java面向对象—抽象类与接口
2015-05-02 00:00
483 查看
摘要: java final关键字的使用 java抽象类 Java接口的实现
1、final关键字在java中被称为完结器,表示最终的意思
2、final能声明类,方法,属性:
使用final声明的类不能被继承
使用final声明的方法不能被重写
使用final声明的变量变成常量,常量是不可以被修改的(常量名全部大写)
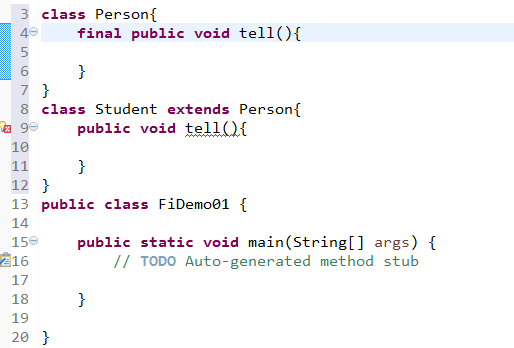

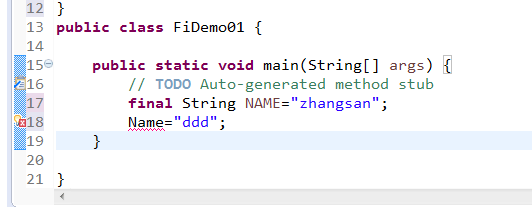
抽象类
1、抽象类概念:
包含一个抽象方法的类就是抽象类
2、抽象方法:
声明而未被实现的方法,抽象方法必须使用abstract关键字声明
3、抽象类被子类继承,子类(如果不是抽象类)必须重写抽象类中的所有抽象方法
4、定义格式:
abstract class className{
属性
方法
抽象方法
}
5、抽象类不能直接实例化,要通过其子类进行实例化
接口
1、接口可以理解为一种特殊的类,里面全部是由全局常量(常量必须大写)和公共的抽象方法所组成
2、接口的格式:
interface interfaceName{
全局常量
抽象方法
}
3、接口的实现也必须通过子类,使用关键字implements,而且接口是可以多实现的
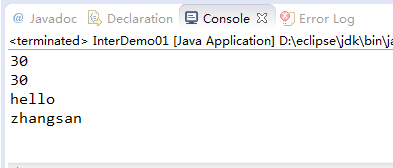
4、一个子类可以同时继承抽象类和实现接口
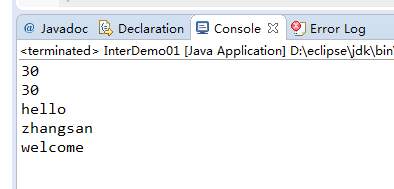
5、一个接口不能继承一个抽象类,但是却可以通过extends关键字同时继承多个接口,实现接口的多继承
1、final关键字在java中被称为完结器,表示最终的意思
2、final能声明类,方法,属性:
使用final声明的类不能被继承
使用final声明的方法不能被重写
使用final声明的变量变成常量,常量是不可以被修改的(常量名全部大写)
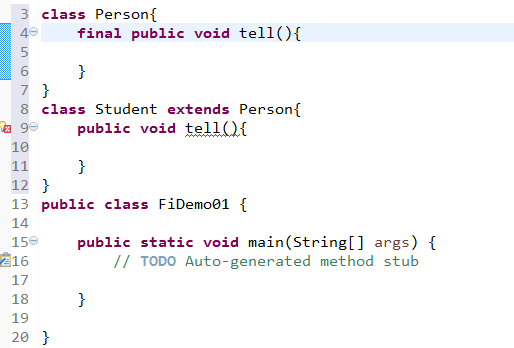

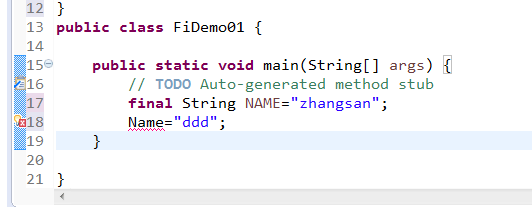
抽象类
1、抽象类概念:
包含一个抽象方法的类就是抽象类
2、抽象方法:
声明而未被实现的方法,抽象方法必须使用abstract关键字声明
3、抽象类被子类继承,子类(如果不是抽象类)必须重写抽象类中的所有抽象方法
4、定义格式:
abstract class className{
属性
方法
抽象方法
}
5、抽象类不能直接实例化,要通过其子类进行实例化
package com.jk.classdemo; abstract class Student{ private String name; private int age; public void tell(){ } public abstract void say(); /** * @return the name */ public String getName() { return name; } /** * @param name the name to set */ public void setName(String name) { this.name = name; } /** * @return the age */ public int getAge() { return age; } /** * @param age the age to set */ public void setAge(int age) { this.age = age; } } class AbsDemo extends Student{ public void say(){ System.out.println("name:"+getName()+" "+"age:"+getAge()); } } public class AbstDemo01 { public static void main(String[] args) { // TODO Auto-generated method stub AbsDemo a=new AbsDemo(); a.setName("ddd"); a.setAge(20); a.say(); } }
接口
1、接口可以理解为一种特殊的类,里面全部是由全局常量(常量必须大写)和公共的抽象方法所组成
2、接口的格式:
interface interfaceName{
全局常量
抽象方法
}
3、接口的实现也必须通过子类,使用关键字implements,而且接口是可以多实现的
package com.jk.classdemo; interface inter1{ public static final int AGE=30; abstract public void say(); } interface inter2{ public static final String NAME="zhangsan"; abstract public void tell(); } class Imp implements inter1,inter2{ public void say(){ System.out.println(AGE); } public void tell(){ System.out.println("hello"); } } public class InterDemo01 { public static void main(String[] args) { // TODO Auto-generated method stub Imp im=new Imp(); im.say(); System.out.println(inter1.AGE); im.tell(); System.out.println(inter2.NAME); } }
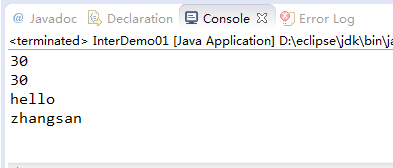
4、一个子类可以同时继承抽象类和实现接口
package com.jk.classdemo; interface inter1{ public static final int AGE=30; abstract public void say(); } interface inter2{ public static final String NAME="zhangsan"; abstract public void tell(); } abstract class Abs1{ public abstract void print(); } class Imp extends Abs1 implements inter1,inter2{ public void say(){ System.out.println(AGE); } public void tell(){ System.out.println("hello"); } public void print(){ System.out.println("welcome"); } } public class InterDemo01 { public static void main(String[] args) { // TODO Auto-generated method stub Imp im=new Imp(); im.say(); System.out.println(inter1.AGE); im.tell(); System.out.println(inter2.NAME); im.print(); } }
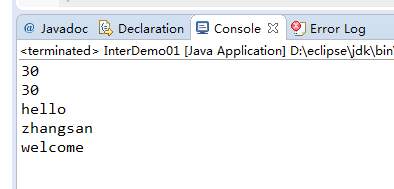
5、一个接口不能继承一个抽象类,但是却可以通过extends关键字同时继承多个接口,实现接口的多继承
interface inter1{ public static final int AGE=30; abstract public void say(); } interface inter2{ public static final String NAME="zhangsan"; abstract public void tell(); } interface inter3 extends inter1,inter2{ } abstract class Abs1{ public abstract void print(); }
相关文章推荐
- java面向对象—抽象类、接口与多继承
- Java学习笔记(六):面向对象、接口和抽象类
- Java重修之路(九)面向对象之final关键字,抽象类(abstract),接口(interface)
- Java基础之面向对象(抽象类、接口、内部类)
- java面向对象(三)之抽象类,接口,向上转型
- Java基础(8):面向对象—抽象类和接口的区别和各自特点
- java面向对象—抽象类、接口与多继承
- Java基础--面向对象(继承、抽象类、接口)
- 黑马程序员--JAVA<面向对象>--构造函数、抽象类、接口、多态
- java面向对象(三)之抽象类,接口
- 黑马程序员——Java基础---面向对象(继承、多态、抽象类、接口、内部类)
- Java基础——面向对象——继承、抽象类、接口、多态、包、内部类、异常等
- java面向对象,接口和抽象类.
- 黑马程序员_Java基础_面向对象(继承、子父类变量和函数以及构造函数特点、final关键字、抽象类、模版方法模式、接口)
- 【Java基础】——java面向对象(中)—继承、抽象类、接口
- JAVA(十二)java面向对象6-抽象类和接口
- 黑马程序员——Java基础---面向对象(封装、继承、单例、抽象类、接口、内部类)
- JAVA基础(八)面向对象3 多态、抽象类、接口
- Java面向对象——抽象类与接口
- JAVA总结(面向对象--抽象类、接口)