Hough直线检测
2016-01-10 21:07
375 查看
需求:直线检测
目的:实现Hough变换
思路:y = kx + b变换成p = xcos(a) + ysin(a)
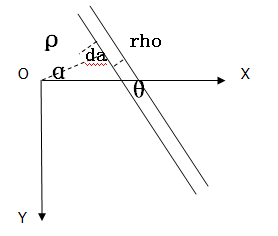
目的:实现Hough变换
思路:y = kx + b变换成p = xcos(a) + ysin(a)
#include "cv.h" #include "highgui.h" #include <iostream> #include <vector> #include <algorithm> #include <exception> using namespace std; typedef struct pLine { float rho; float angle; }pLine; void hough1(CvMat *gray, float theta, float rho, int threshold, int linesMax, vector<pLine>& lines); int main() { IplImage* src = cvLoadImage("1.bmp", 1); const int WIDTH = src->width; const int HEIGHT = src->height; CvMat *gray = cvCreateMat(HEIGHT, WIDTH, CV_8UC1); CvMat *dst = cvCreateMat(HEIGHT, WIDTH, CV_8UC3); cvCvtColor(src, gray, CV_BGR2GRAY); cvCanny(gray, gray, 70, 170); //设置角度精度,距离精度 float theta = 4.0f * CV_PI / 180.0f; float rho = 4.0f; int threshold = 150.0f; int linesMax = 350; vector<pLine>lines; hough1(gray, theta, rho, threshold, linesMax, lines); cout << lines.size(); for (int i = 0; i < lines.size(); i++) { pLine line = lines[i]; CvPoint pt1, pt2; double a = cos(line.angle), b = sin(line.angle); double x0 = a*line.rho, y0 = b*line.rho; pt1.x = cvRound(x0 + 1000*(-b)); pt1.y = cvRound(y0 + 1000*(a)); pt2.x = cvRound(x0 - 1000*(-b)); pt2.y = cvRound(y0 - 1000*(a)); cvLine( src, pt1, pt2, CV_RGB(255,0,0), 3, CV_AA, 0 ); } cvShowImage("SRC", src); cvWaitKey(0); return 0; } void hough1(CvMat *gray, float theta, float rho, int threshold, int linesMax, vector<pLine>& lines) { const int WIDTH = gray->width; const int HEIGHT = gray->height; int numangle = cvRound(CV_PI / theta);; int numrho = cvRound(((WIDTH + HEIGHT) * 2 + 1) / rho); float *tabSin = new float [numangle]; float *tabCos = new float [numangle]; float ang = 0.0f, irho = 1.0f / rho; for(int n = 0; n < numangle; ang += theta, n++ ) { tabSin = (float)(sin((double)ang) * irho); tabCos = (float)(cos((double)ang) * irho); } int * accum = new int [(numangle+2) * (numrho+2)]; memset(accum, 0, (numangle+2) * (numrho+2)*sizeof(int)); // stage 1. fill accumulator for(int j = 0; j < HEIGHT; j ++) { uchar* data = (uchar*)(gray->data.ptr + j * gray->step); for(int i = 0; i < WIDTH; i ++) { if( data[i] != 0 ) { for(int n = 0; n < numangle; n++ ) { int r = cvRound( i * tabCos + j * tabSin ); r += (numrho - 1) / 2; accum[(n+1) * (numrho+2) + r+1]++; } } } } // stage 2. find local maximums vector<int> sort_buf; for(int r = 0; r < numrho; r++ ) { for(int n = 0; n < numangle; n++ ) { int base = (n+1) * (numrho+2) + r+1; if( accum[base] > threshold && accum[base] > accum[base - 1] && accum[base] >= accum[base + 1] && accum[base] > accum[base - numrho - 2] && accum[base] >= accum[base + numrho + 2] ) sort_buf.push_back(base); } } // stage 3. sort the detected lines by accumulator value int total = sort_buf.size(); sort(sort_buf.begin(), sort_buf.end()); reverse(sort_buf.begin(), sort_buf.end()); int linesMax = 150; linesMax = MIN(linesMax, total); float scale = 1.0 / (numrho + 2.0); ; for (int i = 0; i < linesMax; i++) { pLine line; int idx = sort_buf[i]; int n = (int)idx * scale - 1; int r = idx - (n+1)*(numrho+2) - 1; line.rho = (r - (numrho - 1)*0.5f) * rho; line.angle = n * theta; lines.push_back(line); } delete []accum; delete []tabCos; delete []tabSin; }
相关文章推荐
- Hough线变换
- Hough变换检测直线
- Hough直线检测原理及Matlab函数详解
- HOUGH变换检测圆
- Matlab实现Hough直线检测
- 霍夫变换 ( Hough Transform) 直线检测(Matlab 源码)
- 【OpenCV】霍夫线变换
- Kernel-based Hough transform (KHT)移植
- Hough Transform 霍夫变换检测直线
- Hough变换直线检测
- Java 图像智能字符识别技术——【专题三】
- Hough直线检测之我见
- 图像处理之霍夫变换(Hough)
- Hough变换非常详细讲解
- Halcon_Hough变换检测直线,检测圆
- 一种新的直线段检测算法---LSD:a Line Segment Detector
- [图像处理] 实验笔记 - 直线检测(line detection)之霍夫变换
- 【OpenCV笔记 13】OpenCV中Hough霍夫直线检测和同一窗口显示多幅图片
- 【NCRE】——关于Excel中字体的所有属性
- 自学考试-“数据库系统原理”