57. C# -- 字符串(string)
2015-06-02 11:55
459 查看
理论:
C# 字符串(String)
在 C# 中,您可以使用字符数组来表示字符串,但是,更常见的做法是使用 string 关键字来声明一个字符串变量。
string 关键字是 System.String 类的别名。
创建 String 对象 您可以使用以下方法之一来创建 string 对象:
通过给 String 变量指定一个字符串
通过使用 String 类构造函数
通过使用字符串串联运算符( + )
通过检索属性或调用一个返回字符..
1. Chars 在当前 String 对象中获取 Char 对象的指定位置。
2. Length 在当前的 String 对象中获取字符数。
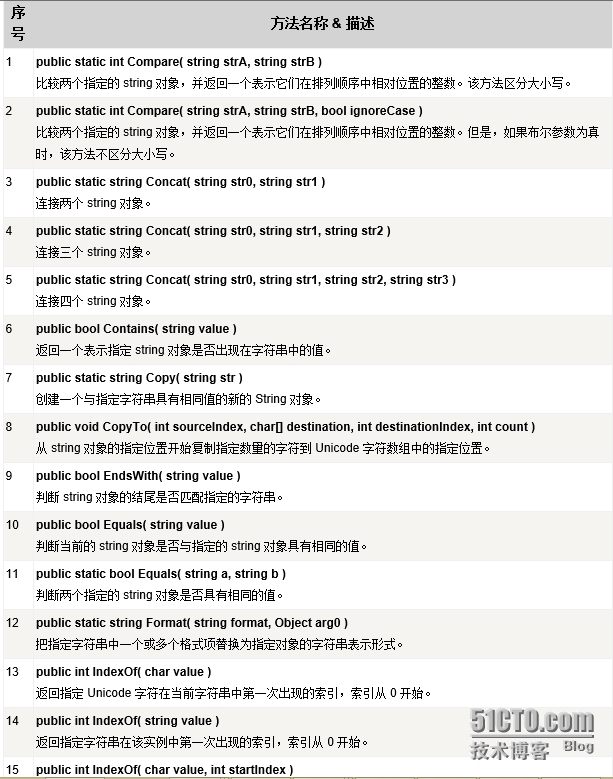
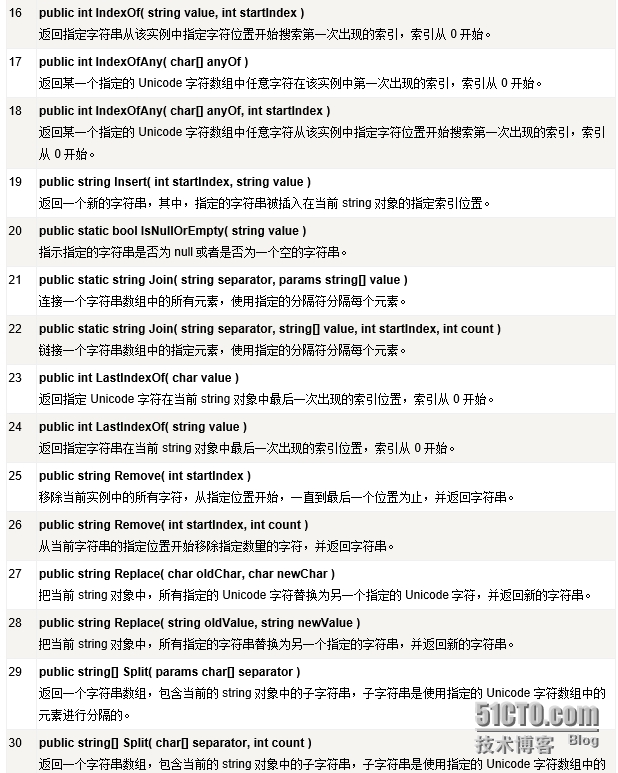
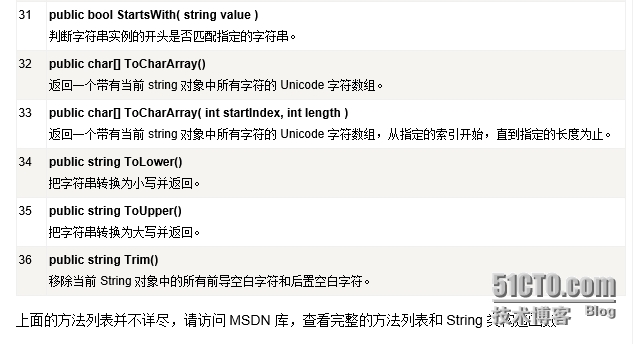
实例1:
比较字符串
字符串包含字符串:
当上面的代码被编译和执行时,它会产生下列结果:
The sequence 'test' was found.
获取子字符串:
参考:
http://outofmemory.cn/csharp/tutorial/csharp-string.html
C# 字符串(String)
在 C# 中,您可以使用字符数组来表示字符串,但是,更常见的做法是使用 string 关键字来声明一个字符串变量。
string 关键字是 System.String 类的别名。
创建 String 对象 您可以使用以下方法之一来创建 string 对象:
通过给 String 变量指定一个字符串
通过使用 String 类构造函数
通过使用字符串串联运算符( + )
通过检索属性或调用一个返回字符..
String 类的属性
String 类有以下两个属性:1. Chars 在当前 String 对象中获取 Char 对象的指定位置。
2. Length 在当前的 String 对象中获取字符数。
String 类的方法
String 类有许多方法用于 string 对象的操作。下面的表格提供了一些最常用的方法: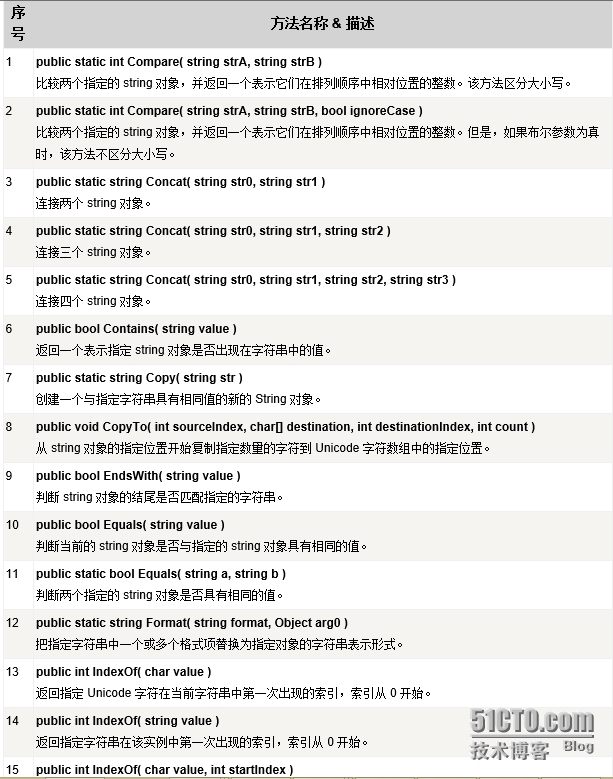
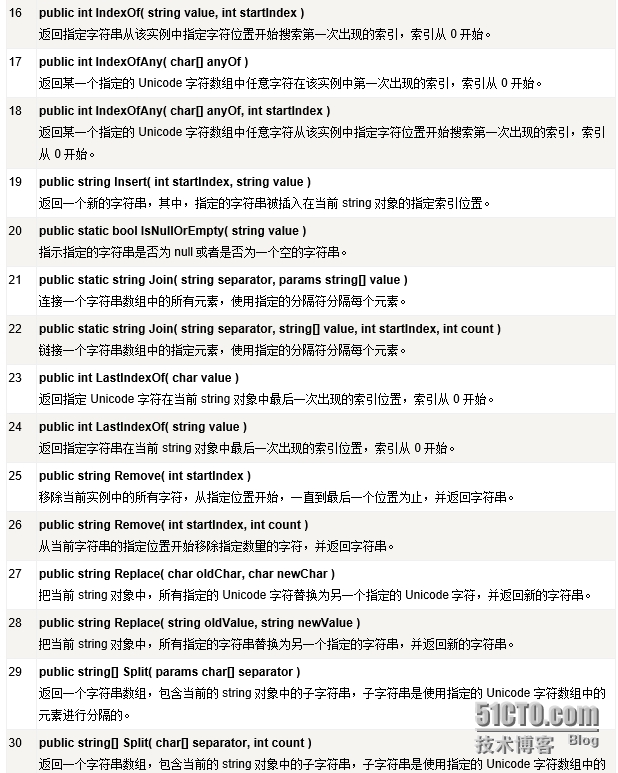
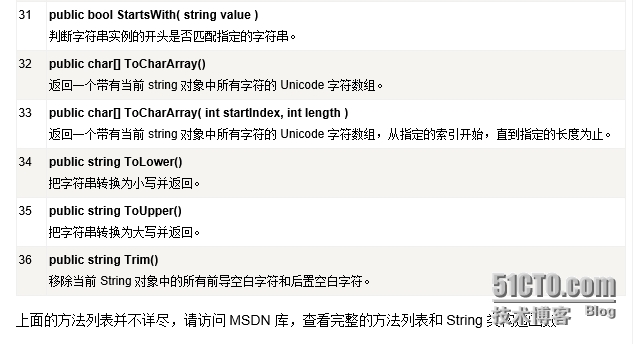
实例1:
using System; namespace StringApplication { class Program { static void Main(string[] args) { //字符串,字符串连接 string fname, lname; fname = "Rowan"; lname = "Atkinson"; string fullname = fname + lname; Console.WriteLine("Full Name: {0}", fullname); //通过使用 string 构造函数 char[] letters = { 'H', 'e', 'l', 'l','o' }; string greetings = new string(letters); Console.WriteLine("Greetings: {0}", greetings); //方法返回字符串 string[] sarray = { "Hello", "From", "Tutorials", "Point" }; string message = String.Join(" ", sarray); Console.WriteLine("Message: {0}", message); //用于转化值的格式化方法 DateTime waiting = new DateTime(2012, 10, 10, 17, 58, 1); string chat = String.Format("Message sent at {0:t} on {0:D}", waiting); Console.WriteLine("Message: {0}", chat); Console.ReadKey() ; } } } //当上面的代码被编译和执行时,它会产生下列结果: //Full Name: Rowan Atkinson //Greetings: Hello //Message: Hello From Tutorials Point //Message: Message sent at 5:58 PM on Wednesday, October 10, 2012
实例2:
下面的实例演示了上面提到的一些方法:比较字符串
using System; namespace StringApplication { class StringProg { static void Main(string[] args) { string str1 = "This is test"; string str2 = "This is text"; if (String.Compare(str1, str2) == 0) { Console.WriteLine(str1 + " and " + str2 + " are equal."); } else { Console.WriteLine(str1 + " and " + str2 + " are not equal."); } Console.ReadKey() ; } } } 当上面的代码被编译和执行时,它会产生下列结果: This is test and This is text are not equal.
字符串包含字符串:
using System; namespace StringApplication { class StringProg { static void Main(string[] args) { string str = "This is test"; if (str.Contains("test")) { Console.WriteLine("The sequence 'test' was found."); } Console.ReadKey() ; } } }
当上面的代码被编译和执行时,它会产生下列结果:
The sequence 'test' was found.
获取子字符串:
using System; namespace StringApplication { class StringProg { static void Main(string[] args) { string str = "Last night I dreamt of San Pedro"; Console.WriteLine(str); string substr = str.Substring(23); Console.WriteLine(substr); } Console.ReadKey() ; } } //当上面的代码被编译和执行时,它会产生下列结果: San Pedro连接字符串:
using System; namespace StringApplication { class StringProg { static void Main(string[] args) { string[] starray = new string[]{"Down the way nights are dark", "And the sun shines daily on the mountain top", "I took a trip on a sailing ship", "And when I reached Jamaica", "I made a stop"}; string str = String.Join("\n", starray); Console.WriteLine(str); } Console.ReadKey() ; } }当上面的代码被编译和执行时,它会产生下列结果:
Down the way nights are dark And the sun shines daily on the mountain top I took a trip on a sailing ship And when I reached Jamaica I made a stop
参考:
http://outofmemory.cn/csharp/tutorial/csharp-string.html
相关文章推荐
- c#调用COM组件
- C#中抽象方法与虚拟方法的区别
- c#中虚函数的相关使用方法
- C#实现多线程的同步方法实例分析
- C#中尾递归的使用、优化及编译器优化
- C#通用邮件发送类分享
- C#中Equality和Identity浅析
- C#生成饼形图及添加文字说明实例代码
- C#判等对象是否相等的方法汇总
- C#简单的向量用法实例教程
- C#托管堆对象实例包含内容分析
- C#实现按照指定长度在数字前补0方法小结
- C#虚方法的声明和使用实例教程
- C#获取文件夹及文件的大小与占用空间的方法
- C#定义简单的反射工厂实例分析
- C#数字图象处理之肤色检测的方法
- C#实现用于操作wav声音文件的类实例
- C#选择排序法实例分析
- C#线程间不能调用剪切板的解决方法
- C#实现WinForm捕获最小化事件的方法