Java界面 SWT基本组件——列表框(List)
2014-03-10 15:31
405 查看
列表框(List)的基本样式与基础应用
本次实验要求设计一个小程序来说明列表框的一些常用方法。
程序的主要功能:
1. 单击 “>” 按钮,将左侧选中的选项转移到右侧;
2. 单击 “<” 按钮,将右侧选中的选项转移到左侧;
3. 单击 “>>” 按钮,将左侧所有选项都转移到右侧;
4. 单击 “<<” 按钮,将右侧所有选项都转移到左侧;
5. 单击 "Up" 按钮,将所选的选项(右侧)向上移动一个位置;
6. 单击 “Down” 按钮,将所选的选项(右侧)向下移动一个位置;
在代码中,包含:
1. 创建事件监听类,为内部类;
2. 选项上移与下移过程中考虑边界;
代码(无static void main(String[] args))如下:(《EclipseSWT/JFace 核心应用》清华大学出版社)
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.List;
import org.eclipse.swt.widgets.Shell;
public class ListSample {
private Shell shell;
public ListSample(Shell sShell) {
shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(350, 250);
shell.setText("ListSample");
String itemLeft[] = new String[20]; // 定义保存左侧列表框中的数据
String itemright[] = new String[0]; // 定义保存右侧列表框中的数据
for(int i = 0; i < itemLeft.length; i++)
itemLeft[i] = "item" + i; // 初始化左侧字符串数组
final List left = new List(shell, SWT.MULTI|SWT.V_SCROLL);
// 定义左侧列表框,可选择多个且带有滚动条
left.setBounds(10, 10, 100, 180); // 设置位置和大小
left.setItems(itemLeft); // 设置选项数据
left.setToolTipText("Choosed List"); // 设置提示
final List right = new List(shell, SWT.MULTI|SWT.V_SCROLL);
// 定义右侧列表框,可选择多个且带有滚动条
right.setBounds(170, 10, 100, 180);
right.setItems(itemright);
right.setToolTipText("Choosed List");
// 创建事件监听类,为内部类
SelectionAdapter listener = new SelectionAdapter() {
// 按钮单击事件处理的方法
public void widgetSelected(SelectionEvent e) {
// 取得触发事件的空间对象(按钮)
Button bt = (Button)e.widget;
if(bt.getText().equals(">")) // 如果是“>”按钮
verifyValue(left.getSelection(), left, right);
else if(bt.getText().equals(">>")) // 如果是“>>”按钮
verifyValue(left.getItems(), left, right);
else if(bt.getText().equals("<")) // 如果是“<”按钮
verifyValue(right.getSelection(), right, left);
else if(bt.getText().equals("<<")) // 如果是“<<”按钮
verifyValue(right.getItems(), right, left);
else if(bt.getText().equals("Up")) // 如果是“Up”按钮
{
int index = right.getSelectionIndex();
// 获得当前选中选项的索引值
if(index <= 0) // 如未选中,则返回
return ;
String currentValue = right.getItem(index);
// 如果选中了选项值,获得当前选项的值
right.setItem(index, right.getItem(index - 1));
right.setItem(index - 1, currentValue);
// 将选中的选项与上一个选项交换值
right.setSelection(index - 1); // 设定上一选项为选中状态
}
else if(bt.getText().equals("Down")) // 如果是“Down”按钮
{
// 与“Up”按钮逻辑相同
int index = right.getSelectionIndex();
if(index >= right.getItemCount() - 1)
return ;
String currentValue = right.getItem(index);
right.setItem(index, right.getItem(index + 1));
right.setItem(index + 1, currentValue);
right.setSelection(index + 1);
}
}
};
// 定义按钮
// 定义(单)右移按钮
Button bt1 = new Button(shell, SWT.NONE);
bt1.setText(">");
bt1.setBounds(130, 20, 25, 20);
bt1.addSelectionListener(listener); // 为按钮注册事件,其他的按钮类似
// 定义(多)右移按钮
Button bt2 = new Button(shell, SWT.NONE);
bt2.setText(">>");
bt2.setBounds(130, 55, 25, 20);
bt2.addSelectionListener(listener);
// 定义(多)左移按钮
Button bt3 = new Button(shell, SWT.NONE);
bt3.setText("<<");
bt3.setBounds(130, 90, 25, 20);
bt3.addSelectionListener(listener);
// 定义(单)左移按钮
Button bt4 = new Button(shell, SWT.NONE);
bt4.setText("<");
bt4.setBounds(130, 125, 25, 20);
bt4.addSelectionListener(listener);
// 定义上移按钮
Button bt5 = new Button(shell, SWT.NONE);
bt5.setText("Up");
bt5.setBounds(285, 70, 40, 20);
bt5.addSelectionListener(listener);
// 定义下移按钮
Button bt6 = new Button(shell, SWT.NONE);
bt6.setText("Down");
bt6.setBounds(285, 105, 40, 20);
bt6.addSelectionListener(listener);
shell.open();
}
private void verifyValue(String[] selected, List from, List to) {
for(int i = 0; i < selected.length; i++)
{
from.remove(selected[i]); // 从一个列表中移除该选项值
to.add(selected[i]); // 添加到另一列表中
}
}
}
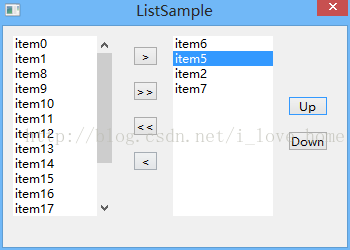
List 类所使用的样式常量
方法·向列表框中添加选项
方法·删除列表框中的选项
方法·获得列表框的状态
方法·对列表框中已选中的选项操作方法
本次实验要求设计一个小程序来说明列表框的一些常用方法。
程序的主要功能:
1. 单击 “>” 按钮,将左侧选中的选项转移到右侧;
2. 单击 “<” 按钮,将右侧选中的选项转移到左侧;
3. 单击 “>>” 按钮,将左侧所有选项都转移到右侧;
4. 单击 “<<” 按钮,将右侧所有选项都转移到左侧;
5. 单击 "Up" 按钮,将所选的选项(右侧)向上移动一个位置;
6. 单击 “Down” 按钮,将所选的选项(右侧)向下移动一个位置;
在代码中,包含:
1. 创建事件监听类,为内部类;
2. 选项上移与下移过程中考虑边界;
代码(无static void main(String[] args))如下:(《EclipseSWT/JFace 核心应用》清华大学出版社)
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.List;
import org.eclipse.swt.widgets.Shell;
public class ListSample {
private Shell shell;
public ListSample(Shell sShell) {
shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(350, 250);
shell.setText("ListSample");
String itemLeft[] = new String[20]; // 定义保存左侧列表框中的数据
String itemright[] = new String[0]; // 定义保存右侧列表框中的数据
for(int i = 0; i < itemLeft.length; i++)
itemLeft[i] = "item" + i; // 初始化左侧字符串数组
final List left = new List(shell, SWT.MULTI|SWT.V_SCROLL);
// 定义左侧列表框,可选择多个且带有滚动条
left.setBounds(10, 10, 100, 180); // 设置位置和大小
left.setItems(itemLeft); // 设置选项数据
left.setToolTipText("Choosed List"); // 设置提示
final List right = new List(shell, SWT.MULTI|SWT.V_SCROLL);
// 定义右侧列表框,可选择多个且带有滚动条
right.setBounds(170, 10, 100, 180);
right.setItems(itemright);
right.setToolTipText("Choosed List");
// 创建事件监听类,为内部类
SelectionAdapter listener = new SelectionAdapter() {
// 按钮单击事件处理的方法
public void widgetSelected(SelectionEvent e) {
// 取得触发事件的空间对象(按钮)
Button bt = (Button)e.widget;
if(bt.getText().equals(">")) // 如果是“>”按钮
verifyValue(left.getSelection(), left, right);
else if(bt.getText().equals(">>")) // 如果是“>>”按钮
verifyValue(left.getItems(), left, right);
else if(bt.getText().equals("<")) // 如果是“<”按钮
verifyValue(right.getSelection(), right, left);
else if(bt.getText().equals("<<")) // 如果是“<<”按钮
verifyValue(right.getItems(), right, left);
else if(bt.getText().equals("Up")) // 如果是“Up”按钮
{
int index = right.getSelectionIndex();
// 获得当前选中选项的索引值
if(index <= 0) // 如未选中,则返回
return ;
String currentValue = right.getItem(index);
// 如果选中了选项值,获得当前选项的值
right.setItem(index, right.getItem(index - 1));
right.setItem(index - 1, currentValue);
// 将选中的选项与上一个选项交换值
right.setSelection(index - 1); // 设定上一选项为选中状态
}
else if(bt.getText().equals("Down")) // 如果是“Down”按钮
{
// 与“Up”按钮逻辑相同
int index = right.getSelectionIndex();
if(index >= right.getItemCount() - 1)
return ;
String currentValue = right.getItem(index);
right.setItem(index, right.getItem(index + 1));
right.setItem(index + 1, currentValue);
right.setSelection(index + 1);
}
}
};
// 定义按钮
// 定义(单)右移按钮
Button bt1 = new Button(shell, SWT.NONE);
bt1.setText(">");
bt1.setBounds(130, 20, 25, 20);
bt1.addSelectionListener(listener); // 为按钮注册事件,其他的按钮类似
// 定义(多)右移按钮
Button bt2 = new Button(shell, SWT.NONE);
bt2.setText(">>");
bt2.setBounds(130, 55, 25, 20);
bt2.addSelectionListener(listener);
// 定义(多)左移按钮
Button bt3 = new Button(shell, SWT.NONE);
bt3.setText("<<");
bt3.setBounds(130, 90, 25, 20);
bt3.addSelectionListener(listener);
// 定义(单)左移按钮
Button bt4 = new Button(shell, SWT.NONE);
bt4.setText("<");
bt4.setBounds(130, 125, 25, 20);
bt4.addSelectionListener(listener);
// 定义上移按钮
Button bt5 = new Button(shell, SWT.NONE);
bt5.setText("Up");
bt5.setBounds(285, 70, 40, 20);
bt5.addSelectionListener(listener);
// 定义下移按钮
Button bt6 = new Button(shell, SWT.NONE);
bt6.setText("Down");
bt6.setBounds(285, 105, 40, 20);
bt6.addSelectionListener(listener);
shell.open();
}
private void verifyValue(String[] selected, List from, List to) {
for(int i = 0; i < selected.length; i++)
{
from.remove(selected[i]); // 从一个列表中移除该选项值
to.add(selected[i]); // 添加到另一列表中
}
}
}
样式常量 | 描述 |
SWT.SINGLE | 只能选中一个选项,为默认样式 |
SWT.MULTI | 可同时选择多个选项的列表框,按住 Ctrl 键即可多选 |
SWT.H_SCROLL | 带有水平滚动条的多行列表框 |
SWT.V_SCROLL | 带有垂直滚动条的多行列表框 |
方法 | 含义 |
add(String string) | 向列表框的末尾添加选项 |
add(String string, int index); setItem(int index, String string) | 向列表指定位置添加选项 |
setItems(String[] items) | 将字符串数组添加到列表框 |
方法 | 含义 |
remove(int index) | 删除指定一个索引出的选项 |
remove(int[] indices) | 删除多个索引的选项 |
remove(int start, int end) | 删除指定开始和结束索引值间的选项 |
remove(String string) | 删除指定字符串的选型 |
removeAll() | 删除列表中所有的选项 |
方法 | 含义 |
String getItem(int index) | 获得指定索引的选项值 |
getItemCount() | 获得选项的总数 |
String[] getItems() | 获得所有的选项值 |
getTopIndex() | 获得第一个选项的索引值 |
indexOf(String string) | 查找是否存在指定的选项,如果存在,测返回所在的索引值 |
indexOf(String string, int start) | 查找指定开始索引后是否存在指定的选项,如存在,则放回索引值 |
方法 | 含义 |
String[] getSelection() | 获取当前所选中的所有选项 |
getSelectionCount() | 获得当前选中的选项数目 |
getSelectionIndex() | 获得当前选中选项的索引值 |
int[] getSelectionIndices | 获得当前选中选项的索引值数组 |
isSelected(int index) | 判断某个索引值的选项是否已被选中:true, false |
select(int index); setSelection(int index) | 设置指定索引值的选项为选中状态 |
select(int[] indices); setSelection(int[] indices) | 设置指定索引值数组的选项为选中状态 |
select(int start, int end); setSelection(int start, int end) | 设置开始索引到结束索引的选项为选中状态 |
selectAll() | 全选 |
setSelection(String[] items) | 设置指定字符串数组中值为选中状态 |
showSelection() | 显示选中的方法 |
deselect(int index) | 反选指定索引的选项 |
deselect(int[] indices) | 反选指定索引数组的选项 |
deselect(int start, int end) | 反选开始索引到结束索引的选项 |
deselectAll() | 全部反选 |