设计模式(六):组合模式(Composite)
2015-12-19 22:31
381 查看
参考书籍:《设计模式 - 可复用面向对象软件的基础》GoF
参考链接:
目录
1.介绍/作用:
2.应用场景:
3.UML类图
4.实现代码
5.扩展/补充
1.介绍/作用:
将对象组合成树形结构以表示“部分 -整体”的层次结构。C o m p o s i t e使得用户对单个对象
和组合对象的使用具有一致性。
2.应用场景:
在绘图编辑器和图形捕捉系统这样的图形应用程序中,用户可以使用简单的组件创建复
杂的图表。用户可以组合多个简单组件以形成一些较大的组件,这些组件又可以组合成更大
的组件。一个简单的实现方法是为 Te x t和L i n e这样的图元定义一些类,另外定义一些类作为这
些图元的容器类( C o n t a i n e r )。
然而这种方法存在一个问题:使用这些类的代码必须区别对待图元对象与容器对象,而
实际上大多数情况下用户认为它们是一样的。对这些类区别使用,使得程序更加复杂。
C o m p o s i t e模式描述了如何使用递归组合,使得用户不必对这些类进行区别,如下图所示。
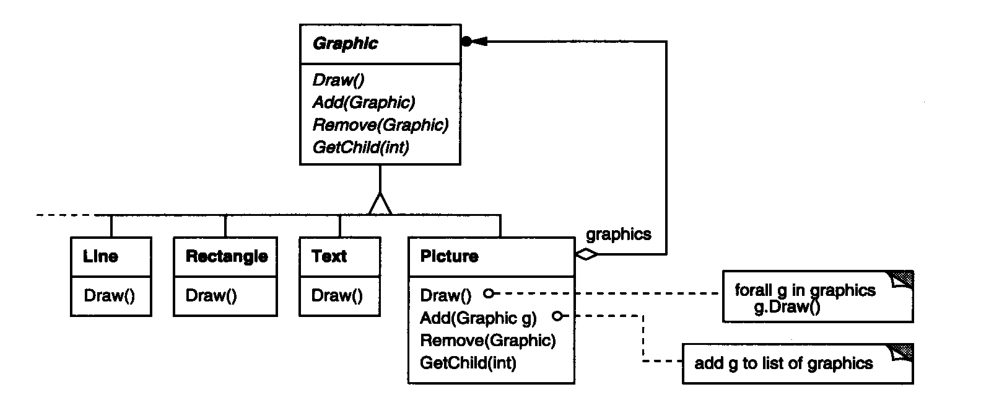
下图是一个典型的由递归组合的G r a p h i c对象组成的组合对象结构。
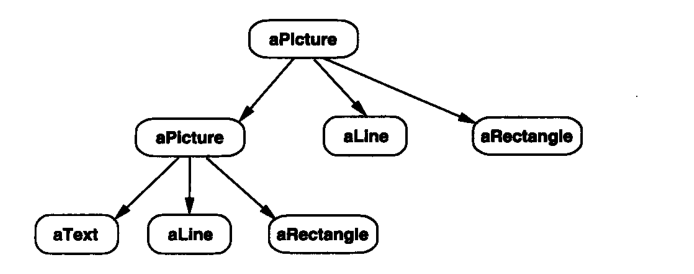
3.UML类图
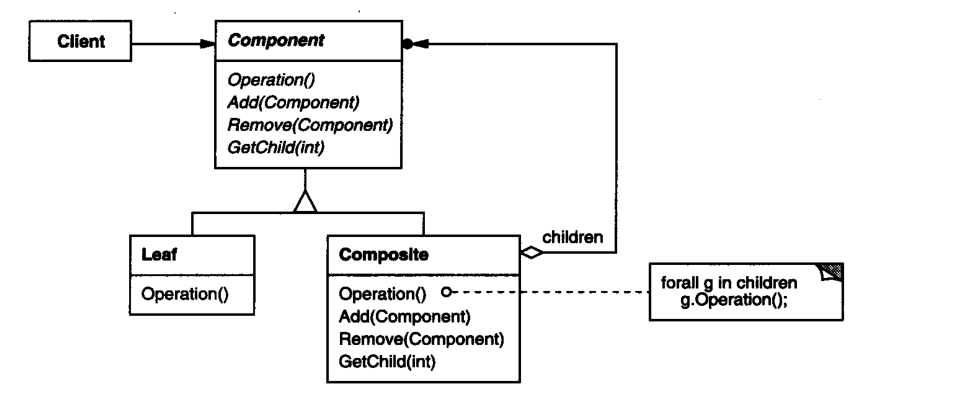
典型的Composite对象结构如下图:
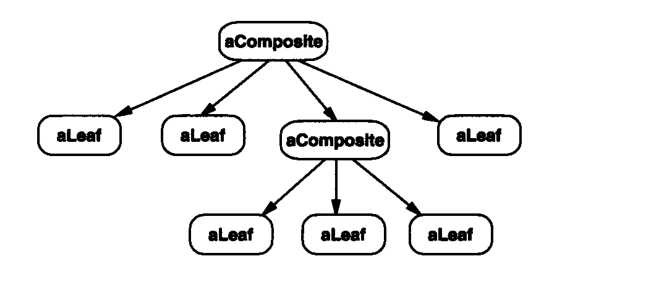
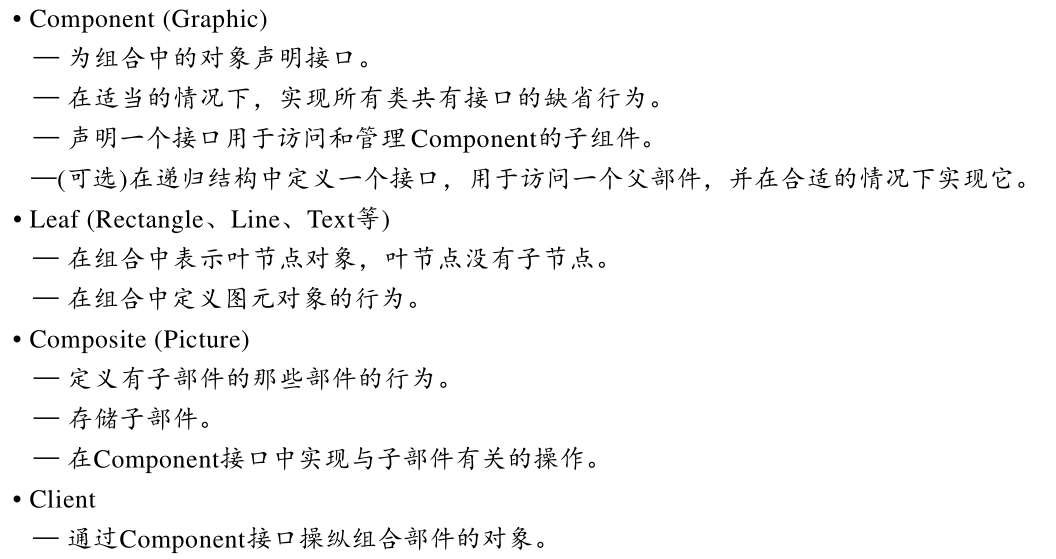
4.实现代码
输出:
Composite Operation
LeafB Operation
LeafA Operation
Composite Operation
LeafB Operation
LeafB Operation
LeafB Operation
LeafA Operation
LeafA Operation
释放list的堆内存
请按任意键继续. . .
5.扩展/补充
参考链接:
目录
1.介绍/作用:
2.应用场景:
3.UML类图
4.实现代码
5.扩展/补充
1.介绍/作用:
将对象组合成树形结构以表示“部分 -整体”的层次结构。C o m p o s i t e使得用户对单个对象
和组合对象的使用具有一致性。
2.应用场景:
在绘图编辑器和图形捕捉系统这样的图形应用程序中,用户可以使用简单的组件创建复
杂的图表。用户可以组合多个简单组件以形成一些较大的组件,这些组件又可以组合成更大
的组件。一个简单的实现方法是为 Te x t和L i n e这样的图元定义一些类,另外定义一些类作为这
些图元的容器类( C o n t a i n e r )。
然而这种方法存在一个问题:使用这些类的代码必须区别对待图元对象与容器对象,而
实际上大多数情况下用户认为它们是一样的。对这些类区别使用,使得程序更加复杂。
C o m p o s i t e模式描述了如何使用递归组合,使得用户不必对这些类进行区别,如下图所示。
下图是一个典型的由递归组合的G r a p h i c对象组成的组合对象结构。
3.UML类图
典型的Composite对象结构如下图:
4.实现代码
#ifndef _COMPOSITE_H_ #define _COMPOSITE_H_ #include <iostream> #include <list> using namespace std; //父类 class Component { public: Component(){}; virtual ~Component(){} virtual void Operation() { cout << "Component Operation" << endl; }; virtual void Add(Component*) {}; virtual void Remove(Component*) {}; virtual Component* GetChild(int){ return nullptr; }; private: }; //组合子类 class Composite :public Component { public: Composite(){}; virtual ~Composite() { cout << "释放list的堆内存" << endl; for each (Component* var in ComponentList)//释放list的堆内存 { if (var) { delete var; var = nullptr; } } } virtual void Operation() { cout << "Composite Operation" << endl; for each (Component* var in ComponentList)//释放list的堆内存 { var->Operation(); } } virtual void Add(Component* pComponent) { ComponentList.push_back(pComponent); } virtual void Remove(Component* pComponent) { ComponentList.remove(pComponent); } virtual Component* GetChild(int nPos) { list<Component*>::iterator iter = ComponentList.begin(); while ((--nPos) > 0) iter++; return *iter; } private: list<Component*> ComponentList; }; //实现功能的子类:A,B... class LeafA :public Component { public: LeafA(){}; virtual ~LeafA(){}; virtual void Operation() { cout << "LeafA Operation" << endl; } private: }; class LeafB :public Component { public: LeafB(){}; virtual ~LeafB(){}; virtual void Operation() { cout << "LeafB Operation" << endl; } private: }; #endif
LeafB* pLeafB1 = new LeafB(); LeafB* pLeafB2 = new LeafB(); LeafB* pLeafB3 = new LeafB(); LeafA* pLeafA1 = new LeafA(); LeafA* pLeafA2 = new LeafA(); //第一个组合 Composite* pComposite = new Composite(); pComposite->Add(pLeafB1); pComposite->Add(pLeafB2); pComposite->Add(pLeafB3); pComposite->Add(pLeafA1); pComposite->Add(pLeafA2); //另一个组合 LeafB* pLeafB4 = new LeafB(); LeafA* pLeafA3 = new LeafA(); Composite* pComposite2 = new Composite(); pComposite2->Add(pLeafB4); pComposite2->Add(pLeafA3); //将组合加入组合 pComposite2->Add(pComposite); //operataion pComposite2->Operation(); if (pComposite) { delete pComposite; pComposite = nullptr; } return 0;
输出:
Composite Operation
LeafB Operation
LeafA Operation
Composite Operation
LeafB Operation
LeafB Operation
LeafB Operation
LeafA Operation
LeafA Operation
释放list的堆内存
请按任意键继续. . .
5.扩展/补充
相关文章推荐
- keil MDK之RTX事件标志组的API函数
- 网络之Http字段介绍
- 数据库SQL优化大总结之百万级数据库优化方案
- LR11录制回放出现中文乱码以及录制时一直跳到360浏览器的解决方法
- 摄影软件“留拍”开发现状与过程遇到的困难
- 常用oracle函数备份
- jquery返回顶部,支持手机
- android中广播的使用
- 线上Nginx状态码为400解决
- MySql索引的优缺点
- 移动互联网时代的数据创业
- 张氏读写锁,c++信号量实现
- 提高WPF程序性能的几条建议
- 用户试用体验报告
- iOS开发中UIPopoverController的使用详解
- [转]Mac OS X El Capitan(10.11)显示隐藏文件命令失效解决方法
- dz论坛程序备份搬家
- ROC曲线与AUC
- 工厂方法模式
- Spark Shuffle内存分析