Android手势识别ViewFlipper触摸动画
2012-03-13 00:00
477 查看
最近项目中用到了ViewFlipper这个类,感觉效果真的很炫,今天自己也试着做了下,确实还不错。
首先在layout下定义viewflipper.xml
这样就OK了 看下效果
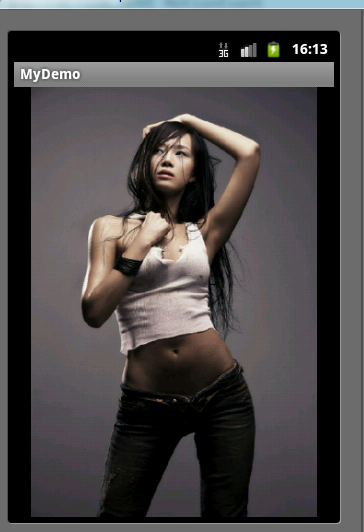
因为是手势识别效果不太好演示,所以大家自己实现了以后就可以看到效果啦!
$(document).ready(function(){dp.SyntaxHighlighter.HighlightAll('code');});
原文链接:
http://blog.csdn.net/wangkuifeng0118/article/details/7098529
首先在layout下定义viewflipper.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <ViewFlipper android:id="@+id/viewFlipper1" android:layout_width="fill_parent" android:layout_height="fill_parent" > <ImageView android:id="@+id/imageView1" android:layout_width="fill_parent" android:layout_height="fill_parent" android:src="@drawable/b" /> <ImageView android:id="@+id/imageView2" android:layout_width="fill_parent" android:layout_height="fill_parent" android:src="@drawable/c" /> <ImageView android:id="@+id/imageView3" android:layout_width="fill_parent" android:layout_height="fill_parent" android:src="@drawable/d" /> <ImageView android:id="@+id/imageView4" android:layout_width="fill_parent" android:layout_height="fill_parent" android:src="@drawable/f" /> <ImageView android:id="@+id/imageView5" android:layout_width="fill_parent" android:layout_height="fill_parent" android:src="@drawable/g" /> </ViewFlipper> </LinearLayout>ViewFlipper中包含了5张图片 用来手势切换。
public class ViewFlipperActivity extends Activity implements OnTouchListener, android.view.GestureDetector.OnGestureListener { private ViewFlipper flipper; GestureDetector mGestureDetector; private int mCurrentLayoutState; private static final int FLING_MIN_DISTANCE = 80; private static final int FLING_MIN_VELOCITY = 150; @Override protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); setContentView(R.layout.viewflipper); flipper=(ViewFlipper) this.findViewById(R.id.viewFlipper1); //注册一个用于手势识别的类 mGestureDetector = new GestureDetector(this); //给mFlipper设置一个listener flipper.setOnTouchListener(this); mCurrentLayoutState = 0; //允许长按住ViewFlipper,这样才能识别拖动等手势 flipper.setLongClickable(true); } /** * 此方法在本例中未用到,可以指定跳转到某个页面 * @param switchTo */ public void switchLayoutStateTo(int switchTo) { while (mCurrentLayoutState != switchTo) { if (mCurrentLayoutState > switchTo) { mCurrentLayoutState--; flipper.setInAnimation(inFromLeftAnimation()); flipper.setOutAnimation(outToRightAnimation()); flipper.showPrevious(); } else { mCurrentLayoutState++; flipper.setInAnimation(inFromRightAnimation()); flipper.setOutAnimation(outToLeftAnimation()); flipper.showNext(); } } } /** * 定义从右侧进入的动画效果 * @return */ protected Animation inFromRightAnimation() { Animation inFromRight = new TranslateAnimation( Animation.RELATIVE_TO_PARENT, +1.0f, Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, 0.0f); inFromRight.setDuration(200); inFromRight.setInterpolator(new AccelerateInterpolator()); return inFromRight; } /** * 定义从左侧退出的动画效果 * @return */ protected Animation outToLeftAnimation() { Animation outtoLeft = new TranslateAnimation( Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, -1.0f, Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, 0.0f); outtoLeft.setDuration(200); outtoLeft.setInterpolator(new AccelerateInterpolator()); return outtoLeft; } /** * 定义从左侧进入的动画效果 * @return */ protected Animation inFromLeftAnimation() { Animation inFromLeft = new TranslateAnimation( Animation.RELATIVE_TO_PARENT, -1.0f, Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, 0.0f); inFromLeft.setDuration(200); inFromLeft.setInterpolator(new AccelerateInterpolator()); return inFromLeft; } /** * 定义从右侧退出时的动画效果 * @return */ protected Animation outToRightAnimation() { Animation outtoRight = new TranslateAnimation( Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, +1.0f, Animation.RELATIVE_TO_PARENT, 0.0f, Animation.RELATIVE_TO_PARENT, 0.0f); outtoRight.setDuration(200); outtoRight.setInterpolator(new AccelerateInterpolator()); return outtoRight; } public boolean onDown(MotionEvent e) { // TODO Auto-generated method stub return false; } /* * 用户按下触摸屏、快速移动后松开即触发这个事件 * e1:第1个ACTION_DOWN MotionEvent * e2:最后一个ACTION_MOVE MotionEvent * velocityX:X轴上的移动速度,像素/秒 * velocityY:Y轴上的移动速度,像素/秒 * 触发条件 : * X轴的坐标位移大于FLING_MIN_DISTANCE,且移动速度大于FLING_MIN_VELOCITY个像素/秒 */ public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX, float velocityY) { if (e1.getX() - e2.getX() > FLING_MIN_DISTANCE && Math.abs(velocityX) > FLING_MIN_VELOCITY) { // 当像左侧滑动的时候 //设置View进入屏幕时候使用的动画 flipper.setInAnimation(inFromRightAnimation()); //设置View退出屏幕时候使用的动画 flipper.setOutAnimation(outToLeftAnimation()); flipper.showNext(); } else if (e2.getX() - e1.getX() > FLING_MIN_DISTANCE && Math.abs(velocityX) > FLING_MIN_VELOCITY) { // 当像右侧滑动的时候 flipper.setInAnimation(inFromLeftAnimation()); flipper.setOutAnimation(outToRightAnimation()); flipper.showPrevious(); } return false; } public void onLongPress(MotionEvent e) { // TODO Auto-generated method stub } public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX, float distanceY) { // TODO Auto-generated method stub return false; } public void onShowPress(MotionEvent e) { // TODO Auto-generated method stub } public boolean onSingleTapUp(MotionEvent e) { // TODO Auto-generated method stub return false; } public boolean onTouch(View v, MotionEvent event) { // TODO Auto-generated method stub // 一定要将触屏事件交给手势识别类去处理(自己处理会很麻烦的) return mGestureDetector.onTouchEvent(event); } }
这样就OK了 看下效果
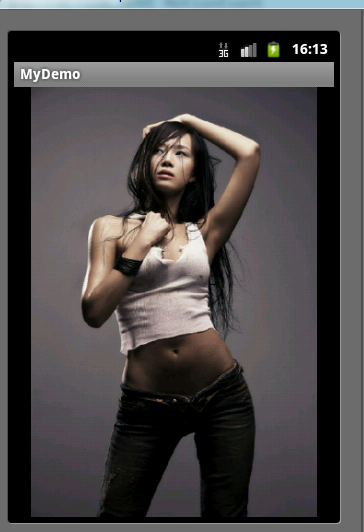
因为是手势识别效果不太好演示,所以大家自己实现了以后就可以看到效果啦!
$(document).ready(function(){dp.SyntaxHighlighter.HighlightAll('code');});
原文链接:
http://blog.csdn.net/wangkuifeng0118/article/details/7098529
相关文章推荐
- Android手势识别ViewFlipper触摸动画
- Android手势识别ViewFlipper触摸动画
- android手势识别ViewFlipper触摸动画
- Android手势识别ViewFlipper触摸动画
- android手势识别ViewFlipper触摸动画
- Android手势识别ViewFlipper触摸动画
- Android手势识别ViewFlipper触摸动画
- Android手势识别ViewFlipper触摸动画
- 【转】Android手势识别ViewFlipper触摸动画
- Android手势识别ViewFlipper触摸动画
- Android手势识别ViewFlipper触摸动画
- android手势识别ViewFlipper触摸动画
- 手势识别ViewFlipper触摸动画
- Android ViewFlipper触摸动画
- Android ViewFlipper触摸动画
- Android ViewFlipper 手势触摸案例
- ViewFlipper(多图层控件)及手势识别,代码创建动画效果
- Android ViewFlipper触摸动画
- Android ViewFlipper 手势触摸案例
- Android ViewFlipper触摸动画