Selenium core & IDE extension functions
2012-07-20 13:10
274 查看
Selenium core extension functions:
Selenium.prototype.doStoreRandom = function (variableName) {
random = Math.floor(Math.random() * 10000000);
storedVars[variableName] = random;
}
Selenium.prototype.doDisplayAlert = function (variableName,value) {
alert(value);
}
//target=variableName; value=len
Selenium.prototype.doStoreRandomDefinedLength = function (variableName,len) {
//alert(len);
//alert(variableName);
var randomDefinedLength = (Math.floor(Math.random() * 10000000)).toString();
//alert(randomDefinedLength);
//alert(randomDefinedLength.length);
if(randomDefinedLength.length<len)
{
var needIncreaseLen = len - randomDefinedLength.length;
//alert(needIncreaseLen);
for(var i =0;i<needIncreaseLen;i++)
{
randomDefinedLength += "a";
}
}else if (randomDefinedLength.length>len)
{
var needCutLen = randomDefinedLength.length - len;
//alert(needCutLen);
randomDefinedLength = randomDefinedLength.substr(needCutLen);
}
storedVars[variableName] = randomDefinedLength;
}
用Selenium IDE做网页的功能测试经常会碰到与日期有关的验证,本人结合实际工作中遇到的应用,写了一个扩展,代码如下:
Selenium.prototype.doStoreDateByFormat = function(format,args){
// format of args: month,day,year
if(args.trim().length < 3)
throw new SeleniumError("arguments must contain ""Month"",""Date"",""Year"" variables!");
var formats = format.split(",");
var days = parseInt(formats[3]);
var sysDate = getSysDate(); //get the sysdate
var specDate = dateAfterDays(sysDate,parseFloat(days),1); //get specified date
var arrArgs = args.split(",");
var month = specDate.getMonth()+1;
var date = specDate.getDate();
var year = specDate.getFullYear();
// get Month string
switch(formats[0].toUpperCase()){
case "MM": // return 2 digits of month number, such as: 01
month = (month+"").length==1?"0"+month:month;
break;
case "MMM": //return the first 3 chars of the month word, such as: Jan
month = this.getMonthShortName(month);
break;
case "MMMM": //return the full word of the month word, such as: January
month = this.getMonthFullName(month);
break;
case "M":
default:
// return 1 digit when month is lower than 10.
// do nothing
}
//get Date string
switch(formats[1].toUpperCase()){
case "DD": //always return 2 digits of the month number, such as: 05
date = (date+"").length==1?"0"+date:date;
break;
case "D":
default:
// return 1 digit when Date is lower than 10.
// do nothing
}
//get Year string
switch(formats[2].toUpperCase()){
case "YY": // return last 2 digits of the year number, such as: 08 (2008)
year = (year+"").substr(2);
break;
case "YYYY":
default:
//return full year number, such: 2008.
}
storedVars[arrArgs[0]] = month;
storedVars[arrArgs[1]] = date;
storedVars[arrArgs[2]] = year;
}
Selenium.prototype.getMonthFullName = function(month){
var monthArr = new Array("January","February","March","April","May","June","July","August","September","October","November","December");
if(month == null){
throw new SeleniumError("you didn't specify a Month");
}
try{
month = parseInt(month);
}catch (e){
throw new SeleniumError("""Month"" is not a Integer!");
}
return monthArr[month-1];
}
/* return the date N days(N*24 hours) before/after some day.
* args : num - positive/negative integer/float number,default is "1";
* type - 0 (second) or 1 (day), default is second.
* return : Date
*/
function dateAfterDays(date, num, type){
date = (date == null?new Date():date);
num = (num == null?1:num);
if(typeof(num)!="number")
throw new SeleniumError("dateAfterDays(date, num, type),""num"" argument must be Number type.");
if(typeof(date)!="object")
throw new SeleniumError("dateAfterDays(date, num, type),""date"" argument must be Date type.");
var iType = (type == null?0:type);
var arr = [1000,86400000];
var dd = date.valueOf();
dd += num * arr[iType];
var d=new Date(dd);
return d;
}
function getSysDate(){
return new Date();
}
the image below shows how to use this function, for details please see the previous code:
使用方法如下,详见以上代码:
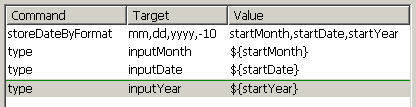
基于数据的功能测试经常要求我们进行数据排序的检验,以下代码是我日常工作中常用的,希望对大家有帮助。
Selenium.prototype.isColumnOrdered = function(locator,order){
var locators = locator.split("|");
var tableLocator = locators[0];
var headNum = parseInt(locators[1]);
var bottomNum = parseInt(locators[2]);
var identifier = locators[3];
var colNum = null;
var elem = null;
if(identifier.indexOf(":") >= 0){
colNum = parseInt(identifier.substring(0,identifier.indexOf(":")));
elem = identifier.substr(identifier.indexOf(":")+1);
}else{
colNum = parseInt(identifier);
}
var table = this.page().findElement(tableLocator);
if(table != null){
for(i=headNum; i<table.rows.length-bottomNum; i++){
if((i+1)<table.rows.length-bottomNum){
var fir = "";
var sec = "";
if(elem != null){
var path1 = tableLocator+"//tr["+ (i+1) +"]/td["+ (colNum+1) +"]//"+elem;
var path2 = tableLocator+"//tr["+ (i+2) +"]/td["+ (colNum+1) +"]//"+elem;
fir = this.getText(path1);
sec = this.getText(path2);
//alert(fir+""n"+sec);
}else{
fir = getText(table.rows[i].cells[colNum]).trim();
sec = getText(table.rows[i+1].cells[colNum]).trim();
}
if(order.toUpperCase() == "A"){
if(fir > sec){
//alert(fir+""n"+sec);
return false;
}
}else if(order.toUpperCase() == "D"){
if( fir < sec){
//alert(fir+""n"+sec);
return false;
}
}
}
}
return true;
}else{
throw new SeleniumError("can't find the element"""+tableLocator+"""");
}
}
how to use:
call function: verifyColumnOrdered
param1 format: tablelocator|rowCountBeforeDataArea|rowCountAfterDataArea
example: //table[2]|2|3
//table[2] - xPath of a table;
2 - there 2 rows before data area in this table;
3 - there are 3 rows after data area in this table
param2: order
A: ascending
D: descending
Selenium.prototype.doStoreRandom = function (variableName) {
random = Math.floor(Math.random() * 10000000);
storedVars[variableName] = random;
}
Selenium.prototype.doDisplayAlert = function (variableName,value) {
alert(value);
}
//target=variableName; value=len
Selenium.prototype.doStoreRandomDefinedLength = function (variableName,len) {
//alert(len);
//alert(variableName);
var randomDefinedLength = (Math.floor(Math.random() * 10000000)).toString();
//alert(randomDefinedLength);
//alert(randomDefinedLength.length);
if(randomDefinedLength.length<len)
{
var needIncreaseLen = len - randomDefinedLength.length;
//alert(needIncreaseLen);
for(var i =0;i<needIncreaseLen;i++)
{
randomDefinedLength += "a";
}
}else if (randomDefinedLength.length>len)
{
var needCutLen = randomDefinedLength.length - len;
//alert(needCutLen);
randomDefinedLength = randomDefinedLength.substr(needCutLen);
}
storedVars[variableName] = randomDefinedLength;
}
(原)Selenium IDE 扩展函数,日期计算与表现。(Selenium IDE extension function: deal with date.)
I use Selenium IDE for performing web functional testing and often work with date, so I created a extension function to deal with date calculate and presentation, the code is listed below:用Selenium IDE做网页的功能测试经常会碰到与日期有关的验证,本人结合实际工作中遇到的应用,写了一个扩展,代码如下:
Selenium.prototype.doStoreDateByFormat = function(format,args){
// format of args: month,day,year
if(args.trim().length < 3)
throw new SeleniumError("arguments must contain ""Month"",""Date"",""Year"" variables!");
var formats = format.split(",");
var days = parseInt(formats[3]);
var sysDate = getSysDate(); //get the sysdate
var specDate = dateAfterDays(sysDate,parseFloat(days),1); //get specified date
var arrArgs = args.split(",");
var month = specDate.getMonth()+1;
var date = specDate.getDate();
var year = specDate.getFullYear();
// get Month string
switch(formats[0].toUpperCase()){
case "MM": // return 2 digits of month number, such as: 01
month = (month+"").length==1?"0"+month:month;
break;
case "MMM": //return the first 3 chars of the month word, such as: Jan
month = this.getMonthShortName(month);
break;
case "MMMM": //return the full word of the month word, such as: January
month = this.getMonthFullName(month);
break;
case "M":
default:
// return 1 digit when month is lower than 10.
// do nothing
}
//get Date string
switch(formats[1].toUpperCase()){
case "DD": //always return 2 digits of the month number, such as: 05
date = (date+"").length==1?"0"+date:date;
break;
case "D":
default:
// return 1 digit when Date is lower than 10.
// do nothing
}
//get Year string
switch(formats[2].toUpperCase()){
case "YY": // return last 2 digits of the year number, such as: 08 (2008)
year = (year+"").substr(2);
break;
case "YYYY":
default:
//return full year number, such: 2008.
}
storedVars[arrArgs[0]] = month;
storedVars[arrArgs[1]] = date;
storedVars[arrArgs[2]] = year;
}
Selenium.prototype.getMonthFullName = function(month){
var monthArr = new Array("January","February","March","April","May","June","July","August","September","October","November","December");
if(month == null){
throw new SeleniumError("you didn't specify a Month");
}
try{
month = parseInt(month);
}catch (e){
throw new SeleniumError("""Month"" is not a Integer!");
}
return monthArr[month-1];
}
/* return the date N days(N*24 hours) before/after some day.
* args : num - positive/negative integer/float number,default is "1";
* type - 0 (second) or 1 (day), default is second.
* return : Date
*/
function dateAfterDays(date, num, type){
date = (date == null?new Date():date);
num = (num == null?1:num);
if(typeof(num)!="number")
throw new SeleniumError("dateAfterDays(date, num, type),""num"" argument must be Number type.");
if(typeof(date)!="object")
throw new SeleniumError("dateAfterDays(date, num, type),""date"" argument must be Date type.");
var iType = (type == null?0:type);
var arr = [1000,86400000];
var dd = date.valueOf();
dd += num * arr[iType];
var d=new Date(dd);
return d;
}
function getSysDate(){
return new Date();
}
the image below shows how to use this function, for details please see the previous code:
使用方法如下,详见以上代码:
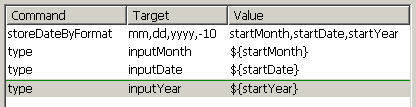
Selenium IDE 扩展函数,判断表格某列是否被排序.(Check whether a column has been ordered.)
a data based functional testing often needs to check the data orderation, I use the function listed below in my daily work, I hope it can help you rise up your efficiency.基于数据的功能测试经常要求我们进行数据排序的检验,以下代码是我日常工作中常用的,希望对大家有帮助。
Selenium.prototype.isColumnOrdered = function(locator,order){
var locators = locator.split("|");
var tableLocator = locators[0];
var headNum = parseInt(locators[1]);
var bottomNum = parseInt(locators[2]);
var identifier = locators[3];
var colNum = null;
var elem = null;
if(identifier.indexOf(":") >= 0){
colNum = parseInt(identifier.substring(0,identifier.indexOf(":")));
elem = identifier.substr(identifier.indexOf(":")+1);
}else{
colNum = parseInt(identifier);
}
var table = this.page().findElement(tableLocator);
if(table != null){
for(i=headNum; i<table.rows.length-bottomNum; i++){
if((i+1)<table.rows.length-bottomNum){
var fir = "";
var sec = "";
if(elem != null){
var path1 = tableLocator+"//tr["+ (i+1) +"]/td["+ (colNum+1) +"]//"+elem;
var path2 = tableLocator+"//tr["+ (i+2) +"]/td["+ (colNum+1) +"]//"+elem;
fir = this.getText(path1);
sec = this.getText(path2);
//alert(fir+""n"+sec);
}else{
fir = getText(table.rows[i].cells[colNum]).trim();
sec = getText(table.rows[i+1].cells[colNum]).trim();
}
if(order.toUpperCase() == "A"){
if(fir > sec){
//alert(fir+""n"+sec);
return false;
}
}else if(order.toUpperCase() == "D"){
if( fir < sec){
//alert(fir+""n"+sec);
return false;
}
}
}
}
return true;
}else{
throw new SeleniumError("can't find the element"""+tableLocator+"""");
}
}
how to use:
call function: verifyColumnOrdered
param1 format: tablelocator|rowCountBeforeDataArea|rowCountAfterDataArea
example: //table[2]|2|3
//table[2] - xPath of a table;
2 - there 2 rows before data area in this table;
3 - there are 3 rows after data area in this table
param2: order
A: ascending
D: descending
相关文章推荐
- Selenium Core and IDE extension
- selenium core/remote control/IDE
- 关于selenium IDE找不到元素
- selenium IDE & Remote Control & Webdriver
- Selenium-IDE,Selenium-RC ,Selenium grid以及 Selenium-Core
- Selenium简介(二)--基于CORE/IDE的简单应用
- Selenium简介(二)--基于CORE/IDE的简单应用
- Selenium简介(二)--基于CORE/IDE的简单应用
- (原)Selenium IDE 扩展函数,日期计算与表现。(Selenium IDE extension function: deal with date.)
- .NET Core+Selenium+Github+Travis CI =&gt; SiteHistory
- 通过添加user-extension.js解决selenium-IDE中的回车和blank问题
- core java 8~9(GUI & AWT事件处理机制)
- Core Graphics使用 >> 绘制心电图
- (OK) Fedora 23——CORE——docker——(5)——> install-core
- selenium ide 安装及操作
- 关于selenium IDE找不到元素
- <PY><core python programming笔记>C5 数字
- selenium IDE录制脚本
- C&C++搭建环境——命令行IDE:git+MinGW
- 菜鸟学自动化测试(二)----selenium IDE 功能扩展