仿腾讯头部切换按钮优化版
2013-09-13 16:27
274 查看
仿QQ头部banner切换按钮组件
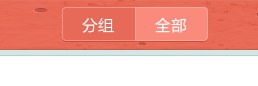
用到的资源文件:
app_top_banner_layout_bg2.png //背景

bbs_posts_switch_bg_night.png //夜晚模式

bbs_posts_switch_bg.png //

bbs_posts_switch_content_bg_night.png
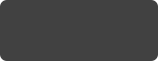
bbs_posts_switch_content_bg.png
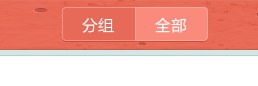
public class SwitchView extends LinearLayout implements OnClickListener { private static final int FLAG_MOVE_TRUE = 1; // 向左滑动标识 private static final int FLAG_MOVE_FALSE = 2; // 向右滑动标识 private static final int HANDLE_LAYOUT_CURSOR = 100; // 处理调用开关的layout方法 private Context context; // 上下文对象 private RelativeLayout sv_container; // SwitchView的外层Layout private ImageView iv_switch_cursor; // 开关邮标的ImageView private TextView switch_text_true; // true的文字信息控件 private TextView switch_text_false; // false的文字信息控件 private boolean isChecked = true; // 是否已开 private boolean checkedChange = false; // isChecked是否有改变 private OnCheckedChangeListener onCheckedChangeListener; // 用于监听isChecked是否有改变 private boolean isNight = false; private int margin = 1; // 游标离边缘位置(这个值视图片而定, 主要是为了图片能显示正确) private int bg_left; // 背景左 private int bg_right; // 背景右 private int cursor_left; // 游标左部 private int cursor_top; // 游标顶部 private int cursor_right; // 游标右部 private int cursor_bottom; // 游标底部 private Animation animation; // 移动动画 private int currentFlag = FLAG_MOVE_TRUE; // 当前移动方向flag private RelativeLayout relativeLayout; public SwitchView(Context context) { super(context); this.context = context; initView(); } @TargetApi(Build.VERSION_CODES.HONEYCOMB) public SwitchView(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); // TODO Auto-generated constructor stub this.context = context; initView(); } public SwitchView(Context context, AttributeSet attrs) { super(context, attrs); // TODO Auto-generated constructor stub this.context = context; initView(); } @Override protected void onLayout(boolean changed, int l, int t, int r, int b) { super.onLayout(changed, l, t, r, b); // 获取所需要的值 bg_left = sv_container.getLeft(); bg_right = sv_container.getRight(); cursor_left = iv_switch_cursor.getLeft(); cursor_top = iv_switch_cursor.getTop(); cursor_right = iv_switch_cursor.getRight(); cursor_bottom = iv_switch_cursor.getBottom(); } private Handler mHandler = new Handler() { @Override public void handleMessage(Message msg) { switch(msg.what) { case HANDLE_LAYOUT_CURSOR: iv_switch_cursor.layout(cursor_left, cursor_top, cursor_right, cursor_bottom); break; } } }; public void onClick(View v) { // 控件点击时触发改变checked值 if(v == this) { changeChecked(!isChecked); } } /** * 初始化控件 */ private void initView() { LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE); View view = inflater.inflate(R.layout.switchview_layout, this); view.setOnClickListener(this); sv_container = (RelativeLayout) view.findViewById(R.id.switch_layout); switch_text_true = (TextView) view.findViewById(R.id.switch_text_true); switch_text_false = (TextView) view.findViewById(R.id.switch_text_false); changeTextColor(); iv_switch_cursor = (ImageView) view.findViewById(R.id.iv_switch_cursor); iv_switch_cursor.setClickable(false); iv_switch_cursor.setOnTouchListener(new OnTouchListener() { int lastX; // 最后的X坐标 public boolean onTouch(View v, MotionEvent event) { switch(event.getAction()) { case MotionEvent.ACTION_DOWN: lastX = (int) event.getRawX(); cursor_left = v.getLeft(); cursor_top = v.getTop(); cursor_right = v.getRight(); cursor_bottom = v.getBottom(); break; case MotionEvent.ACTION_MOVE: int dx = (int) event.getRawX() - lastX; cursor_left = v.getLeft() + dx; cursor_right = v.getRight() + dx; // 超出边界处理 if(cursor_left <= bg_left + margin) { cursor_left = bg_left + margin; cursor_right = cursor_left + v.getWidth(); } if(cursor_right >= bg_right - margin) { cursor_right = bg_right - margin; cursor_left = cursor_right - v.getWidth(); } v.layout(cursor_left, cursor_top, cursor_right, cursor_bottom); lastX = (int) event.getRawX(); break; case MotionEvent.ACTION_UP: calculateIscheck(); break; } return true; } }); } /** * 计算处于true或是false区域, 并做改变处理 */ private void calculateIscheck() { float center = (float) ((bg_right - bg_left) / 2.0); float cursor_center = (float) ((cursor_right - cursor_left) / 2.0); if(cursor_left + cursor_center <= center) { changeChecked(true); } else { changeChecked(false); } } /** * 改变checked, 根据checked移动游标 * @param isChecked */ private void changeChecked(boolean isChecked) { if(this.isChecked != isChecked) { checkedChange = true; } else { checkedChange = false; } if(isChecked) { currentFlag = FLAG_MOVE_TRUE; } else { currentFlag = FLAG_MOVE_FALSE; } cursorMove(); } /** * 游标移动 */ private void cursorMove() { // 这里说明一点, 动画本可设置animation.setFillAfter(true) // 令动画进行完后停在最后位置. 但这里使用这样方式的话. // 再次拖动图片会出现异常(具体原因我没找到) // 所以最后只能使用onAnimationEnd回调方式再layout游标 animation = null; final int toX; if(currentFlag == FLAG_MOVE_TRUE) { toX = cursor_left - bg_left - margin; animation = new TranslateAnimation(0, -toX, 0, 0); } else { toX = bg_right - margin - cursor_right; animation = new TranslateAnimation(0, toX, 0, 0); } animation.setDuration(100); animation.setInterpolator(new LinearInterpolator()); animation.setAnimationListener(new AnimationListener() { public void onAnimationStart(Animation animation) { } public void onAnimationRepeat(Animation animation) { } public void onAnimationEnd(Animation animation) { // 计算动画完成后游标应在的位置 if(currentFlag == FLAG_MOVE_TRUE) { cursor_left -= toX; cursor_right = cursor_left + iv_switch_cursor.getWidth(); } else { cursor_right = bg_right - margin; cursor_left = cursor_right - iv_switch_cursor.getWidth(); } // 这里不能马上layout游标正确位置, 否则会有一点点闪屏 // 为了美观, 这里迟了一点点调用layout方法, 便不会闪屏 mHandler.sendEmptyMessageDelayed(HANDLE_LAYOUT_CURSOR, 5); // 这里是根据是不是改变了isChecked值进行一些操作 if(checkedChange) { isChecked = !isChecked; if(onCheckedChangeListener != null) { onCheckedChangeListener.onCheckedChanged(isChecked); } changeTextColor(); } } }); iv_switch_cursor.startAnimation(animation); } /** * 改变字体显示颜色 */ private void changeTextColor() { if(isChecked) { if(isNight){ switch_text_true.setTextColor(Color.parseColor("#A8A8A8")); switch_text_false.setTextColor(Color.parseColor("#535353")); }else{ switch_text_true.setTextColor(Color.BLACK); switch_text_false.setTextColor(Color.GRAY); } } else { if(isNight){ switch_text_true.setTextColor(Color.parseColor("#535353")); switch_text_false.setTextColor(Color.parseColor("#A8A8A8")); }else{ switch_text_true.setTextColor(Color.GRAY); switch_text_false.setTextColor(Color.BLACK); } } } /** * layout游标 */ private void layoutCursor() { if(isChecked) { cursor_left = bg_left + margin; cursor_right = bg_left + margin + iv_switch_cursor.getWidth(); } else { cursor_left = bg_right - margin - iv_switch_cursor.getWidth(); cursor_right = bg_right - margin; } iv_switch_cursor.layout(cursor_left, cursor_top, cursor_right, cursor_bottom); changeTextColor(); } /** * isChecked值改变监听器 */ public interface OnCheckedChangeListener { void onCheckedChanged(boolean isChecked); } public boolean isChecked() { return isChecked; } public void setChecked(boolean isChecked) { if(this.isChecked != isChecked) { this.isChecked = isChecked; if(onCheckedChangeListener != null) { onCheckedChangeListener.onCheckedChanged(isChecked); } layoutCursor(); } } public void setOnCheckedChangeListener( OnCheckedChangeListener onCheckedChangeListener) { this.onCheckedChangeListener = onCheckedChangeListener; } public void changeMode(boolean isNight){ this.isNight = isNight; if(isNight){ sv_container.setBackgroundResource(R.drawable.bbs_posts_switch_bg_night); iv_switch_cursor.setBackgroundResource(R.drawable.bbs_posts_switch_content_bg_night); }else{ sv_container.setBackgroundResource(R.drawable.bbs_posts_switch_bg); iv_switch_cursor.setBackgroundResource(R.drawable.bbs_posts_switch_content_bg); } changeTextColor(); } }
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/switch_layout" android:layout_width="170dp" android:layout_height="wrap_content" android:layout_gravity="center" android:background="@drawable/bbs_posts_switch_bg"> <ImageView android:id="@+id/iv_switch_cursor" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:background="@drawable/bbs_posts_switch_content_bg" android:contentDescription="@null"/> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:layout_centerVertical="true"> <TextView android:id="@+id/switch_text_true" android:layout_width="85dp" android:layout_height="wrap_content" android:textSize="14sp" android:gravity="center" android:textColor="#ff000000" android:text="分组" /> <TextView android:id="@+id/switch_text_false" android:layout_width="85dp" android:layout_height="wrap_content" android:textSize="14sp" android:gravity="center" android:textColor="#ff000000" android:text="全部" /> </LinearLayout> </RelativeLayout>以上是布局文件。
用到的资源文件:
app_top_banner_layout_bg2.png //背景

bbs_posts_switch_bg_night.png //夜晚模式

bbs_posts_switch_bg.png //

bbs_posts_switch_content_bg_night.png
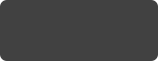
bbs_posts_switch_content_bg.png

相关文章推荐
- JS+CSS带数字和左右按钮可控制切换的图片幻灯
- 仿腾讯图片切换
- 手机影音第三天,通过监听底部radiogroup按钮状态来切换中间FragmentLayout里的内容
- 切换按钮图片
- iOS 去掉导航左侧按钮系统默认的文字 只留 默认箭头 (而且解决了 切换应用是 闪桌面的问题)
- ecshop优化5-多语言切换中英文切换
- 激活对话框中tab键切换选项实现蓝色按钮切换
- 标题栏按钮滑动或点击切换fragment
- 一次关于切换场景的加载速度的优化的报告文档。
- 自己整理的腾讯网站的新闻导航【鼠标经过就切换分类】
- UIBarButtonItem 上按钮切换/隐藏的简单例子
- ext4 学习笔记(第一天:按钮的切换事件)
- jquery图片滚动带左右按钮控制焦点图片切换滚动
- freemarker文件下,bootstrap 点击按钮,切换按钮上的图标
- cocos2d-x中按钮点击后切换图片功能
- jquery 点击按钮切换样式on方法使用
- DGallery比较流行带按钮图片切换效果
- 做一个计时器示例 在页面上有一个文本框和一个普通按钮 在状态栏上显示,单击按钮,开始计时的提示信息 单击按钮时,在文本框显示计数的过程,同时要求按钮上的字,显示为“单击暂停” 此时单击按钮,暂停计时,同时按钮上的字显示为“继续计时”,要求这两种状态来回切换,来完成计数器的功能
- Vue.js点击切换按钮改变内容的实例讲解
- 做tab切换DIV时防止点击时回到头部的方法