java小程序: 简易计算器
2015-08-10 10:22
645 查看
这是《java核心技术 卷一》里面的例9-1,本人觉得写得很好,特收藏在这里。
分析:
1、添加窗体Frame,作为容器在上面添加控件
2、整个界面可分为上下两个面板Panel,可先在上面的面板添加文本显示TextField,再在下面的面板采用4x4网格布局.
3、在下面的面板添加按钮(Button),按钮分数字符号(insert)和运算符号(command)两种,这个动作可以写成一个方法addButton(String label, ActionListener listener)
4、数字按钮监听事件将数字以字符串方式追加显示,运算按钮进行运算(这个动作写成一个方法在外面)。注意:在这一步里面,我注释了原来的代码,导致不能连续运算。
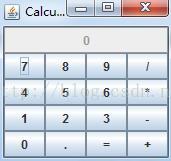
这个是网上看得的:
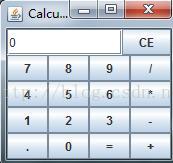
package project;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* A simple calculator program.
* <p>I saw this program in a QQ group, and help a friend correct it.</p>
*
* @author Singyuen Yip
* @version 1.00 12/29/2009
* @see JFrame
* @see ActionListener
*/
public class JCalculator extends JFrame implements ActionListener {
private static final long serialVersionUID = -169068472193786457L;
/**
* This class help close the Window.
* @author Singyuen Yip
*
*/
private class WindowCloser extends WindowAdapter {
public void windowClosing(WindowEvent we) {
System.exit(0);
}
}
int i;
// Strings for Digit & Operator buttons.
private final String[] str = { "7", "8", "9", "/", "4", "5", "6", "*", "1",
"2", "3", "-", ".", "0", "=", "+" };
// Build buttons.
JButton[] buttons = new JButton[str.length];
// For cancel or reset.
JButton reset = new JButton("CE");
// Build the text field to show the result.
JTextField display = new JTextField("0");
/**
* Constructor without parameters.
*/
public JCalculator() {
super("Calculator");
// Add a panel.
JPanel panel1 = new JPanel(new GridLayout(4, 4));
// panel1.setLayout(new GridLayout(4,4));
for (i = 0; i < str.length; i++) {
buttons[i] = new JButton(str[i]);
panel1.add(buttons[i]);
}
JPanel panel2 = new JPanel(new BorderLayout());
// panel2.setLayout(new BorderLayout());
panel2.add("Center", display);
panel2.add("East", reset);
// JPanel panel3 = new Panel();
getContentPane().setLayout(new BorderLayout());
getContentPane().add("North", panel2);
getContentPane().add("Center", panel1);
// Add action listener for each digit & operator button.
for (i = 0; i < str.length; i++)
buttons[i].addActionListener(this);
// Add listener for "reset" button.
reset.addActionListener(this);
// Add listener for "display" button.
display.addActionListener(this);
// The "close" button "X".
addWindowListener(new WindowCloser());
// Initialize the window size.
setSize(800, 800);
// Show the window.
// show(); Using show() while JDK version is below 1.5.
setVisible(true);
// Fit the certain size.
pack();
}
public void actionPerformed(ActionEvent e) {
Object target = e.getSource();
String label = e.getActionCommand();
if (target == reset)
handleReset();
else if ("0123456789.".indexOf(label) > 0)
handleNumber(label);
else
handleOperator(label);
}
// Is the first digit pressed?
boolean isFirstDigit = true;
/**
* Number handling.
* @param key the key of the button.
*/
public void handleNumber(String key) {
if (isFirstDigit)
display.setText(key);
else if ((key.equals(".")) && (display.getText().indexOf(".") < 0))
display.setText(display.getText() + ".");
else if (!key.equals("."))
display.setText(display.getText() + key);
isFirstDigit = false;
}
a022
/**
* Reset the calculator.
*/
public void handleReset() {
display.setText("0");
isFirstDigit = true;
operator = "=";
}
double number = 0.0;
String operator = "=";
/**
* Handling the operation.
* @param key pressed operator's key.
*/
public void handleOperator(String key) {
if (operator.equals("+"))
number += Double.valueOf(display.getText());
else if (operator.equals("-"))
number -= Double.valueOf(display.getText());
else if (operator.equals("*"))
number *= Double.valueOf(display.getText());
else if (operator.equals("/"))
number /= Double.valueOf(display.getText());
else if (operator.equals("="))
number = Double.valueOf(display.getText());
display.setText(String.valueOf(number));
operator = key;
isFirstDigit = true;
}
public static void main(String[] args) {
new JCalculator();
}
}
分析:
1、添加窗体Frame,作为容器在上面添加控件
2、整个界面可分为上下两个面板Panel,可先在上面的面板添加文本显示TextField,再在下面的面板采用4x4网格布局.
3、在下面的面板添加按钮(Button),按钮分数字符号(insert)和运算符号(command)两种,这个动作可以写成一个方法addButton(String label, ActionListener listener)
4、数字按钮监听事件将数字以字符串方式追加显示,运算按钮进行运算(这个动作写成一个方法在外面)。注意:在这一步里面,我注释了原来的代码,导致不能连续运算。
<span style="color:#3333ff;">import java.awt.*; import java.awt.event.*; import javax.swing.*; /** * @version 1.33 2007-06-12 * @author Cay Horstmann */ public class Calculator { public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { CalculatorFrame frame = new CalculatorFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }); } } /** * A frame with a calculator panel. */ class CalculatorFrame extends JFrame { public CalculatorFrame() { setTitle("Calculator"); CalculatorPanel panel = new CalculatorPanel(); add(panel); pack(); } } /** * A panel with calculator buttons and a result display. */ class CalculatorPanel extends JPanel { public CalculatorPanel() { setLayout(new BorderLayout()); result = 0; lastCommand = "="; start = true; // add the display display = new JButton("0"); display.setEnabled(false); add(display, BorderLayout.NORTH); ActionListener insert = new InsertAction(); ActionListener command = new CommandAction(); // add the buttons in a 4 x 4 grid panel = new JPanel(); panel.setLayout(new GridLayout(4, 4)); addButton("7", insert); addButton("8", insert); addButton("9", insert); addButton("/", command); addButton("4", insert); addButton("5", insert); addButton("6", insert); addButton("*", command); addButton("1", insert); addButton("2", insert); addButton("3", insert); addButton("-", command); addButton("0", insert); addButton(".", insert); addButton("=", command); addButton("+", command); add(panel, BorderLayout.CENTER); } /** * Adds a button to the center panel. * @param label the button label * @param listener the button listener */ private void addButton(String label, ActionListener listener) { JButton button = new JButton(label); button.addActionListener(listener); panel.add(button); } /** * This action inserts the button action string to the end of the display text. */ private class InsertAction implements ActionListener { public void actionPerformed(ActionEvent event) { String input = event.getActionCommand(); if (start) { display.setText(""); start = false; } display.setText(display.getText() + input); } } /** * This action executes the command that the button action string denotes. */ private class CommandAction implements ActionListener { public void actionPerformed(ActionEvent event) { String command = event.getActionCommand(); if (start) { /*if (command.equals("-")) { display.setText(command); start = false; } else lastCommand = command;*/ display.setText("please input number first!"); } else { calculate(Double.parseDouble(display.getText())); lastCommand = command; start = true; } } } /** * Carries out the pending calculation. * @param x the value to be accumulated with the prior result. */ public void calculate(double x) { if (lastCommand.equals("+")) result += x; else if (lastCommand.equals("-")) result -= x; else if (lastCommand.equals("*")) result *= x; else if (lastCommand.equals("/")) result /= x; else if (lastCommand.equals("=")) result = x; //display.setText(result); display.setText(""+result); } private JButton display; private JPanel panel; private double result; private String lastCommand; private boolean start; }</span>
这个是网上看得的:
package project;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* A simple calculator program.
* <p>I saw this program in a QQ group, and help a friend correct it.</p>
*
* @author Singyuen Yip
* @version 1.00 12/29/2009
* @see JFrame
* @see ActionListener
*/
public class JCalculator extends JFrame implements ActionListener {
private static final long serialVersionUID = -169068472193786457L;
/**
* This class help close the Window.
* @author Singyuen Yip
*
*/
private class WindowCloser extends WindowAdapter {
public void windowClosing(WindowEvent we) {
System.exit(0);
}
}
int i;
// Strings for Digit & Operator buttons.
private final String[] str = { "7", "8", "9", "/", "4", "5", "6", "*", "1",
"2", "3", "-", ".", "0", "=", "+" };
// Build buttons.
JButton[] buttons = new JButton[str.length];
// For cancel or reset.
JButton reset = new JButton("CE");
// Build the text field to show the result.
JTextField display = new JTextField("0");
/**
* Constructor without parameters.
*/
public JCalculator() {
super("Calculator");
// Add a panel.
JPanel panel1 = new JPanel(new GridLayout(4, 4));
// panel1.setLayout(new GridLayout(4,4));
for (i = 0; i < str.length; i++) {
buttons[i] = new JButton(str[i]);
panel1.add(buttons[i]);
}
JPanel panel2 = new JPanel(new BorderLayout());
// panel2.setLayout(new BorderLayout());
panel2.add("Center", display);
panel2.add("East", reset);
// JPanel panel3 = new Panel();
getContentPane().setLayout(new BorderLayout());
getContentPane().add("North", panel2);
getContentPane().add("Center", panel1);
// Add action listener for each digit & operator button.
for (i = 0; i < str.length; i++)
buttons[i].addActionListener(this);
// Add listener for "reset" button.
reset.addActionListener(this);
// Add listener for "display" button.
display.addActionListener(this);
// The "close" button "X".
addWindowListener(new WindowCloser());
// Initialize the window size.
setSize(800, 800);
// Show the window.
// show(); Using show() while JDK version is below 1.5.
setVisible(true);
// Fit the certain size.
pack();
}
public void actionPerformed(ActionEvent e) {
Object target = e.getSource();
String label = e.getActionCommand();
if (target == reset)
handleReset();
else if ("0123456789.".indexOf(label) > 0)
handleNumber(label);
else
handleOperator(label);
}
// Is the first digit pressed?
boolean isFirstDigit = true;
/**
* Number handling.
* @param key the key of the button.
*/
public void handleNumber(String key) {
if (isFirstDigit)
display.setText(key);
else if ((key.equals(".")) && (display.getText().indexOf(".") < 0))
display.setText(display.getText() + ".");
else if (!key.equals("."))
display.setText(display.getText() + key);
isFirstDigit = false;
}
a022
/**
* Reset the calculator.
*/
public void handleReset() {
display.setText("0");
isFirstDigit = true;
operator = "=";
}
double number = 0.0;
String operator = "=";
/**
* Handling the operation.
* @param key pressed operator's key.
*/
public void handleOperator(String key) {
if (operator.equals("+"))
number += Double.valueOf(display.getText());
else if (operator.equals("-"))
number -= Double.valueOf(display.getText());
else if (operator.equals("*"))
number *= Double.valueOf(display.getText());
else if (operator.equals("/"))
number /= Double.valueOf(display.getText());
else if (operator.equals("="))
number = Double.valueOf(display.getText());
display.setText(String.valueOf(number));
operator = key;
isFirstDigit = true;
}
public static void main(String[] args) {
new JCalculator();
}
}
相关文章推荐
- 源码推荐(8.10):iOS 大文件断点下载库,仿微信发布语音
- 微信开发(2)OAuth2.0
- 如何抓微信的请求
- 微信开发(1)自定义菜单
- 微信更新---素材库
- 微信接口获取昵称中的表情符的过滤
- 微信公众号抢现金红包活动的核心代码分析(asp.net C#)
- 微信Curl获取信息简单案例(PHP版)
- 基于微信硬件公众平台的智能控制开发流程
- 微信分享 apicloud方式 中遇到的坎
- 沐雪微信2.0最新版本升级啦
- 仿支付宝/微信的密码输入框效果GridPasswordView解析
- 键盘操作、小程序查看器
- 添加按钮\小程序查看器.java
- 绘图.java(小程序查看器)
- java小程序查看器
- 小程序查看器的用法.java
- 微信开发中不出现“该公众号无法提供服务”
- 微信公众号平台之自定义菜单开发
- 用C实现打字机模式的一个小程序