ExtJS带进度条的登陆验证+验证码+回车提交
2012-08-22 17:29
357 查看
如下是ExtJS的登陆界面效果图:
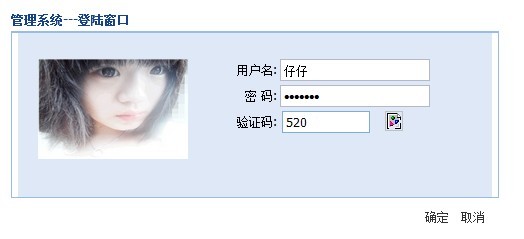

以下是程序代码:
/**************************************login.html***************************************/
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>login.html</title>
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="this is my page">
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<link rel="stylesheet" href="JS/ext/resources/css/ext-all.css" type="text/css"></link>
<script type="text/javascript" src="JS/ext/adapter/ext/ext-base.js"></script>
<script type="text/javascript" src="JS/ext/ext-all.js"></script>
<script type="text/javascript" src="JS/js/login.js"></script>
</head>
<body>
<!-- <div id="divLogin" ></div> -->
<img src="Image/22214Z262N.gif" id="leftImg" width="150" height="100" style="display:none"/>
</body>
</html>
/*********************************实体类 User***************************************/
package aa.bb.cc.vo;
public class User {
private int id;
private String userName;
private String password;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
}
/*********************获得数据库连接Connection对象*****************/
package aa.bb.cc.dao;
import java.sql.*;
public class ConnectionManager {
/**
* 获得数据库连接
*
* @return
*/
public static Connection getConn() {
Connection conn = null;
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
String url = "jdbc:sqlserver://127.0.0.1:1433;database=test";
String username = "sa";
String password = "123456";
conn = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException e) {
System.out.println("加载驱动发生异常");
e.printStackTrace();
} catch (SQLException e) {
System.out.println("获取连接发生异常");
e.printStackTrace();
}
return conn;
}
public static void close(Connection conn) {
try {
if (conn != null)
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(PreparedStatement pstmt) {
try {
if (pstmt != null)
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(ResultSet rs) {
try {
if (rs != null)
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(ResultSet rs,PreparedStatement pstmt,Connection conn) {
close(rs);
close(pstmt);
close(conn);
}
}
/*************************************UserDao**************************************/
package aa.bb.cc.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import aa.bb.cc.vo.User;
public class UserDao {
public boolean ValidateLogin(User user){
Connection conn=null;
PreparedStatement pstmt=null;
ResultSet rs=null;
String sql="SELECT COUNT(*) FROM [User] WHERE username=? and password=? ";
boolean isExist=false;
try {
conn=ConnectionManager.getConn();
pstmt=conn.prepareStatement(sql);
pstmt.setString(1, user.getUserName());
pstmt.setString(2, user.getPassword());
rs=pstmt.executeQuery();
if(rs!=null&&rs.next()){
int count=rs.getInt(1);
if(count==1){
isExist=true;
}
else{
isExist=false;
}
}
} catch (SQLException e) {
e.printStackTrace();
}
finally{
ConnectionManager.close(rs, pstmt, conn);
}
return isExist;
}
}
/************************************selvlet*****************************************/
package aa.bb.cc.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import aa.bb.cc.dao.UserDao;
import aa.bb.cc.vo.User;
public class LoginServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/xml");
response.setCharacterEncoding("utf-8");
PrintWriter out = response.getWriter();
HttpSession session = request.getSession();
String userName=request.getParameter("UserName");
String pwd=request.getParameter("Password");
String validatecode = request.getParameter("ValidateCode");
String realcode = session.getAttribute("rand").toString();
if(!userName.trim().equals("") && !pwd.trim().equals("")&& !validatecode.trim().equals("")){
User user=new User();
user.setUserName(userName);
user.setPassword(pwd);
boolean isExist=false;
try {
isExist=new UserDao().ValidateLogin(user);
} catch (RuntimeException e) {
out.println("{success:false,msg:'验证用户登陆失败'" + "}");
e.printStackTrace();
}
if(validatecode.equalsIgnoreCase(realcode)){
if (isExist) {
out.println("{success:true,msg:'ok'" + "}");
} else {
out.println("{success:true,msg:'用户名或者密码错误!'" + "}");
}
}
else{
out.println("{success:true,msg:'验证码输入错误!'" + "}");
}
}
out.flush();
out.close();
}
}
*************************验证码valiCode.jsp****************************
<%@ page contentType="image/jpeg" import="java.util.*,java.awt.*,java.io.*,java.awt.image.*,javax.imageio.*" pageEncoding="utf-8"%>
<%!
Color getRandColor(int fc,int bc){//给定范围获得随机颜色
Random random = new Random();
if(fc>255) fc=255;
if(bc>255) bc=255;
int r=fc+random.nextInt(bc-fc);
int g=fc+random.nextInt(bc-fc);
int b=fc+random.nextInt(bc-fc);
return new Color(r,g,b);
}
%>
<%
//设置页面不缓存
response.setHeader("Pragma","No-cache");
response.setHeader("Cache-Control","no-cache");
response.setDateHeader("Expires", 0);
// 在内存中创建图象
int width=60, height=20;
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
// 获取图形上下文
Graphics g = image.getGraphics();
//生成随机类
Random random = new Random();
// 设定背景色
g.setColor(getRandColor(200,250));
g.fillRect(0, 0, width, height);
//设定字体
g.setFont(new Font("Times New Roman",Font.PLAIN,18));
//画边框
//g.setColor(new Color());
//g.drawRect(0,0,width-1,height-1);
// 随机产生155条干扰线,使图象中的认证码不易被其它程序探测到
g.setColor(getRandColor(160,200));
for (int i=0;i<155;i++)
{
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = random.nextInt(12);
int yl = random.nextInt(12);
g.drawLine(x,y,x+xl,y+yl);
}
// 取随机产生的认证码(4位数字)
String sRand="";
for (int i=0;i<4;i++){
String rand=String.valueOf(random.nextInt(10));
sRand+=rand;
// 将认证码显示到图象中
g.setColor(new Color(20+random.nextInt(110),20+random.nextInt(110),20+random.nextInt(110)));// 调用函数出来的颜色相同,可能是因为种子太接近,所以只能直接生成
g.drawString(rand,13*i+6,16);
}
// 将认证码存入SESSION
session.setAttribute("rand",sRand);
// 图象生效
g.dispose();
OutputStream output=response.getOutputStream();
// 输出图象到页面
ImageIO.write(image, "JPEG", response.getOutputStream());
output.flush();
out.clear();
out = pageContext.pushBody();
%>
/**************************************login.js*********************************/
/**
* js file for login form
* ExtJS带进度条的登陆验证+验证码+回车提交
*/
//========================================================//
function LoginDemo()
{
Ext.QuickTips.init();
Ext.form.Field.prototype.msgTarget = "side";
//点击图片,更换验证码。
scode=function(){
Ext.getDom("imgcode").src="valiCode.jsp?dt=" + (new Date()).getTime();
}
//左面显示图片
var leftPanel = new Ext.Panel({
id:"leftPanel",
contentEl:"leftImg",
bodyStyle:"padding:20px",
columnWidth:.4
});
//验证码
var validate=new Ext.Panel({
layout : "column",
items:[{
columnWidth:.65,
layout:"form",
labelWidth:50,
defaultType:"textfield",
items:[{
fieldLabel:"验证码",
name:"ValidateCode",
//maxLength:4,
width:"80",
allowBlank:false,
blankText:"验证码不能为空!",
regex:/^[0-9][0-9_]{0,3}$/,
regexText:"验证码由4位数字组成!"
}]
},{
columnWidth:.35,
layout:"form",
items:[{
xtype:"panel",
html:"<a href='javascript:scode()'><img src='valiCode.jsp' id='imgcode' /></a>",
width:"60",
height:"20"
}]
}]
});
//右方显示输入框
var rightPanel = new Ext.form.FormPanel({
id:"rightPanel",
labelPad:0,
labelWidth:50,
labelAlign :"right",
bodyStyle:"padding:20px",
layout:"form",
columnWidth:.6,
defaultType:"textfield",
keys:[{ //键盘回车提交功能
key: [10,13],
fn:surely
}],
items:[{
id:"username",
name:"UserName",
fieldLabel:"用户名" ,
width :150,
allowBlank:false,
blankText:"请输入用户名!",
selectFocus:true
//value:"accp"
},
{
name:"Password",
fieldLabel:"密 码" ,
width :150,
inputType:"password",
allowBlank:false,
blankText:"密码不能为空!"
},validate
]
});
//登陆窗体将左面和右面组合在一起,采用layout:"column",的布局方式
var loginPanel = new Ext.Panel
({
id:"loginPanel",
height :500,
frame:true,
layout:"column",
items:
[
leftPanel,rightPanel
]
});
function surely() { // 定义表单提交函数
// 如果通过验证
if (rightPanel.form.isValid()) {
//this.disabled = true;
//alert("ok");
// 验证合法后使用加载进度条
Ext.MessageBox.show({
// 等待标题
title : "请等待正在登陆",
// 允许进度条
progress : true,
// 设置宽度
width : 300
});
// 控制进度速度
var f = function(v) {
// 返回进度条 状态
return function() {
// 到了11 隐藏
if (v == 12) {
Ext.MessageBox.hide();
} else {
var i = v / 11;
Ext.MessageBox.updateProgress(
i, Math.round(100 * i)
+ '%提交');
}
};
};
// 循环
for (var i = 0; i < 13; i++) {
// 定时器
setTimeout(f(i), i * 500);
}
// 提交到服务器操作
rightPanel.form.doAction('submit', {
// 提交路径 我配置的一个Servlet
url :"http://127.0.0.1:8080/TestExtJS/servlet/LoginServlet",
// 等待消息标题
//waitTitle : "等待中",
// 提交方式 分为POST 和GET
method : "POST",
// 等待消息信息
//waitMsg : "正在提交请稍后......",
// 提交成功的回调函数
success : function(form, action) {
//alert("ok");
if (action.result.msg == 'ok') {
Ext.Msg.alert("登陆提示", "登陆成功!!");
} else {
Ext.Msg.alert("登陆失败",action.result.msg);
// rightPanel.form.reset();
// scode();
//Ext.getCmp("username").focus(true);
}
} ,
failure : function(form, action) { // 提交失败的回调函数
Ext.Msg.alert('错误','服务器出现错误请稍后再试!');
}
});
}
}
//通过Window窗口的方式显示登陆窗体
var loginWindow = new Ext.Window ({
id:"loginWindow",
title:"管理系统---登陆窗口",
width:500,
height:230,
resizable:false,
items:
[
loginPanel
],
buttons:
[{
type:"submit",
text:"确定",
width:25,
handler : surely
},
{
type:"button",
text:"取消",
width:25,
handler : function() {
//loginWindow.close(); //重置表单:var one = Ext.getCmp('rightPanel');
rightPanel.form.reset(); // one.getForm().reset(); 或 rightPanel.form.reset();
scode();
}
}],
buttonAlign:"right"
});
Ext.getDom("leftImg").style.display="block";
loginWindow.show();
}
Ext.onReady(LoginDemo);
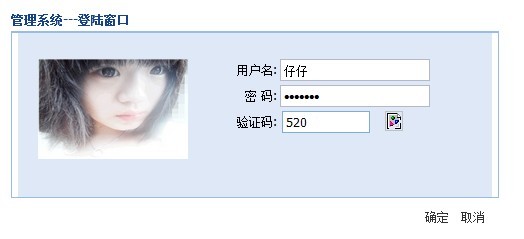

以下是程序代码:
/**************************************login.html***************************************/
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>login.html</title>
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="this is my page">
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<link rel="stylesheet" href="JS/ext/resources/css/ext-all.css" type="text/css"></link>
<script type="text/javascript" src="JS/ext/adapter/ext/ext-base.js"></script>
<script type="text/javascript" src="JS/ext/ext-all.js"></script>
<script type="text/javascript" src="JS/js/login.js"></script>
</head>
<body>
<!-- <div id="divLogin" ></div> -->
<img src="Image/22214Z262N.gif" id="leftImg" width="150" height="100" style="display:none"/>
</body>
</html>
/*********************************实体类 User***************************************/
package aa.bb.cc.vo;
public class User {
private int id;
private String userName;
private String password;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
}
/*********************获得数据库连接Connection对象*****************/
package aa.bb.cc.dao;
import java.sql.*;
public class ConnectionManager {
/**
* 获得数据库连接
*
* @return
*/
public static Connection getConn() {
Connection conn = null;
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
String url = "jdbc:sqlserver://127.0.0.1:1433;database=test";
String username = "sa";
String password = "123456";
conn = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException e) {
System.out.println("加载驱动发生异常");
e.printStackTrace();
} catch (SQLException e) {
System.out.println("获取连接发生异常");
e.printStackTrace();
}
return conn;
}
public static void close(Connection conn) {
try {
if (conn != null)
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(PreparedStatement pstmt) {
try {
if (pstmt != null)
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(ResultSet rs) {
try {
if (rs != null)
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(ResultSet rs,PreparedStatement pstmt,Connection conn) {
close(rs);
close(pstmt);
close(conn);
}
}
/*************************************UserDao**************************************/
package aa.bb.cc.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import aa.bb.cc.vo.User;
public class UserDao {
public boolean ValidateLogin(User user){
Connection conn=null;
PreparedStatement pstmt=null;
ResultSet rs=null;
String sql="SELECT COUNT(*) FROM [User] WHERE username=? and password=? ";
boolean isExist=false;
try {
conn=ConnectionManager.getConn();
pstmt=conn.prepareStatement(sql);
pstmt.setString(1, user.getUserName());
pstmt.setString(2, user.getPassword());
rs=pstmt.executeQuery();
if(rs!=null&&rs.next()){
int count=rs.getInt(1);
if(count==1){
isExist=true;
}
else{
isExist=false;
}
}
} catch (SQLException e) {
e.printStackTrace();
}
finally{
ConnectionManager.close(rs, pstmt, conn);
}
return isExist;
}
}
/************************************selvlet*****************************************/
package aa.bb.cc.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import aa.bb.cc.dao.UserDao;
import aa.bb.cc.vo.User;
public class LoginServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/xml");
response.setCharacterEncoding("utf-8");
PrintWriter out = response.getWriter();
HttpSession session = request.getSession();
String userName=request.getParameter("UserName");
String pwd=request.getParameter("Password");
String validatecode = request.getParameter("ValidateCode");
String realcode = session.getAttribute("rand").toString();
if(!userName.trim().equals("") && !pwd.trim().equals("")&& !validatecode.trim().equals("")){
User user=new User();
user.setUserName(userName);
user.setPassword(pwd);
boolean isExist=false;
try {
isExist=new UserDao().ValidateLogin(user);
} catch (RuntimeException e) {
out.println("{success:false,msg:'验证用户登陆失败'" + "}");
e.printStackTrace();
}
if(validatecode.equalsIgnoreCase(realcode)){
if (isExist) {
out.println("{success:true,msg:'ok'" + "}");
} else {
out.println("{success:true,msg:'用户名或者密码错误!'" + "}");
}
}
else{
out.println("{success:true,msg:'验证码输入错误!'" + "}");
}
}
out.flush();
out.close();
}
}
*************************验证码valiCode.jsp****************************
<%@ page contentType="image/jpeg" import="java.util.*,java.awt.*,java.io.*,java.awt.image.*,javax.imageio.*" pageEncoding="utf-8"%>
<%!
Color getRandColor(int fc,int bc){//给定范围获得随机颜色
Random random = new Random();
if(fc>255) fc=255;
if(bc>255) bc=255;
int r=fc+random.nextInt(bc-fc);
int g=fc+random.nextInt(bc-fc);
int b=fc+random.nextInt(bc-fc);
return new Color(r,g,b);
}
%>
<%
//设置页面不缓存
response.setHeader("Pragma","No-cache");
response.setHeader("Cache-Control","no-cache");
response.setDateHeader("Expires", 0);
// 在内存中创建图象
int width=60, height=20;
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
// 获取图形上下文
Graphics g = image.getGraphics();
//生成随机类
Random random = new Random();
// 设定背景色
g.setColor(getRandColor(200,250));
g.fillRect(0, 0, width, height);
//设定字体
g.setFont(new Font("Times New Roman",Font.PLAIN,18));
//画边框
//g.setColor(new Color());
//g.drawRect(0,0,width-1,height-1);
// 随机产生155条干扰线,使图象中的认证码不易被其它程序探测到
g.setColor(getRandColor(160,200));
for (int i=0;i<155;i++)
{
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = random.nextInt(12);
int yl = random.nextInt(12);
g.drawLine(x,y,x+xl,y+yl);
}
// 取随机产生的认证码(4位数字)
String sRand="";
for (int i=0;i<4;i++){
String rand=String.valueOf(random.nextInt(10));
sRand+=rand;
// 将认证码显示到图象中
g.setColor(new Color(20+random.nextInt(110),20+random.nextInt(110),20+random.nextInt(110)));// 调用函数出来的颜色相同,可能是因为种子太接近,所以只能直接生成
g.drawString(rand,13*i+6,16);
}
// 将认证码存入SESSION
session.setAttribute("rand",sRand);
// 图象生效
g.dispose();
OutputStream output=response.getOutputStream();
// 输出图象到页面
ImageIO.write(image, "JPEG", response.getOutputStream());
output.flush();
out.clear();
out = pageContext.pushBody();
%>
/**************************************login.js*********************************/
/**
* js file for login form
* ExtJS带进度条的登陆验证+验证码+回车提交
*/
//========================================================//
function LoginDemo()
{
Ext.QuickTips.init();
Ext.form.Field.prototype.msgTarget = "side";
//点击图片,更换验证码。
scode=function(){
Ext.getDom("imgcode").src="valiCode.jsp?dt=" + (new Date()).getTime();
}
//左面显示图片
var leftPanel = new Ext.Panel({
id:"leftPanel",
contentEl:"leftImg",
bodyStyle:"padding:20px",
columnWidth:.4
});
//验证码
var validate=new Ext.Panel({
layout : "column",
items:[{
columnWidth:.65,
layout:"form",
labelWidth:50,
defaultType:"textfield",
items:[{
fieldLabel:"验证码",
name:"ValidateCode",
//maxLength:4,
width:"80",
allowBlank:false,
blankText:"验证码不能为空!",
regex:/^[0-9][0-9_]{0,3}$/,
regexText:"验证码由4位数字组成!"
}]
},{
columnWidth:.35,
layout:"form",
items:[{
xtype:"panel",
html:"<a href='javascript:scode()'><img src='valiCode.jsp' id='imgcode' /></a>",
width:"60",
height:"20"
}]
}]
});
//右方显示输入框
var rightPanel = new Ext.form.FormPanel({
id:"rightPanel",
labelPad:0,
labelWidth:50,
labelAlign :"right",
bodyStyle:"padding:20px",
layout:"form",
columnWidth:.6,
defaultType:"textfield",
keys:[{ //键盘回车提交功能
key: [10,13],
fn:surely
}],
items:[{
id:"username",
name:"UserName",
fieldLabel:"用户名" ,
width :150,
allowBlank:false,
blankText:"请输入用户名!",
selectFocus:true
//value:"accp"
},
{
name:"Password",
fieldLabel:"密 码" ,
width :150,
inputType:"password",
allowBlank:false,
blankText:"密码不能为空!"
},validate
]
});
//登陆窗体将左面和右面组合在一起,采用layout:"column",的布局方式
var loginPanel = new Ext.Panel
({
id:"loginPanel",
height :500,
frame:true,
layout:"column",
items:
[
leftPanel,rightPanel
]
});
function surely() { // 定义表单提交函数
// 如果通过验证
if (rightPanel.form.isValid()) {
//this.disabled = true;
//alert("ok");
// 验证合法后使用加载进度条
Ext.MessageBox.show({
// 等待标题
title : "请等待正在登陆",
// 允许进度条
progress : true,
// 设置宽度
width : 300
});
// 控制进度速度
var f = function(v) {
// 返回进度条 状态
return function() {
// 到了11 隐藏
if (v == 12) {
Ext.MessageBox.hide();
} else {
var i = v / 11;
Ext.MessageBox.updateProgress(
i, Math.round(100 * i)
+ '%提交');
}
};
};
// 循环
for (var i = 0; i < 13; i++) {
// 定时器
setTimeout(f(i), i * 500);
}
// 提交到服务器操作
rightPanel.form.doAction('submit', {
// 提交路径 我配置的一个Servlet
url :"http://127.0.0.1:8080/TestExtJS/servlet/LoginServlet",
// 等待消息标题
//waitTitle : "等待中",
// 提交方式 分为POST 和GET
method : "POST",
// 等待消息信息
//waitMsg : "正在提交请稍后......",
// 提交成功的回调函数
success : function(form, action) {
//alert("ok");
if (action.result.msg == 'ok') {
Ext.Msg.alert("登陆提示", "登陆成功!!");
} else {
Ext.Msg.alert("登陆失败",action.result.msg);
// rightPanel.form.reset();
// scode();
//Ext.getCmp("username").focus(true);
}
} ,
failure : function(form, action) { // 提交失败的回调函数
Ext.Msg.alert('错误','服务器出现错误请稍后再试!');
}
});
}
}
//通过Window窗口的方式显示登陆窗体
var loginWindow = new Ext.Window ({
id:"loginWindow",
title:"管理系统---登陆窗口",
width:500,
height:230,
resizable:false,
items:
[
loginPanel
],
buttons:
[{
type:"submit",
text:"确定",
width:25,
handler : surely
},
{
type:"button",
text:"取消",
width:25,
handler : function() {
//loginWindow.close(); //重置表单:var one = Ext.getCmp('rightPanel');
rightPanel.form.reset(); // one.getForm().reset(); 或 rightPanel.form.reset();
scode();
}
}],
buttonAlign:"right"
});
Ext.getDom("leftImg").style.display="block";
loginWindow.show();
}
Ext.onReady(LoginDemo);
相关文章推荐
- ExtJS带验证码登录框[新增回车提交]
- ExtJS带验证码登录框[新增回车提交]
- .net 实现三种验证码(汉字验证码,数字验证码,数字+英文验证)附带登陆验证实例
- net 实现三种验证码(汉字验证码,数字验证码,数字+英文验证)附带登陆验证实例
- JS表单提交验证、input(type=number) 去三角 刷新验证码
- 表单提交验证 回车验证 ! 示例
- Ajax提交表单时验证码自动验证 php后端验证码检测
- ExtJs怎样提交验证
- 登陆验证实例——提交数据到服务器
- 三层架构(MVC)实现简单登陆注册验证(含验证码)
- ExtJs 表单提交登陆实现代码
- 软件工程课程设计问题总结——医院门诊系统(二):jsp中验证码的实现&设置验证失败不提交表单
- Ajax提交表单时验证码自动验证 php后端验证码检测
- ExtJS学习笔记3:载入、提交和验证表单
- extjs 含有验证码的登陆窗口
- extJs+json实现的一个登陆验证的简单例子
- [置顶] ExtJs4.2 登陆界面(点击验证码自动刷新,label实现click事件)
- Ajax提交表单时验证码自动验证 php后端验证码检测
- yii2.0 ajax登陆验证失败验证码不自动刷新
- 【Extjs登陆】表单ajax提交,登录实例 后台为servlet