牛腩购物网25:购物车的实现
2012-04-13 16:57
295 查看
第二部分:购物车的实现
我们用session来保存购物车,用一个哈希表来保存商品 购物车
购物车中的每一个商品,都是一个对象,这个对象,有单价,和数量这2个属性,购物车是很多对象的集合。
我们在model层中,增加一个 ShopItem 类, 这个就是表示 购物车 商品类
还增加一个 ShopCart 类,这个就是购物车类,里面有很多对 购物车商品的方法
ShopItem.cs
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
ShopCart.cs
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
我们来建立一个测试页面,来测试购物车。
前台test.aspx 代码
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
后台代码:
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
前台的样式如下,但是,这里的girdview是我们自带的,如果我们想要修改,怎么弄呢?
.png]
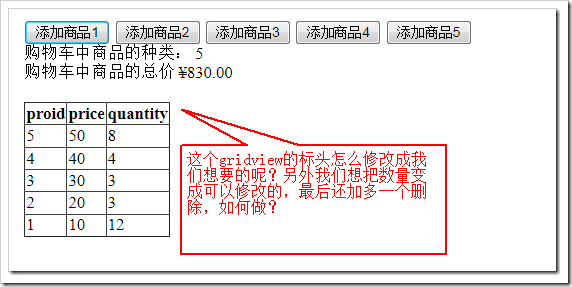
我们先禁用girdview的 自动显示字段, AutoGenerateColumns="false" 然后我们开始编辑列
.png]
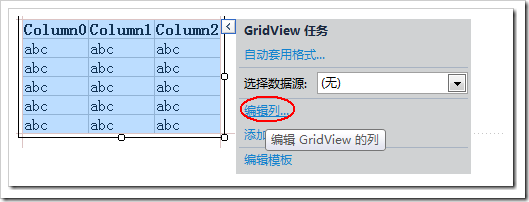
.png]
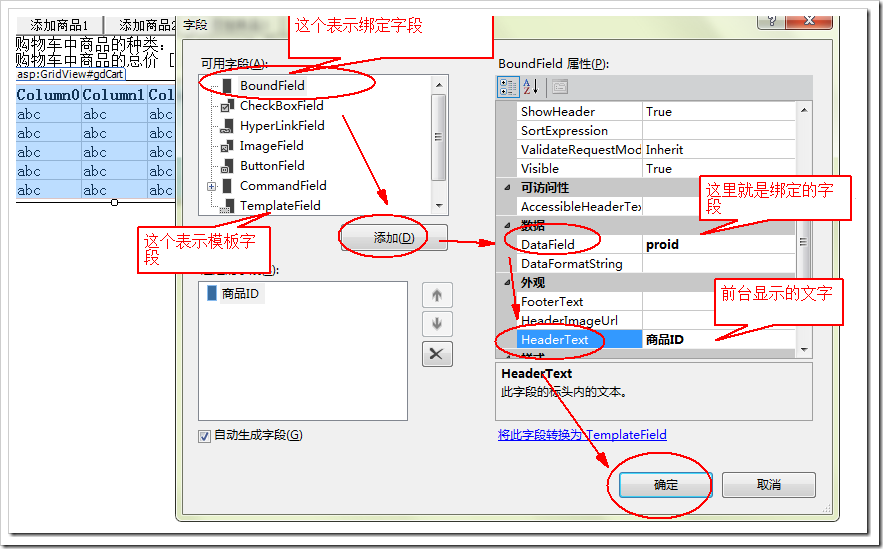
.png]
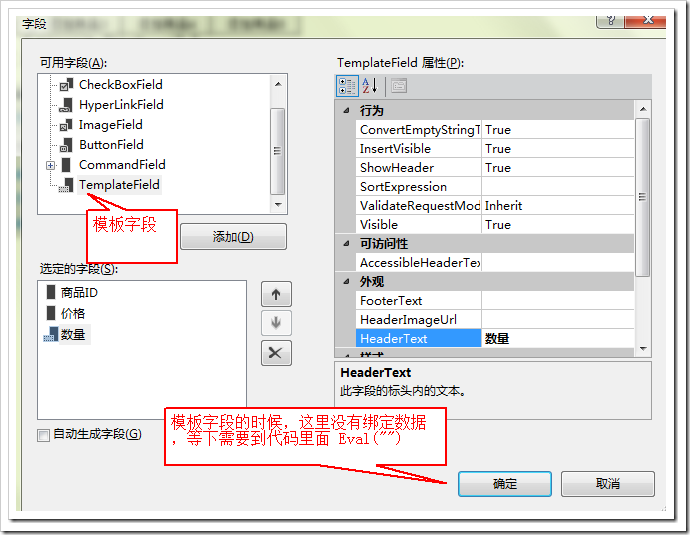
.png]
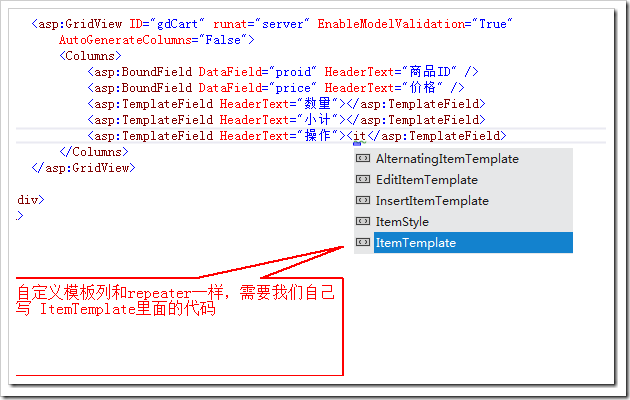
最后,前台代码如下:
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
后台代码:
我们用session来保存购物车,用一个哈希表来保存商品 购物车
商品1:单价,数量 商品2:单价,数量 商品3:单价,数量
购物车中的每一个商品,都是一个对象,这个对象,有单价,和数量这2个属性,购物车是很多对象的集合。
我们在model层中,增加一个 ShopItem 类, 这个就是表示 购物车 商品类
还增加一个 ShopCart 类,这个就是购物车类,里面有很多对 购物车商品的方法
ShopItem.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Niunan.Shop.Model
{
public class ShopItem
{
//这里用到 asp.net 3.5的 自动属性
public int proid { get; set; } //商品ID
public decimal price { get; set; } //商品的价格
public int quantity { set; get; }//商品的数量
}
}
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
ShopCart.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Collections;// 如果我们要使用 哈希表 ,就需要引用这个集合
namespace Niunan.Shop.Model
{
/// <summary>购物车类
///
///</summary>
public class ShopCart
{
private Hashtable _sc = new Hashtable();
///<summary>向购物车中,添加商品
///
///</summary>
///<param name="proid">商品的ID</param>
///<param name="item">商品</param>
public void Add(int proid, ShopItem item)
{
//先判断这个商品是否存在
if (_sc[proid] == null)
{
//不存在
_sc.Add(proid, item);
}
else
{
//如果存在,我们先把商品取出来,然后给商品的数量+1
ShopItem si = _sc[proid] as ShopItem; //这里的 _sc[proid] 是指根据商品ID,获取对应的值,
这个值是个object 我们需要转换为 shopitem
si.quantity += 1;
_sc[proid] = si;
}
}
///<summary>删除购物车中的商品
///
///</summary>
///<param name="proid">商品的ID</param>
public void Delete(int proid)
{
if (_sc[proid] != null)
{
_sc.Remove(proid);
}
}
///<summary>修改购物车中商品的数量
///
///</summary>
///<param name="proid">商品id</param>
///<param name="quantity">数量</param>
public void Update(int proid, int quantity)
{
//先判断有无商品
if (_sc[proid] != null)
{
ShopItem si = _sc[proid] as ShopItem;
//如果数量>0 那么就直接修改数量,否则数量为0的话,就删除掉这个商品
if (quantity > 0)
{
si.quantity = quantity;
}
else
{
_sc.Remove(proid);
}
}
}
///<summary>获取购物车中商品的种类的数量,例如是有多少种商品
///
///</summary>
///<returns></returns>
public int GetItemCount()
{
return _sc.Count; //返回的是 哈希table 键/值 对的数量
}
///<summary>返回购物车中商品的总价
///
///</summary>
///<returns></returns>
public decimal GetTotalPrice()
{
decimal total = 0;
//这里的 _sc.Value 是一个 iCollection, i 表示 Interface, 也就是表示是一个集合的接口
//如果是要绑定 gridview 或者是 repeater 等数据控件 ,必须要实现 icollection接口
foreach (ShopItem item in _sc.Values)
{
total += item.price * item.quantity;
}
return total;
}
///<summary>获取购物车中商品的集合,用于绑定数据控件
///
///</summary>
///<returns></returns>
public ICollection GetItemList()
{
//这里的 _sc.Value 是一个 iCollection, i 表示 Interface, 也就是表示是一个集合的接口
//如果是要绑定 gridview 或者是 repeater 等数据控件 ,必须要实现 icollection接口
return _sc.Values;
}
}
}
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
我们来建立一个测试页面,来测试购物车。
前台test.aspx 代码
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="test.aspx.cs" Inherits="Niunan.Shop.Web.test" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Button ID="Button1" runat="server" Text="添加商品1" CommandArgument="1" OnClick="buy"/>
<asp:Button ID="Button2" runat="server" Text="添加商品2" CommandArgument="2" OnClick="buy"/>
<asp:Button ID="Button3" runat="server" Text="添加商品3" CommandArgument="3" OnClick="buy"/>
<asp:Button ID="Button4" runat="server" Text="添加商品4" CommandArgument="4" OnClick="buy"/>
<asp:Button ID="Button5" runat="server" Text="添加商品5" CommandArgument="5" OnClick="buy"/>
</div>
<div>
购物车中商品的种类:
<asp:Literal ID="litCount" runat="server"></asp:Literal><br />
购物车中商品的总价
<asp:Literal ID="litTotalPrice" runat="server"></asp:Literal>
<br />
<br />
<asp:GridView ID="gdCart" runat="server">
</asp:GridView>
</div>
</form>
</body>
</html>
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
后台代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace Niunan.Shop.Web
{
public partial class test : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (Session["cart"]!=null)
{
Niunan.Shop.Model.ShopCart sc = Session["cart"] as Niunan.Shop.Model.ShopCart;
litCount.Text = sc.GetItemCount().ToString();
litTotalPrice.Text = sc.GetTotalPrice().ToString("c2");
gdCart.DataSource = sc.GetItemList();
gdCart.DataBind();
}
}
protected void buy(object sender, EventArgs e)
{
string proid = (sender as Button).CommandArgument;
decimal price = int.Parse(proid) * 10;
if (Session["cart"] != null)
{
//如果不等于空的话,我们就先取出来,然后再加进去
Niunan.Shop.Model.ShopCart sc = Session["cart"] as Niunan.Shop.Model.ShopCart;
sc.Add(int.Parse(proid),new Model.ShopItem{
proid=int.Parse(proid),price=price,quantity=1
});
Session["cart"] = sc;
}
else
{
Niunan.Shop.Model.ShopCart sc = new Model.ShopCart();
sc.Add(int.Parse(proid), new Model.ShopItem {
proid = int.Parse(proid),
price = price,
quantity = 1
});
//然后把购物车,放到session里面
Session["cart"] = sc;
}
Response.Redirect(Request.Url.ToString());
}
}
}
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
前台的样式如下,但是,这里的girdview是我们自带的,如果我们想要修改,怎么弄呢?
.png]
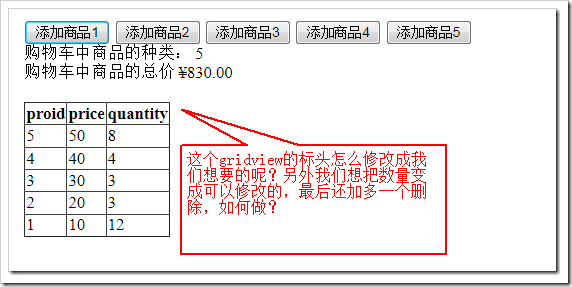
我们先禁用girdview的 自动显示字段, AutoGenerateColumns="false" 然后我们开始编辑列
.png]
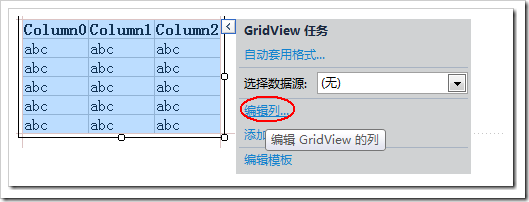
.png]
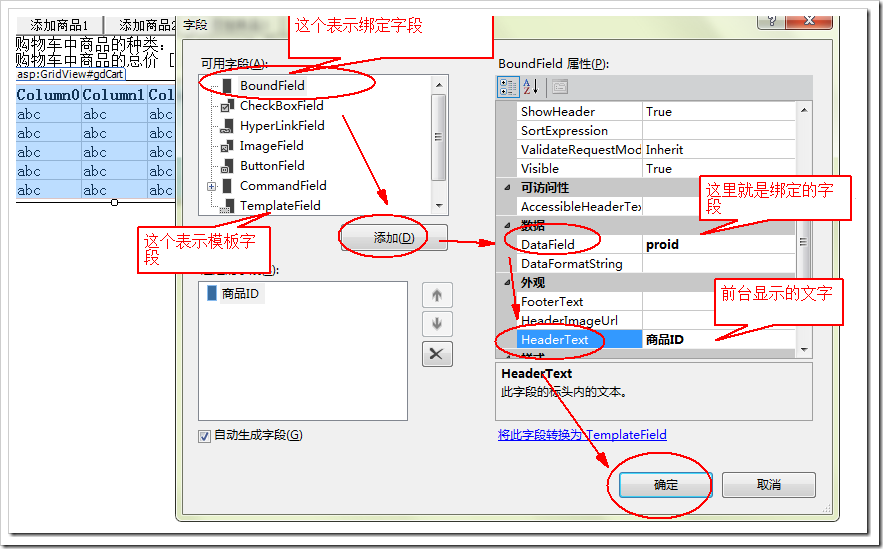
.png]
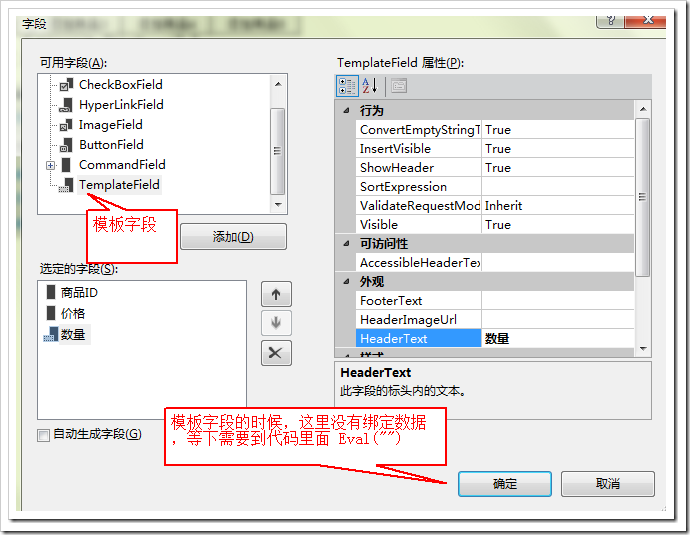
.png]
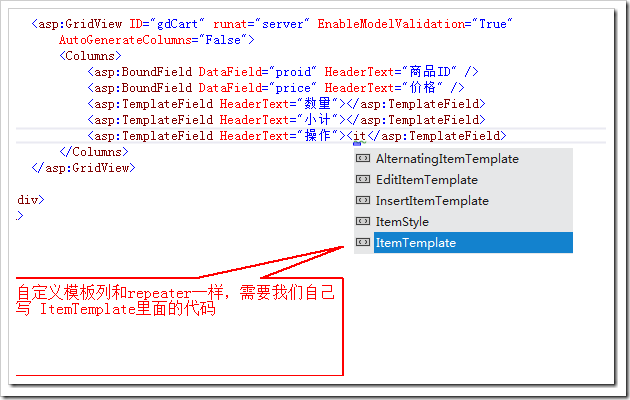
最后,前台代码如下:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="test.aspx.cs" Inherits="Niunan.Shop.Web.test" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Button ID="Button1" runat="server" Text="添加商品1" CommandArgument="1" OnClick="buy" />
<asp:Button ID="Button2" runat="server" Text="添加商品2" CommandArgument="2" OnClick="buy" />
<asp:Button ID="Button3" runat="server" Text="添加商品3" CommandArgument="3" OnClick="buy" />
<asp:Button ID="Button4" runat="server" Text="添加商品4" CommandArgument="4" OnClick="buy" />
<asp:Button ID="Button5" runat="server" Text="添加商品5" CommandArgument="5" OnClick="buy" />
</div>
<div>
购物车中商品的种类:
<asp:Literal ID="litCount" runat="server"></asp:Literal><br />
购物车中商品的总价
<asp:Literal ID="litTotalPrice" runat="server"></asp:Literal>
<br />
<br />
<asp:GridView ID="gdCart" runat="server" EnableModelValidation="True" AutoGenerateColumns="False">
<Columns>
<asp:BoundField DataField="proid" HeaderText="商品ID" />
<asp:BoundField DataField="price" HeaderText="价格" />
<asp:TemplateField HeaderText="数量">
<ItemTemplate>
<asp:TextBox ID="TextBox1" runat="server" Text='<%#Eval("quantity") %>' ToolTip='<%#Eval("proid") %>' AutoPostBack="true" OnTextChanged="Mod"></asp:TextBox>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="小计">
<ItemTemplate>
<%# decimal.Parse(Eval("price").ToString()) * int.Parse(Eval("quantity").ToString()) %>¥
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="操作">
<ItemTemplate>
<asp:LinkButton ID="LinkButton1" runat="server" OnClick="delete" CommandArgument='<%#Eval("proid") %>'>删除</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
<br />
<br />
</div>
</form>
</body>
</html>
.codearea{ color:black; background-color:white; line-height:18px; border:1px solid #4f81bd; margin:0; width:auto !important; width:100%; overflow:auto; text-align:left; font-size:12px; font-family: "Courier New","Consolas","Fixedsys","BitStream Vera Sans Mono", courier,monospace,serif}
.codearea pre{ color:black; line-height:18px; padding:0 0 0 12px !important; margin:0em; background-color:#fff !important}
.linewrap pre{white-space:pre-wrap; white-space:-moz-pre-wrap; white-space:-pre-wrap; white-space:-o-pre-wrap; word-wrap:break-word; word-break:normal}
.codearea pre.alt{ background-color:#f7f7ff !important}
.codearea .lnum{color:#4f81bd;line-height:18px}
后台代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace Niunan.Shop.Web
{
public partial class test : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack) //回发的时候,我们会在page_load 看到页面上所有的控件的值,在回发的时候都被保存了下来,并且可以访问
{
if (Session["cart"] != null)
{
Niunan.Shop.Model.ShopCart sc = Session["cart"] as Niunan.Shop.Model.ShopCart;
litCount.Text = sc.GetItemCount().ToString();
litTotalPrice.Text = sc.GetTotalPrice().ToString("c2");
gdCart.DataSource = sc.GetItemList();
gdCart.DataBind();
}
}
}
//购买
protected void buy(object sender, EventArgs e)
{
string proid = (sender as Button).CommandArgument;
decimal price = int.Parse(proid) * 10;
if (Session["cart"] != null)
{
//如果不等于空的话,我们就先取出来,然后再加进去
Niunan.Shop.Model.ShopCart sc = Session["cart"] as Niunan.Shop.Model.ShopCart;
sc.Add(int.Parse(proid), new Model.ShopItem
{
proid = int.Parse(proid),
price = price,
quantity = 1
});
Session["cart"] = sc;
}
else
{
Niunan.Shop.Model.ShopCart sc = new Model.ShopCart();
sc.Add(int.Parse(proid), new Model.ShopItem
{
proid = int.Parse(proid),
price = price,
quantity = 1
});
//然后把购物车,放到session里面
Session["cart"] = sc;
}
Response.Redirect(Request.Url.ToString());
}
//修改购物车中商品的数量
protected void Mod(object sender, EventArgs e)
{
TextBox txt = (sender as TextBox); //这里是 TextBox 获取的方法 ,先转换为一个 TextBox 对象
string proid = txt.ToolTip;
string quantity = txt.Text;
int x;
//如果数量不能转换,我们就强制数量为1
if (!int.TryParse(quantity, out x))
{
x = 1;
}
if (Session["cart"] != null)
{
//如果不等于空的话,我们就先取出来,然后再加进去
Niunan.Shop.Model.ShopCart sc = Session["cart"] as Niunan.Shop.Model.ShopCart;
sc.Update(int.Parse(proid), x);
Session["cart"] = sc;
}
Response.Redirect(Request.Url.ToString());
}
//删除购物车中的商品
protected void delete(object sender, EventArgs e)
{
string proid = (sender as LinkButton).CommandArgument;
if (Session["cart"] != null)
{
Niunan.Shop.Model.ShopCart sc = Session["cart"] as Niunan.Shop.Model.ShopCart;
sc.Delete(int.Parse(proid));
Session["cart"] = sc;
Response.Redirect(Request.Url.ToString());
}
}
}
}
相关文章推荐
- 牛腩购物网25:购物车的实现,订单表,订单详情表,建立外键和级联删除,记住一定要写 !page.ispostback 回发判断
- Android开发(25)--framebyframe帧动画并实现启动界面到主界面的跳转
- java web开发之购物车功能实现示例代码
- android购物车二级列表实现+MVP+Okhttp
- python根据BM25实现文本检索
- 句子相似 BM25 python 实现
- android添加购物车动画实现
- vue实现商城购物车功能
- Android记录25-WebView实现离线缓存阅读
- JS 实现 购物车客户端 计算
- 模拟网易邮箱实现全选,全不的功能/使用DataList实现 加入购物车,编辑,删除,更新,取消功能。/试完成Datalist使用存储过程来分页
- 购物车实现过程中的技术
- jQuery使用cookie与json简单实现购物车功能
- View Controller Transition实现京东加购物车效果
- 购物车订单实现
- jQuery实现购物车物品数量的加减
- 每日一题_JavaScript.利用Js操作frameset框架集对象实现购物车?
- ecshop 实现购物车退出不清空
- android购物车逻辑的多种实现
- 购物车选中得到价格实现示例