黑马程序员_java集合2
2015-07-21 12:48
477 查看
Map:
该集合存储键值对,一对一对的往里存,而且要保证键的唯一性。
添加:
put(k key,v value)
putAll(Map<? extends k,? extends v> m)
删除:
clear();
remove<Object key>
判断:
containsValue(Object value)
containsKey(Object key)
isEmpty()
获取:
get(Object key)
size()
Values(),用collection接收
entrySet()
keySet()
Map集合的两种取出方式:
1.Set<Map.Entry<k,v>> entrySet:将Map集合中的映射关系存入到了Set集合中,而这个关系的数据类型就是:Map.Entry。
2.Set<k> keySet:将Map中所有的键存入到Set集合,因为Set集合具备迭代器,用迭代方法取出所有的键,再根据get方法,获取每一个键对应的值。
Map集合取出原理:将Map集合转换成Set集合,,再通过迭代 器取出。
示例:
package maptest;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
public class MapDemo {
public static void main(String[] args) {
Map<String,String> m1 = new HashMap<String,String>();//定义一个map集合
//给map集合添加元素
m1.put("01", "uzi");
m1.put("02", "cool");
m1.put("03", "pawn");
//m1.clear();//清除
m1.remove("01");//根据key移除
System.out.println(m1.containsKey("01"));//是否包含01这个键
System.out.println(m1.containsValue("pawn"));//是否包含pawn这个值
System.out.println(m1.size());//判断长度
Collection<String> cl = m1.values();//获取m1集合中的值
System.out.println(cl);
//keySet取出方式
Set<String> s1 = m1.keySet();
Iterator<String> it = s1.iterator();
while(it.hasNext())
{
String key = it.next();
String value = m1.get(key);
System.out.println(key+"="+value);
}
System.out.println("--------------------------------------");
//增强for循坏迭代
for(String s : s1)
{
String key = s;
String value = m1.get(s);
System.out.println(key+"="+value);
}
System.out.println("--------------------------------------");
//entrySet取出方式
Set<Map.Entry<String, String>> s2 = m1.entrySet();
Iterator<Map.Entry<String, String>> it2 = s2.iterator();
while(it2.hasNext())
{
Map.Entry<String, String> me =it2.next();
String key = me.getKey();
String value = me.getValue();
System.out.println(key+"==="+value);
}
System.out.println("--------------------------------------");
//增强for循坏迭代
for(Map.Entry<String,String> me : s2)
{
String key = me.getKey();
String value = me.getValue();
System.out.println(key+"="+value);
}
}
}
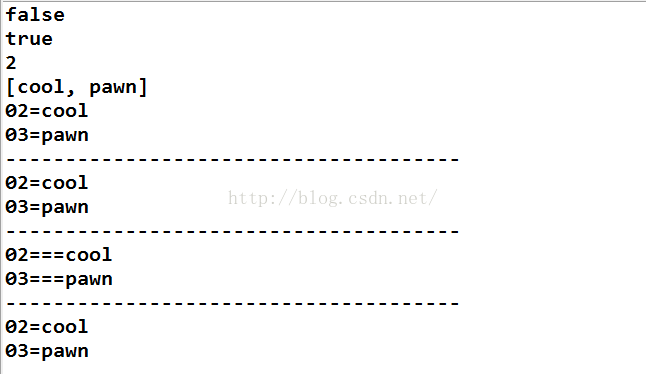
|--Hashtable:底层是哈希表数据结构,不可以存入null键null值,该集合线程是同步的。Jdk1.0效率低。
|--Properties:属性集,键和值都是字符串,
而且可以结合流进行键值的操作。
示例:
|--HashMap:底层是哈希表数据结构,可以存入null键null值,该集合线程不同步。Jdk1.2效率高。
示例:
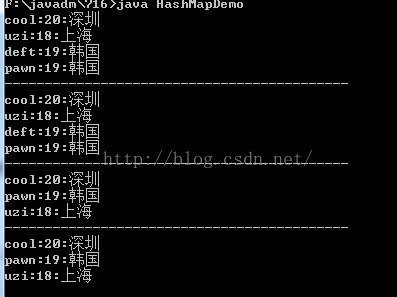
|--TreeMap:底层是二叉树数据结构,线程不同步,用于给Map集合中的键进行排序。
注意:和Set很像,Set底层就是使用了Map集合。
题目:获取字符串中,字母出现的次数,希望打印结果a(1)b(2)c(2)......
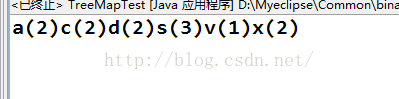
增强for的概述和使用
格式for( 数组或Collection集合中元素类型 变量名 :数组或Collection集合对象 )
{
使用变量名即可
}
工具类:
集合工具类:Collections
是集合框架中的用于操作集合的工具类
里面有很多静态方法,如二分法,排序,给集合加锁,得到集合中的最值
示例:
该集合存储键值对,一对一对的往里存,而且要保证键的唯一性。
添加:
put(k key,v value)
putAll(Map<? extends k,? extends v> m)
删除:
clear();
remove<Object key>
判断:
containsValue(Object value)
containsKey(Object key)
isEmpty()
获取:
get(Object key)
size()
Values(),用collection接收
entrySet()
keySet()
Map集合的两种取出方式:
1.Set<Map.Entry<k,v>> entrySet:将Map集合中的映射关系存入到了Set集合中,而这个关系的数据类型就是:Map.Entry。
2.Set<k> keySet:将Map中所有的键存入到Set集合,因为Set集合具备迭代器,用迭代方法取出所有的键,再根据get方法,获取每一个键对应的值。
Map集合取出原理:将Map集合转换成Set集合,,再通过迭代 器取出。
示例:
package maptest;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
public class MapDemo {
public static void main(String[] args) {
Map<String,String> m1 = new HashMap<String,String>();//定义一个map集合
//给map集合添加元素
m1.put("01", "uzi");
m1.put("02", "cool");
m1.put("03", "pawn");
//m1.clear();//清除
m1.remove("01");//根据key移除
System.out.println(m1.containsKey("01"));//是否包含01这个键
System.out.println(m1.containsValue("pawn"));//是否包含pawn这个值
System.out.println(m1.size());//判断长度
Collection<String> cl = m1.values();//获取m1集合中的值
System.out.println(cl);
//keySet取出方式
Set<String> s1 = m1.keySet();
Iterator<String> it = s1.iterator();
while(it.hasNext())
{
String key = it.next();
String value = m1.get(key);
System.out.println(key+"="+value);
}
System.out.println("--------------------------------------");
//增强for循坏迭代
for(String s : s1)
{
String key = s;
String value = m1.get(s);
System.out.println(key+"="+value);
}
System.out.println("--------------------------------------");
//entrySet取出方式
Set<Map.Entry<String, String>> s2 = m1.entrySet();
Iterator<Map.Entry<String, String>> it2 = s2.iterator();
while(it2.hasNext())
{
Map.Entry<String, String> me =it2.next();
String key = me.getKey();
String value = me.getValue();
System.out.println(key+"==="+value);
}
System.out.println("--------------------------------------");
//增强for循坏迭代
for(Map.Entry<String,String> me : s2)
{
String key = me.getKey();
String value = me.getValue();
System.out.println(key+"="+value);
}
}
}
|--Hashtable:底层是哈希表数据结构,不可以存入null键null值,该集合线程是同步的。Jdk1.0效率低。
|--Properties:属性集,键和值都是字符串,
而且可以结合流进行键值的操作。
示例:
import java.io.*; import java.util.*; class PropertiesDemo { public static void main(String[] args) throws IOException { setProp(); } public static void setProp()throws IOException { //要求:把文本上的信息用setProperties修改后保存起来。 FileInputStream fis = new FileInputStream("2.txt"); Properties p = new Properties(); p.load(fis);//从输出流中读取属性列表 FileOutputStream fos = new FileOutputStream("2.txt"); p.setProperty("wangwu","GG");//设置键值对 p.store(fos,"信息名");//把读取到的属性列表写入输出流 System.out.println(p); Set<String> st = p.stringPropertyNames();//返回列表中的键集, for(String s : st) { System.out.println(s+"::"+p.getProperty(s));//通过键得到值 } } }
|--HashMap:底层是哈希表数据结构,可以存入null键null值,该集合线程不同步。Jdk1.2效率高。
示例:
import java.util.*; class HashMapDemo { public static void main(String[] args) { //用hashmap存储数据 HashMap<Student,String> m1 = new HashMap<Student,String>(); //添加元素 m1.put(new Student("uzi",18), "上海"); m1.put(new Student("cool",20), "深圳"); m1.put(new Student("pawn",19), "韩国"); m1.put(new Student("deft",19), "韩国"); //keySet取出方式 Set<Student> s1 = m1.keySet(); Iterator<Student> it1 = s1.iterator(); while(it1.hasNext()) { Student s11 = it1.next(); String name = s11.getName(); int age = s11.getAge(); String ads =m1.get(s11); System.out.println(name+":"+age+":"+ads); } System.out.println("-------------------------------------------"); //Map.Entry取出方式 Set<Map.Entry<Student, String>> s2 =m1.entrySet(); Iterator<Map.Entry<Student, String>> it2 = s2.iterator(); while(it2.hasNext()) { Map.Entry<Student, String> me = it2.next(); Student s22 = me.getKey(); String adr2 = me.getValue(); String name = s22.getName(); int age = s22.getAge(); System.out.println(name+":"+age+":"+adr2); } System.out.println("-------------------------------------------"); //用Treemap存储数据 TreeMap<Student,String> t1 = new TreeMap<Student,String>(new MyComparator());//使用比较器 //添加元素 t1.put(new Student("uzi",18), "上海"); t1.put(new Student("cool",20), "深圳"); t1.put(new Student("pawn",19), "韩国"); t1.put(new Student("pawn",19), "韩国"); //keySet取出方式 Set<Student> ts1 = t1.keySet(); Iterator<Student> tit1 =ts1.iterator(); while(tit1.hasNext()) { Student sd1 = tit1.next(); String name = sd1.getName(); int age = sd1.getAge(); String address = t1.get(sd1); System.out.println(name+":"+age+":"+address); } System.out.println("-------------------------------------------"); //Map.Entry取出方式 Set<Map.Entry<Student, String>> sss = t1.entrySet(); Iterator<Map.Entry<Student, String>> itss = sss.iterator(); while(itss.hasNext()) { Map.Entry<Student, String> me = itss.next(); Student s = me.getKey(); String address = me.getValue(); String name = s.getName(); int age = s.getAge(); System.out.println(name+":"+age+":"+address); } } } //实现Comparator接口,定义一个比较器 class MyComparator implements Comparator<Student> { public int compare(Student s1 ,Student s2) { int num = s1.getName().compareTo(s2.getName());//先比较名字 if(num==0) return new Integer(s1.getAge()).compareTo(new Integer(s2.getAge()));//名字相同则比较年龄 return num; } } //定义一个学生类 class Student implements Comparable<Student>//实现Comparable接口 { private String name; private int age; public Student(String name,int age) { this.name=name; this.age=age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String toString() { return name+":"+age; } //复写hashCode方法 public int hashCode() { return this.name.hashCode()+this.age*33; } //复写equals方法 public boolean equals(Object obj) { if(!(obj instanceof Student)) throw new RuntimeException("类型不正确"); Student s = (Student)obj; return this.name.equals(s.name)&&this.age==s.age; } //复写compareTo方法 public in 4000 t compareTo(Student s) { int num = new Integer(this.age).compareTo(new Integer(s.age)); if(num==0) return this.name.compareTo(s.name); return num; } }
|--TreeMap:底层是二叉树数据结构,线程不同步,用于给Map集合中的键进行排序。
注意:和Set很像,Set底层就是使用了Map集合。
题目:获取字符串中,字母出现的次数,希望打印结果a(1)b(2)c(2)......
package treemap; import java.util.Iterator; import java.util.Map; import java.util.TreeMap; public class TreeMapTest { public static void main(String[] args) { //获取字符串中,字母出现的次数,希望打印结果a(1)b(2)c(2)...... TreeMap<Character,Integer> tm = new TreeMap<Character,Integer>();//定义一个TreeMap集合 char[] ch = "avcxsdsadcxs".toCharArray();//把字符串转成字符数组 int count = 0;//定义标记 for(int i=0;i<ch.length;i++)//对字符数组进行遍历 { Integer value = tm.get(ch[i]);//通过键得到值 if(value!=null)//如果原集合中已经存在,则赋值 count=value; count++;//自增 tm.put(ch[i], count);//添加键值对进去 count=0;//清空计数器 } StringBuilder sb = new StringBuilder();//定义一个容器 //用迭代器遍历集合 Iterator<Map.Entry<Character,Integer>> it = tm.entrySet().iterator(); while(it.hasNext()) { Map.Entry<Character, Integer> me = it.next(); Integer value = me.getValue(); Character key = me.getKey(); sb.append(key+"("+value+")");//把集合中的键值对按照格式存入容器中 } System.out.println(sb.toString());//返回字符串并且打印 } }
增强for的概述和使用
格式for( 数组或Collection集合中元素类型 变量名 :数组或Collection集合对象 )
{
使用变量名即可
}
工具类:
集合工具类:Collections
是集合框架中的用于操作集合的工具类
里面有很多静态方法,如二分法,排序,给集合加锁,得到集合中的最值
示例:
import java.util.ArrayList; import java.util.List; import static java.util.Collections.*; public class Conllections { public static void main(String[] args) { //定义一个List集合 List<Integer> l = new ArrayList<Integer>(); l.add(5); l.add(4); l.add(1); l.add(2); l.add(3); sort(l);//给数组排序 reverse(l);//把排序的顺序倒过来 for(Integer it : l) { System.out.println(it); } } }
相关文章推荐
- 黑马程序员——java基础——多线程的学习总结
- 黑马程序员_java集合1
- Android面试题Activity部分
- 黑马程序员_javaAPI
- java基础面试题
- 如何提高程序员的逼格
- 黑马程序员--iOS--C基础(一)
- 剑指offer-面试题5.从尾到头打印链表
- 黑马程序员---面向对象中的内部类
- 黑马程序员—JAVA基础—String类 javaAPI学习
- 面试时可以向面试官提那些问题
- 推荐!国外程序员整理的 PHP 资源大全
- 剑指offer-面试题4.替换空格
- 告别迷茫与忧伤的程序员
- 面试-阻塞队列及线程池
- 面试-锁相关
- 面试-并发概述
- 面试-JVM
- 黑马程序员—— 6,多态
- 面试-MySQL