lambda function
2015-06-24 11:33
417 查看
Some basic knowledge of lambda function is
here. A lambda is an ad-hoc, locally-scoped function. Basically, lambdas are syntactic sugar, designed to reduce a lot of the work required in creating ad-hoc functor classes. There are three parts of lambda function
[capture list] (parameter list) {function body}
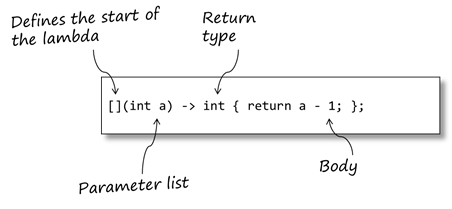
The brackets ([]) mark the declaration of the lambda; it can have parameters, and it should be followed by its body (the same as any other function). One difference between lambdas and functions: lambda parameters
can’t have defaults. The body of the lambda is just a normal function body, and can be arbitrarily complex (although, it’s generally good practice to keep lambda bodies relatively simple).
A lambda is an object and has a type and can be stored. However, the type of the lambda is only known by the compiler (since it is compiler-generated), so you must use auto for declaration instances of the lambda.
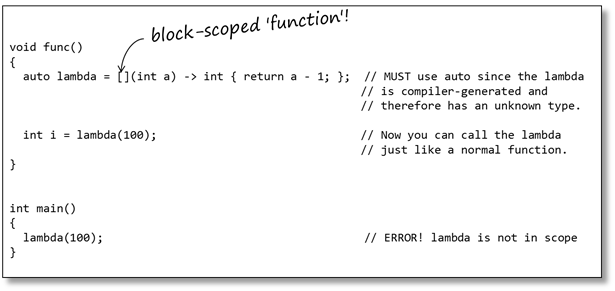
Sometimes it’s useful and convenient to be able to access objects from the lambda’s containing scope – that is, the scope within which the lambda was defined. If you were writing your own functor you could do this by passing in the appropriate parameters
to the constructor of the functor. C++ provides a convenient mechanism for achieving this with lambdas called ‘capturing the context’. The context of a lambda is the set of objects that are in scope when the lambda is called. The context objects may becaptured
then used as part of the lambda’s processing. Capturing an object by name makes a lambda-local copy of the object,
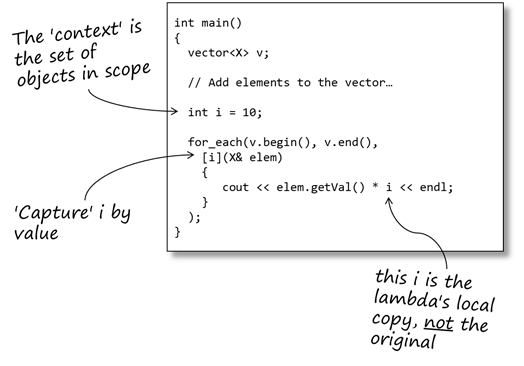
Capturing an object by reference allows the lambda to manipulate its context. That is, the lambda can change the values of the objects it has captured by reference.
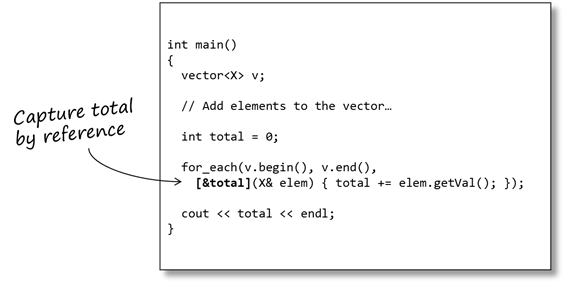
All variables in scope can be captured using a default-capture. This makes available all automatic variables currently in scope.
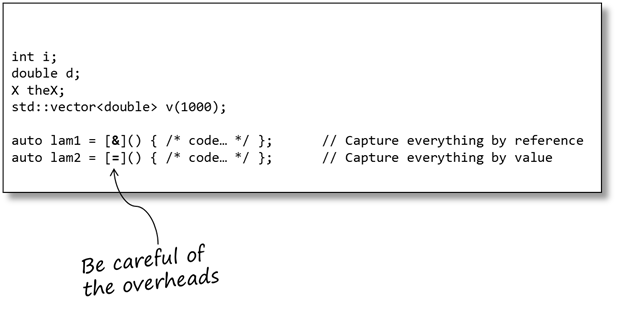
We could also add a capture list to a lambda
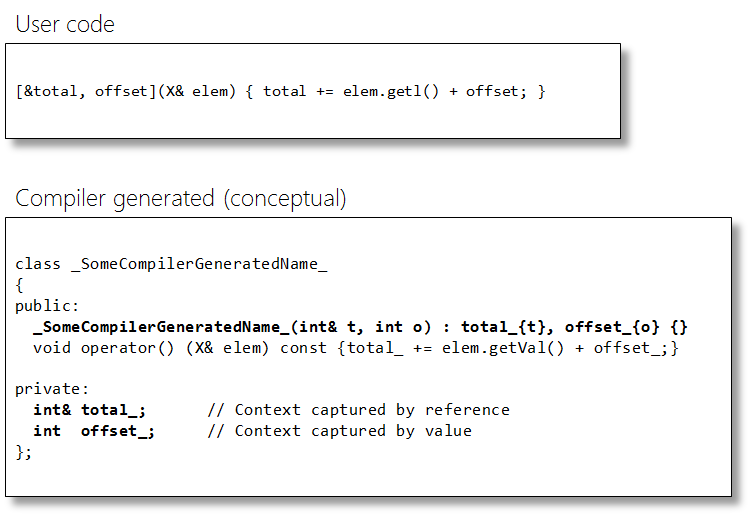
It is perfectly possible (and quite likely) that we may want to use lambdas inside class member functions. Remember, a lambda is a unique (and separate) class of its own so when it executes it has its own context. Therefore, it does not have direct access
to any of the class’ member variables. To capture the class’ member variables we must capture the this pointer of the class. We now have full access to all the class’ data (including private data, as we are inside a member function).
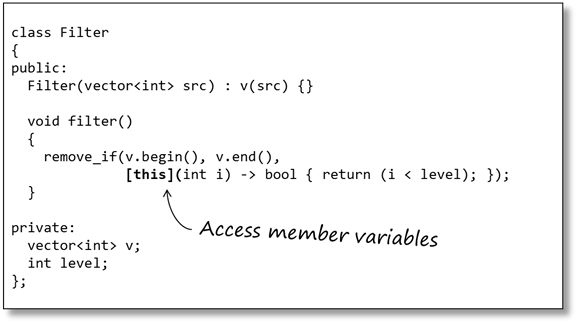
One concrete example is given by
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main(){
// Create a vector object that contains 10 elements.
vector<int> v;
for (int i = 1; i < 10; ++i) {
v.push_back(i);
}
// Count the number of even numbers in the vector by
// using the for_each function and a function object.
int evenCount = 0;
for_each(v.begin(), v.end(), [&evenCount](const int& item){
if (item%2==0) evenCount++;
});
// Print the count of even numbers to the console.
cout << "There are " << evenCount << " even numbers in the vector." << endl;
}
Lambda functions are usually used along with STL algorithms,
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main(){
// Create a vector object that contains 10 elements.
vector<int> v;
for (int i = 1; i < 10; ++i) {
v.push_back(i);
}
// Find all even numbers
vector<int> evennumber;
auto pos = find_if(v.begin(), v.end(), [](const int& item){return item%2==0;});
while(pos!=v.end()){
evennumber.push_back(*pos);
pos++;
pos = find_if(pos, v.end(), [](const int& item){return item%2==0;});
}
// Print the count of even numbers to the console.
cout << "There are " << evennumber.size() << " even numbers in the vector." << endl;
for(pos=evennumber.begin();pos!=evennumber.end();++pos){
cout << *pos << " ";
}
cout << endl;
}
here. A lambda is an ad-hoc, locally-scoped function. Basically, lambdas are syntactic sugar, designed to reduce a lot of the work required in creating ad-hoc functor classes. There are three parts of lambda function
[capture list] (parameter list) {function body}
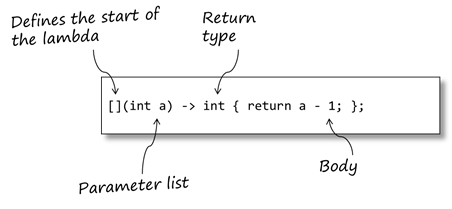
The brackets ([]) mark the declaration of the lambda; it can have parameters, and it should be followed by its body (the same as any other function). One difference between lambdas and functions: lambda parameters
can’t have defaults. The body of the lambda is just a normal function body, and can be arbitrarily complex (although, it’s generally good practice to keep lambda bodies relatively simple).
A lambda is an object and has a type and can be stored. However, the type of the lambda is only known by the compiler (since it is compiler-generated), so you must use auto for declaration instances of the lambda.
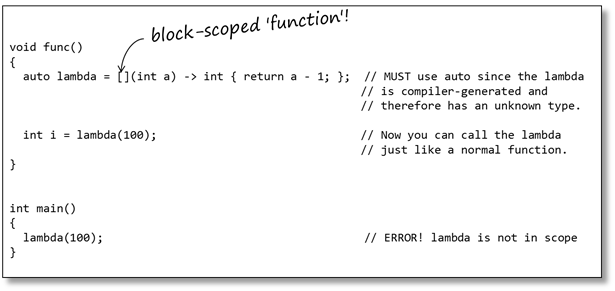
Sometimes it’s useful and convenient to be able to access objects from the lambda’s containing scope – that is, the scope within which the lambda was defined. If you were writing your own functor you could do this by passing in the appropriate parameters
to the constructor of the functor. C++ provides a convenient mechanism for achieving this with lambdas called ‘capturing the context’. The context of a lambda is the set of objects that are in scope when the lambda is called. The context objects may becaptured
then used as part of the lambda’s processing. Capturing an object by name makes a lambda-local copy of the object,
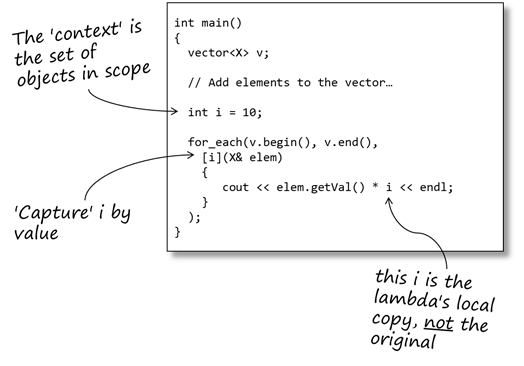
Capturing an object by reference allows the lambda to manipulate its context. That is, the lambda can change the values of the objects it has captured by reference.
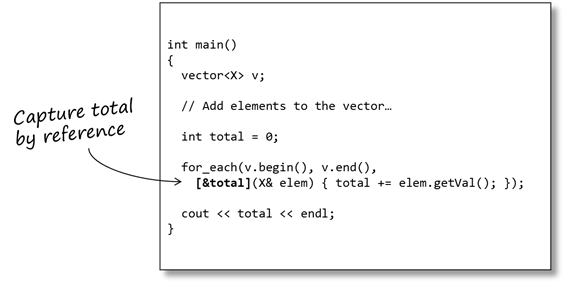
All variables in scope can be captured using a default-capture. This makes available all automatic variables currently in scope.
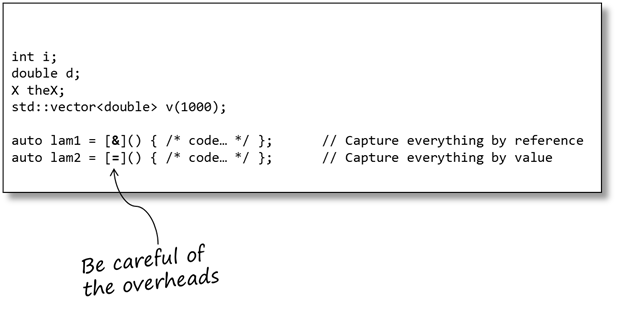
We could also add a capture list to a lambda
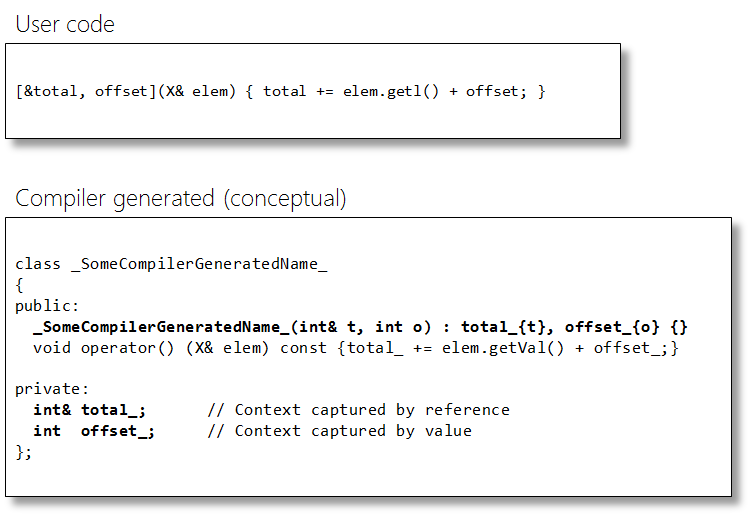
It is perfectly possible (and quite likely) that we may want to use lambdas inside class member functions. Remember, a lambda is a unique (and separate) class of its own so when it executes it has its own context. Therefore, it does not have direct access
to any of the class’ member variables. To capture the class’ member variables we must capture the this pointer of the class. We now have full access to all the class’ data (including private data, as we are inside a member function).
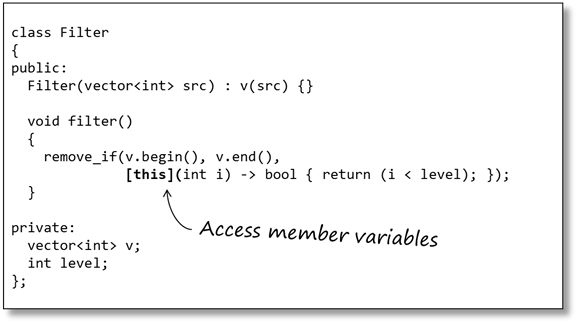
One concrete example is given by
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main(){
// Create a vector object that contains 10 elements.
vector<int> v;
for (int i = 1; i < 10; ++i) {
v.push_back(i);
}
// Count the number of even numbers in the vector by
// using the for_each function and a function object.
int evenCount = 0;
for_each(v.begin(), v.end(), [&evenCount](const int& item){
if (item%2==0) evenCount++;
});
// Print the count of even numbers to the console.
cout << "There are " << evenCount << " even numbers in the vector." << endl;
}
Lambda functions are usually used along with STL algorithms,
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main(){
// Create a vector object that contains 10 elements.
vector<int> v;
for (int i = 1; i < 10; ++i) {
v.push_back(i);
}
// Find all even numbers
vector<int> evennumber;
auto pos = find_if(v.begin(), v.end(), [](const int& item){return item%2==0;});
while(pos!=v.end()){
evennumber.push_back(*pos);
pos++;
pos = find_if(pos, v.end(), [](const int& item){return item%2==0;});
}
// Print the count of even numbers to the console.
cout << "There are " << evennumber.size() << " even numbers in the vector." << endl;
for(pos=evennumber.begin();pos!=evennumber.end();++pos){
cout << *pos << " ";
}
cout << endl;
}
相关文章推荐
- 浅析STL中的常用算法
- c++ STL容器总结之:vertor与list的应用
- C++在成员函数中使用STL的find_if函数实例
- 关于STL中list容器的一些总结
- 关于STL中的map容器的一些总结
- 浅析stl序列容器(map和set)的仿函数排序
- STL list链表的用法详细解析
- stl容器set,map,vector之erase用法与返回值详细解析
- STl中的排序算法详细解析
- 关于STL中vector容器的一些总结
- 关于STL中set容器的一些总结
- 简单说说STL的内存管理
- CppUtest发现的STL容器内存泄漏问题
- STL中算法
- STL简单应用
- vector-list-deque
- 三十分钟掌握STL
- 1.sort()
- 刚刚接触到一个STL的函数make_pair()
- 简单使用C++的STL