[Swift A] - 实战-豆瓣电台总结
2014-09-18 20:34
183 查看
最近在学Swift,也是刚刚开始。这里对自己最近所学做个简单的总结:视频和代码都在下面
http://pan.baidu.com/s/1sjHd5qX
1.String和NSString的不同
2.异步获取数据
3.UI焦点的易操作
在UI的界面种可以直接在View上点击鼠标右键,并拖入相应的Controller的代码中,且生成@IBOutlet和@IBAction。代码都不用手写了,很方便。
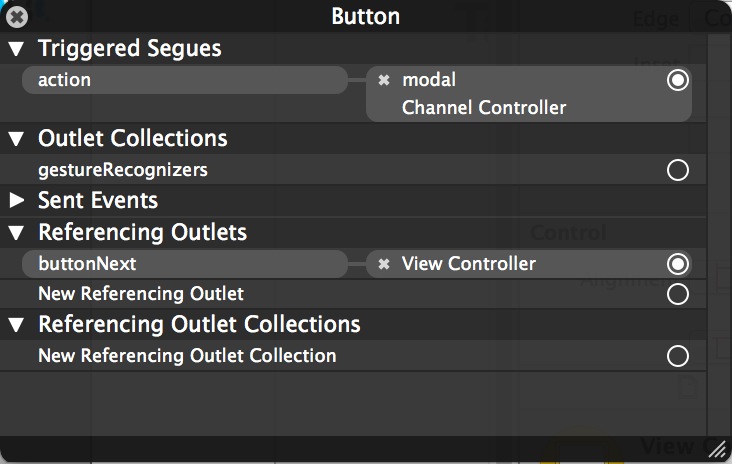
4.视图之间的跳转,传参,回跳
5.MP3在线播放
6.TableView列表Item的出现动画
7.UI控件汇总
8.最后把代码贴出来以供学习
http://pan.baidu.com/s/1sjHd5qX
1.String和NSString的不同
Swift的String类型是值类型。如果你创建了一个新的字符串值,那么当其进行常量、变量赋值操作或在函数/方法中传递时,会进行值拷贝。 在不同的情况下,都会对已有字符串值创建新的副本,并对该新副本进行传递或赋值。 这和OC中的NSString不同,当您在OC创建了一个NSString实例,并将其传递给一个函数/方法,或者赋给一个变量,您永远都是传递或赋值同一个NSString实例的一个引用。 除非您特别要求其进行值拷贝,否则字符串不会进行赋值新副本操作。
2.异步获取数据
NSURLConnection.sendAsynchronousRequest(request, queue: NSOperationQueue.mainQueue(), completionHandler:{(response:NSURLResponse!, data:NSData!, error:NSError!)->Void in var jsonResult:NSDictionary = NSJSONSerialization.JSONObjectWithData(data!, options: NSJSONReadingOptions.MutableContainers, error: nil) as NSDictionary //why just miss an excalmatory mark, the code isn't compile //self.delegate?.didRecieveResults(jsonResult) })
3.UI焦点的易操作
在UI的界面种可以直接在View上点击鼠标右键,并拖入相应的Controller的代码中,且生成@IBOutlet和@IBAction。代码都不用手写了,很方便。
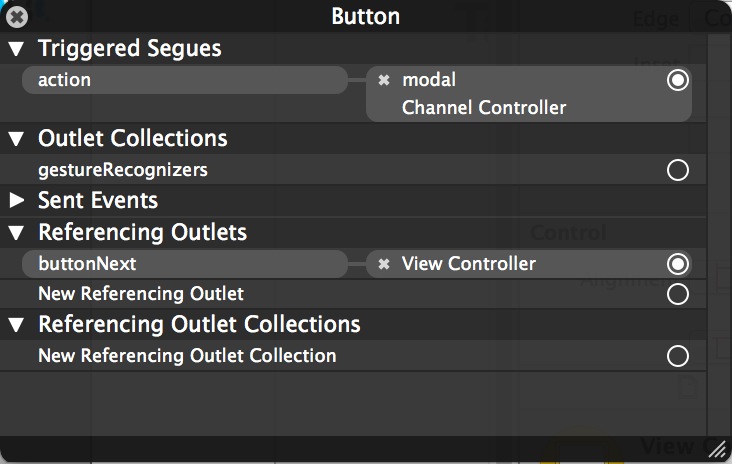
4.视图之间的跳转,传参,回跳
//页面跳转时,将数据传过去 override func prepareForSegue(segue: UIStoryboardSegue!, sender: AnyObject!) { var channelC:ChannelController = segue.destinationViewController as ChannelController channelC.delegate = self// What TODO channelC.channelData = self.channelData }
//点击事件 func tableView(tableView:UITableView!, didSelectRowAtIndexPath indexPath:NSIndexPath!) { //what is do this var rowData:NSDictionary = self.channelData[indexPath.row] as NSDictionary let channelId:AnyObject = rowData["channel_id"] as AnyObject let channel:String = "channel=\(channelId)" delegate?.onChangeChannel(channel) self.dismissViewControllerAnimated(true, completion: nil) }
5.MP3在线播放
//定义一个播放器 var audioPlayer:MPMoviePlayerController = MPMoviePlayerController() //设置音乐 func onSetAudio(url:String) { timer?.invalidate() timeLabel.text = "00:00" self.audioPlayer.stop() self.audioPlayer.contentURL = NSURL(string:url) self.audioPlayer.play() timer = NSTimer.scheduledTimerWithTimeInterval(0.4 , target : self, selector : "onUpdate", userInfo: nil, repeats :true) playBtn.removeGestureRecognizer(tap) logo.addGestureRecognizer(tap) playBtn.hidden = true }
6.TableView列表Item的出现动画
func tableView(tableView: UITableView!, willDisplayCell cell: UITableViewCell!, forRowAtIndexPath indexPath: NSIndexPath!) { cell.layer.transform = CATransform3DMakeScale(0.1, 0.1, 1) UIView.animateWithDuration(0.25, animations:{cell.layer.transform=CATransform3DMakeScale(1, 1, 1)}) }
7.UI控件汇总
UIViewController UITableViewDataSource UITableViewDelegate UIImageView UILabel UIButton UIProgressView UITableView UITapGestureRecognizer UIImage MediaPlayer
8.最后把代码贴出来以供学习
import UIKit import MediaPlayer import QuartzCore class ViewController: UIViewController ,UITableViewDataSource, UITableViewDelegate, HttpProtocol, ChannelProtocol{ @IBOutlet var logo: UIImageView @IBOutlet var timeLabel: UILabel @IBOutlet var buttonNext: UIButton @IBOutlet var progressBar: UIProgressView @IBOutlet var itemLayout: UITableView //开始点击事件 @IBOutlet var tap: UITapGestureRecognizer = nil //这里必须为空,否则报错 @IBOutlet var playBtn: UIImageView //to cache the picture of songs var imageCache = Dictionary<String, UIImage>() var eHttp:HttpController = HttpController() //主界面 var tableData:NSArray = NSArray() //频道列表 var channelData:NSArray = NSArray() //定义一个播放器 var audioPlayer:MPMoviePlayerController = MPMoviePlayerController() var timer:NSTimer? @IBAction func onTap(sender: UITapGestureRecognizer) { if sender.view == playBtn { playBtn.hidden = true audioPlayer.play() playBtn.removeGestureRecognizer(tap) logo.addGestureRecognizer(tap) } else if sender.view == logo{ playBtn.hidden = false audioPlayer.pause() playBtn.addGestureRecognizer(tap) logo.removeGestureRecognizer(tap) } } override func viewDidLoad() { super.viewDidLoad() //视图加载完之后,获取数据 eHttp.delegate = self eHttp.onSearch("http://www.douban.com/j/app/radio/channels") eHttp.onSearch("http://douban.fm/j/mine/playlist?channel=0") progressBar.progress = 0.0 logo.addGestureRecognizer(tap) } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() // Dispose of any resources that can be recreated. } func tableView(tableView: UITableView!, willDisplayCell cell: UITableViewCell!, forRowAtIndexPath indexPath: NSIndexPath!) { cell.layer.transform = CATransform3DMakeScale(0.1, 0.1, 1) UIView.animateWithDuration(0.25, animations:{cell.layer.transform=CATransform3DMakeScale(1, 1, 1)}) } //TODO override func prepareForSegue(segue: UIStoryboardSegue!, sender: AnyObject!) { var channelC:ChannelController = segue.destinationViewController as ChannelController channelC.delegate = self// What TODO channelC.channelData = self.channelData } func onChangeChannel(channelId:String) { let url:String = "http://douban.fm/j/mine/playlist?\(channelId)" eHttp.onSearch(url) } func tableView(tableView: UITableView!, numberOfRowsInSection section: Int) -> Int { return tableData.count } //列表展示 func tableView(tableView: UITableView!, cellForRowAtIndexPath indexPath: NSIndexPath!) -> UITableViewCell! { let cell = UITableViewCell(style: UITableViewCellStyle.Subtitle, reuseIdentifier: "songs") let rowData:NSDictionary = self.tableData[indexPath.row] as NSDictionary cell.text = rowData["title"] as String cell.detailTextLabel.text = rowData["artist"] as String //默认图片 cell.image = UIImage(named:"detail.jpg") //缩略图 let url = rowData["picture"] as String let image = self.imageCache[url] as? UIImage if !image?{// why do this let imgURL:NSURL = NSURL(string:url) let request:NSURLRequest = NSURLRequest(URL:imgURL) NSURLConnection.sendAsynchronousRequest(request, queue: NSOperationQueue.mainQueue(), completionHandler: {(response:NSURLResponse!, data:NSData!, error:NSError!) -> Void in let img = UIImage(data:data) cell.image = img //缓存图片 self.imageCache[url] = img }) } else {// if no this else, the item will be default image cell.image = image } return cell } func didRecieveResults(results:NSDictionary) { //println(results); if(results["channels"]) { self.channelData = results["channels"] as NSArray //self.itemLayout.reloadData() } else if(results["song"]) { self.tableData = results["song"] as NSArray self.itemLayout.reloadData() let firDict:NSDictionary = self.tableData[0] as NSDictionary let audioUrl:String = firDict["url"] as String onSetAudio(audioUrl) let imgUrl:String = firDict["picture"] as String onSetImage(imgUrl) } } func tableView(tableView:UITableView!, didSelectRowAtIndexPath indexPath:NSIndexPath!) { let rowData:NSDictionary = self.tableData[indexPath.row] as NSDictionary let audioUrl:String = rowData["url"] as String onSetAudio(audioUrl) let imgUrl:String = rowData["picture"] as String onSetImage(imgUrl) } //设置音乐 func onSetAudio(url:String) { timer?.invalidate() timeLabel.text = "00:00" self.audioPlayer.stop() self.audioPlayer.contentURL = NSURL(string:url) self.audioPlayer.play() timer = NSTimer.scheduledTimerWithTimeInterval(0.4 , target : self, selector : "onUpdate", userInfo: nil, repeats :true) playBtn.removeGestureRecognizer(tap) logo.addGestureRecognizer(tap) playBtn.hidden = true } func onUpdate() { let c = audioPlayer.currentPlaybackTime if c > 0.0 { let t = audioPlayer.duration let p:CFloat=CFloat(c/t) progressBar.setProgress(p, animated: true) let allSecond:Int = Int(c) let s:Int = allSecond%60 let m:Int = Int(allSecond/60) var time:String = "" if(m < 10) { time = "0\(m)" } else { time = "\(m)" } if(s < 10) { time += ":0\(s)" } else { time += ":\(s)" } timeLabel.text = time } } //设置图片 func onSetImage(url:String) { let image = self.imageCache[url] as? UIImage if !image?{// why do this let imgURL:NSURL = NSURL(string:url) let request:NSURLRequest = NSURLRequest(URL:imgURL) NSURLConnection.sendAsynchronousRequest(request, queue: NSOperationQueue.mainQueue(), completionHandler: {(response:NSURLResponse!, data:NSData!, error:NSError!) -> Void in let img = UIImage(data:data) self.logo.image = img //缓存图片 self.imageCache[url] = img }) } else {// if no this else, the item will be default image self.logo.image = image } } }
import UIKit protocol HttpProtocol { func didRecieveResults(results:NSDictionary) } class HttpController : NSObject { var delegate:HttpProtocol? func onSearch(url:String) { var nsUrl:NSURL = NSURL(string:url) var request:NSURLRequest = NSURLRequest(URL:nsUrl) //1.Closure Expression Syntax //2.异步获取数据 NSURLConnection.sendAsynchronousRequest(request, queue: NSOperationQueue.mainQueue(), completionHandler:{(response:NSURLResponse!, data:NSData!, error:NSError!)->Void in var jsonResult:NSDictionary = NSJSONSerialization.JSONObjectWithData(data!, options: NSJSONReadingOptions.MutableContainers, error: nil) as NSDictionary //why just miss an excalmatory mark, the code isn't compile //self.delegate?.didRecieveResults(jsonResult) }) } }
import UIKit import QuartzCore protocol ChannelProtocol { func onChangeChannel(channelId:String)// why is String, is not NSString } class ChannelController: UIViewController, UITableViewDataSource, UITableViewDelegate { @IBOutlet var listLayout: UITableView var channelData:NSArray = NSArray() var delegate:ChannelProtocol? override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view, typically from a nib. } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() // Dispose of any resources that can be recreated. } func tableView(tableView: UITableView!, numberOfRowsInSection section: Int) -> Int { return 10 } func tableView(tableView: UITableView!, willDisplayCell cell: UITableViewCell!, forRowAtIndexPath indexPath: NSIndexPath!) { cell.layer.transform = CATransform3DMakeScale(0.1, 0.1, 1) UIView.animateWithDuration(0.25, animations:{cell.layer.transform=CATransform3DMakeScale(1, 1, 1)}) } func tableView(tableView: UITableView!, cellForRowAtIndexPath indexPath: NSIndexPath!) -> UITableViewCell! { let cell = UITableViewCell(style: UITableViewCellStyle.Subtitle, reuseIdentifier: "channel") let rowData:NSDictionary = self.channelData[indexPath.row] as NSDictionary cell.text = rowData["name"] as String return cell } func tableView(tableView:UITableView!, didSelectRowAtIndexPath indexPath:NSIndexPath!) { //what is do this var rowData:NSDictionary = self.channelData[indexPath.row] as NSDictionary let channelId:AnyObject = rowData["channel_id"] as AnyObject let channel:String = "channel=\(channelId)" delegate?.onChangeChannel(channel) self.dismissViewControllerAnimated(true, completion: nil) } }
相关文章推荐
- Swift实战-豆瓣电台(三)获取网络数据
- Swift实战-豆瓣电台(五)播放音乐
- iOS开发——完整项目实战Swift篇&百思不得姐Swift版总结(一)
- Swift实战-豆瓣电台(一)准备
- Swift实战-豆瓣电台(八)播放进度与时间
- Swift实战-豆瓣电台(七)显示动画
- Swift实战-豆瓣电台(五)播放音乐
- swift实战-豆瓣电台
- Swift实战-豆瓣电台(九)简单手势控制暂停播放(全文完)
- Swift实战-豆瓣电台(九)简单手势控制暂停播放(全文完)
- Swift实战-豆瓣电台(四)歌曲列表的展现
- Swift实战-豆瓣电台(四)歌曲列表的展现
- Swift实战-豆瓣电台(八)播放进度与时间
- Swift实战-豆瓣电台(二)界面布局
- Swift实战总结1
- Swift实战-豆瓣电台(六)视图跳转,传参及回跳
- iOS开发——完整项目实战Swift篇&百思不得姐Swift版总结(二)
- Swift实战-豆瓣电台(七)显示动画
- Swift实战-豆瓣电台(三)获取网络数据
- Swift实战-豆瓣电台(二)界面布局