Reading Text-based Files In ASP.NET
2015-09-16 15:49
716 查看
Friday, July 17, 2015 1:43 PM
Every time I need to work with the contents of text-based files in an ASP.NET application I invariably start off thinking about using the various static methods on the
The Microsoft.VisualBasic library is a collection of namespaces containing a miscellany of components and utilities. Most of them seem to provide VB6 developers with something that they will be familiar with such as .NET implementations of the string-related
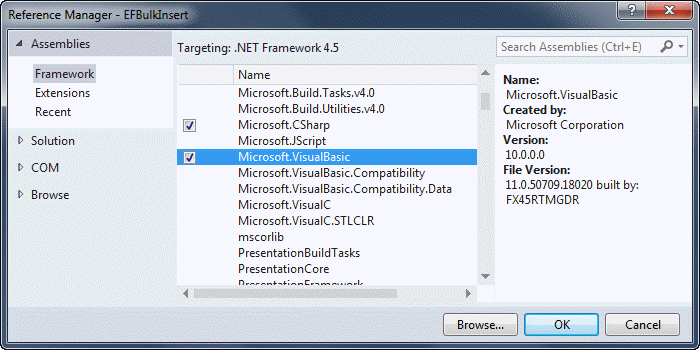
A
The
Finally, here's an example of the constructor that accepts a concrete implementation of the
Having instantiated and configured a
The sample above doesn't have a header. The following sample features the same data with a header row, and illustrates how to copy the whole thing into a DataTable:
The final example shows how to apply the
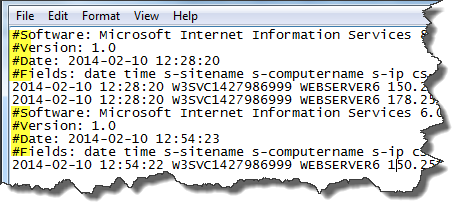
In this case, because the parser is configured to ignore lines beginning with the specified comment token, a bit of additional code is used to extract the field headers for the
原文链接:http://www.mikesdotnetting.com/article/279/reading-text-based-files-in-asp-net
Every time I need to work with the contents of text-based files in an ASP.NET application I invariably start off thinking about using the various static methods on the
System.IO.Fileclass to extract the text and then some string manipulation or Regex to parse the content into some kind of structure. And, just in time, I remember the
TextFieldParserclass that hides itself away in the
Microsoft.VisualBasicassembly. The purpose of this article is to introduce this component to a wider audience, but also to serve me as an aide-mémoire in terms of the basic usage, which I always have to look up.
The Microsoft.VisualBasic library is a collection of namespaces containing a miscellany of components and utilities. Most of them seem to provide VB6 developers with something that they will be familiar with such as .NET implementations of the string-related
Leftand
Rightmethods, but despite its name (and the fact that MSDN examples are all VB-only), the library is pure .NET code and can be used with any .NET compliant language. C# projects do not include a reference to Microsoft.VisualBasic by default, so you need to use the Add References dialog to add it yourself:
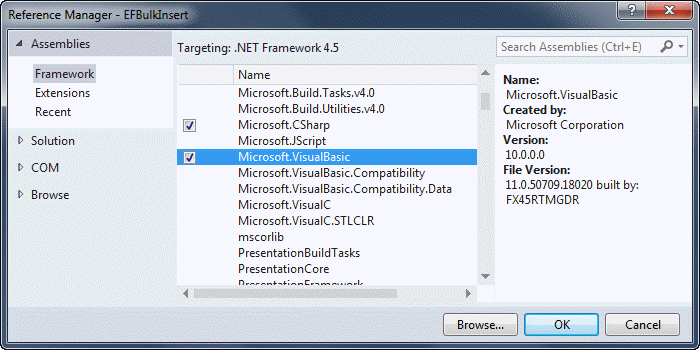
A
TextFieldParserinstance can be initialised from a number of sources: a stream, a physical file on disk, or a
TextReader. The first two options are likely to be used more often in ASP.NET applications. This first example illustrates creating a
TextFieldParserfrom an uploaded file in an MVC application:
[HttpPost] public ActionResult Index(HttpPostedFileBase file) { if (file != null) { if (file.ContentLength > 0) { using (var parser = new TextFieldParser(file.InputStream)) { // ... } } } return View(); }
The
TextFieldParseris instantiated within a
usingblock because it implements
IDisposable, and the
usingblock ensures that the object will be displosed of safely and correctly. The next example sees a file path passed to the
TextFieldParserconstructor:
var file = @"C:\test.csv"; using (var parser = new TextFieldParser(file)) { //.. }
Finally, here's an example of the constructor that accepts a concrete implementation of the
TextReader:
var csv = @"1,Mike,Brind,www.mikesdotnetting.com"; using (var parser = new TextFieldParser(new StringReader(csv))) { // ... }
Configuration
Configuration options are set through properties and methods. The key options are featured below:Option | Description | Default |
---|---|---|
Delimiters(property) | Specifies the field delimiters used in the text file. | null |
SetDelimiters(method) | Alterntative way to specify the field delimiters used in the file | |
TextFieldType(property) | Specify whether the file is Delimitedor FixedWidth | TextFieldType.Delimited |
HasFieldsEnclosedInQuotes(property) | Booleanindicating whether text fields are enclosed in quotes | true |
FieldWidths(property) | An array of intsspecifying the widths of individual fields in a fixed width file | null |
SetFieldWidths(method) | An alternative way to specify the widths of individual fields in a fixed width file | |
CommentTokens(property) | An array specifying the tokens used to indicate comments in the file | null |
TrimWhiteSpace(property) | Booleanindicating whether leading and trailing white space should be removed from fields | true |
TextFieldParser, you will want to start accessing the data in the text file. The parser has a
ReadFieldsmethod that gobbles up content a line at a time. It returns an array of strings. It also has an
EndOfDataproperty which indicates whether there are any more lines of data to be read. The following code shows how to use this property and method in combination to read each line of data in a simple example:
var data = @"1,Potato,Vegetable 2,Strawberry,Fruit 3,Carrot,Vegetable 4,Milk,Dairy, 5,Apple,Fruit 6,Bread,Cereal"; using (var parser = new TextFieldParser(new StringReader(data))) { parser.Delimiters = new[] { "," }; while (!parser.EndOfData) { var row = parser.ReadFields(); var foodType = row[2]; } }
The sample above doesn't have a header. The following sample features the same data with a header row, and illustrates how to copy the whole thing into a DataTable:
var data = @"Id,Food,FoodType 1,Potato,Vegetable 2,Strawberry,Fruit 3,Carrot,Vegetable 4,Milk,Dairy, 5,Apple,Fruit 6,Bread,Cereal"; using (var parser = new TextFieldParser(new StringReader(data))) { var headerRow = true; var dt = new DataTable(); parser.Delimiters = new[] { "," }; while (!parser.EndOfData) { var currentRow = parser.ReadFields(); if (headerRow) { foreach (var field in currentRow) { dt.Columns.Add(field, typeof(object)); } headerRow = false; } else { dt.Rows.Add(currentRow); } } }
The final example shows how to apply the
CommentTokensproperty to be able to read a standard IIS log file into a
DataTablewhere comment lines are prefixed with a hash (
#) symbol:
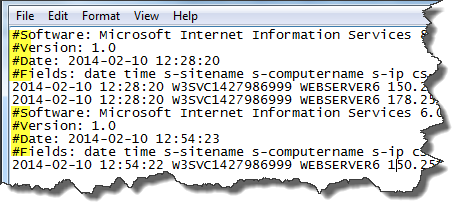
var file = @"C:\Logs\W3SVC6\ex140210.log"; var commentTokens = new[] { "#" }; var headerRow = File.ReadAllLines(file).First(l => l.StartsWith("#Fields:")); using (var parser = new TextFieldParser(file)) { var dt = new DataTable(); var columns = headerRow.Replace("#Fields: ", string.Empty).Split(new[] { ' ' }, StringSplitOptions.RemoveEmptyEntries); foreach (var column in columns) { dt.Columns.Add(column, typeof(object)); } parser.SetDelimiters(" "); parser.CommentTokens = commentTokens; while (!parser.EndOfData) { { dt.Rows.Add(parser.ReadFields()); } } }
In this case, because the parser is configured to ignore lines beginning with the specified comment token, a bit of additional code is used to extract the field headers for the
DataTablecolumn names.
Summary
Next time you need to parse a text file in a .NET application, rather than reaching for string manipulation functions, you could consider using theTextFieldParserin the Microsoft.VisualBasic library.
原文链接:http://www.mikesdotnetting.com/article/279/reading-text-based-files-in-asp-net
相关文章推荐
- ASP.NET MVC4系列验证机制、伙伴类共享源数据信息(数据注解和验证)
- ASP.NET MVC3手把手教你构建Web
- [Asp.net]Uploadify上传大文件
- ASP.NET MVC5网站开发文章管理架构(七)
- ASP.NET MVC5网站开发用户修改资料和密码(六)
- Asp.Net获取GridView当前行的方法
- Asp编码优化技巧
- ASP提速五大技巧
- asp.net request 获取前台数据 disabled
- ASP.NET MVC中的统一化自定义异常处理
- asp.net 站内搜索功能的实现及利用datatable绑定数据并进行分页
- 给ASP.NET MVC及WebApi添加路由优先级
- asp.net MVC中form提交和控制器接受form提交过来的数据
- ASP.NET整个执行过程
- ASP.NET配置文件
- ASP.NET页面生命周期
- ASP.NET页面跳转方法的集合
- ASP.NET Request对象
- ASP.NET中异常处理
- 文本框中输入小写字母转换为大写