【转载】一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
2012-11-23 10:13
573 查看
一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
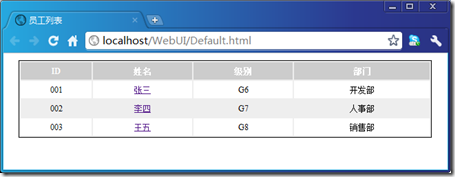
1: using System.Collections.Generic;
2: using System.ServiceModel;
3: using System.ServiceModel.Web;
4: namespace Artech.WcfServices.Service.Interface
5: {
6: [ServiceContract]
7: public interface IEmployees
8: {
9: [WebGet(UriTemplate = "all",ResponseFormat =WebMessageFormat.Json)]
10: IEnumerable<Employee> GetAll();
11: }
12: public class Employee
13: {
14: public string Id { get; set; }
15: public string Name { get; set; }
16: public string Department { get; set; }
17: public string Grade { get; set; }
18: }
19: }[/code]在如下所示的服务类型EmployeesService 中,我们直接让服务操作GetAll返回一个包含3个Employee对象的列表。
1: using System.Collections.Generic;
2: using Artech.WcfServices.Service.Interface;
3: namespace Artech.WcfServices.Service
4: {
5: public class EmployeesService : IEmployees
6: {
7: public IEnumerable<Employee> GetAll()
8: {
9: return new List<Employee>
10: {
11: new Employee{ Id = "001", Name="张三", Department="开发部", Grade = "G6"},
12: new Employee{ Id = "002", Name="李四", Department="人事部", Grade = "G7"},
13: new Employee{ Id = "003", Name="王五", Department="销售部", Grade = "G8"}
14: };
15: }
16: }
17: }[/code]我们通过控制台程序对服务进行寄宿。从下面的配置可以看到我们采用了标准终结点WebHttpEndpoint。为了让服务具有跨域支持的能力,我们必须将标准终结点的crossDomainScriptAccessEnabled属性设置为True。WebHttpBinding也具有同名的属性,如果直接使用WebHttpBinding也需要将该属性设置为True。
1: <configuration>
2: <system.serviceModel>
3: <standardEndpoints>
4: <webHttpEndpoint>
5: <standardEndpoint crossDomainScriptAccessEnabled="true"/>
6: </webHttpEndpoint>
7: </standardEndpoints>
8: <bindings>
9: <webHttpBinding>
10: <binding crossDomainScriptAccessEnabled="true" />
11: </webHttpBinding>
12: </bindings>
13: <services>
14: <service name="Artech.WcfServices.Service.EmployeesService">
15: <endpoint kind="webHttpEndpoint"
16: address="http://127.0.0.1:3721/employees"
17: contract="Artech.WcfServices.Service.Interface.IEmployees"/>
18: </service>
19: </services>
20: </system.serviceModel>
21: </configuration>[/code]在客户端,我们在一个Web页面中通过jQuery进行Ajax调用这个服务,并将得到的员工列表显示在一个表格中。出CSS之外的页面代码如下所示,需要注意的是在进行Ajax调用的使用将dataType选项设置成“jsonp”,而不是“json”。
1: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
2: <html xmlns="http://www.w3.org/1999/xhtml">
3: <head>
4: <title>员工列表</title>
5: <style type="text/css">
6: ...
7: </style>
8: <script src="Scripts/jquery-1.7.1.js" type="text/javascript"></script>
9: <script type="text/javascript">
10: $(function () {
11: $.ajax({
12: type: "get",
13: url: "http://127.0.0.1:3721/employees/all",
14: dataType: "jsonp",
15: success: function (employees) {
16: $.each(employees, function (index, value) {
17: var detailUrl = "detail.html?id=" + value.Id;
18: var html = "<tr><td>";
19: html += value.Id + "</td><td>";
20: html += "<a href='" + detailUrl + "'>" + value.Name + "</a></td><td>";
21: html += value.Grade + "</td><td>";
22: html += value.Department + "</td></tr>";
23: $("#employees").append(html);
24: });
25: $("#employees tr:odd").addClass("oddRow");
26: }
27: });
28: });
29: </script>
30: </head>
31: <body>
32: <table id="employees" width="600px">
33: <tr>
34: <th>ID</th>
35: <th>姓名</th>
36: <th>级别</th>
37: <th>部门</th>
38: </tr>
39: </table>
40: </body>
41: </html>[/code]当服务启动后在浏览器中显示上面这个Web页面,会得到如下所示的员工列表。
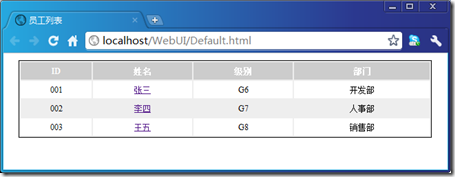
相关文章推荐
- 一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
- 一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
- 一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
- 一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
- 一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
- 一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
- 一个通过JSONP跨域调用WCF REST服务的例子(以jQuery为例)
- 通过jsonp调用wcf服务的例子
- JQUERY 跨域调用WCF REST服务
- 实现jquery.ajax及原生的XMLHttpRequest跨域调用WCF服务的方法
- 关于jquery跨域调用REST服务的问题
- 一步一个脚印学习WCF系列之WCF概要—WCF服务的创建与调用HelloWorld实例,通过编码方式(四)
- 【转载】[WCF REST] 一个简单的REST服务实例
- JQuery使用$.ajax跨域调用winform托管的WCF服务(原创)
- WCF RESTful 服务+Jquery 客户端 跨域调用 大坑及解决方案汇总
- 一步一个脚印学习WCF系列之WCF概要—WCF服务的创建与调用HelloWorld实例,通过配置文件方式(六)
- JQuery跨域调用Rest服务接口
- JQuery 跨域调用 WCF 服务
- C# sliverlight调用WCF服务出现的一个错误
- jQuery实现ajax调用WCF服务的方法(附带demo下载)