DWR3使用
2015-12-29 17:24
357 查看
导入官方demo里面的jar包
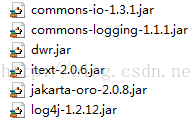
新建DWRScriptSessionListener
package com.dwr.test;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpSession;
import org.directwebremoting.ScriptSession;
import org.directwebremoting.WebContext;
import org.directwebremoting.WebContextFactory;
import org.directwebremoting.event.ScriptSessionEvent;
import org.directwebremoting.event.ScriptSessionListener;
public class DWRScriptSessionListener implements ScriptSessionListener {
// 维护一个Map key为session的Id, value为ScriptSession对象
public static final Map<String, ScriptSession> scriptSessionMap = new HashMap<String, ScriptSession>();
/**
* ScriptSession创建事件
*/
@Override
public void sessionCreated(ScriptSessionEvent event) {
WebContext webContext = WebContextFactory.get();
HttpSession session = webContext.getSession();
ScriptSession scriptSession = event.getSession();
scriptSessionMap.put(session.getId(), scriptSession); // 添加scriptSession
System.out.println("session: " + session.getId() + " scriptSession: " + scriptSession.getId() + "is created!");
}
/**
* ScriptSession销毁事件
*/
@Override
public void sessionDestroyed(ScriptSessionEvent event) {
WebContext webContext = WebContextFactory.get();
HttpSession session = webContext.getSession();
ScriptSession scriptSession = scriptSessionMap.remove(session.getId()); // 移除scriptSession
System.out.println("session: " + session.getId() + " scriptSession: " + scriptSession.getId() + "is destroyed!");
}
/**
* 获取所有ScriptSession
*/
public static Collection<ScriptSession> getScriptSessions() {
return scriptSessionMap.values();
}
}
新建DWRScriptSessionManager
package com.dwr.test;
import org.directwebremoting.impl.DefaultScriptSessionManager;
public class DWRScriptSessionManager extends DefaultScriptSessionManager {
public DWRScriptSessionManager() {
// 绑定一个ScriptSession增加销毁事件的监听器
this.addScriptSessionListener(new DWRScriptSessionListener());
System.out.println("bind DWRScriptSessionListener");
}
}
新建主类MessagePush
package com.dwr.test;
import java.util.Collection;
import org.directwebremoting.Browser;
import org.directwebremoting.ScriptBuffer;
import org.directwebremoting.ScriptSession;
import org.directwebremoting.ScriptSessionFilter;
import org.directwebremoting.WebContextFactory;
public class MessagePush {
public void onPageLoad(final String tag) {
// 获取当前的ScriptSession
ScriptSession scriptSession = WebContextFactory.get().getScriptSession();
scriptSession.setAttribute("tag", tag);
System.out.println("setAttribute");
}
public void send(String msg) {
if(null==msg){
msg="this is a message!";
}
final String content=msg;
// 过滤器
ScriptSessionFilter filter = new ScriptSessionFilter() {
public boolean match(ScriptSession scriptSession) {
String tag = (String) scriptSession.getAttribute
4000
("tag");
System.out.println("tag="+tag);
return "receiverTag".equals(tag);
}
};
Runnable run = new Runnable() {
private ScriptBuffer script = new ScriptBuffer();
public void run() {
// 设置要调用的 js及参数
script.appendCall("show", content);
// 得到所有ScriptSession
Collection<ScriptSession> sessions = DWRScriptSessionListener.getScriptSessions();
// 遍历每一个ScriptSession
for (ScriptSession scriptSession : sessions) {
scriptSession.addScript(script);
}
}
};
// 执行推送
Browser.withAllSessionsFiltered(filter, run); // 注意这里调用了有filter功能的方法
}
}
在web.xml同目录新建dwr.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE dwr PUBLIC
"-//GetAhead Limited//DTD Direct Web Remoting 1.0//EN"
"http://www.getahead.ltd.uk/dwr/dwr30.dtd">
<dwr>
<allow>
<create creator="new" javascript="MessagePush">
<param name="class" value="com.dwr.test.MessagePush" />
</create>
</allow>
</dwr>
在web.xml文件中添加一下配置
<servlet>
<servlet-name>dwr-invoker</servlet-name>
<servlet-class>org.directwebremoting.servlet.DwrServlet</servlet-class>
<init-param>
<param-name>org.directwebremoting.extend.ScriptSessionManager</param-name>
<param-value>com.dwr.test.DWRScriptSessionManager </param-value>
</init-param>
<init-param>
<param-name>debug</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>crossDomainSessionSecurity</param-name>
<param-value>false</param-value>
</init-param>
<init-param>
<param-name>allowScriptTagRemoting</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>pollAndCometEnabled</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>activeReverseAjaxEnabled</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<!-- dwr配置文件路径 -->
<param-name>config</param-name>
<param-value>/WEB-INF/dwr.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dwr-invoker</servlet-name>
<url-pattern>/dwr/*</url-pattern>
</servlet-mapping>
jsp页面
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
<script type="text/javascript" src="js/jquery-1.8.3.min.js"></script>
<script type= "text/javascript" src ="dwr/util.js"></script>
<script type="text/javascript" src= "dwr/engine.js"></script >
<script type="text/javascript" src= "dwr/interface/MessagePush.js" ></script>
<script>
dwr.engine.setActiveReverseAjax(true);
dwr.engine.setNotifyServerOnPageUnload(true,true);
var tag = "receiverTag"; //自定义一个标签
MessagePush.onPageLoad(tag);
dwr.engine.setErrorHandler(function(){});
function show(content){
alert(content);
}
function sendMessage(){
var message = dwr.util.getValue("msg");
MessagePush.send(message);
}
</script>
</head>
<body>
<div align="center">
<p>
Your Message:
<input id="msg" />
<input type="button" value="Send" onclick="sendMessage()" />
</p>
</div>
</body>
</html>
注意需要引用一下几个js
jquery-1.8.3.min.js--页面上使用到了jquery
dwr/util.js-----与下面的js都是dwr内置的,固定写法
dwr/engine.js
dwr/interface/MessagePush.js---这个是我们在dwr.xml中配置的 javascript="MessagePush",名字对应
效果
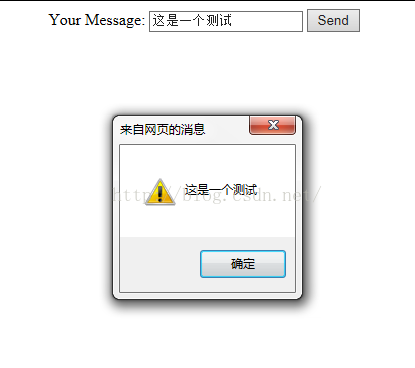
在文本框中输入一句话,然后点击send按钮,后台接收到消息后推送到前台页面
新建DWRScriptSessionListener
package com.dwr.test;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpSession;
import org.directwebremoting.ScriptSession;
import org.directwebremoting.WebContext;
import org.directwebremoting.WebContextFactory;
import org.directwebremoting.event.ScriptSessionEvent;
import org.directwebremoting.event.ScriptSessionListener;
public class DWRScriptSessionListener implements ScriptSessionListener {
// 维护一个Map key为session的Id, value为ScriptSession对象
public static final Map<String, ScriptSession> scriptSessionMap = new HashMap<String, ScriptSession>();
/**
* ScriptSession创建事件
*/
@Override
public void sessionCreated(ScriptSessionEvent event) {
WebContext webContext = WebContextFactory.get();
HttpSession session = webContext.getSession();
ScriptSession scriptSession = event.getSession();
scriptSessionMap.put(session.getId(), scriptSession); // 添加scriptSession
System.out.println("session: " + session.getId() + " scriptSession: " + scriptSession.getId() + "is created!");
}
/**
* ScriptSession销毁事件
*/
@Override
public void sessionDestroyed(ScriptSessionEvent event) {
WebContext webContext = WebContextFactory.get();
HttpSession session = webContext.getSession();
ScriptSession scriptSession = scriptSessionMap.remove(session.getId()); // 移除scriptSession
System.out.println("session: " + session.getId() + " scriptSession: " + scriptSession.getId() + "is destroyed!");
}
/**
* 获取所有ScriptSession
*/
public static Collection<ScriptSession> getScriptSessions() {
return scriptSessionMap.values();
}
}
新建DWRScriptSessionManager
package com.dwr.test;
import org.directwebremoting.impl.DefaultScriptSessionManager;
public class DWRScriptSessionManager extends DefaultScriptSessionManager {
public DWRScriptSessionManager() {
// 绑定一个ScriptSession增加销毁事件的监听器
this.addScriptSessionListener(new DWRScriptSessionListener());
System.out.println("bind DWRScriptSessionListener");
}
}
新建主类MessagePush
package com.dwr.test;
import java.util.Collection;
import org.directwebremoting.Browser;
import org.directwebremoting.ScriptBuffer;
import org.directwebremoting.ScriptSession;
import org.directwebremoting.ScriptSessionFilter;
import org.directwebremoting.WebContextFactory;
public class MessagePush {
public void onPageLoad(final String tag) {
// 获取当前的ScriptSession
ScriptSession scriptSession = WebContextFactory.get().getScriptSession();
scriptSession.setAttribute("tag", tag);
System.out.println("setAttribute");
}
public void send(String msg) {
if(null==msg){
msg="this is a message!";
}
final String content=msg;
// 过滤器
ScriptSessionFilter filter = new ScriptSessionFilter() {
public boolean match(ScriptSession scriptSession) {
String tag = (String) scriptSession.getAttribute
4000
("tag");
System.out.println("tag="+tag);
return "receiverTag".equals(tag);
}
};
Runnable run = new Runnable() {
private ScriptBuffer script = new ScriptBuffer();
public void run() {
// 设置要调用的 js及参数
script.appendCall("show", content);
// 得到所有ScriptSession
Collection<ScriptSession> sessions = DWRScriptSessionListener.getScriptSessions();
// 遍历每一个ScriptSession
for (ScriptSession scriptSession : sessions) {
scriptSession.addScript(script);
}
}
};
// 执行推送
Browser.withAllSessionsFiltered(filter, run); // 注意这里调用了有filter功能的方法
}
}
在web.xml同目录新建dwr.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE dwr PUBLIC
"-//GetAhead Limited//DTD Direct Web Remoting 1.0//EN"
"http://www.getahead.ltd.uk/dwr/dwr30.dtd">
<dwr>
<allow>
<create creator="new" javascript="MessagePush">
<param name="class" value="com.dwr.test.MessagePush" />
</create>
</allow>
</dwr>
在web.xml文件中添加一下配置
<servlet>
<servlet-name>dwr-invoker</servlet-name>
<servlet-class>org.directwebremoting.servlet.DwrServlet</servlet-class>
<init-param>
<param-name>org.directwebremoting.extend.ScriptSessionManager</param-name>
<param-value>com.dwr.test.DWRScriptSessionManager </param-value>
</init-param>
<init-param>
<param-name>debug</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>crossDomainSessionSecurity</param-name>
<param-value>false</param-value>
</init-param>
<init-param>
<param-name>allowScriptTagRemoting</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>pollAndCometEnabled</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>activeReverseAjaxEnabled</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<!-- dwr配置文件路径 -->
<param-name>config</param-name>
<param-value>/WEB-INF/dwr.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dwr-invoker</servlet-name>
<url-pattern>/dwr/*</url-pattern>
</servlet-mapping>
jsp页面
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
<script type="text/javascript" src="js/jquery-1.8.3.min.js"></script>
<script type= "text/javascript" src ="dwr/util.js"></script>
<script type="text/javascript" src= "dwr/engine.js"></script >
<script type="text/javascript" src= "dwr/interface/MessagePush.js" ></script>
<script>
dwr.engine.setActiveReverseAjax(true);
dwr.engine.setNotifyServerOnPageUnload(true,true);
var tag = "receiverTag"; //自定义一个标签
MessagePush.onPageLoad(tag);
dwr.engine.setErrorHandler(function(){});
function show(content){
alert(content);
}
function sendMessage(){
var message = dwr.util.getValue("msg");
MessagePush.send(message);
}
</script>
</head>
<body>
<div align="center">
<p>
Your Message:
<input id="msg" />
<input type="button" value="Send" onclick="sendMessage()" />
</p>
</div>
</body>
</html>
注意需要引用一下几个js
jquery-1.8.3.min.js--页面上使用到了jquery
dwr/util.js-----与下面的js都是dwr内置的,固定写法
dwr/engine.js
dwr/interface/MessagePush.js---这个是我们在dwr.xml中配置的 javascript="MessagePush",名字对应
效果
在文本框中输入一句话,然后点击send按钮,后台接收到消息后推送到前台页面
相关文章推荐
- 神经网络中的BPTT算法简单介绍
- 判断两个UIColor的颜色值是否相等
- Android之JAVASe基础篇-面向对象-对象数组(三)
- php取数组随机数
- TexturePacker非常棒的游戏资源图像处理工具
- TCP慢启动、拥塞避免、快速重传、快速回复
- NAS环境中的备份:服务器、NDMP协议
- notebook 的使用
- Python 使用 xlrd 读取 Excel格式文件
- UIAlertView使用
- HTML5的新特性
- CoreGraphics --- 翻转坐标系
- Ios - 计算Label文字中字体的frame - 实用代码
- Access restriction:The type JPEGCodec is not accessible due to restriction on required library C:\Program Files\Java\jre6\lib\rt.jar
- libevent 编译 Windows
- Educational Codeforces Round 3 B. The Best Gift
- 在Android的webview中定制js的alert,confirm和prompt对话框的方法
- Cron表达式
- js 实现ReplaceAll 的方法
- 识别二维码图片