面试笔试杂项积累-leetcode 271-280
2016-02-13 23:03
603 查看
273.273-Integer to English Words-Difficulty: Medium
Convert a non-negative integer to its english words representation. Given input is guaranteed to be less than 231 - 1.For example,
123 -> "One Hundred Twenty Three" 12345 -> "Twelve Thousand Three Hundred Forty Five" 1234567 -> "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven"
Hint:
Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000.
Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words.
There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out)
思路
之前做过几个类似的题,比如罗马数字什么的映射的问题
public class Solution {
private string[] belowTen = new String[] {"", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
private string[] belowTwenty = new String[] {"Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"};
private string[] belowHundred = new String[] {"", "Ten", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};
public string NumberToWords(int num) {
if (num == 0) return "Zero";
return helper(num);
}
private String helper(int num) {
string result = "";
if (num < 10) result = belowTen[num];
else if (num < 20) result = belowTwenty[num -10];
else if (num < 100) result = belowHundred[num/10] + " " + helper(num % 10);
else if (num < 1000) result = helper(num/100) + " Hundred " + helper(num % 100);
else if (num < 1000000) result = helper(num/1000) + " Thousand " + helper(num % 1000);
else if (num < 1000000000) result = helper(num/1000000) + " Million " + helper(num % 1000000);
else result = helper(num/1000000000) + " Billion " + helper(num % 1000000000);
return result.Trim(' ');
}
}
274.274-H-Index-Difficulty: Medium
Given an array of citations (each citation is a non-negative integer) of a researcher, write a function to compute the researcher's h-index.According to the
definition of h-index on Wikipedia: "A scientist has index h if
h of his/her N papers have at least h citations each, and the other
N − h papers have no more than h citations each."
For example, given
citations = [3, 0, 6, 1, 5], which means the researcher has
5papers in total and each of them had received
3, 0, 6, 1, 5citations respectively. Since the researcher has
3papers with at least
3citations each and the remaining two with
no more than
3citations each, his h-index is
3.
Note: If there are several possible values for
h, the maximum one is taken as the h-index.
Hint:
An easy approach is to sort the array first.
What are the possible values of h-index?
A faster approach is to use extra space.
思路
根据wiki的公式来做h-index (f) =
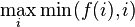
public class Solution {
public int HIndex(int[] citations) {
Array.Sort(citations);
int max = 0;
for(int i = 0;i<citations.Length;i++ )
{
max = Math.Max(Math.Min(citations.Length-i,citations[i]),max);
}
return max;
}
}
275.275-H-Index II-Difficulty: Medium
Follow up forH-Index: What if the
citationsarray is sorted in ascending order? Could you optimize your algorithm?
Hint:
Expected runtime complexity is in
O(log n) and the input is sorted.
思路
和274相同,不用排序了public class Solution {
public int HIndex(int[] citations) {
int max = 0;
for(int i = 0;i<citations.Length;i++ )
{
max = Math.Max(Math.Min(citations.Length-i,citations[i]),max);
}
return max;
}
}
278.278-First Bad Version-Difficulty: Easy
You are a product manager and currently leading a team to develop a new product. Unfortunately, the latest version of your product fails the quality check. Since each version is developed based on the previous version, all theversions after a bad version are also bad.
Suppose you have
nversions
[1, 2, ..., n]and you want to find out the first bad one, which causes all the following ones to be bad.
You are given an API
bool isBadVersion(version)which will return whether
versionis bad. Implement a function to find the first bad version. You should minimize the number of calls to the API.
思路
使用二分查找来解决/* The isBadVersion API is defined in the parent class VersionControl.
bool IsBadVersion(int version); */
public class Solution : VersionControl {
public int FirstBadVersion(int n) {
int start = 0;
int end = n;
while (start < end)
{
int mid = start + (end-start ) / 2;
if (IsBadVersion(mid))
{
end = mid;
}
else
{
start = mid + 1;
}
}
return start;
}
}
279.279-Perfect Squares-Difficulty: Medium
Given a positive integer n, find the least number of perfect square numbers (for example,1, 4, 9, 16, ...) which sum to n.
For example, given n =
12, return
3because
12 = 4 + 4 + 4; given n =
13, return
2because
13 = 4 + 9.
思路
返回一个数最少的完美平方数之和的数量动态规划
If the number is already a perfect square, then dp[number] can be 1 directly. This is just a optimization for this DP solution.
To get the value of dp
, we should choose the min
value from:
dp[n - 1] + 1,
dp[n - 4] + 1,
dp[n - 9] + 1,
dp[n - 16] + 1
and so on...
参考:
https://leetcode.com/discuss/72205/java-dp-solution-with-explanation
public class Solution {
public int NumSquares(int n) {
int[] dp = new int[n + 1];
for (int i = 1; i <= n; i++) {
dp[i] = int.MaxValue;
}
for (int i = 1; i <= n; i++) {
int sqrt = (int)Math.Sqrt(i);
if (sqrt * sqrt == i) {
dp[i] = 1;
continue;
}
for (int j = 1; j <= sqrt; j++) {
int dif = i - j * j;
dp[i] = Math.Min(dp[i], (dp[dif] + 1));
}
}
return dp
;
}
}