第3周项目2 建设“顺序表”算法库
2015-09-18 12:46
591 查看
问题及描述:
1)main.cpp
/*
*文件名称:main.cpp,list.cpp,list.h
*作者:郑孚嘉
*问题描述:建设“顺序表”算法库
领会“0207将算法变程序”部分建议的方法,建设自己的专业基础设施算法库。这一周,建的是顺序表的算法库。
算法库包括两个文件:
头文件:list.h,包含定义顺序表数据结构的代码、宏定义、要实现算法的函数的声明;
源文件:list.cpp,包含实现各种算法的函数的定义
请采用程序的多文件组织形式,在项目1的基础上,建立如上的两个文件,另外再建立一个源文件,编制main函数,完成相关的测试工作。
*/
#include "list.h"
int main()
{
SqList *Sq;
ElemType a[5]={1,2,3,4,5};
CreateList(Sq,a,5);
if(ListEmpty(Sq)==true)
{
printf("False !");
return 0;
}
printf("CreatList !\n");
DispList(Sq);
printf("ListLength:%d\n",ListLength(Sq));
ElemType e;
if(GetElem(Sq,3,e)==true)
{
printf("GetElem 3: %d\n",e);
}
printf("LocateElem 3: %d\n",LocateElem(Sq,3));
if(ListInsert(Sq,2,5)==true)
{
printf("ListInsert 5 !\n");
DispList(Sq);
}
if(ListDelete(Sq,4,e)==true)
{
printf("ListDelete 3 !\n");
DispList(Sq);
}
return 0;
}
2)list.h
3)list.cpp
运行结果:
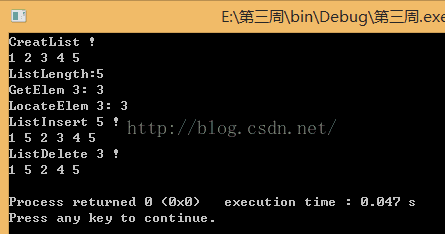
知识点总结:
list.cpp是对顺序表各种算法函数的总结。
ListInsert 和ListDelete中的e,后者采取引用,是因为Delete的值要传给e,所以运用引用。
1)main.cpp
/*
*文件名称:main.cpp,list.cpp,list.h
*作者:郑孚嘉
*问题描述:建设“顺序表”算法库
领会“0207将算法变程序”部分建议的方法,建设自己的专业基础设施算法库。这一周,建的是顺序表的算法库。
算法库包括两个文件:
头文件:list.h,包含定义顺序表数据结构的代码、宏定义、要实现算法的函数的声明;
源文件:list.cpp,包含实现各种算法的函数的定义
请采用程序的多文件组织形式,在项目1的基础上,建立如上的两个文件,另外再建立一个源文件,编制main函数,完成相关的测试工作。
*/
#include "list.h"
int main()
{
SqList *Sq;
ElemType a[5]={1,2,3,4,5};
CreateList(Sq,a,5);
if(ListEmpty(Sq)==true)
{
printf("False !");
return 0;
}
printf("CreatList !\n");
DispList(Sq);
printf("ListLength:%d\n",ListLength(Sq));
ElemType e;
if(GetElem(Sq,3,e)==true)
{
printf("GetElem 3: %d\n",e);
}
printf("LocateElem 3: %d\n",LocateElem(Sq,3));
if(ListInsert(Sq,2,5)==true)
{
printf("ListInsert 5 !\n");
DispList(Sq);
}
if(ListDelete(Sq,4,e)==true)
{
printf("ListDelete 3 !\n");
DispList(Sq);
}
return 0;
}
2)list.h
#include <stdio.h> #include <malloc.h> #define MaxSize 50 typedef int ElemType; typedef struct { int length; ElemType data[MaxSize]; }SqList; void CreateList(SqList *&L,ElemType a[],int n); bool ListEmpty(SqList *L); void DispList(SqList *L); void InitList(SqList *&L); void DestroyList(SqList *&L); int ListLength(SqList *L); bool GetElem(SqList *L,int i,ElemType &e); int LocateElem(SqList *L,ElemType e); bool ListInsert(SqList *&L,int i,ElemType e); bool ListDelete(SqList *&L,int i,ElemType &e);
3)list.cpp
#include "list.h" void CreateList(SqList *&L,ElemType a[],int n) { int i; L=(SqList *) malloc (sizeof(SqList)); for(i=0;i<n;i++) { L->data[i]=a[i]; } L->length=n; } bool ListEmpty(SqList *L) { return (L->length==0); } void DispList(SqList *L) { int i; for(i=0;i<L->length;i++) { printf("%d ",L->data[i]); } printf("\n"); } void InitList(SqList *&L) { L=(SqList *) malloc (sizeof(SqList)); L->length=0; } void DestroyList(SqList *&L) { free(L); } int ListLength(SqList *L) { return(L->length); } bool GetElem(SqList *L,int i,ElemType &e) { if(i<1||i>L->length) { return false; } e=L->data[i-1]; return true; } int LocateElem(SqList *L,ElemType e) { int i=0; while(i<L->length&&L->data[i]!=e) { i++; } if(i>=L->length) { return 0; } else { return i+1; } } bool ListInsert(SqList *&L,int i,ElemType e) { int j; if(i<1||i>L->length+1) { return false; } i--; for(j=L->length;j>i;j--) { L->data[j]=L->data[j-1]; } L->data[i]=e; L->length++; return true; } bool ListDelete(SqList *&L,int i,ElemType &e) { int j; if(i<1||i>L->length) { return false; } i--; e=L->data[i]; for(j=i;j<L->length-1;j++) { L->data[j]=L->data[j+1]; } L->length--; return true; }
运行结果:
知识点总结:
list.cpp是对顺序表各种算法函数的总结。
ListInsert 和ListDelete中的e,后者采取引用,是因为Delete的值要传给e,所以运用引用。
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- 关于指针的一些事情
- c++ primer 第五版 笔记前言
- share_ptr的几个注意点
- Lua中调用C++函数示例
- Lua和C语言的交互详解
- Lua教程(一):在C++中嵌入Lua脚本
- Lua教程(二):C++和Lua相互传递数据示例
- C++联合体转换成C#结构的实现方法
- 关于C语言中参数的传值问题
- 简要对比C语言中三个用于退出进程的函数
- 深入C++中API的问题详解
- 基于C语言string函数的详解
- C++编写简单的打靶游戏
- C++ 自定义控件的移植问题
- C语言中fchdir()函数和rewinddir()函数的使用详解
- C语言内存对齐实例详解
- C++变位词问题分析
- C/C++数据对齐详细解析
- C++基于栈实现铁轨问题