Truthy and Falsy: When All is Not Equal in JavaScript
2014-09-06 04:46
561 查看
http://www.sitepoint.com/javascript-truthy-falsy/

Craig Buckler
Published July 1, 2009
TweetSubscribe
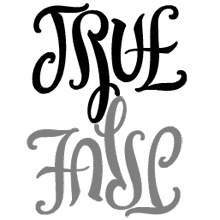
Like
most computer languages, JavaScript supports Boolean data types; values which can be set to true or false. In addition, everything in JavaScript has an inherent Boolean value, generally known as either truthy orfalsy.
Handling truthy and falsy values can be a little quirky, especially when comparing variables. Understanding some of the more bizarre rules can help when debugging complex client-side applications.
The following values are always falsy:
false
0 (zero)
"" (empty string)
null
undefined
NaN (a special Number value meaning Not-a-Number!)
All other values are truthy, including "0" (zero in quotes), "false" (false in quotes), empty functions, empty arrays, and empty objects.
Falsy values follow some slightly odd comparison rules which can lead to errors in program logic.
The falsy values false, 0 (zero), and "" (empty string) are all equivalent and can be compared against each other:
The falsy values null and undefined are not equivalent to anything except themselves:
Finally, the falsy value NaN is not equivalent to anything — including NaN!
You should also be aware that
function
If in doubt…
Use strict equal (===) and strict not equal (!==) in situations where truthy or falsy values could lead to logic errors. These operators ensure that the objects are compared by type and by value.
Has truthy or falsy logic ever caused you grief in JavaScript code?
Craig Buckler
Published July 1, 2009
TweetSubscribe
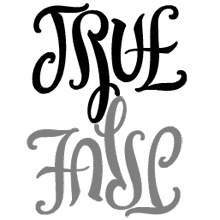
Like
most computer languages, JavaScript supports Boolean data types; values which can be set to true or false. In addition, everything in JavaScript has an inherent Boolean value, generally known as either truthy orfalsy.
Handling truthy and falsy values can be a little quirky, especially when comparing variables. Understanding some of the more bizarre rules can help when debugging complex client-side applications.
Truthy and Falsy Values
The following values are always falsy:false
0 (zero)
"" (empty string)
null
undefined
NaN (a special Number value meaning Not-a-Number!)
All other values are truthy, including "0" (zero in quotes), "false" (false in quotes), empty functions, empty arrays, and empty objects.
var a = !!(0); // variable is set to false var b = !!("0"); // true
Comparing Falsy Values
Falsy values follow some slightly odd comparison rules which can lead to errors in program logic.The falsy values false, 0 (zero), and "" (empty string) are all equivalent and can be compared against each other:
var c = (false == 0); // true var d = (false == ""); // true var e = (0 == ""); // true
The falsy values null and undefined are not equivalent to anything except themselves:
var f = (null == false); // false var g = (null == null); // true var h = (undefined == undefined); // true var i = (undefined == null); // true
Finally, the falsy value NaN is not equivalent to anything — including NaN!
var j = (NaN == null); // false var k = (NaN == NaN); // false
You should also be aware that
typeof(NaN)returns "number". Fortunately, the core JavaScript
function
isNaN()can be used to evaluate whether a value is NaN or not.
If in doubt…
Use strict equal (===) and strict not equal (!==) in situations where truthy or falsy values could lead to logic errors. These operators ensure that the objects are compared by type and by value.
var l = (false == 0); // true var m = (false === 0); // false
Has truthy or falsy logic ever caused you grief in JavaScript code?
相关文章推荐
- Truthy and Falsy Values in JavaScript
- Java - Why wait, notify and notifyAll is defined in Object Class and not on Thread class
- Pair programming is the most difficult and important thing in all agile actions
- Po price is NOT equal to RCV price and MMT price
- $.ajax not working in chrome but is working in firefox and IE
- Serial number is not available when doing any inventory transaction but shows in on hand quantity in
- is not abstract and does not override abstract method getIncludes() in org.apache.jasper.runtime.HttpJspBase 问题
- Only one expression can be specified in the select list when the subquery is not introduced with EXI
- WP7“Navigation is not allowed when the task is not in the foreground.”解决方案
- FTP not working on Java 7 on Windows 7 and VISTA when Windows Firewall is enabled
- FAQ: Why is file and line information available for some warnings in FxCop but not for others?
- use noscript html tag when user disable the javascript in browser, guide user how to enable the js in different browser and retu
- PRB: "Requested Registry Access Is Not Allowed" Error Message When ASP.NET Application Tries to Write New EventSource in the Eve
- 解决 Cross domain javascript callback is not supported in authenticated services
- Error occurred in deployment step 'Activate Features': Feature with Id '{GUID}' is not installed in this farm, and cannot be added to this scope.
- calculate all controls size and position in the form when the form resize
- Internet Information Services is not installed. You must have Internet Information Services installed in order to use the SharePoint Products and Technologies Configuration Wizard
- There is not a header with name ServiceContext and namespace http://schemas.microsoft.com/sharepoint/servicecontext in the messa
- Javascript truthy and falsy , Javascript logic operators || and &&
- WP7“Navigation is not allowed when the task is not in the foreground.”解决方案