soap消息分析和soap消息的传递和处理(一)
2014-10-21 20:48
381 查看
WebService传递的时候实际上是传递一个SOAPMessage,我们来探究一下SOAPMessage的组成。
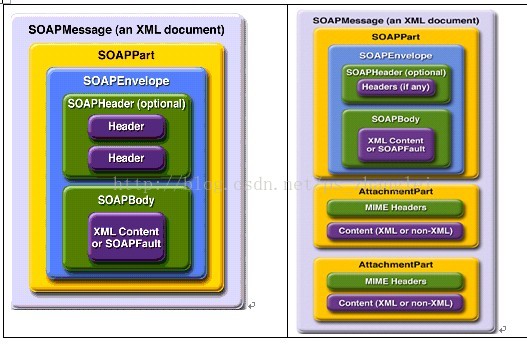
SOAPMessage由一个个的SOAP块组成,这些SOAPPart被封装到一个SOAPEnvelope(信封)中,信封中包括head和body。我们可以自己创建一个SOAPMessage发送到服务提供端,从而返回WebService调用结果。
WebService服务创建代码参考上篇,本篇探讨SOAPMessage的组成和发送。
TestClient.java
package com.smile.service;
import java.io.IOException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.soap.MessageFactory;
import javax.xml.soap.SOAPBody;
import javax.xml.soap.SOAPBodyElement;
import javax.xml.soap.SOAPEnvelope;
import javax.xml.soap.SOAPException;
import javax.xml.soap.SOAPMessage;
import javax.xml.soap.SOAPPart;
import javax.xml.ws.Dispatch;
import javax.xml.ws.Service;
import org.junit.Test;
public class TestClient {
private String wsdlUrl = "http://localhost:8889/ns?wsdl";
private String ns = "http://service.smile.com/";
@Test
public void test(){
try {
//创建消息工厂
MessageFactory factory = MessageFactory.newInstance();
//根据消息工厂创建SOAPMessage
SOAPMessage message = factory.createMessage();
//创建SOAPPart
SOAPPart part = message.getSOAPPart();
//获取SOAPEnvelope soap信封
SOAPEnvelope envelope = part.getEnvelope();
//可以通过SOAPEnvelope获取head body等信息
SOAPBody body = envelope.getBody();
//根据QName 创建相应的节点
QName qname = new QName("http://smile.web.com/webservice", "add", "ws");
//<ws:add xmlns="http://smile.web.com/webservice"/>
//使用以下方式设置< > 会被转义
// body.addBodyElement(qname).setValue("<a>1</a><b>2</b>");
SOAPBodyElement element = body.addBodyElement(qname);
element.addChildElement("a").setValue("1");
element.addChildElement("b").setValue("2");
try {
message.writeTo(System.out);
} catch (IOException e) {
e.printStackTrace();
}
} catch (SOAPException e) {
e.printStackTrace();
}
}
@Test
public void test2(){
try {
//创建服务(Service)
URL url = new URL(wsdlUrl);
QName sname = new QName(ns,"MyServiceImplService");
Service service = Service.create(url, sname);
//创建Dispatch
Dispatch<SOAPMessage> dispatch = service.createDispatch(new QName(ns,"MyServiceImplPort"),
SOAPMessage.class,Service.Mode.MESSAGE);
//创建消息工厂
MessageFactory factory = MessageFactory.newInstance();
//根据消息工厂创建SOAPMessage
SOAPMessage message = factory.createMessage();
//创建SOAPPart
SOAPPart part = message.getSOAPPart();
//获取SOAPEnvelope soap信封
SOAPEnvelope envelope = part.getEnvelope();
//可以通过SOAPEnvelope获取head body等信息
SOAPBody body = envelope.getBody();
//根据QName 创建相应的节点
QName ename = new QName(ns, "add", "ws");
//<ws:add xmlns="http://smile.web.com/webservice"/>
//使用以下方式设置< > 会被转义
// body.addBodyElement(qname).setValue("<a>1</a><b>2</b>");
SOAPBodyElement element = body.addBodyElement(ename);
element.addChildElement("a").setValue("22");
element.addChildElement("b").setValue("33");
message.writeTo(System.out);
System.out.println("\t");
System.out.println("Invoking.....");
SOAPMessage response = dispatch.invoke(message);
response.writeTo(System.out);
} catch (Exception e) {
e.printStackTrace();
}
}
}
SOAPMessage由一个个的SOAP块组成,这些SOAPPart被封装到一个SOAPEnvelope(信封)中,信封中包括head和body。我们可以自己创建一个SOAPMessage发送到服务提供端,从而返回WebService调用结果。
WebService服务创建代码参考上篇,本篇探讨SOAPMessage的组成和发送。
TestClient.java
package com.smile.service;
import java.io.IOException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.soap.MessageFactory;
import javax.xml.soap.SOAPBody;
import javax.xml.soap.SOAPBodyElement;
import javax.xml.soap.SOAPEnvelope;
import javax.xml.soap.SOAPException;
import javax.xml.soap.SOAPMessage;
import javax.xml.soap.SOAPPart;
import javax.xml.ws.Dispatch;
import javax.xml.ws.Service;
import org.junit.Test;
public class TestClient {
private String wsdlUrl = "http://localhost:8889/ns?wsdl";
private String ns = "http://service.smile.com/";
@Test
public void test(){
try {
//创建消息工厂
MessageFactory factory = MessageFactory.newInstance();
//根据消息工厂创建SOAPMessage
SOAPMessage message = factory.createMessage();
//创建SOAPPart
SOAPPart part = message.getSOAPPart();
//获取SOAPEnvelope soap信封
SOAPEnvelope envelope = part.getEnvelope();
//可以通过SOAPEnvelope获取head body等信息
SOAPBody body = envelope.getBody();
//根据QName 创建相应的节点
QName qname = new QName("http://smile.web.com/webservice", "add", "ws");
//<ws:add xmlns="http://smile.web.com/webservice"/>
//使用以下方式设置< > 会被转义
// body.addBodyElement(qname).setValue("<a>1</a><b>2</b>");
SOAPBodyElement element = body.addBodyElement(qname);
element.addChildElement("a").setValue("1");
element.addChildElement("b").setValue("2");
try {
message.writeTo(System.out);
} catch (IOException e) {
e.printStackTrace();
}
} catch (SOAPException e) {
e.printStackTrace();
}
}
@Test
public void test2(){
try {
//创建服务(Service)
URL url = new URL(wsdlUrl);
QName sname = new QName(ns,"MyServiceImplService");
Service service = Service.create(url, sname);
//创建Dispatch
Dispatch<SOAPMessage> dispatch = service.createDispatch(new QName(ns,"MyServiceImplPort"),
SOAPMessage.class,Service.Mode.MESSAGE);
//创建消息工厂
MessageFactory factory = MessageFactory.newInstance();
//根据消息工厂创建SOAPMessage
SOAPMessage message = factory.createMessage();
//创建SOAPPart
SOAPPart part = message.getSOAPPart();
//获取SOAPEnvelope soap信封
SOAPEnvelope envelope = part.getEnvelope();
//可以通过SOAPEnvelope获取head body等信息
SOAPBody body = envelope.getBody();
//根据QName 创建相应的节点
QName ename = new QName(ns, "add", "ws");
//<ws:add xmlns="http://smile.web.com/webservice"/>
//使用以下方式设置< > 会被转义
// body.addBodyElement(qname).setValue("<a>1</a><b>2</b>");
SOAPBodyElement element = body.addBodyElement(ename);
element.addChildElement("a").setValue("22");
element.addChildElement("b").setValue("33");
message.writeTo(System.out);
System.out.println("\t");
System.out.println("Invoking.....");
SOAPMessage response = dispatch.invoke(message);
response.writeTo(System.out);
} catch (Exception e) {
e.printStackTrace();
}
}
}
相关文章推荐
- twitter storm 源码走读之5 -- worker进程内部消息传递处理和数据结构分析
- soap消息的传递和处理(三)——包含header和异常的处理
- soap消息的分析与处理
- SOAP消息的创建、传递和处理(MESSAGE方式)
- twitter storm 源码走读之5 -- worker进程内部消息传递处理和数据结构分析
- soap消息传递和处理(基于Message和Payload的方式)
- Webservice_12_传递SOAP的消息和处理
- soap消息的传递与处理(二)(基于payload的方式)
- Webservice06---SOAP消息的传递和处理(基于Message方式)
- SOAP消息的传递和处理(PAYLOAD方式)
- Webservice_13_Payload的方式传递SOAP的消息和处理 和 用SOAPMessage传递SOAP的消息和处理(无参数)
- SOAP消息的创建,传递,处理的学习笔记
- 在PostMessage或SendMessage中通过WPARAM或者LPARAM将数组传递给消息处理函数
- 第二人生的源码分析(四十二)实现消息处理的线程类
- 第二人生的源码分析(三十七)消息处理的完整流程
- 蔡军生先生第二人生的源码分析(二十四)人物向前走的键盘消息处理
- 第二人生的源码分析(二十四)人物向前走的键盘消息处理
- 第二人生的源码分析(四十二)实现消息处理的线程类
- 为何在自定义消息处理函数中无法利用wParam或lParam传递指针?
- TOMCAT源码分析(消息处理)