C# 训练场(三)WinForm 练习:字体选择器,文本框,按钮,超链接
2010-06-03 01:15
435 查看
先看一下效果:
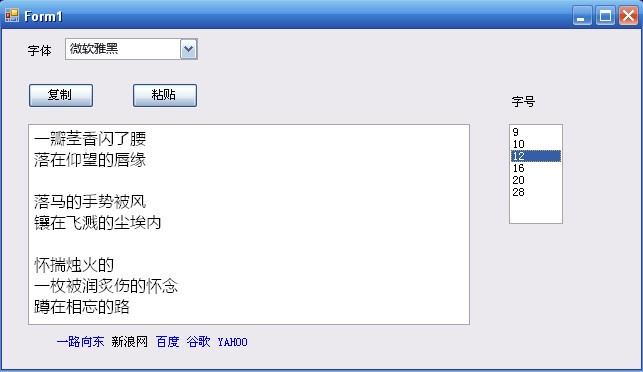
第一部分:Form1.cs
第二部分:Form1.designer.cs
程序入口:program.cs (系统自动)
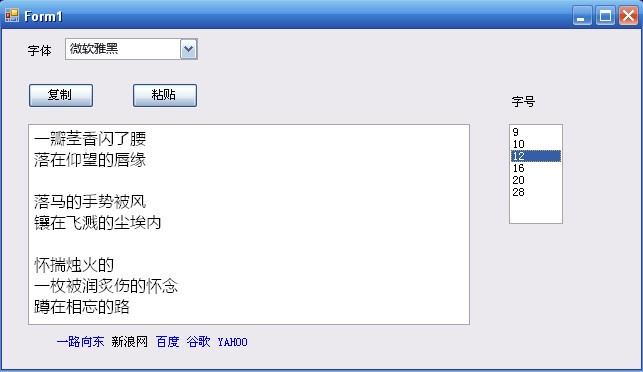
第一部分:Form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace WindowsFormsApplication1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { comboBox1.DrawItem += new DrawItemEventHandler(comboBox1_DrawItem); System.Drawing.Text.InstalledFontCollection ifc = new System.Drawing.Text.InstalledFontCollection(); FontFamily[] ffs = ifc.Families; foreach (FontFamily ff in ffs) //在这里的样式里表示Regular可能使用的字体 if (ff.IsStyleAvailable(FontStyle.Regular)) comboBox1.Items.Add(ff.Name); } private void comboBox1_DrawItem(object sender, DrawItemEventArgs e) { e.DrawBackground(); ComboBox cmb = (ComboBox)sender; string txt = e.Index > -1 ? cmb.Items[e.Index].ToString() : cmb.Text; Font f = new Font(txt, cmb.Font.Size); //使用格式刷 Brush b = new SolidBrush(e.ForeColor); //字符串描绘 float ym = (e.Bounds.Height - e.Graphics.MeasureString(txt, f).Height) / 2; e.Graphics.DrawString(txt, f, b, e.Bounds.X, e.Bounds.Y + ym); f.Dispose(); b.Dispose(); //描绘四角表示焦点的形状 e.DrawFocusRectangle(); } //combobox改变选择事件 private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) { // if (comboBox1.SelectedIndex == -1) // fontname = comboBox1.Items[32].ToString();//未被选择时设置默认,如果下面fontname设置了默认值,这里的if,else就没有必要要了。 // else fontname = comboBox1.Text; textBox1.Font = new Font(fontname,fontsize); } //listbox选择事件 private void listBox1_SelectedIndexChanged(object sender, EventArgs e) { // if (listBox1.SelectedIndex == -1) // fontsize = float.Parse(listBox1.Items[1].ToString());//未被选择时默认为第一个,如果下面fontsize设置了默认值,这里的if,else就没有必要要了。 // else fontsize = float.Parse(listBox1.SelectedItem.ToString()); textBox1.Font = new Font(fontname, fontsize); } //设定fontname,fontsize的默认值 public string fontname = "宋体"; public float fontsize = 9; //按钮1复制_事件 private void button1_Click(object sender, EventArgs e) { if (textBox1.SelectionLength > 0) textBox1.Copy(); } //按钮2粘贴_事件 private void button2_Click(object sender, EventArgs e) { textBox1.Paste(); } //linklabel的点击事件 private void linkLabel1_LinkClicked(object sender, LinkLabelLinkClickedEventArgs e) { linkLabel1.Links[linkLabel1.Links.IndexOf(e.Link)].Visited = true; string targetUrl = e.Link.LinkData as string; if (string.IsNullOrEmpty(targetUrl)) MessageBox.Show("没有链接URL!"); else Help.ShowHelp(this, targetUrl); } } }
第二部分:Form1.designer.cs
namespace WindowsFormsApplication1 { partial class Form1 { /// <summary> /// Required designer variable. /// </summary> private System.ComponentModel.IContainer components = null; /// <summary> /// Clean up any resources being used. /// </summary> /// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param> protected override void Dispose(bool disposing) { if (disposing && (components != null)) { components.Dispose(); } base.Dispose(disposing); } #region Windows Form Designer generated code /// <summary> /// Required method for Designer support - do not modify /// the contents of this method with the code editor. /// </summary> private void InitializeComponent() { this.label1 = new System.Windows.Forms.Label(); this.label2 = new System.Windows.Forms.Label(); this.listBox1 = new System.Windows.Forms.ListBox(); this.comboBox1 = new System.Windows.Forms.ComboBox(); this.button1 = new System.Windows.Forms.Button(); this.button2 = new System.Windows.Forms.Button(); this.textBox1 = new System.Windows.Forms.TextBox(); this.linkLabel1 = new System.Windows.Forms.LinkLabel(); this.SuspendLayout(); // // label1 // this.label1.AutoSize = true; this.label1.Location = new System.Drawing.Point(24, 16); this.label1.Name = "label1"; this.label1.Size = new System.Drawing.Size(29, 12); this.label1.TabIndex = 0; this.label1.Text = "字体"; // // label2 // this.label2.AutoSize = true; this.label2.Location = new System.Drawing.Point(508, 67); this.label2.Name = "label2"; this.label2.Size = new System.Drawing.Size(29, 12); this.label2.TabIndex = 2; this.label2.Text = "字号"; // // listBox1 // this.listBox1.FormattingEnabled = true; this.listBox1.ItemHeight = 12; this.listBox1.Items.AddRange(new object[] { "9", "10", "12", "16", "20", "28"}); this.listBox1.Location = new System.Drawing.Point(507, 95); this.listBox1.Name = "listBox1"; this.listBox1.Size = new System.Drawing.Size(54, 100); this.listBox1.TabIndex = 3; this.listBox1.SelectedIndexChanged += new System.EventHandler(this.listBox1_SelectedIndexChanged); // // comboBox1 // this.comboBox1.DrawMode = System.Windows.Forms.DrawMode.OwnerDrawVariable; this.comboBox1.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList; this.comboBox1.FormattingEnabled = true; this.comboBox1.Location = new System.Drawing.Point(63, 9); this.comboBox1.Name = "comboBox1"; this.comboBox1.Size = new System.Drawing.Size(133, 22); this.comboBox1.TabIndex = 4; this.comboBox1.DrawItem += new System.Windows.Forms.DrawItemEventHandler(this.comboBox1_DrawItem); this.comboBox1.SelectedIndexChanged += new System.EventHandler(this.comboBox1_SelectedIndexChanged); // // button1 // this.button1.Location = new System.Drawing.Point(26, 54); this.button1.Name = "button1"; this.button1.Size = new System.Drawing.Size(66, 25); this.button1.TabIndex = 5; this.button1.Text = "复制"; this.button1.UseVisualStyleBackColor = true; this.button1.Click += new System.EventHandler(this.button1_Click); // // button2 // this.button2.Location = new System.Drawing.Point(130, 54); this.button2.Name = "button2"; this.button2.Size = new System.Drawing.Size(66, 25); this.button2.TabIndex = 6; this.button2.Text = "粘贴"; this.button2.UseVisualStyleBackColor = true; this.button2.Click += new System.EventHandler(this.button2_Click); // // textBox1 // this.textBox1.Location = new System.Drawing.Point(26, 95); this.textBox1.Multiline = true; this.textBox1.Name = "textBox1"; this.textBox1.Size = new System.Drawing.Size(442, 201); this.textBox1.TabIndex = 7; // // linkLabel1 // this.linkLabel1.AutoSize = true; this.linkLabel1.LinkBehavior = System.Windows.Forms.LinkBehavior.HoverUnderline; this.linkLabel1.Location = new System.Drawing.Point(53, 307); this.linkLabel1.Name = "linkLabel1"; this.linkLabel1.Size = new System.Drawing.Size(79, 19); this.linkLabel1.TabIndex = 8; this.linkLabel1.TabStop = true; this.linkLabel1.Text = "一路向东 新浪网 百度 谷歌 YAHOO"; this.linkLabel1.UseCompatibleTextRendering = true; this.linkLabel1.Links.Add(0, 4, "http://blog.csdn.net/imbiz/"); this.linkLabel1.Links.Add(9, 2, "http://www.baidu.com/"); this.linkLabel1.Links.Add(12, 2, "http://www.google.cn/"); this.linkLabel1.Links.Add(15, 5, ""); //以上代码使用links.add方法对一个label添加多个链接,第一,二个参数分别是Text的起始,终点位置 this.linkLabel1.LinkClicked += new System.Windows.Forms.LinkLabelLinkClickedEventHandler(this.linkLabel1_LinkClicked); // // Form1 // this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F); this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font; this.ClientSize = new System.Drawing.Size(639, 340); this.Controls.Add(this.linkLabel1); this.Controls.Add(this.textBox1); this.Controls.Add(this.button2); this.Controls.Add(this.button1); this.Controls.Add(this.comboBox1); this.Controls.Add(this.listBox1); this.Controls.Add(this.label2); this.Controls.Add(this.label1); this.Name = "Form1"; this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen; this.Text = "Form1"; this.Load += new System.EventHandler(this.Form1_Load); this.ResumeLayout(false); this.PerformLayout(); } #endregion private System.Windows.Forms.Label label1; private System.Windows.Forms.Label label2; private System.Windows.Forms.ListBox listBox1; private System.Windows.Forms.ComboBox comboBox1; private System.Windows.Forms.Button button1; private System.Windows.Forms.Button button2; private System.Windows.Forms.TextBox textBox1; private System.Windows.Forms.LinkLabel linkLabel1; } }
程序入口:program.cs (系统自动)
using System; using System.Collections.Generic; using System.Linq; using System.Windows.Forms; namespace WindowsFormsApplication1 { static class Program { /// <summary> /// The main entry point for the application. /// </summary> [STAThread] static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); Application.Run(new Form1()); } } }
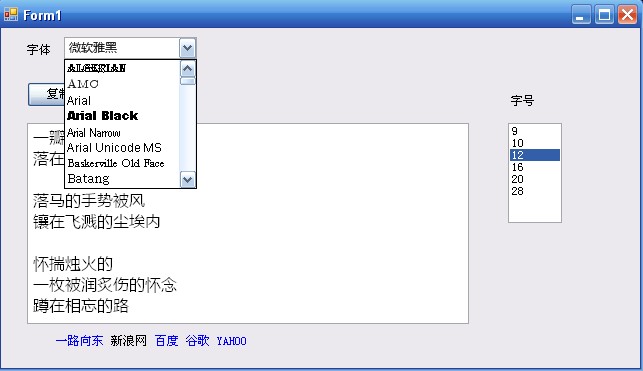
相关文章推荐
- 。窗体上有一个文本框(多行、且带有垂直滚动条)、一个标签(字体颜色红色、字号16)、一个按钮(该按钮被单击时,实现将文本框中选择文本复制至标签。
- C# WinForm Label 控件拓展—变色字体、超链接
- C# WinForm下DataGridView单选按钮列和支持三种选择状态的复选框列的实现
- C# WinForm下DataGridView单选按钮列和支持三种选择状态的复选框列的实现
- 点击button按钮后,启动一个实现鼠标拖动另一个button的线程 (JFrame/C# WinForm)
- C# winform TreeView中关于checkbox选择的完美类(转载)
- XP系统下设置禁止选择更改显示在屏幕上的窗口和按钮的字体大小
- C#对话框 保存对话框、打开对话框的实现、颜色文件框、字体文本框
- 英语音标的录入。点击按钮,点击图片,点击图标等,在RTF文本框中显示字体中的字符。
- c# winform取消右上角关闭按钮的实现方法
- C# winform 按钮设置左边图标
- C#实现在WinForm窗口标题栏上添加按钮
- c#winform选择文件,文件夹,打开指定目录方法
- C# WinForm开发 取消窗体关闭按钮
- WinForm开发中针对TreeView控件改变当前选择节点的字体与颜色
- C# WinForm开发 取消窗体关闭按钮
- 在C#.net中做页面上传的程序。用Dhtml的控件:(创建文件上载控件,该控件带有一个文本框和一个浏览按钮。)和类HtmlInputFile的两种方法
- C#Winform限制TextBox文本框只能输入文本的格式
- C#(WinForm)ComboBox和ListBox添加项及设置默认选择项
- C#|Winform应用程序之:超链接标签(linkLabel)控件