设计模式----Command模式
2010-02-04 23:35
337 查看
命令模式(Command)的目标是将一个请求封装成一个对象,因此可以参数化多个客户的不同请求,将请求排除,记录请求日志,并支持撤消操作。 结构图如下:
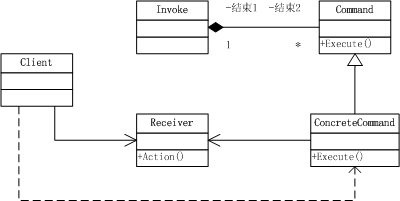
其实现思想是将一个请求封装到一个类中(Command),再提供接收对象(Receiver),最后Command命令由Invoker调用。
以一个电灯开关为例,命令的执行、不执行相对于开关的打开、关闭操作,由开关发出命令,电灯接收命令,结构图如下:
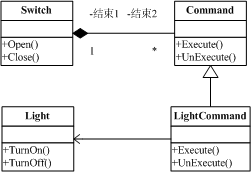
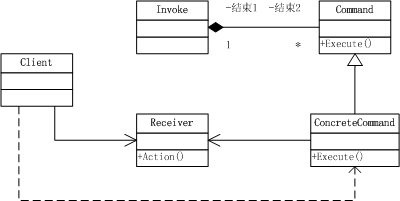
其实现思想是将一个请求封装到一个类中(Command),再提供接收对象(Receiver),最后Command命令由Invoker调用。
以一个电灯开关为例,命令的执行、不执行相对于开关的打开、关闭操作,由开关发出命令,电灯接收命令,结构图如下:
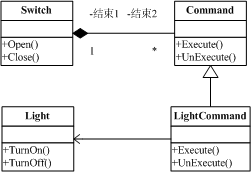
#include <iostream> using namespace std; class Light { public: Light(){}; virtual ~Light(){}; void TurnOn() { cout << "电灯打开了" << endl; } void TurnOff() { cout << "电灯关闭了" << endl; } }; class Command { public: virtual ~Command(){} virtual void Execute() = 0; virtual void UnExecute() = 0; protected: Command(){} }; class LightCommand : public Command { public: LightCommand(Light * pLight) { m_pLight = pLight; } virtual ~LightCommand() { if(m_pLight != NULL) { delete m_pLight; m_pLight = NULL; } } void Execute() { m_pLight->TurnOn(); } void UnExecute() { m_pLight->TurnOff(); } private: Light* m_pLight; }; class Switch { public: Switch(Command* pCommand) { m_pCommand = pCommand; } virtual ~Switch(){} void Open() { m_pCommand->Execute(); } void Close() { m_pCommand->UnExecute(); } private: Command* m_pCommand; }; int main(int argc, char* argv[]) { Light* pLight = new Light; Command* pCommand = new LightCommand(pLight); Switch* pSwitch = new Switch(pCommand); pSwitch->Open(); pSwitch->Close(); return 0; }
相关文章推荐
- 设计模式之Command模式(学习笔记)
- PHP设计模式:命令Command模式
- 我是如何学习设计模式的七:中介者模式—和command模式,观察者模式有一定关系
- 设计模式—Command模式
- 设计模式学习笔记十五——Command模式
- 设计模式----Command模式
- 【JAVA】设计模式之命令模式(Command模式)的使用分析
- [GoF设计模式]抽象工厂模式和Command模式的C++实现
- 设计模式——command模式
- 设计模式-command模式
- 设计模式-----COMMAND模式
- 设计模式----Command模式
- 设计模式实战:在WinForm中用Command模式实现可以撤销的数据操作
- Command模式详解--设计模式(19)
- 常见设计模式的解析和实现(C++)之十四-Command模式
- 设计模式之command模式(看了好多文章,只有这篇让我豁然开朗。)
- 设计模式(c++)笔记之十九(Command模式)
- 设计模式学习之command模式
- 设计模式之Command模式(笔记)
- 设计模式:利用Command模式实现无限次数的Undo/Redo功能