Google笔试题:哈希表的一个意想不到的应用--在字符串中查找第一个只出现一次的字符
2012-11-29 22:13
726 查看
1.问题描述
在一个字符串中找到第一个只出现一次的字符。如输入AbAssvfFCCBbhV,则输出v。
2.解题思路
如果按照传统比较的办法,时间复杂度是O(n*n),这显然不是Google面试官希望的答案,本题实际上是哈希表的一个应用,我们举个简单例子
比如:输入AbAcc
我们建立哈希表
0 1 2 3 ... 25 26 27 28 .... 51
字符 b c A
出现次数 1 2 2
这样只要我们构造好哈希表,我们可以在O(n)内找到b,而构造哈希表的时间复杂度是O(n)
话又说回来,世界上没有完美的事,我们虽然节省了时间,却要用额外的空间来换
3.代码
4 测试

C代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
/*
* to find the first char which only once ocurred in a string
* use the Hash method to solve the problem
* */
int HashTable[52]; // contains A-F and a-z character
/*
* hash function
* */
int Hash(char c) {
if(isupper(c)) {
return c - 'A';
}
else if(islower(c)) {
return 26 + c - 'a';
}
}
/*
* scan the str and load the hash table
* */
void LoadHashTable(const char *str) {
int i;
int pos; // the hash address
for(i = 0;i < strlen(str);i++) {
pos = Hash(str[i]);
HashTable[pos] += 1;
}
}
/*
* to search the target
* */
int Locate(const char *str, char &X) {
int i;
int pos;
int val;
int find = 0; // whether find the result
for(i = 0;i < strlen(str);i++) {
pos = Hash(str[i]);
val = HashTable[pos];
if(val == 1) {
/*
* to find the target character
* */
switch(pos/26) {
case 0:
X = 'A' + pos%26;
break;
case 1:
X = 'a' + pos%26;
break;
}
find = 1;
}
}
return find;
}
void main() {
char buf[1024];
char c;
int i;
gets(buf);
for(i = 0;i < strlen(buf);i++) {
if(!isupper(buf[i]) && !islower(buf[i])) {
perror("string only can contains character!\n");
exit(1);
}
}
LoadHashTable(buf);
if(Locate(buf, c)) {
putchar(c);
}
else perror("没有出现一次的字母!");
printf("\n");
}
测试:
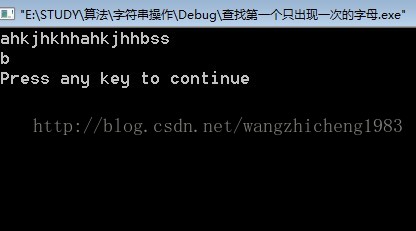
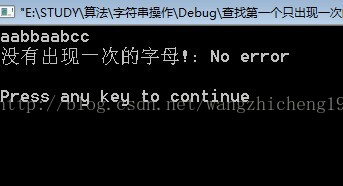
在一个字符串中找到第一个只出现一次的字符。如输入AbAssvfFCCBbhV,则输出v。
2.解题思路
如果按照传统比较的办法,时间复杂度是O(n*n),这显然不是Google面试官希望的答案,本题实际上是哈希表的一个应用,我们举个简单例子
比如:输入AbAcc
我们建立哈希表
0 1 2 3 ... 25 26 27 28 .... 51
字符 b c A
出现次数 1 2 2
这样只要我们构造好哈希表,我们可以在O(n)内找到b,而构造哈希表的时间复杂度是O(n)
话又说回来,世界上没有完美的事,我们虽然节省了时间,却要用额外的空间来换
3.代码
/* This is a free Program, You can modify or redistribute it under the terms of GNU *Description:利用哈希表查找第一个只出现一次的字符 *Language: C++ *Development Environment: VC6.0 *Author: Wangzhicheng *E-mail: 2363702560@qq.com *Date: 2012/11/29 */ #include <iostream> #include <cstdlib> #include <string> #include <cctype> using namespace std; class Solution { private: int n; //共52个大小写字母 string s; //输入的字符串,只能含有大小写 /* *哈希表 *@ch:存放字母 *@cur:字母出现的次数 */ typedef struct Hash { char ch; int cur; }; Hash *HashTable; char answer; //第一次只出现一次的字符 /* *哈希函数 *小写字母存放0-25的位置 *大写字母存放26-51的位置 */ int HashFunction(char ch) { if(islower(ch)) { return ch-'a'; } else return ch-'A'+n/2; } /* *装载哈希表 */ void LoadHashTable() { int i; int pos; for(i=0;s[i];i++) { pos=HashFunction(s[i]); if(!HashTable[pos].ch) { //该位置为空 HashTable[pos].ch=s[i]; HashTable[pos].cur=1; } else HashTable[pos].cur++; } } public: /* *构造函数 *为哈希表分配空间 *初始化字符串 */ Solution(const string &str) { n=52; HashTable=new Hash ; if(!HashTable) { cerr<<"内存不足,程序退出!"; exit(1); } int i; for(i=0;i<n;i++) { HashTable[i].ch='\0'; HashTable[i].cur=0; } s=str; answer='\0'; } /* *查找第一次只出现一次的字符的函数 *利用哈希表进行查找 */ void JustDoIt() { LoadHashTable(); int i; int pos; for(i=0;i<n;i++) { pos=HashFunction(s[i]); if(HashTable[pos].cur==1) { //此时该字符出现的次数为1 answer=s[i]; return; } } } char get() const { return answer; } void Answer() { if(!get()) cout<<"本字符串中没有第一次只出现一次的字符"<<endl; else cout<<"第一次只出现一次的字符是:"<<get()<<endl; } ~Solution() { delete []HashTable; } }; void main() { string str; cout<<"请输入一个字符串:"; cin>>str; if(!cin.good()) { cerr<<"输入异常!"<<endl; exit(1); } int i; for(i=0;str[i];i++) { if(!islower(str[i]) && !isupper(str[i])) { cerr<<"字符串只能含有大小写字符!"<<endl; return; } } Solution solution(str); solution.JustDoIt(); solution.Answer(); }
4 测试

C代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
/*
* to find the first char which only once ocurred in a string
* use the Hash method to solve the problem
* */
int HashTable[52]; // contains A-F and a-z character
/*
* hash function
* */
int Hash(char c) {
if(isupper(c)) {
return c - 'A';
}
else if(islower(c)) {
return 26 + c - 'a';
}
}
/*
* scan the str and load the hash table
* */
void LoadHashTable(const char *str) {
int i;
int pos; // the hash address
for(i = 0;i < strlen(str);i++) {
pos = Hash(str[i]);
HashTable[pos] += 1;
}
}
/*
* to search the target
* */
int Locate(const char *str, char &X) {
int i;
int pos;
int val;
int find = 0; // whether find the result
for(i = 0;i < strlen(str);i++) {
pos = Hash(str[i]);
val = HashTable[pos];
if(val == 1) {
/*
* to find the target character
* */
switch(pos/26) {
case 0:
X = 'A' + pos%26;
break;
case 1:
X = 'a' + pos%26;
break;
}
find = 1;
}
}
return find;
}
void main() {
char buf[1024];
char c;
int i;
gets(buf);
for(i = 0;i < strlen(buf);i++) {
if(!isupper(buf[i]) && !islower(buf[i])) {
perror("string only can contains character!\n");
exit(1);
}
}
LoadHashTable(buf);
if(Locate(buf, c)) {
putchar(c);
}
else perror("没有出现一次的字母!");
printf("\n");
}
测试:
相关文章推荐
- 【google 2006年笔试题】 在一个字符串中找到第一个只出现一次的字符(包括普通汉字)
- 查找一个字符串中第一个只出现两/一次的字符
- 一个字符串中查找第一个只出现一次的字符 ,时间复杂度为O(N)
- 在一个字符串中查找第一个只出现一次的字符,要求复杂度为O(N)
- 哈希表对字符串的高效处理1:在一个字符串中找到第一个只出现一次字符
- 一个字符串中查找第一个只出现一次的字符。 要求复杂度为O(N).
- c::在一个字符串中查找第一个只出现一次的字符
- [置顶] 在一个字符串中查找第一个只出现一次的字符。要求复杂度为O(N).
- 一个字符串中查找第一个只出现一次的字符。 要求复杂度为O(N).
- 查找一个字符串中,出现的第一个单身字符。/一个字符串中查找第一个只出现一次的字符。 //要求复杂度为O(N).
- 一个字符串中查找第一个只出现一次的字符。 要求复杂度为O(N).
- 在一个字符串中找到第一个只出现一次的字符
- 26.在一个字符串(1<=字符串长度<=10000,全部由大写字母组成)中找到第一个只出现一次的字符,并返回它的位置
- 在一个字符串中找到第一个只出现一次的字符。
- 查找字符串中第一个只出现一次的字符
- 查找字符串中第一个出现一次的字符
- 在一个字符串中找到第一个只出现一次的字符。如输入abaccdeff,则输出b。
- 【剑指Offer-时间效率平衡】在一个字符串(1<=字符串长度<=10000,全部由字母组成)中找到第一个只出现一次的字符,并返回它的位置
- 给定一个字符串,查找该字符串中第一个重复出现的字符索引。要求:时间复杂度为O(n)。
- 在一个字符串中找到第一个只出现一次的字符