理时,则block
调用message的Handler(target)处理消息
基本上,我们可以把Looper理解为一个死循环,Looper开始work以后,线程就进入了以消息为驱动的工作模型。
小结:
每个线程最多可以有一个Looper。
每个Looper有且仅有一个MessageQueue
每个Handler关联一个MessageQueue,由该MessageQueue关联的Looper执行(调用Hanlder.dispatchMessage)
每个MessageQueue可以关联任意多个Handler
Looper API的调用顺序:Looper.prepare >> Looper.loop >> Looper.quit
Looper的核心函数是Looper.loop,一般loop不会返回,直到线程退出,所以需要线程完成某个work时,请发送消息给Message(或者说Handler)
MessageQueue类是唯一包含native函数的类,我们先大致看一下,稍后C++的部分在详细解释:
[java]
view plain
copy
print?
private native void nativeInit(); //初始化
private native void nativeDestroy(); //销毁
private native void nativePollOnce(int ptr, int timeoutMillis); //等待<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">timeoutMillis指定的时间</SPAN>
private native void nativeWake(int ptr);//唤醒<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">nativePollOnce的等待</SPAN>
然后,我们再从构造函数看起:
[java]
view plain
copy
print?
Message mMessages;//数据域mMessages的类型虽然是Message,但是因为Message.next数据域的原因,其实mMessage是链表的第一个元素
MessageQueue(boolean quitAllowed) {
mQuitAllowed = quitAllowed;
nativeInit();//初始化nativeMessageQueue
}
[java]
view plain
copy
print?
@Override
protected void finalize() throws Throwable {
try {
nativeDestroy();//销毁nativeMessageQueue
} finally {
super.finalize();
}
}
此外,MessageQueue提供了一组函数(e.g. hasMessage, removeMessage)来查询和移除待处理的消息,我们在前面的Handler类上看到的对应函数的实现就是调用这组函数。
接下来,看看enqueueMessage函数,Handler函数就是调用这个函数把message放到MessageQueue中:
[java]
view plain
copy
print?
final boolean enqueueMessage(Message msg, long when) {
if (msg.isInUse()) {//检查msg是否在使用中,一会我们可以看到MessageQueue.next()在返回前通过Message.makeInUse函数设置msg为使用状态,而我们之前看到过Looper.loop中通过调用调用Message.recycle(),把Message重置为未使用的状态。
throw new AndroidRuntimeException(msg + " This message is already in use.");
}
if (msg.target == null) {//msg必须知道由那个Handler负责处理它
throw new AndroidRuntimeException("Message must have a target.");
}
boolean needWake;
synchronized (this) {
if (mQuiting) {//如果已经调用MessageQueue.quit,那么不再接收新的Message
RuntimeException e = new RuntimeException(
msg.target + " sending message to a Handler on a dead thread");
Log.w("MessageQueue", e.getMessage(), e);
return false;
}
msg.when = when;
Message p = mMessages;
if (p == null || when == 0 || when < p.when) {//插到列表头
// New head, wake up the event queue if blocked.
msg.next = p;
mMessages = msg;
needWake = mBlocked;//当前MessageQueue处于block状态,所以需要唤醒
} else {
// Inserted within the middle of the queue. Usually we don't have to wake
// up the event queue unless there is a barrier at the head of the queue
// and the message is the earliest asynchronous message in the queue.
needWake = mBlocked && p.target == null && msg.isAsynchronous();//当且仅当MessageQueue因为Sync Barrier而block,并且msg为异步消息时,唤醒。 关于msg.isAsyncChronous(),请回去看看Handler.enqueueMessage函数和构造函数
Message prev;
for (;;) {<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">// 根据when的大小顺序,插入到合适的位置</SPAN>
prev = p;
p = p.next;
if (p == null || when < p.when) {
break;
}
if (needWake && p.isAsynchronous()) {//如果在插入位置以前,发现异步消息,则不需要唤醒
needWake = false;
}
}
msg.next = p; // invariant: p == prev.next
prev.next = msg;
}
}
if (needWake) {
nativeWake(mPtr);//唤醒nativeMessageQueue
}
return true;
}
final void quit() {
if (!mQuitAllowed) {//UI线程的Looper消息队列不可退出
throw new RuntimeException("Main thread not allowed to quit.");
}
synchronized (this) {
if (mQuiting) {
return;
}
mQuiting = true;
}
nativeWake(mPtr);//唤醒nativeMessageQueue
}
[java]
view plain
copy
print?
final int enqueueSyncBarrier(long when) {
// Enqueue a new sync barrier token.
// We don't need to wake the queue because the purpose of a barrier is to stall it.
synchronized (this) {
final int token = mNextBarrierToken++;
final Message msg = Message.obtain();
msg.arg1 = token;
Message prev = null;
Message p = mMessages;
if (when != 0) {
while (p != null && p.when <= when) {
prev = p;
p = p.next;
}
}
if (prev != null) { // invariant: p == prev.next
msg.next = p;
prev.next = msg;
} else {
msg.next = p;
mMessages = msg;
}
return token;
}
}
final void removeSyncBarrier(int token) {
// Remove a sync barrier token from the queue.
// If the queue is no longer stalled by a barrier then wake it.
final boolean needWake;
synchronized (this) {
Message prev = null;
Message p = mMessages;
while (p != null && (p.target != null || p.arg1 != token)) {
prev = p;
p = p.next;
}
if (p == null) {
throw new IllegalStateException("The specified message queue synchronization "
+ " barrier token has not been posted or has already been removed.");
}
if (prev != null) {
prev.next = p.next;
needWake = false;
} else {
mMessages = p.next;
needWake = mMessages == null || mMessages.target != null;//其实我觉得这边应该是needWake = mMessages != null && mMessages.target != null
}
p.recycle();
}
if (needWake) {
nativeWake(mPtr);//有需要的话,唤醒nativeMessageQueue
}
}
重头戏又来了:
[java]
view plain
copy
print?
final Message next() {
int pendingIdleHandlerCount = -1; // -1 only during first iteration
int nextPollTimeoutMillis = 0;
for (;;) {
if (nextPollTimeoutMillis != 0) {
Binder.flushPendingCommands();//不太理解,选择性无视
}
nativePollOnce(mPtr, nextPollTimeoutMillis);//等待nativeMessageQueue返回,最多等待nextPollTimeoutMillis毫秒
synchronized (this) {
if (mQuiting) {//如果要退出,则返回null
return null;
}
// Try to retrieve the next message. Return if found.
final long now = SystemClock.uptimeMillis();
Message prevMsg = null;
Message msg = mMessages;
if (msg != null && msg.target == null) {//下一个消息为sync barrier
// Stalled by a barrier. Find the next asynchronous message in the queue.
do {
prevMsg = msg;
msg = msg.next;
} while (msg != null && !msg.isAsynchronous());//因为存在sync barrier,仅有异步消息可以执行,所以寻在最近的异步消息
}
if (msg != null) {
if (now < msg.when) {
// Next message is not ready. Set a timeout to wake up when it is ready.
nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE);//消息还没到执行的时间,所以我们继续等待msg.when - now毫秒
} else {
// Got a message.
mBlocked = false;//开始处理消息了,所以不再是blocked状态
if (prevMsg != null) {
prevMsg.next = msg.next;//从链表中间移除message
} else {
mMessages = msg.next;//从链表头移除message
}
msg.next = null;
if (false) Log.v("MessageQueue", "Returning message: " + msg);
msg.markInUse();//标记msg正在使用
return msg;//返回到Looper.loop函数
}
} else {
// No more messages.
nextPollTimeoutMillis = -1;//没有消息可以处理,所以无限制的等待
}
// If first time idle, then get the number of idlers to run.
// Idle handles only run if the queue is empty or if the first message
// in the queue (possibly a barrier) is due to be handled in the future.
if (pendingIdleHandlerCount < 0
&& (mMessages == null || now < mMessages.when)) {<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">// 目前无消息可以处理,可以执行IdleHandler</SPAN>
pendingIdleHandlerCount = mIdleHandlers.size();
}
if (pendingIdleHandlerCount <= 0) {
// No idle handlers to run. Loop and wait some more.
mBlocked = true;
continue;
}
if (mPendingIdleHandlers == null) {
mPendingIdleHandlers = new IdleHandler[Math.max(pendingIdleHandlerCount, 4)];
}
mPendingIdleHandlers = mIdleHandlers.toArray(mPendingIdleHandlers);
}
// Run the idle handlers.
// We only ever reach this code block during the first iteration.
for (int i = 0; i < pendingIdleHandlerCount; i++) {
final IdleHandler idler = mPendingIdleHandlers[i];
mPendingIdleHandlers[i] = null; // release the reference to the handler
boolean keep = false;
try {
keep = idler.queueIdle();
} catch (Throwable t) {
Log.wtf("MessageQueue", "IdleHandler threw exception", t);
}
if (!keep) {
synchronized (this) {
mIdleHandlers.remove(idler);
}
}
}
// Reset the idle handler count to 0 so we do not run them again.
pendingIdleHandlerCount = 0;//Looper.looper调用一次MessageQueue.next(),只允许调用一轮IdleHandler
// While calling an idle handler, a new message could have been delivered
// so go back and look again for a pending message without waiting.
nextPollTimeoutMillis = 0;//因为执行IdleHandler的过程中,可能有新的消息到来,所以把等待时间设置为0
}
}
nativePollOnce(mPtr, nextPollTimeoutMillis);暂时无视mPtr参数,阻塞等待nextPollTimeoutMillis毫秒的时间返回,与Object.wait(long timeout)相似
nativeWake(mPtr);暂时无视mPtr参数,唤醒等待的nativePollOnce函数返回的线程,从这个角度解释nativePollOnce函数应该是最多等待nextPollTimeoutMillis毫秒
小结:
MessageQueue作为一个容器,保存了所有待执行的消息。
MessageQueue中的Message包含三种类型:普通的同步消息,Sync barrier(target = null),异步消息(isAsynchronous() = true)。
MessageQueue的核心函数为enqueueMessage和next,前者用于向容器内添加Message,而Looper通过后者从MessageQueue中获取消息,并实现无消息情况下的等待。
MessageQueue把Android消息机制的Java实现和C++实现联系起来。
本来我是想一口气把java实现和C++实现都写完的,但是,无奈最近工作和个人事务都比较多,稍后为大家奉上C++实现的解析。
Handler和Message是Android开发者常用的两个API,我一直对于它的内部实现比较好奇,所以用空闲的时间,阅读了一下他们的源码。
相关的Java Class:
android.os.Message
android.os.MessageQueue
android.os.Looper
android.os.Handler
相关的C++ Class:
android.NativeMessageQueue
android.Looper
android.LooperCallback
android.SimpleLooperCallback
android.Message
android.MessageHandler
首先,来看看这些类之间的关系:
首先,让我们从相对简单的java实现开始看起:
Message
Message类可以说是最简单的,主要提供了一些成员,用以保存消息数据。[java]
view plain
copy
print?
public int what;//用以表示消息类别
public int arg1;//消息数据
public int arg2;//消息数据
public Object obj;//消息数据
public int what;//用以表示消息类别 public int arg1;//消息数据 public int arg2;//消息数据 public Object obj;//消息数据
[java]
view plain
copy
print?
/*package*/ long when;//消息应该被处理的时间
/*package*/ Bundle data;//消息数据
/*package*/ Handler target;//处理这个消息的handler
/*package*/ Runnable callback;//回调函数
// sometimes we store linked lists of these things
/*package*/ Message next;//形成链表,保存Message实例
/*package*/ long when;//消息应该被处理的时间 /*package*/ Bundle data;//消息数据 /*package*/ Handler target;//处理这个消息的handler /*package*/ Runnable callback;//回调函数 // sometimes we store linked lists of these things /*package*/ Message next;//形成链表,保存Message实例
值得一提的是,Android提供了一个简单,但是有用的消息池,对于Message这种使用频繁的类型,可以有效的减少内存申请和释放的次数,提高性能。
[java]
view plain
copy
print?
private static final Object sPoolSync = new Object();
private static Message sPool;
private static int sPoolSize = 0;
private static final int MAX_POOL_SIZE = 50;
private static final Object sPoolSync = new Object(); private static Message sPool; private static int sPoolSize = 0; private static final int MAX_POOL_SIZE = 50;
[java]
view plain
copy
print?
/**
* Return a new Message instance from the global pool. Allows us to
* avoid allocating new objects in many cases.
*/
public static Message obtain() {
synchronized (sPoolSync) {
if (sPool != null) {//消息池不为空,则从消息池中获取实例
Message m = sPool;
sPool = m.next;
m.next = null;
sPoolSize--;
return m;
}
}
return new Message();
}
/**
* Return a Message instance to the global pool. You MUST NOT touch
* the Message after calling this function -- it has effectively been
* freed.
*/
public void recycle() {
clearForRecycle();
synchronized (sPoolSync) {
if (sPoolSize < MAX_POOL_SIZE) {//消息池大小未满,则放入消息池
next = sPool;
sPool = this;
sPoolSize++;
}
}
}
/*package*/ void clearForRecycle() {
flags = 0;
what = 0;
arg1 = 0;
arg2 = 0;
obj = null;
replyTo = null;
when = 0;
target = null;
callback = null;
data = null;
}
/** * Return a new Message instance from the global pool. Allows us to * avoid allocating new objects in many cases. */ public static Message obtain() { synchronized (sPoolSync) { if (sPool != null) {//消息池不为空,则从消息池中获取实例 Message m = sPool; sPool = m.next; m.next = null; sPoolSize--; return m; } } return new Message(); } /** * Return a Message instance to the global pool. You MUST NOT touch * the Message after calling this function -- it has effectively been * freed. */ public void recycle() { clearForRecycle(); synchronized (sPoolSync) { if (sPoolSize < MAX_POOL_SIZE) {//消息池大小未满,则放入消息池 next = sPool; sPool = this; sPoolSize++; } } } /*package*/ void clearForRecycle() { flags = 0; what = 0; arg1 = 0; arg2 = 0; obj = null; replyTo = null; when = 0; target = null; callback = null; data = null; }
小结:
Message的核心在于它的数据域,Handler根据这些内容来识别和处理消息
应该使用Message.obtain(或者Handler.obtainMessage)函数获取message实例
Handler
首先看看构造函数:[java]
view plain
copy
print?
public interface Callback {
public boolean handleMessage(Message msg);
}
public interface Callback { public boolean handleMessage(Message msg); }
[java]
view plain
copy
print?
public Handler() {
this(null, false);
}
public Handler() { this(null, false); }
[java]
view plain
copy
print?
public Handler(Callback callback, boolean async) {
if (FIND_POTENTIAL_LEAKS) {
final Class<? extends Handler> klass = getClass();
if ((klass.isAnonymousClass() || klass.isMemberClass() || klass.isLocalClass()) &&
(klass.getModifiers() & Modifier.STATIC) == 0) {
Log.w(TAG, "The following Handler class should be static or leaks might occur: " +
klass.getCanonicalName());
}
}
mLooper = Looper.myLooper();
if (mLooper == null) {
throw new RuntimeException(
"Can't create handler inside thread that has not called Looper.prepare()");
}
mQueue = mLooper.mQueue;
mCallback = callback; //使用Callback可以拦截Handler处理消息,之后会在dispatchMessage函数中,大展身手
mAsynchronous = async;//设置handler的消息为异步消息,暂时先无视这个变量
}
public Handler(Callback callback, boolean async) { if (FIND_POTENTIAL_LEAKS) { final Class<? extends Handler> klass = getClass(); if ((klass.isAnonymousClass() || klass.isMemberClass() || klass.isLocalClass()) && (klass.getModifiers() & Modifier.STATIC) == 0) { Log.w(TAG, "The following Handler class should be static or leaks might occur: " + klass.getCanonicalName()); } } mLooper = Looper.myLooper(); if (mLooper == null) { throw new RuntimeException( "Can't create handler inside thread that has not called Looper.prepare()"); } mQueue = mLooper.mQueue; mCallback = callback; //使用Callback可以拦截Handler处理消息,之后会在dispatchMessage函数中,大展身手 mAsynchronous = async;//设置handler的消息为异步消息,暂时先无视这个变量 }
Handler的构造函数最主要的就是初始化成员变量:mLooper和mQueue。 这边需要注意的一个问题是:Looper.myLooper()不能返回null,否则抛出RuntimeExeception。稍后详解Looper.myLooper();函数在何种情况下会抛出异常。
Handler.obtainMessage系列的函数都会调用Message类中对应的静态方法,从消息池中获取一个可用的消息实例。典型实现如下:
[java]
view plain
copy
print?
public final Message obtainMessage()
{
return Message.obtain(this);
}
public final Message obtainMessage() { return Message.obtain(this); }
Handler.post系列和send系列函数最终都会调用enqueueMessage函数,把message入列,不同之处在于post系列函数会以Runable参数构建一个Message实例。
[java]
view plain
copy
print?
private static Message getPostMessage(Runnable r) {
Message m = Message.obtain();
m.callback = r;//一会我们会看到callback非空的message和callback为空的mesage在处理时的差异
return m;
}
public final boolean post(Runnable r)
{
return sendMessageDelayed(getPostMessage(r), 0);
}
public final boolean sendMessage(Message msg)
{
return sendMessageDelayed(msg, 0);
}
public final boolean sendMessageDelayed(Message msg, long delayMillis)
{
if (delayMillis < 0) {
delayMillis = 0;
}
return sendMessageAtTime(msg, SystemClock.uptimeMillis() + delayMillis);
}
public boolean sendMessageAtTime(Message msg, long uptimeMillis) {
MessageQueue queue = mQueue;
if (queue == null) {
RuntimeException e = new RuntimeException(
this + " sendMessageAtTime() called with no mQueue");
Log.w("Looper", e.getMessage(), e);
return false;
}
return enqueueMessage(queue, msg, uptimeMillis);
}
//最终都会调用这个函数,把message入列
private boolean enqueueMessage(MessageQueue queue, Message msg, long uptimeMillis) {
msg.target = this;
if (mAsynchronous) {
msg.setAsynchronous(true);//Handler的<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">mAsynchronous属性,决定了msg是否为asynchronous,稍后在MessageQueue.next函数中,可以看到</SPAN><SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">asynchronous对于消息处理的影响</SPAN><SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">
AN> }
return queue.enqueueMessage(msg, uptimeMillis);
}
private static Message getPostMessage(Runnable r) { Message m = Message.obtain(); m.callback = r;//一会我们会看到callback非空的message和callback为空的mesage在处理时的差异 return m; } public final boolean post(Runnable r) { return sendMessageDelayed(getPostMessage(r), 0); } public final boolean sendMessage(Message msg) { return sendMessageDelayed(msg, 0); } public final boolean sendMessageDelayed(Message msg, long delayMillis) { if (delayMillis < 0) { delayMillis = 0; } return sendMessageAtTime(msg, SystemClock.uptimeMillis() + delayMillis); } public boolean sendMessageAtTime(Message msg, long uptimeMillis) { MessageQueue queue = mQueue; if (queue == null) { RuntimeException e = new RuntimeException( this + " sendMessageAtTime() called with no mQueue"); Log.w("Looper", e.getMessage(), e); return false; } return enqueueMessage(queue, msg, uptimeMillis); } //最终都会调用这个函数,把message入列 private boolean enqueueMessage(MessageQueue queue, Message msg, long uptimeMillis) { msg.target = this; if (mAsynchronous) { msg.setAsynchronous(true);//Handler的<span style="FONT-FAMILY: Arial, Helvetica, sans-serif">mAsynchronous属性,决定了msg是否为asynchronous,稍后在MessageQueue.next函数中,可以看到</span><span style="FONT-FAMILY: Arial, Helvetica, sans-serif">asynchronous对于消息处理的影响</span><span style="FONT-FAMILY: Arial, Helvetica, sans-serif"> </span> } return queue.enqueueMessage(msg, uptimeMillis); }除了这些之外,Handler还提供了hasMessage系列和removeMessages系列函数用以管理Handler对应的MessageQueue中的消息。
接下来主角登场,Handler.dispatchMessage:
[java]
view plain
copy
print?
private static void handleCallback(Message message) {
message.callback.run();
}
/**
* Subclasses must implement this to receive messages.
*/
public void handleMessage(Message msg) {
}
/**
* Handle system messages here.
*/
public void dispatchMessage(Message msg) {
if (msg.callback != null) {//message的callback不为null,则执行
handleCallback(msg);
} else {
if (mCallback != null) {//如果Hanlder的mCallback成员不为null,则调用
if (mCallback.handleMessage(msg)) {//如果handleMessage返回值为true,则拦截消息
return;
}
}
handleMessage(msg);//处理消息
}
}
private static void handleCallback(Message message) { message.callback.run(); } /** * Subclasses must implement this to receive messages. */ public void handleMessage(Message msg) { } /** * Handle system messages here. */ public void dispatchMessage(Message msg) { if (msg.callback != null) {//message的callback不为null,则执行 handleCallback(msg); } else { if (mCallback != null) {//如果Hanlder的mCallback成员不为null,则调用 if (mCallback.handleMessage(msg)) {//如果handleMessage返回值为true,则拦截消息 return; } } handleMessage(msg);//处理消息 } }
注释应该比较清楚,不多说。 小结:
Handler类最为核心的函数是enqueueMessage和dispatcherMessage,前者把待处理的消息放入MessageQueue,而Looper调用后者来处理从MessageQueue获取的消息。
callback不为null(通过post系列函数添加到消息队列中)的message无法被拦截,而callback为null的函数可以被Handler的mCallback拦截
Looper
同样从构造函数看起:[java]
view plain
copy
print?
private Looper(boolean quitAllowed) {
mQueue = new MessageQueue(quitAllowed);//每个Looper有一个MessageQueue
mRun = true;
mThread = Thread.currentThread();
}
private Looper(boolean quitAllowed) { mQueue = new MessageQueue(quitAllowed);//每个Looper有一个MessageQueue mRun = true; mThread = Thread.currentThread(); }
[java]
view plain
copy
print?
** Initialize the current thread as a looper.
* This gives you a chance to create handlers that then reference
* this looper, before actually starting the loop. Be sure to call
* {@link #loop()} after calling this method, and end it by calling
* {@link #quit()}.
*/
public static void prepare() {
prepare(true);//后台线程的looper都允许退出
}
private static void prepare(boolean quitAllowed) {
if (sThreadLocal.get() != null) {
throw new RuntimeException("Only one Looper may be created per thread");//每个线程只能有一个Looper
}
sThreadLocal.set(new Looper(quitAllowed));//把实例保存到TLS(Thread Local Save),仅有每个线程访问自己的Looper
}
/**
* Initialize the current thread as a looper, marking it as an
* application's main looper. The main looper for your application
* is created by the Android environment, so you should never need
* to call this function yourself. See also: {@link #prepare()}
*/
public static void prepareMainLooper() {
prepare(false);//主线程的lopper不可以退出
synchronized (Looper.class) {
if (sMainLooper != null) {
throw new IllegalStateException("The main Looper has already been prepared.");
}
sMainLooper = myLooper();
}
}
** Initialize the current thread as a looper. * This gives you a chance to create handlers that then reference * this looper, before actually starting the loop. Be sure to call * {@link #loop()} after calling this method, and end it by calling * {@link #quit()}. */ public static void prepare() { prepare(true);//后台线程的looper都允许退出 } private static void prepare(boolean quitAllowed) { if (sThreadLocal.get() != null) { throw new RuntimeException("Only one Looper may be created per thread");//每个线程只能有一个Looper } sThreadLocal.set(new Looper(quitAllowed));//把实例保存到TLS(Thread Local Save),仅有每个线程访问自己的Looper } /** * Initialize the current thread as a looper, marking it as an * application's main looper. The main looper for your application * is created by the Android environment, so you should never need * to call this function yourself. See also: {@link #prepare()} */ public static void prepareMainLooper() { prepare(false);//主线程的lopper不可以退出 synchronized (Looper.class) { if (sMainLooper != null) { throw new IllegalStateException("The main Looper has already been prepared."); } sMainLooper = myLooper(); } }因为是私有的构造函数,所以理论上来说只能通过prepare和prepareMainLooper两个函数来实例化Looper,但是google的注释也说的很清楚:prepareMainLooper()应该由系统调用(有兴趣的同学可以去看看AtivityThread类的main函数),所以,应用开发者可以使用的只剩下prepare函数。
好了,Looper的实例是构造出来,但是如何获取构造出来的实例呢?
[java]
view plain
copy
print?
/** Returns the application's main looper, which lives in the main thread of the application.
*/
public static Looper getMainLooper() {
synchronized (Looper.class) {
return sMainLooper;
}
}
/**
* Return the Looper object associated with the current thread. Returns
* null if the calling thread is not associated with a Looper.
*/
public static Looper myLooper() {
return sThreadLocal.get();
}
/** Returns the application's main looper, which lives in the main thread of the application. */ public static Looper getMainLooper() { synchronized (Looper.class) { return sMainLooper; } } /** * Return the Looper object associated with the current thread. Returns * null if the calling thread is not associated with a Looper. */ public static Looper myLooper() { return sThreadLocal.get(); }现在,我们应该知道如何防止Handler实例化的时候,抛出RuntimeException:在守护线程中实例化Handler之前,需要先调用Looper.perpare函数来构造Looper实例。
然后,重头戏来了:
[java]
view plain
copy
print?
/**
* Quits the looper.
*
* Causes the {@link #loop} method to terminate as soon as possible.
*/
public void quit() {
mQueue.quit();
}
/**
* Run the message queue in this thread. Be sure to call
* {@link #quit()} to end the loop.
*/
public static void loop() {
final Looper me = myLooper();
if (me == null) {//调用looper之前,需要先调用perpare,否则您懂的...
throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread.");
}
final MessageQueue queue = me.mQueue;
// Make sure the identity of this thread is that of the local process,
// and keep track of what that identity token actually is.
Binder.clearCallingIdentity();//不太明白这个函数,但不是重点可以无视
final long ident = Binder.clearCallingIdentity();
for (;;) {
Message msg = queue.next(); // might block 获取一个下一个消息,如果当前没有要处理的消息,则block,之后我们会看到这个API的实现
if (msg == null) {//调用了MessgeQueu的quit函数后,MessageQueue.next会返回null
// No message indicates that the message queue is quitting.
return;
}
// This must be in a local variable, in case a UI event sets the logger
Printer logging = me.mLogging;
if (logging != null) {//借助logging我们可以打印Looper中处理的消息
logging.println(">>>>> Dispatching to " + msg.target + " " +
msg.callback + ": " + msg.what);
}
msg.target.dispatchMessage(msg);//调用handler处理消息
if (logging != null) {
logging.println("<<<<< Finished to " + msg.target + " " + msg.callback);
}
// Make sure that during the course of dispatching the
// identity of the thread wasn't corrupted.
final long newIdent = Binder.clearCallingIdentity();//选择性无视
if (ident != newIdent) {
Log.wtf(TAG, "Thread identity changed from 0x"
+ Long.toHexString(ident) + " to 0x"
+ Long.toHexString(newIdent) + " while dispatching to "
+ msg.target.getClass().getName() + " "
+ msg.callback + " what=" + msg.what);
}
msg.recycle();//回收消息到消息池
}
}
/** * Quits the looper. * * Causes the {@link #loop} method to terminate as soon as possible. */ public void quit() { mQueue.quit(); } /** * Run the message queue in this thread. Be sure to call * {@link #quit()} to end the loop. */ public static void loop() { final Looper me = myLooper(); if (me == null) {//调用looper之前,需要先调用perpare,否则您懂的... throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread."); } final MessageQueue queue = me.mQueue; // Make sure the identity of this thread is that of the local process, // and keep track of what that identity token actually is. Binder.clearCallingIdentity();//不太明白这个函数,但不是重点可以无视 final long ident = Binder.clearCallingIdentity(); for (;;) { Message msg = queue.next(); // might block 获取一个下一个消息,如果当前没有要处理的消息,则block,之后我们会看到这个API的实现 if (msg == null) {//调用了MessgeQueu的quit函数后,MessageQueue.next会返回null // No message indicates that the message queue is quitting. return; } // This must be in a local variable, in case a UI event sets the logger Printer logging = me.mLogging; if (logging != null) {//借助logging我们可以打印Looper中处理的消息 logging.println(">>>>> Dispatching to " + msg.target + " " + msg.callback + ": " + msg.what); } msg.target.dispatchMessage(msg);//调用handler处理消息 if (logging != null) { logging.println("<<<<< Finished to " + msg.target + " " + msg.callback); } // Make sure that during the course of dispatching the // identity of the thread wasn't corrupted. final long newIdent = Binder.clearCallingIdentity();//选择性无视 if (ident != newIdent) { Log.wtf(TAG, "Thread identity changed from 0x" + Long.toHexString(ident) + " to 0x" + Long.toHexString(newIdent) + " while dispatching to " + msg.target.getClass().getName() + " " + msg.callback + " what=" + msg.what); } msg.recycle();//回收消息到消息池 } }Looper.loop()函数是Looper类的核心函数,主要循环进行两个操作:
从MessageQueue中获取一个消息,当前没有消息需要处理时,则block
调用message的Handler(target)处理消息
基本上,我们可以把Looper理解为一个死循环,Looper开始work以后,线程就进入了以消息为驱动的工作模型。
小结:
每个线程最多可以有一个Looper。
每个Looper有且仅有一个MessageQueue
每个Handler关联一个MessageQueue,由该MessageQueue关联的Looper执行(调用Hanlder.dispatchMessage)
每个MessageQueue可以关联任意多个Handler
Looper API的调用顺序:Looper.prepare >> Looper.loop >> Looper.quit
Looper的核心函数是Looper.loop,一般loop不会返回,直到线程退出,所以需要线程完成某个work时,请发送消息给Message(或者说Handler)
MessageQueue
MessageQueue类是唯一包含native函数的类,我们先大致看一下,稍后C++的部分在详细解释:
[java]
view plain
copy
print?
private native void nativeInit(); //初始化
private native void nativeDestroy(); //销毁
private native void nativePollOnce(int ptr, int timeoutMillis); //等待<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">timeoutMillis指定的时间</SPAN>
private native void nativeWake(int ptr);//唤醒<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">nativePollOnce的等待</SPAN>
private native void nativeInit(); //初始化 private native void nativeDestroy(); //销毁 private native void nativePollOnce(int ptr, int timeoutMillis); //等待<span style="FONT-FAMILY: Arial, Helvetica, sans-serif">timeoutMillis指定的时间</span> private native void nativeWake(int ptr);//唤醒<span style="FONT-FAMILY: Arial, Helvetica, sans-serif">nativePollOnce的等待</span>
然后,我们再从构造函数看起:
[java]
view plain
copy
print?
Message mMessages;//数据域mMessages的类型虽然是Message,但是因为Message.next数据域的原因,其实mMessage是链表的第一个元素
MessageQueue(boolean quitAllowed) {
mQuitAllowed = quitAllowed;
nativeInit();//初始化nativeMessageQueue
}
Message mMessages;//数据域mMessages的类型虽然是Message,但是因为Message.next数据域的原因,其实mMessage是链表的第一个元素 MessageQueue(boolean quitAllowed) { mQuitAllowed = quitAllowed; nativeInit();//初始化nativeMessageQueue }对应的,在销毁的时候:
[java]
view plain
copy
print?
@Override
protected void finalize() throws Throwable {
try {
nativeDestroy();//销毁nativeMessageQueue
} finally {
super.finalize();
}
}
@Override protected void finalize() throws Throwable { try { nativeDestroy();//销毁nativeMessageQueue } finally { super.finalize(); } }
此外,MessageQueue提供了一组函数(e.g. hasMessage, removeMessage)来查询和移除待处理的消息,我们在前面的Handler类上看到的对应函数的实现就是调用这组函数。
接下来,看看enqueueMessage函数,Handler函数就是调用这个函数把message放到MessageQueue中:
[java]
view plain
copy
print?
final boolean enqueueMessage(Message msg, long when) {
if (msg.isInUse()) {//检查msg是否在使用中,一会我们可以看到MessageQueue.next()在返回前通过Message.makeInUse函数设置msg为使用状态,而我们之前看到过Looper.loop中通过调用调用Message.recycle(),把Message重置为未使用的状态。
throw new AndroidRuntimeException(msg + " This message is already in use.");
}
if (msg.target == null) {//msg必须知道由那个Handler负责处理它
throw new AndroidRuntimeException("Message must have a target.");
}
boolean needWake;
synchronized (this) {
if (mQuiting) {//如果已经调用MessageQueue.quit,那么不再接收新的Message
RuntimeException e = new RuntimeException(
msg.target + " sending message to a Handler on a dead thread");
Log.w("MessageQueue", e.getMessage(), e);
return false;
}
msg.when = when;
Message p = mMessages;
if (p == null || when == 0 || when < p.when) {//插到列表头
// New head, wake up the event queue if blocked.
msg.next = p;
mMessages = msg;
needWake = mBlocked;//当前MessageQueue处于block状态,所以需要唤醒
} else {
// Inserted within the middle of the queue. Usually we don't have to wake
// up the event queue unless there is a barrier at the head of the queue
// and the message is the earliest asynchronous message in the queue.
needWake = mBlocked && p.target == null && msg.isAsynchronous();//当且仅当MessageQueue因为Sync Barrier而block,并且msg为异步消息时,唤醒。 关于msg.isAsyncChronous(),请回去看看Handler.enqueueMessage函数和构造函数
Message prev;
for (;;) {<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">// 根据when的大小顺序,插入到合适的位置</SPAN>
prev = p;
p = p.next;
if (p == null || when < p.when) {
break;
}
if (needWake && p.isAsynchronous()) {//如果在插入位置以前,发现异步消息,则不需要唤醒
needWake = false;
}
}
msg.next = p; // invariant: p == prev.next
prev.next = msg;
}
}
if (needWake) {
nativeWake(mPtr);//唤醒nativeMessageQueue
}
return true;
}
final void quit() {
if (!mQuitAllowed) {//UI线程的Looper消息队列不可退出
throw new RuntimeException("Main thread not allowed to quit.");
}
synchronized (this) {
if (mQuiting) {
return;
}
mQuiting = true;
}
nativeWake(mPtr);//唤醒nativeMessageQueue
}
final boolean enqueueMessage(Message msg, long when) { if (msg.isInUse()) {//检查msg是否在使用中,一会我们可以看到MessageQueue.next()在返回前通过Message.makeInUse函数设置msg为使用状态,而我们之前看到过Looper.loop中通过调用调用Message.recycle(),把Message重置为未使用的状态。 throw new AndroidRuntimeException(msg + " This message is already in use."); } if (msg.target == null) {//msg必须知道由那个Handler负责处理它 throw new AndroidRuntimeException("Message must have a target."); } boolean needWake; synchronized (this) { if (mQuiting) {//如果已经调用MessageQueue.quit,那么不再接收新的Message RuntimeException e = new RuntimeException( msg.target + " sending message to a Handler on a dead thread"); Log.w("MessageQueue", e.getMessage(), e); return false; } msg.when = when; Message p = mMessages; if (p == null || when == 0 || when < p.when) {//插到列表头 // New head, wake up the event queue if blocked. msg.next = p; mMessages = msg; needWake = mBlocked;//当前MessageQueue处于block状态,所以需要唤醒 } else { // Inserted within the middle of the queue. Usually we don't have to wake // up the event queue unless there is a barrier at the head of the queue // and the message is the earliest asynchronous message in the queue. needWake = mBlocked && p.target == null && msg.isAsynchronous();//当且仅当MessageQueue因为Sync Barrier而block,并且msg为异步消息时,唤醒。 关于msg.isAsyncChronous(),请回去看看Handler.enqueueMessage函数和构造函数 Message prev; for (;;) {<span style="FONT-FAMILY: Arial, Helvetica, sans-serif">// 根据when的大小顺序,插入到合适的位置</span> prev = p; p = p.next; if (p == null || when < p.when) { break; } if (needWake && p.isAsynchronous()) {//如果在插入位置以前,发现异步消息,则不需要唤醒 needWake = false; } } msg.next = p; // invariant: p == prev.next prev.next = msg; } } if (needWake) { nativeWake(mPtr);//唤醒nativeMessageQueue } return true; } final void quit() { if (!mQuitAllowed) {//UI线程的Looper消息队列不可退出 throw new RuntimeException("Main thread not allowed to quit."); } synchronized (this) { if (mQuiting) { return; } mQuiting = true; } nativeWake(mPtr);//唤醒nativeMessageQueue }关于sync barrier,再补充点解释: sync barrier是起到了一个阻塞器的作用,它可以阻塞when>它(即执行时间比它晚)的同步消息的执行,但不影响异步消息。sync barrier的特征是targe为null,所以它只能被remove,无法被执行。MessageQueue提供了下面两个函数来控制MessageQueue中的sync barrier(如何觉得sync barrier和异步消息难以理解的话,选择性无视就好,因为它们不妨碍我们理解Android消息机制的原理):
[java]
view plain
copy
print?
final int enqueueSyncBarrier(long when) {
// Enqueue a new sync barrier token.
// We don't need to wake the queue because the purpose of a barrier is to stall it.
synchronized (this) {
final int token = mNextBarrierToken++;
final Message msg = Message.obtain();
msg.arg1 = token;
Message prev = null;
Message p = mMessages;
if (when != 0) {
while (p != null && p.when <= when) {
prev = p;
p = p.next;
}
}
if (prev != null) { // invariant: p == prev.next
msg.next = p;
prev.next = msg;
} else {
msg.next = p;
mMessages = msg;
}
return token;
}
}
final void removeSyncBarrier(int token) {
// Remove a sync barrier token from the queue.
// If the queue is no longer stalled by a barrier then wake it.
final boolean needWake;
synchronized (this) {
Message prev = null;
Message p = mMessages;
while (p != null && (p.target != null || p.arg1 != token)) {
prev = p;
p = p.next;
}
if (p == null) {
throw new IllegalStateException("The specified message queue synchronization "
+ " barrier token has not been posted or has already been removed.");
}
if (prev != null) {
prev.next = p.next;
needWake = false;
} else {
mMessages = p.next;
needWake = mMessages == null || mMessages.target != null;//其实我觉得这边应该是needWake = mMessages != null && mMessages.target != null
}
p.recycle();
}
if (needWake) {
nativeWake(mPtr);//有需要的话,唤醒nativeMessageQueue
}
}
final int enqueueSyncBarrier(long when) { // Enqueue a new sync barrier token. // We don't need to wake the queue because the purpose of a barrier is to stall it. synchronized (this) { final int token = mNextBarrierToken++; final Message msg = Message.obtain(); msg.arg1 = token; Message prev = null; Message p = mMessages; if (when != 0) { while (p != null && p.when <= when) { prev = p; p = p.next; } } if (prev != null) { // invariant: p == prev.next msg.next = p; prev.next = msg; } else { msg.next = p; mMessages = msg; } return token; } } final void removeSyncBarrier(int token) { // Remove a sync barrier token from the queue. // If the queue is no longer stalled by a barrier then wake it. final boolean needWake; synchronized (this) { Message prev = null; Message p = mMessages; while (p != null && (p.target != null || p.arg1 != token)) { prev = p; p = p.next; } if (p == null) { throw new IllegalStateException("The specified message queue synchronization " + " barrier token has not been posted or has already been removed."); } if (prev != null) { prev.next = p.next; needWake = false; } else { mMessages = p.next; needWake = mMessages == null || mMessages.target != null;//其实我觉得这边应该是needWake = mMessages != null && mMessages.target != null } p.recycle(); } if (needWake) { nativeWake(mPtr);//有需要的话,唤醒nativeMessageQueue } }
重头戏又来了:
[java]
view plain
copy
print?
final Message next() {
int pendingIdleHandlerCount = -1; // -1 only during first iteration
int nextPollTimeoutMillis = 0;
for (;;) {
if (nextPollTimeoutMillis != 0) {
Binder.flushPendingCommands();//不太理解,选择性无视
}
nativePollOnce(mPtr, nextPollTimeoutMillis);//等待nativeMessageQueue返回,最多等待nextPollTimeoutMillis毫秒
synchronized (this) {
if (mQuiting) {//如果要退出,则返回null
return null;
}
// Try to retrieve the next message. Return if found.
final long now = SystemClock.uptimeMillis();
Message prevMsg = null;
Message msg = mMessages;
if (msg != null && msg.target == null) {//下一个消息为sync barrier
// Stalled by a barrier. Find the next asynchronous message in the queue.
do {
prevMsg = msg;
msg = msg.next;
} while (msg != null && !msg.isAsynchronous());//因为存在sync barrier,仅有异步消息可以执行,所以寻在最近的异步消息
}
if (msg != null) {
if (now < msg.when) {
// Next message is not ready. Set a timeout to wake up when it is ready.
nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE);//消息还没到执行的时间,所以我们继续等待msg.when - now毫秒
} else {
// Got a message.
mBlocked = false;//开始处理消息了,所以不再是blocked状态
if (prevMsg != null) {
prevMsg.next = msg.next;//从链表中间移除message
} else {
mMessages = msg.next;//从链表头移除message
}
msg.next = null;
if (false) Log.v("MessageQueue", "Returning message: " + msg);
msg.markInUse();//标记msg正在使用
return msg;//返回到Looper.loop函数
}
} else {
// No more messages.
nextPollTimeoutMillis = -1;//没有消息可以处理,所以无限制的等待
}
// If first time idle, then get the number of idlers to run.
// Idle handles only run if the queue is empty or if the first message
// in the queue (possibly a barrier) is due to be handled in the future.
if (pendingIdleHandlerCount < 0
&& (mMessages == null || now < mMessages.when)) {<SPAN style="FONT-FAMILY: Arial, Helvetica, sans-serif">// 目前无消息可以处理,可以执行IdleHandler</SPAN>
pendingIdleHandlerCount = mIdleHandlers.size();
}
if (pendingIdleHandlerCount <= 0) {
// No idle handlers to run. Loop and wait some more.
mBlocked = true;
continue;
}
if (mPendingIdleHandlers == null) {
mPendingIdleHandlers = new IdleHandler[Math.max(pendingIdleHandlerCount, 4)];
}
mPendingIdleHandlers = mIdleHandlers.toArray(mPendingIdleHandlers);
}
// Run the idle handlers.
// We only ever reach this code block during the first iteration.
for (int i = 0; i < pendingIdleHandlerCount; i++) {
final IdleHandler idler = mPendingIdleHandlers[i];
mPendingIdleHandlers[i] = null; // release the reference to the handler
boolean keep = false;
try {
keep = idler.queueIdle();
} catch (Throwable t) {
Log.wtf("MessageQueue", "IdleHandler threw exception", t);
}
if (!keep) {
synchronized (this) {
mIdleHandlers.remove(idler);
}
}
}
// Reset the idle handler count to 0 so we do not run them again.
pendingIdleHandlerCount = 0;//Looper.looper调用一次MessageQueue.next(),只允许调用一轮IdleHandler
// While calling an idle handler, a new message could have been delivered
// so go back and look again for a pending message without waiting.
nextPollTimeoutMillis = 0;//因为执行IdleHandler的过程中,可能有新的消息到来,所以把等待时间设置为0
}
}
final Message next() { int pendingIdleHandlerCount = -1; // -1 only during first iteration int nextPollTimeoutMillis = 0; for (;;) { if (nextPollTimeoutMillis != 0) { Binder.flushPendingCommands();//不太理解,选择性无视 } nativePollOnce(mPtr, nextPollTimeoutMillis);//等待nativeMessageQueue返回,最多等待nextPollTimeoutMillis毫秒 synchronized (this) { if (mQuiting) {//如果要退出,则返回null return null; } // Try to retrieve the next message. Return if found. final long now = SystemClock.uptimeMillis(); Message prevMsg = null; Message msg = mMessages; if (msg != null && msg.target == null) {//下一个消息为sync barrier // Stalled by a barrier. Find the next asynchronous message in the queue. do { prevMsg = msg; msg = msg.next; } while (msg != null && !msg.isAsynchronous());//因为存在sync barrier,仅有异步消息可以执行,所以寻在最近的异步消息 } if (msg != null) { if (now < msg.when) { // Next message is not ready. Set a timeout to wake up when it is ready. nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE);//消息还没到执行的时间,所以我们继续等待msg.when - now毫秒 } else { // Got a message. mBlocked = false;//开始处理消息了,所以不再是blocked状态 if (prevMsg != null) { prevMsg.next = msg.next;//从链表中间移除message } else { mMessages = msg.next;//从链表头移除message } msg.next = null; if (false) Log.v("MessageQueue", "Returning message: " + msg); msg.markInUse();//标记msg正在使用 return msg;//返回到Looper.loop函数 } } else { // No more messages. nextPollTimeoutMillis = -1;//没有消息可以处理,所以无限制的等待 } // If first time idle, then get the number of idlers to run. // Idle handles only run if the queue is empty or if the first message // in the queue (possibly a barrier) is due to be handled in the future. if (pendingIdleHandlerCount < 0 && (mMessages == null || now < mMessages.when)) {<span style="FONT-FAMILY: Arial, Helvetica, sans-serif">// 目前无消息可以处理,可以执行IdleHandler</span> pendingIdleHandlerCount = mIdleHandlers.size(); } if (pendingIdleHandlerCount <= 0) { // No idle handlers to run. Loop and wait some more. mBlocked = true; continue; } if (mPendingIdleHandlers == null) { mPendingIdleHandlers = new IdleHandler[Math.max(pendingIdleHandlerCount, 4)]; } mPendingIdleHandlers = mIdleHandlers.toArray(mPendingIdleHandlers); } // Run the idle handlers. // We only ever reach this code block during the first iteration. for (int i = 0; i < pendingIdleHandlerCount; i++) { final IdleHandler idler = mPendingIdleHandlers[i]; mPendingIdleHandlers[i] = null; // release the reference to the handler boolean keep = false; try { keep = idler.queueIdle(); } catch (Throwable t) { Log.wtf("MessageQueue", "IdleHandler threw exception", t); } if (!keep) { synchronized (this) { mIdleHandlers.remove(idler); } } } // Reset the idle handler count to 0 so we do not run them again. pendingIdleHandlerCount = 0;//Looper.looper调用一次MessageQueue.next(),只允许调用一轮IdleHandler // While calling an idle handler, a new message could have been delivered // so go back and look again for a pending message without waiting. nextPollTimeoutMillis = 0;//因为执行IdleHandler的过程中,可能有新的消息到来,所以把等待时间设置为0 } }为了方便大家理解Message的工作原理,先简单描述nativeWake,和natePollonce的作用:
nativePollOnce(mPtr, nextPollTimeoutMillis);暂时无视mPtr参数,阻塞等待nextPollTimeoutMillis毫秒的时间返回,与Object.wait(long timeout)相似
nativeWake(mPtr);暂时无视mPtr参数,唤醒等待的nativePollOnce函数返回的线程,从这个角度解释nativePollOnce函数应该是最多等待nextPollTimeoutMillis毫秒
小结:
MessageQueue作为一个容器,保存了所有待执行的消息。
MessageQueue中的Message包含三种类型:普通的同步消息,Sync barrier(target = null),异步消息(isAsynchronous() = true)。
MessageQueue的核心函数为enqueueMessage和next,前者用于向容器内添加Message,而Looper通过后者从MessageQueue中获取消息,并实现无消息情况下的等待。
MessageQueue把Android消息机制的Java实现和C++实现联系起来。
本来我是想一口气把java实现和C++实现都写完的,但是,无奈最近工作和个人事务都比较多,稍后为大家奉上C++实现的解析。
相关文章推荐
- Gprinter Android SDK V2.1.4 使用说明
- Android Studio -修改LogCat的颜色
- Android开发之基本控件
- Android之ListView的高级封装!
- 从setContentView方法分析Android加载布局流程
- Android:Animation专题:1.alpha、scale、translate、rotate、set的xml属性及用法
- Android使用Messenger进行Service IPC通信分析
- Android控件ListView
- Android 播放提示音
- android 下的多线程
- 【安卓基础四】adb命令使用Heap检测和分析Android应用内存
- Android开发中的View类的视图属性focusableInTouchMode这个属性跟focusable有什么区别?
- ViewGroup的事件分发机制
- Android新手入门2016(1)--创建和运行helloworld
- Android L+ Theme 与 Toolbar
- Android Studio 上传SVN标准全过程
- Android工程的编译过程
- Android应用程序资源
- Android应用开发之使用Socket进行大文件断点上传续传
- Xp 下使用 Fiddler 抓取android手机包