jdbc 链接方式 DBUtil获得链接的三种写法
2013-08-13 11:37
344 查看
普通项目(非javaweb项目),项目文件路径如图
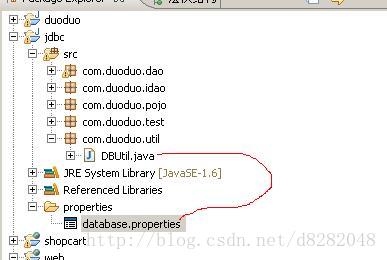
database.properties
driver=oracle.jdbc.driver.OracleDriver
username=neu
password=oracle
url=jdbc\:oracle\:thin\:@127.0.0.1\:1521\:ORACLE
DBUtil
package com.duoduo.util;
import java.io.FileInputStream;
import java.io.IOException;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Logger;
public class DBUtil {
private static Connection connection;
private static PreparedStatement pstmt;
private static ResultSet resultSet;
private static String password;
private static String username;
private static String driver;
private static String url;
static {
try {
init();
register();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
/***
* 注册驱动 *
**/
private static void register() throws ClassNotFoundException {
Class.forName(driver);
}
/***
* 初始化配置 *
**/
private static void init() throws IOException {
Properties properties = new Properties();
FileInputStream in = new FileInputStream(
"properties/database.properties");
properties.load(in);
in.close();
driver = properties.getProperty("driver");
username = properties.getProperty("username");
password = properties.getProperty("password");
url = properties.getProperty("url");
Logger logger = Logger.getLogger("com.duoduo.jdbc");
logger.info(driver);
logger.info(username);
logger.info(password);
logger.info(url);
}
/***
* 获得连接 *
**/
public static Connection getConnection2() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
return connection;
}
/***
* 获得连接 *
**/
public static void getConnection() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
// return connection;
}
/***
* 开始事务 *
**/
public static void startTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.setAutoCommit(false);
}
}
public static void startTrans() throws SQLException {
if (connection != null) {
connection.setAutoCommit(false);
}
}
/***
* 结束事务 *
*
* @throws SQLException
**/
public static void endTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.commit();
}
}
public static void endTrans() throws SQLException {
if (connection != null) {
connection.commit();
}
}
/***
* 回滚事务 *
*
* @throws SQLException
**/
public static void rollbackTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.rollback();
}
}
public static void rollbackTrans() throws SQLException {
if (connection != null) {
connection.rollback();
}
}
/***
* 清空资源 *
*
* @throws SQLException
**/
public static void clear(Connection conn) throws SQLException {
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps)
throws SQLException {
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps, ResultSet rs)
throws SQLException {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
public static void clear() throws SQLException {
if (resultSet != null) {
resultSet.close();
resultSet = null;
}
if (pstmt != null) {
pstmt.close();
pstmt = null;
}
if (connection != null) {
connection.close();
connection = null;
}
}
private static void setParamsToPreparedStatement(PreparedStatement pstmt,
List<Object> params) throws SQLException {
int index = 1;
if (params != null && !params.isEmpty()) {
for (int i = 0; i < params.size(); i++) {
pstmt.setObject(index++, params.get(i));
}
}
}
public static Map<String, Object> findSimpleResult(String sql,
List<Object> params) throws SQLException {
Map<String, Object> map = new HashMap<String, Object>();
// index:占位符地址
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
}
return map;
}
public static List<Map<String, Object>> findMoreResultSet(String sql,
List<Object> params) throws SQLException {
List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
Map<String, Object> map = new HashMap<String, Object>();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
list.add(map);
}
return list;
}
private static <T> void ReflectSetFieldValue(Object target, String column,
Object value, Class<T> cls) throws Exception {
Field field = cls.getDeclaredField(column);
field.setAccessible(true);
String valueString = "";
if (value != null) {
valueString = value.toString();
}
field.set(target, valueString);
}
// 反射的方式封装
public static <T> T findSimpleRefResult(String sql, List<Object> params,
Class<T> cls) throws Exception {
T resultObject = null;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
}
return resultObject;
}
public static <T> List<T> findMoreRefResultSet(String sql,
List<Object> params, Class<T> cls) throws Exception {
List<T> list = new ArrayList<T>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
int N = 0;
while (resultSet.next()) {
N++;
T resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
list.add(resultObject);
}
Logger logger = Logger.getLogger("com.duoduo.util");
logger.info(N + "");
return list;
}
public static boolean updateByPreparedStatement(String sql,
List<Object> params) throws SQLException {
boolean flag = false;
// result:当用户执行添加删除修改时所影响数据库的行数
int result = -1;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
result = pstmt.executeUpdate();
flag = result > 0 ? true : false;
return flag;
}
}
javaweb项目方式1
和普通项目差不多,properties文件路径更改了,init方法中获得InputStream的方式也改了
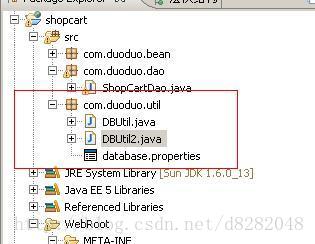
package com.duoduo.util;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Logger;
public class DBUtil2 {
private static Connection connection;
private static PreparedStatement pstmt;
private static ResultSet resultSet;
private static String password;
private static String username;
private static String driver;
private static String url;
static {
try {
init();
register();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
/***
* 注册驱动 *
**/
private static void register() throws ClassNotFoundException {
Class.forName(driver);
}
/***
* 初始化配置 *
**/
private static void init() throws IOException {
Logger logger = Logger.getLogger("com.duoduo");
InputStream in = DBUtil2.class
.getResourceAsStream("database.properties");
Properties properties = new Properties();
properties.load(in);
in.close();
driver = properties.getProperty("driver");
username = properties.getProperty("username");
password = properties.getProperty("password");
url = properties.getProperty("url");
logger.info(driver);
logger.info(username);
logger.info(password);
logger.info(url);
}
/***
* 获得连接 *
**/
public static Connection getConnection2() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
return connection;
}
/***
* 获得连接 *
**/
public static void getConnection() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
// return connection;
}
/***
* 开始事务 *
**/
public static void startTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.setAutoCommit(false);
}
}
public static void startTrans() throws SQLException {
if (connection != null) {
connection.setAutoCommit(false);
}
}
/***
* 结束事务 *
*
* @throws SQLException
**/
public static void endTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.commit();
}
}
public static void endTrans() throws SQLException {
if (connection != null) {
connection.commit();
}
}
/***
* 回滚事务 *
*
* @throws SQLException
**/
public static void rollbackTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.rollback();
}
}
public static void rollbackTrans() throws SQLException {
if (connection != null) {
connection.rollback();
}
}
/***
* 清空资源 *
*
* @throws SQLException
**/
public static void clear(Connection conn) throws SQLException {
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps)
throws SQLException {
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps, ResultSet rs)
throws SQLException {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
public static void clear() throws SQLException {
if (resultSet != null) {
resultSet.close();
resultSet = null;
}
if (pstmt != null) {
pstmt.close();
pstmt = null;
}
if (connection != null) {
connection.close();
connection = null;
}
}
private static void setParamsToPreparedStatement(PreparedStatement pstmt,
List<Object> params) throws SQLException {
int index = 1;
if (params != null && !params.isEmpty()) {
for (int i = 0; i < params.size(); i++) {
pstmt.setObject(index++, params.get(i));
}
}
}
public static Map<String, Object> findSimpleResult(String sql,
List<Object> params) throws SQLException {
Map<String, Object> map = new HashMap<String, Object>();
// index:占位符地址
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
}
return map;
}
public static List<Map<String, Object>> findMoreResultSet(String sql,
List<Object> params) throws SQLException {
List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
Map<String, Object> map = new HashMap<String, Object>();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
list.add(map);
}
return list;
}
private static <T> void ReflectSetFieldValue(Object target, String column,
Object value, Class<T> cls) throws Exception {
Field field = cls.getDeclaredField(column);
field.setAccessible(true);
String valueString = "";
if (value != null) {
valueString = value.toString();
}
field.set(target, valueString);
}
// 反射的方式封装
public static <T> T findSimpleRefResult(String sql, List<Object> params,
Class<T> cls) throws Exception {
T resultObject = null;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
}
return resultObject;
}
public static <T> List<T> findMoreRefResultSet(String sql,
List<Object> params, Class<T> cls) throws Exception {
List<T> list = new ArrayList<T>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
int N = 0;
while (resultSet.next()) {
N++;
T resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
list.add(resultObject);
}
Logger logger = Logger.getLogger("com.duoduo.util");
logger.info(N + "");
return list;
}
public static boolean updateByPreparedStatement(String sql,
List<Object> params) throws SQLException {
boolean flag = false;
// result:当用户执行添加删除修改时所影响数据库的行数
int result = -1;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
result = pstmt.executeUpdate();
flag = result > 0 ? true : false;
return flag;
}
}
javaweb项目方式2
使用tomcat的数据库连接池,因此不使用properties配置文件,而在META-INF文件夹下添加context.xml,DBUtil中的一些东西也变了,例如没有了register方法,
不要忘记在tomcat的lib文件夹里把driver的jar包考进去。
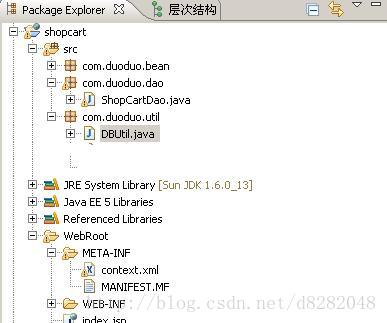
context.xml
<?xml version="1.0" encoding="UTF-8"?>
<Context>
<Resource name="jdbc/ums" type="javax.sql.DataSource"
username="neu" password="oracle" url="jdbc:oracle:thin:@127.0.0.1:1521:ORACLE"
driverClassName="oracle.jdbc.driver.OracleDriver" maxIdle="2" maxWait="5000"
maxActive="4" />
<WatchedResource>WEB-INF/web.xml</WatchedResource>
</Context>
package com.duoduo.util;
import java.io.IOException;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
public class DBUtil {
private static Connection connection;
private static PreparedStatement pstmt;
private static ResultSet resultSet;
private static DataSource ds;
static {
try {
init();
} catch (IOException e) {
e.printStackTrace();
} catch (NamingException e) {
e.printStackTrace();
}
}
/***
* 初始化配置
*
* @throws NamingException
* *
**/
private static void init() throws IOException, NamingException {
Context context = new InitialContext();
ds = (DataSource) context.lookup("java:comp/env/jdbc/ums");
}
/***
* 获得连接 *
**/
public static Connection getConnection2() throws SQLException {
// 确保资源已经释放
clear();
connection = ds.getConnection();
return connection;
}
/***
* 获得连接 *
**/
public static void getConnection() throws SQLException {
// 确保资源已经释放
clear();
connection = ds.getConnection();
// return connection;
}
/***
* 开始事务 *
**/
public static void startTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.setAutoCommit(false);
}
}
public static void startTrans() throws SQLException {
if (connection != null) {
connection.setAutoCommit(false);
}
}
/***
* 结束事务 *
*
* @throws SQLException
**/
public static void endTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.commit();
}
}
public static void endTrans() throws SQLException {
if (connection != null) {
connection.commit();
}
}
/***
* 回滚事务 *
*
* @throws SQLException
**/
public static void rollbackTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.rollback();
}
}
public static void rollbackTrans() throws SQLException {
if (connection != null) {
connection.rollback();
}
}
/***
* 清空资源 *
*
* @throws SQLException
**/
public static void clear(Connection conn) throws SQLException {
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps)
throws SQLException {
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps, ResultSet rs)
throws SQLException {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
public static void clear() throws SQLException {
if (resultSet != null) {
resultSet.close();
resultSet = null;
}
if (pstmt != null) {
pstmt.close();
pstmt = null;
}
if (connection != null) {
connection.close();
connection = null;
}
}
private static void setParamsToPreparedStatement(PreparedStatement pstmt,
List<Object> params) throws SQLException {
int index = 1;
if (params != null && !params.isEmpty()) {
for (int i = 0; i < params.size(); i++) {
pstmt.setObject(index++, params.get(i));
}
}
}
public static Map<String, Object> findSimpleResult(String sql,
List<Object> params) throws SQLException {
Map<String, Object> map = new HashMap<String, Object>();
// index:占位符地址
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
}
return map;
}
public static List<Map<String, Object>> findMoreResultSet(String sql,
List<Object> params) throws SQLException {
List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
Map<String, Object> map = new HashMap<String, Object>();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
list.add(map);
}
return list;
}
private static <T> void ReflectSetFieldValue(Object target, String column,
Object value, Class<T> cls) throws Exception {
Field field = cls.getDeclaredField(column);
field.setAccessible(true);
String valueString = "";
if (value != null) {
valueString = value.toString();
}
field.set(target, valueString);
}
// 反射的方式封装
public static <T> T findSimpleRefResult(String sql, List<Object> params,
Class<T> cls) throws Exception {
T resultObject = null;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
}
return resultObject;
}
public static <T> List<T> findMoreRefResultSet(String sql,
List<Object> params, Clas
9aa4
s<T> cls) throws Exception {
List<T> list = new ArrayList<T>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
int N = 0;
while (resultSet.next()) {
N++;
T resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
list.add(resultObject);
}
Logger logger = Logger.getLogger("com.duoduo.util");
logger.info(N + "");
return list;
}
public static boolean updateByPreparedStatement(String sql,
List<Object> params) throws SQLException {
boolean flag = false;
// result:当用户执行添加删除修改时所影响数据库的行数
int result = -1;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
result = pstmt.executeUpdate();
flag = result > 0 ? true : false;
return flag;
}
}
database.properties
driver=oracle.jdbc.driver.OracleDriver
username=neu
password=oracle
url=jdbc\:oracle\:thin\:@127.0.0.1\:1521\:ORACLE
DBUtil
package com.duoduo.util;
import java.io.FileInputStream;
import java.io.IOException;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Logger;
public class DBUtil {
private static Connection connection;
private static PreparedStatement pstmt;
private static ResultSet resultSet;
private static String password;
private static String username;
private static String driver;
private static String url;
static {
try {
init();
register();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
/***
* 注册驱动 *
**/
private static void register() throws ClassNotFoundException {
Class.forName(driver);
}
/***
* 初始化配置 *
**/
private static void init() throws IOException {
Properties properties = new Properties();
FileInputStream in = new FileInputStream(
"properties/database.properties");
properties.load(in);
in.close();
driver = properties.getProperty("driver");
username = properties.getProperty("username");
password = properties.getProperty("password");
url = properties.getProperty("url");
Logger logger = Logger.getLogger("com.duoduo.jdbc");
logger.info(driver);
logger.info(username);
logger.info(password);
logger.info(url);
}
/***
* 获得连接 *
**/
public static Connection getConnection2() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
return connection;
}
/***
* 获得连接 *
**/
public static void getConnection() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
// return connection;
}
/***
* 开始事务 *
**/
public static void startTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.setAutoCommit(false);
}
}
public static void startTrans() throws SQLException {
if (connection != null) {
connection.setAutoCommit(false);
}
}
/***
* 结束事务 *
*
* @throws SQLException
**/
public static void endTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.commit();
}
}
public static void endTrans() throws SQLException {
if (connection != null) {
connection.commit();
}
}
/***
* 回滚事务 *
*
* @throws SQLException
**/
public static void rollbackTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.rollback();
}
}
public static void rollbackTrans() throws SQLException {
if (connection != null) {
connection.rollback();
}
}
/***
* 清空资源 *
*
* @throws SQLException
**/
public static void clear(Connection conn) throws SQLException {
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps)
throws SQLException {
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps, ResultSet rs)
throws SQLException {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
public static void clear() throws SQLException {
if (resultSet != null) {
resultSet.close();
resultSet = null;
}
if (pstmt != null) {
pstmt.close();
pstmt = null;
}
if (connection != null) {
connection.close();
connection = null;
}
}
private static void setParamsToPreparedStatement(PreparedStatement pstmt,
List<Object> params) throws SQLException {
int index = 1;
if (params != null && !params.isEmpty()) {
for (int i = 0; i < params.size(); i++) {
pstmt.setObject(index++, params.get(i));
}
}
}
public static Map<String, Object> findSimpleResult(String sql,
List<Object> params) throws SQLException {
Map<String, Object> map = new HashMap<String, Object>();
// index:占位符地址
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
}
return map;
}
public static List<Map<String, Object>> findMoreResultSet(String sql,
List<Object> params) throws SQLException {
List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
Map<String, Object> map = new HashMap<String, Object>();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
list.add(map);
}
return list;
}
private static <T> void ReflectSetFieldValue(Object target, String column,
Object value, Class<T> cls) throws Exception {
Field field = cls.getDeclaredField(column);
field.setAccessible(true);
String valueString = "";
if (value != null) {
valueString = value.toString();
}
field.set(target, valueString);
}
// 反射的方式封装
public static <T> T findSimpleRefResult(String sql, List<Object> params,
Class<T> cls) throws Exception {
T resultObject = null;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
}
return resultObject;
}
public static <T> List<T> findMoreRefResultSet(String sql,
List<Object> params, Class<T> cls) throws Exception {
List<T> list = new ArrayList<T>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
int N = 0;
while (resultSet.next()) {
N++;
T resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
list.add(resultObject);
}
Logger logger = Logger.getLogger("com.duoduo.util");
logger.info(N + "");
return list;
}
public static boolean updateByPreparedStatement(String sql,
List<Object> params) throws SQLException {
boolean flag = false;
// result:当用户执行添加删除修改时所影响数据库的行数
int result = -1;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
result = pstmt.executeUpdate();
flag = result > 0 ? true : false;
return flag;
}
}
javaweb项目方式1
和普通项目差不多,properties文件路径更改了,init方法中获得InputStream的方式也改了
package com.duoduo.util;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Logger;
public class DBUtil2 {
private static Connection connection;
private static PreparedStatement pstmt;
private static ResultSet resultSet;
private static String password;
private static String username;
private static String driver;
private static String url;
static {
try {
init();
register();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
/***
* 注册驱动 *
**/
private static void register() throws ClassNotFoundException {
Class.forName(driver);
}
/***
* 初始化配置 *
**/
private static void init() throws IOException {
Logger logger = Logger.getLogger("com.duoduo");
InputStream in = DBUtil2.class
.getResourceAsStream("database.properties");
Properties properties = new Properties();
properties.load(in);
in.close();
driver = properties.getProperty("driver");
username = properties.getProperty("username");
password = properties.getProperty("password");
url = properties.getProperty("url");
logger.info(driver);
logger.info(username);
logger.info(password);
logger.info(url);
}
/***
* 获得连接 *
**/
public static Connection getConnection2() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
return connection;
}
/***
* 获得连接 *
**/
public static void getConnection() throws SQLException {
// 确保资源已经释放
clear();
connection = DriverManager.getConnection(url, username, password);
// return connection;
}
/***
* 开始事务 *
**/
public static void startTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.setAutoCommit(false);
}
}
public static void startTrans() throws SQLException {
if (connection != null) {
connection.setAutoCommit(false);
}
}
/***
* 结束事务 *
*
* @throws SQLException
**/
public static void endTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.commit();
}
}
public static void endTrans() throws SQLException {
if (connection != null) {
connection.commit();
}
}
/***
* 回滚事务 *
*
* @throws SQLException
**/
public static void rollbackTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.rollback();
}
}
public static void rollbackTrans() throws SQLException {
if (connection != null) {
connection.rollback();
}
}
/***
* 清空资源 *
*
* @throws SQLException
**/
public static void clear(Connection conn) throws SQLException {
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps)
throws SQLException {
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps, ResultSet rs)
throws SQLException {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
public static void clear() throws SQLException {
if (resultSet != null) {
resultSet.close();
resultSet = null;
}
if (pstmt != null) {
pstmt.close();
pstmt = null;
}
if (connection != null) {
connection.close();
connection = null;
}
}
private static void setParamsToPreparedStatement(PreparedStatement pstmt,
List<Object> params) throws SQLException {
int index = 1;
if (params != null && !params.isEmpty()) {
for (int i = 0; i < params.size(); i++) {
pstmt.setObject(index++, params.get(i));
}
}
}
public static Map<String, Object> findSimpleResult(String sql,
List<Object> params) throws SQLException {
Map<String, Object> map = new HashMap<String, Object>();
// index:占位符地址
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
}
return map;
}
public static List<Map<String, Object>> findMoreResultSet(String sql,
List<Object> params) throws SQLException {
List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
Map<String, Object> map = new HashMap<String, Object>();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
list.add(map);
}
return list;
}
private static <T> void ReflectSetFieldValue(Object target, String column,
Object value, Class<T> cls) throws Exception {
Field field = cls.getDeclaredField(column);
field.setAccessible(true);
String valueString = "";
if (value != null) {
valueString = value.toString();
}
field.set(target, valueString);
}
// 反射的方式封装
public static <T> T findSimpleRefResult(String sql, List<Object> params,
Class<T> cls) throws Exception {
T resultObject = null;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
}
return resultObject;
}
public static <T> List<T> findMoreRefResultSet(String sql,
List<Object> params, Class<T> cls) throws Exception {
List<T> list = new ArrayList<T>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
int N = 0;
while (resultSet.next()) {
N++;
T resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
list.add(resultObject);
}
Logger logger = Logger.getLogger("com.duoduo.util");
logger.info(N + "");
return list;
}
public static boolean updateByPreparedStatement(String sql,
List<Object> params) throws SQLException {
boolean flag = false;
// result:当用户执行添加删除修改时所影响数据库的行数
int result = -1;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
result = pstmt.executeUpdate();
flag = result > 0 ? true : false;
return flag;
}
}
javaweb项目方式2
使用tomcat的数据库连接池,因此不使用properties配置文件,而在META-INF文件夹下添加context.xml,DBUtil中的一些东西也变了,例如没有了register方法,
不要忘记在tomcat的lib文件夹里把driver的jar包考进去。
context.xml
<?xml version="1.0" encoding="UTF-8"?>
<Context>
<Resource name="jdbc/ums" type="javax.sql.DataSource"
username="neu" password="oracle" url="jdbc:oracle:thin:@127.0.0.1:1521:ORACLE"
driverClassName="oracle.jdbc.driver.OracleDriver" maxIdle="2" maxWait="5000"
maxActive="4" />
<WatchedResource>WEB-INF/web.xml</WatchedResource>
</Context>
package com.duoduo.util;
import java.io.IOException;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
public class DBUtil {
private static Connection connection;
private static PreparedStatement pstmt;
private static ResultSet resultSet;
private static DataSource ds;
static {
try {
init();
} catch (IOException e) {
e.printStackTrace();
} catch (NamingException e) {
e.printStackTrace();
}
}
/***
* 初始化配置
*
* @throws NamingException
* *
**/
private static void init() throws IOException, NamingException {
Context context = new InitialContext();
ds = (DataSource) context.lookup("java:comp/env/jdbc/ums");
}
/***
* 获得连接 *
**/
public static Connection getConnection2() throws SQLException {
// 确保资源已经释放
clear();
connection = ds.getConnection();
return connection;
}
/***
* 获得连接 *
**/
public static void getConnection() throws SQLException {
// 确保资源已经释放
clear();
connection = ds.getConnection();
// return connection;
}
/***
* 开始事务 *
**/
public static void startTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.setAutoCommit(false);
}
}
public static void startTrans() throws SQLException {
if (connection != null) {
connection.setAutoCommit(false);
}
}
/***
* 结束事务 *
*
* @throws SQLException
**/
public static void endTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.commit();
}
}
public static void endTrans() throws SQLException {
if (connection != null) {
connection.commit();
}
}
/***
* 回滚事务 *
*
* @throws SQLException
**/
public static void rollbackTrans(Connection conn) throws SQLException {
if (conn != null) {
conn.rollback();
}
}
public static void rollbackTrans() throws SQLException {
if (connection != null) {
connection.rollback();
}
}
/***
* 清空资源 *
*
* @throws SQLException
**/
public static void clear(Connection conn) throws SQLException {
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps)
throws SQLException {
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
/***
* 清空资源 *
**/
public static void clear(Connection conn, PreparedStatement ps, ResultSet rs)
throws SQLException {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
public static void clear() throws SQLException {
if (resultSet != null) {
resultSet.close();
resultSet = null;
}
if (pstmt != null) {
pstmt.close();
pstmt = null;
}
if (connection != null) {
connection.close();
connection = null;
}
}
private static void setParamsToPreparedStatement(PreparedStatement pstmt,
List<Object> params) throws SQLException {
int index = 1;
if (params != null && !params.isEmpty()) {
for (int i = 0; i < params.size(); i++) {
pstmt.setObject(index++, params.get(i));
}
}
}
public static Map<String, Object> findSimpleResult(String sql,
List<Object> params) throws SQLException {
Map<String, Object> map = new HashMap<String, Object>();
// index:占位符地址
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
}
return map;
}
public static List<Map<String, Object>> findMoreResultSet(String sql,
List<Object> params) throws SQLException {
List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
Map<String, Object> map = new HashMap<String, Object>();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
map.put(cols_name, cols_value);
}
list.add(map);
}
return list;
}
private static <T> void ReflectSetFieldValue(Object target, String column,
Object value, Class<T> cls) throws Exception {
Field field = cls.getDeclaredField(column);
field.setAccessible(true);
String valueString = "";
if (value != null) {
valueString = value.toString();
}
field.set(target, valueString);
}
// 反射的方式封装
public static <T> T findSimpleRefResult(String sql, List<Object> params,
Class<T> cls) throws Exception {
T resultObject = null;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
while (resultSet.next()) {
resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
}
return resultObject;
}
public static <T> List<T> findMoreRefResultSet(String sql,
List<Object> params, Clas
9aa4
s<T> cls) throws Exception {
List<T> list = new ArrayList<T>();
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
resultSet = pstmt.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int col_len = metaData.getColumnCount();
int N = 0;
while (resultSet.next()) {
N++;
T resultObject = cls.newInstance();
for (int i = 0; i < col_len; i++) {
String cols_name = metaData.getColumnName(i + 1);
Object cols_value = resultSet.getObject(cols_name);
if (cols_value == null) {
cols_value = "";
}
ReflectSetFieldValue(resultObject, cols_name.toLowerCase(),
cols_value, cls);
}
list.add(resultObject);
}
Logger logger = Logger.getLogger("com.duoduo.util");
logger.info(N + "");
return list;
}
public static boolean updateByPreparedStatement(String sql,
List<Object> params) throws SQLException {
boolean flag = false;
// result:当用户执行添加删除修改时所影响数据库的行数
int result = -1;
pstmt = connection.prepareStatement(sql);
setParamsToPreparedStatement(pstmt, params);
result = pstmt.executeUpdate();
flag = result > 0 ? true : false;
return flag;
}
}
相关文章推荐
- java jdbc/ojdbc 链接oracle的三种方式
- CAS提供的三种JDBC认证方式配置文件写法
- jdbc mysql数据库链接 及 三种不同的注册驱动的方式
- JDBC 链接oracle的三种URL写法
- JDBC 链接oracle的三种URL写法
- JDBC 链接oracle的三种URL写法
- 大数据(二十三)Hive【Hive三种启动方式 、 HIVE Server2详解 、 jdbc链接HIVE】
- jdbc链接数据库的三种方式
- JDBC 链接oracle的三种URL写法
- a链接标记的三种方式使用我的个人理解
- JDBC注册驱动程序三种方式
- Android中获得Message对象三种方式的去呗
- Android获得图片资源的三种方式
- 注册JDBC驱动的三种方式
- JDBC注册驱动程序三种方式
- 获得xml解析器LayoutInflater 实例的三种方式
- 注册jdbc驱动程序的三种方式
- hive 三种启动方式及用途,关注通过jdbc连接的启动
- 注册jdbc驱动程序的三种方式
- 获得 LayoutInflater 实例的三种方式