List vs IEnumerable vs IQueryable vs ICollection vs IDictionary
2014-10-25 15:36
471 查看
原文:http://www.codeproject.com/Articles/832189/List-vs-IEnumerable-vs-IQueryable-vs-ICollection-v
IList vs IEnumerable vs IQueryable vs ICollection vs IDictionary
IList vs IEnumerable vs IQueryable vs ICollection vs IDictionary - 494.3 KB
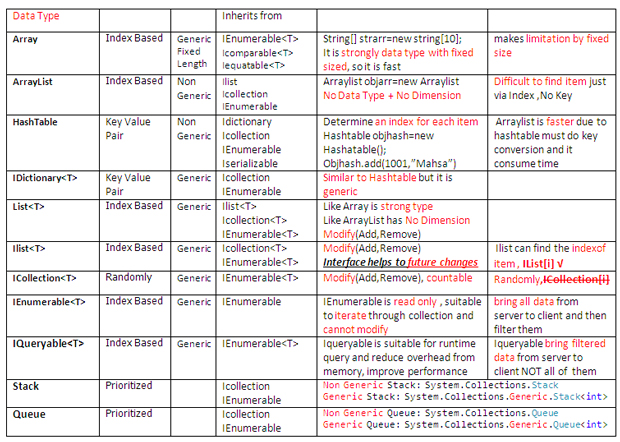
Set or group of records which makes one meaningful unit: In fetch and modification progress we need appropriate container to store them temporary in it. Appropriate container depends on: 1. Our action which we want to do on data ( just read, do modification
such as insert, delete, etc ) 2. Number of records which should be transfer

1. Fixed Length -> difficult to change the nember of count 2. Type Safe -> you determin its type at coding process (it has better performance due to do not consume time at run time to spesific type countainer ) 3. Use “for” keyword to fill and iterate through
array Array is faster than Arraylist because it is fixed sized and srong type, so it needs less memory due to its data type and dimenssion is determined
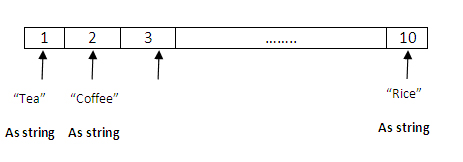

Collapse | Copy
Code
1. It does not have spesific length -> It is possible to grow data , it is good feature because of at the first we are not likely to sure about length of collection 2. Arraylist does not have any spesific type and it will be determined at run time, its adbantage
is when you are not sure about type of data untill in run time user detemines it, and its disadvantage is to reduce performance by consuming time , so use it just when you need it it is like var or object 3. Use “foreach” keyword for iterate through it


Collapse | Copy
Code
ArrayList can accept string , integer and decimal at the same time

Collapse | Copy
Code


Collapse | Copy
Code


Collapse | Copy
Code

Collapse | Copy
Code


Collapse | Copy
Code

Collapse | Copy
Code
Why we needList? 1. List does not have spesific length à It is possible to have data without knowing its size , it is good feature because of at the first we are not likely to sure about length of collection. 2. List has Spesific type so it is strong type,
therfore it has better perfomance and high speed in comparison with ArrayList and reduce unwanted error in compile time. 3. Use “foreach” keyword for iterate through it.


Collapse | Copy
Code
Why we need IList? Ilist is an interface of list (does everything that List does) and to observe polymorphism in order to decouple and control on add or remove method that implement on the client side, everything else are similar to List. Whenever we want to
change on some operation “IList” allow us to do that easily with at least changing in the whole of codes. Ilist can not be instantiate from Ilist , so it should be instantiate from List

Collapse | Copy
Code
1.Abstract inherit from just one class but Interface implements from one or more interfaces 2.Abstract can have non concrete method (expand method) but you have to define just signature inside interface 3.Inside Abstract class you can define variable and value
but you are not allowed to define variable inside interface 4.An abstract class can have constructor but inside interface you are not allowed to define constructor 5.You can write scope for member of interface, red ones donot allow but blue one allow Ilist
is an interface and as I mentioned that how a class cannot be driven from two class it just can be driven from only one class, so whenever you want to drive from two class it is not possible to inherit from two abstract class but it is possible to drive from
one class and another one Ilist as interface Later in future you decide add some features on your class and inherit it from another class So because of this reason we always prefer to use interface of collection so that if you want to change your code and
enhance its abilities it is possible to grow it On the otherhand interface keeps your program extensible and Decouple: The classes are independent from each other error, exeption and failur will happen rarly in future changing code Polymorphism: When you are
using interface you absolutly observe and do polymorphism and oop it means encapsulate your designer Interface means a point or a node to join two part to eachother it means making low dependency from two part and make a joint section to make felxible chang
in future

Collapse | Copy
Code
IList is similar to List but with this difference that we prefer to use INTERFACE instead of abstract in programming because future changing is easy
IEnumerable is suitable just for iterate through collection and you can not modify (Add or Remove) it IEnumerable bring ALL data from server to client then filter them, assume that you have a lot of records so IEnumerable puts overhead on your memory


Collapse | Copy
Code

Follow "Entity Framework DatabaseFirst" from this article for making DBContext

Collapse | Copy
Code
Data Access

Collapse | Copy
Code

Collapse | Copy
Code

Collapse | Copy
Code
MVC Tutorial: http://technical.cosmicverse.info/Article/Index/3
Data Access Layer Tutorial: http://technical.cosmicverse.info/Article/Index/4
http://www.codeproject.com/Articles/732425/IEnumerable-Vs-IQueryable
http://www.dotnet-tricks.com/Tutorial/linq/I8SY160612-IEnumerable-VS-IQueryable.html
http://www.claudiobernasconi.ch/2013/07/22/when-to-use-ienumerable-icollection-ilist-and-list
IList vs IEnumerable vs IQueryable vs ICollection vs IDictionary
IList vs IEnumerable vs IQueryable vs ICollection vs IDictionary - 494.3 KB
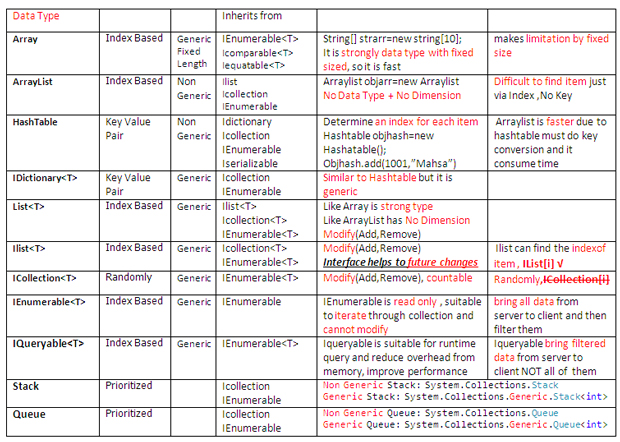
Collection
Set or group of records which makes one meaningful unit: In fetch and modification progress we need appropriate container to store them temporary in it. Appropriate container depends on: 1. Our action which we want to do on data ( just read, do modificationsuch as insert, delete, etc ) 2. Number of records which should be transfer

Array
1. Fixed Length -> difficult to change the nember of count 2. Type Safe -> you determin its type at coding process (it has better performance due to do not consume time at run time to spesific type countainer ) 3. Use “for” keyword to fill and iterate througharray Array is faster than Arraylist because it is fixed sized and srong type, so it needs less memory due to its data type and dimenssion is determined
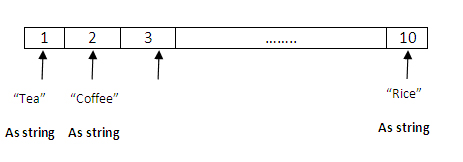

Collapse | Copy
Code
//It is obvious that strArray is //1. string --> Strongly Type //2. Sized=10 --> Fixed Size string[] strArray = new string[10]; for (int i = 0; i < 10; i++) { if (strArray[i]==null) { strArray[i] = (i+1).ToString(); } } this.ListBoxArray.DataSource = null; this.ListBoxArray.Items.Clear(); this.ListBoxArray.DataSource = strArray; this.ListBoxArray.DataBind();
ArrayList
1. It does not have spesific length -> It is possible to grow data , it is good feature because of at the first we are not likely to sure about length of collection 2. Arraylist does not have any spesific type and it will be determined at run time, its adbantageis when you are not sure about type of data untill in run time user detemines it, and its disadvantage is to reduce performance by consuming time , so use it just when you need it it is like var or object 3. Use “foreach” keyword for iterate through it


Collapse | Copy
Code
public class Product { public Product() { } public Product(string Code, string Name) { _Code = Code; _Name = Name; } public string _Code {get; set;} public string _Name { get; set; } }
ArrayList can accept string , integer and decimal at the same time

Collapse | Copy
Code
//It is NOT obvious that strArrayList is 1. string? int? object? decimal? --> NOT Strongly Type // 2. Sized=10? 20? 100? -->NOT Fixed Size // Namespace: System.Collections System.Collections.ArrayList strArrayList = new System.Collections.ArrayList(); //System.Linq.IQueryable type of data is not specific runtime defered support strArrayList.Add("Mahsa"); // "Mahsa": is string strArrayList.Add(1); // 1 : is integer strArrayList.Add(0.89); // 0.89: is decimal this.ListBoxArrayList.DataSource = null; this.ListBoxArrayList.Items.Clear(); this.ListBoxArrayList.DataSource = strArrayList; this.ListBoxArrayList.DataBind(); System.Text.StringBuilder str= new System.Text.StringBuilder(); foreach (var item in strArrayList) { str.Append(" , "+item); } this.lblArrayList.Text = str.ToString(); //Below is old way to fill obj from product , in Arraylist you need to create more than one instance // Product objProduct = new Product(); // objProduct.Code = "1001"; // objProduct.Name = "Chair"; //It is NOT obvious that strArrayList is //1. string? int? object? decimal? OR OBJECT?? --> NOT Strongly Type //2. Sized=10? 20? 100? -->NOT Fixed Size // Namespace: System.Collections System.Collections.ArrayList objArrayList = new System.Collections.ArrayList(); objArrayList.Add(new Product("1001", "Chair")); objArrayList.Add(new Product("1002", "Sofa")); objArrayList.Add(new Product("1003", "Carpet")); this.DropDownListArrayListObject.DataSource = null; this.DropDownListArrayListObject.Items.Clear(); this.DropDownListArrayListObject.DataSource = objArrayList; //* Finding among Object of Array List is difficult , you have to find your specific item by index Product objTemp = (Product)objArrayList[0]; objArrayList.Remove(objTemp); //* this.DropDownListArrayListObject.DataTextField = "_Name"; this.DropDownListArrayListObject.DataValueField = "_Code"; this.DropDownListArrayListObject.DataBind(); this.GridViewArrayListObject.DataSource = objArrayList; this.GridViewArrayListObject.DataBind();
HashTable


Collapse | Copy
Code
//It is NOT obvious that strArrayList is //1. string? int? object? decimal? OR OBJECT?? --> NOT Strongly Type //2. Sized=10? 20? 100? -->NOT Fixed Size // Namespace: System.Collections //Hashtable solve the problem in Arraylist when we are looking for specific item //Hashtable dedicate a key for each item, then finding item is easier and faster System.Collections.Hashtable objHashTable = new System.Collections.Hashtable(); objHashTable.Add("1001","Chair"); objHashTable.Add("1002", "Sofa"); objHashTable.Add("1003", "Carpet"); this.DropDownListHashTable.DataSource = null; this.DropDownListHashTable.Items.Clear(); this.DropDownListHashTable.DataSource = objHashTable; //* finding item is easier you just need to point to it by call its key objHashTable.Remove("1002"); //* this.DropDownListHashTable.DataTextField = "Value"; this.DropDownListHashTable.DataValueField = "Key"; this.DropDownListHashTable.DataBind();
Stack

Stack - Push

Collapse | Copy
Code
//Stack is LIFO: Last in First Out System.Collections.Stack objStackPush = new System.Collections.Stack(); //By Push method you can insert item at the top of the stack objStackPush.Push("Mahsa"); objStackPush.Push("Hassankashi"); this.lblPop.Text = ""; this.ListBoxStack.DataSource = objStackPush.ToArray(); this.ListBoxStack.DataBind();
Stack - Pop

Collapse | Copy
Code
System.Collections.Stack objStackPop = new System.Collections.Stack(); objStackPop.Push("Mahsa"); objStackPop.Push("Hassankashi"); //By Pop method you can remove item from the top of the stack --> Last in First in this.lblPop.Text = objStackPop.Pop().ToString(); this.ListBoxStack.DataSource = objStackPop.ToArray(); this.ListBoxStack.DataBind();
Queue

Queue - Enqueue

Collapse | Copy
Code
//Queue is FIFO: First in First Out System.Collections.Queue objQueue = new System.Collections.Queue(); //By Enqueue method you can insert item at the END of the Queue objQueue.Enqueue("Mahsa"); objQueue.Enqueue("Hassankashi"); objQueue.Enqueue("Cosmic"); objQueue.Enqueue("Verse"); this.lblQueue.Text = ""; this.ListBoxQueue.DataSource = objQueue.ToArray(); this.ListBoxQueue.DataBind();
Queue - Dequeue

Collapse | Copy
Code
System.Collections.Queue objQueue = new System.Collections.Queue(); objQueue.Enqueue("Mahsa"); objQueue.Enqueue("Hassankashi"); objQueue.Enqueue("Cosmic"); objQueue.Enqueue("Verse"); //By Dequeue method you can remove item from the BEGINING of the Queue --> First in First out FIFO this.lblQueue.Text=objQueue.Dequeue().ToString(); this.ListBoxQueue.DataSource = objQueue.ToArray(); this.ListBoxQueue.DataBind();
List
Why we needList? 1. List does not have spesific length à It is possible to have data without knowing its size , it is good feature because of at the first we are not likely to sure about length of collection. 2. List has Spesific type so it is strong type,therfore it has better perfomance and high speed in comparison with ArrayList and reduce unwanted error in compile time. 3. Use “foreach” keyword for iterate through it.


Collapse | Copy
Code
//Like Array is Strong Type //Like ArrayList with No Dimension System.Collections.Generic.List<string> strList = new List<string>(); strList.Add("Mahsa"); strList.Add("Hassankashi"); strList.Add("Cosmic"); strList.Add("Verse"); this.ListBoxListGeneric.DataSource = strList; this.ListBoxListGeneric.DataBind(); System.Text.StringBuilder str = new System.Text.StringBuilder(); foreach (var item in strList) { str.Append(" , " + item); } this.lblList.Text = str.ToString();
IList
Why we need IList? Ilist is an interface of list (does everything that List does) and to observe polymorphism in order to decouple and control on add or remove method that implement on the client side, everything else are similar to List. Whenever we want tochange on some operation “IList” allow us to do that easily with at least changing in the whole of codes. Ilist can not be instantiate from Ilist , so it should be instantiate from List

Collapse | Copy
Code
System.Collections.Generic.IList<string> strIList = new List<string>();
Difference between Abstract and Interface
1.Abstract inherit from just one class but Interface implements from one or more interfaces 2.Abstract can have non concrete method (expand method) but you have to define just signature inside interface 3.Inside Abstract class you can define variable and valuebut you are not allowed to define variable inside interface 4.An abstract class can have constructor but inside interface you are not allowed to define constructor 5.You can write scope for member of interface, red ones donot allow but blue one allow Ilist
is an interface and as I mentioned that how a class cannot be driven from two class it just can be driven from only one class, so whenever you want to drive from two class it is not possible to inherit from two abstract class but it is possible to drive from
one class and another one Ilist as interface Later in future you decide add some features on your class and inherit it from another class So because of this reason we always prefer to use interface of collection so that if you want to change your code and
enhance its abilities it is possible to grow it On the otherhand interface keeps your program extensible and Decouple: The classes are independent from each other error, exeption and failur will happen rarly in future changing code Polymorphism: When you are
using interface you absolutly observe and do polymorphism and oop it means encapsulate your designer Interface means a point or a node to join two part to eachother it means making low dependency from two part and make a joint section to make felxible chang
in future

Collapse | Copy
Code
//Ilist can not be instantiate from Ilist , so it should be instantiate from List System.Collections.Generic.IList<string> strIList = new List<string>(); strIList.Add("Mahsa"); strIList.Add("Hassankashi"); strIList.Add("Cosmic"); strIList.Add("Verse"); this.ListBoxListGeneric.DataSource = strIList; this.ListBoxListGeneric.DataBind(); System.Text.StringBuilder str = new System.Text.StringBuilder(); foreach (var item in strIList) { str.Append(" , " + item); } this.lblList.Text = str.ToString();
IList is similar to List but with this difference that we prefer to use INTERFACE instead of abstract in programming because future changing is easy
IEnumerable
IEnumerable is suitable just for iterate through collection and you can not modify (Add or Remove) it IEnumerable bring ALL data from server to client then filter them, assume that you have a lot of records so IEnumerable puts overhead on your memory

Collapse | Copy
Code
//IEnumerable can not be instantiate from Enumerable , so it should be instantiate from List System.Collections.Generic.IEnumerable<Employee> empIEnumerable = new List<Employee> { new Employee { ID = 1001, Name="Mahsa"}, new Employee { ID = 1002, Name = "Hassankashi" }, new Employee { ID = 1003, Name = "CosmicVerse" }, new Employee { ID = 1004, Name = "Technical" } }; this.GridViewIEnumerable.DataSource = empIEnumerable; this.GridViewIEnumerable.DataBind(); System.Text.StringBuilder str = new System.Text.StringBuilder(); foreach (Employee item in empIEnumerable) { str.Append(" , " + item.ID +"-"+item.Name); } this.lblIEnumerable.Text = str.ToString();
IQueryable

Follow "Entity Framework DatabaseFirst" from this article for making DBContext

Collapse | Copy
Code
DataAccessEntities ctx = new DataAccessEntities(); var ctx = new DataAccessEntities();
Data Access

Collapse | Copy
Code
//Difference between IQueryable and IEnumerable //You can instantiate IEnumerable from List IEnumerable<Employee> queryIEnumerable = new List<Employee>() ; //Bring ALL records from server --> to client then filter collection queryIEnumerable = (from m in ctx.Employees where m.ID == 1 select m).ToList(); //You can not instantiate IQueryable IQueryable<Employee> queryIQueryable=null; //Bring just ONE record from server --> to client queryIQueryable = (from m in ctx.Employees where m.ID == 1 select m); this.GridViewIQueryable.DataSource = queryIQueryable.ToList(); this.GridViewIQueryable.DataBind();
ICollection

Collapse | Copy
Code
//IList {indexer and Modify} vs ICollection {randomly and Modify} //Collection can not be instantiate from ICollection , so it should be instantiate from List System.Collections.Generic.ICollection<string> strICollection = new List<string>(); strICollection.Add("Mahsa"); strICollection.Add("Hassankashi"); //**Countable int ICollectionCount=strICollection.Count; this.ListBoxICollection.DataSource = strICollection; this.ListBoxICollection.DataBind(); System.Text.StringBuilder str = new System.Text.StringBuilder(); foreach (var item in strICollection) { str.Append(" , " + item); } this.lblICollection.Text = str.ToString(); //***IList System.Collections.Generic.IList<Employee> objIList = new List<Employee>(); objIList = (from m in ctx.Employees select m).ToList(); Employee obj = objIList.Where(i => i.Name == "Sara").FirstOrDefault(); int indexofSara= objIList.IndexOf(obj); int cIList = objIList.Count; //***ICollection System.Collections.Generic.ICollection<Employee> objICollection = new List<Employee>(); objICollection = (from m in ctx.Employees select m).ToList(); Employee objIC = objICollection.Where(i => i.Name == "Sara").FirstOrDefault(); //You can not get index of object , if you clear comment from below code appears error // int indexofSaraICollection = objIC.IndexOf(objIC); int cICollection = objICollection.Count;
Dictionary

Collapse | Copy
Code
//Dictionary can instantiate from Dictionary , Dictionary is similar to Hashtable, //Dictionary is GENERIC but Hashtable is NON GENERIC //Such Hashtable you can find object by its key System.Collections.Generic.Dictionary<int, string=""> objDictionary = new Dictionary<int, string="">(); objDictionary.Add(1001, "Mahsa"); objDictionary.Add(1002, "Hassankashi"); objDictionary.Add(1003, "Cosmicverse"); string str = objDictionary[1002]; this.ListBoxDictionary.DataSource = objDictionary; this.ListBoxDictionary.DataBind();</int,></int,>
MVC Tutorial: http://technical.cosmicverse.info/Article/Index/3
Data Access Layer Tutorial: http://technical.cosmicverse.info/Article/Index/4
References
http://www.codeproject.com/Articles/732425/IEnumerable-Vs-IQueryablehttp://www.dotnet-tricks.com/Tutorial/linq/I8SY160612-IEnumerable-VS-IQueryable.html
http://www.claudiobernasconi.ch/2013/07/22/when-to-use-ienumerable-icollection-ilist-and-list
相关文章推荐
- 最全数据结构详述: List VS IEnumerable VS IQueryable VS ICollection VS IDictionary
- 最全数据结构详述: List VS IEnumerable VS IQueryable VS ICollection VS IDictionary
- List vs IEnumerable vs IQueryable vs ICollection vs IDictionary
- 最全数据结构详述: List VS IEnumerable VS IQueryable VS ICollection VS IDictionary
- IEnumerable vs IQueryable
- [C#] IEnumerable vs IQueryable
- 对IEnumerable<T>,IDictionary<Tkey,TValue>,ICollection<T>,IList<T>的总结
- 解决 ”不允许在查询中显式构造实体类型“问题及使用其他方法实现返回 List<Model对象>或者IQueryable<Model对象>对象
- LINQ : IEnumerable<T> and IQueryable<T>区别
- IEnumerable/ICollection/IList/List继承关系
- List<T> 和IQueryable<T>关键性的不同在哪?
- linq中查询列表的使用及iqueryable和list集合之间的转换
- MVC分页控件之二,为IQueryable定义一个扩展方法,直接反回PagedList<T>结果集
- IEnumerable<T>与IQueryable<T>以及.net的扩展方法
- IList to IQueryable
- IEnumerable<T>和IQueryable<T>区分
- IQueryable和list本地集合区别
- "IQueryable and IEnumerable"区别
- 【转载】MVC分页控件之二,为IQueryable定义一个扩展方法,直接反回PagedList<T>结果集
- IEnumerable<T>和IQueryable<T>区分