WCF:如何创建带有异步访问委托的代理类
2009-12-10 15:16
253 查看
1. 合约
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
2. 服务
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
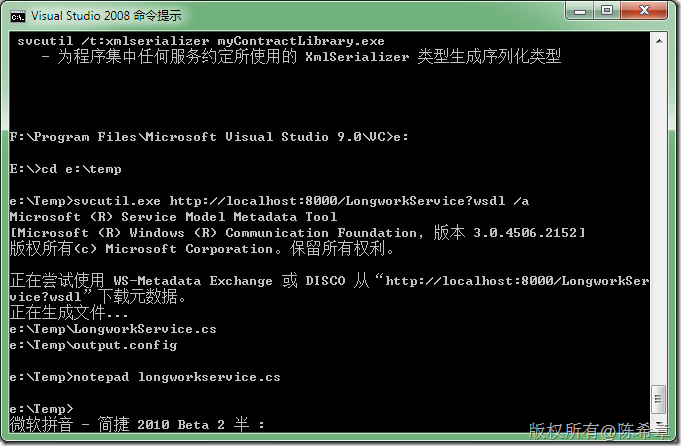
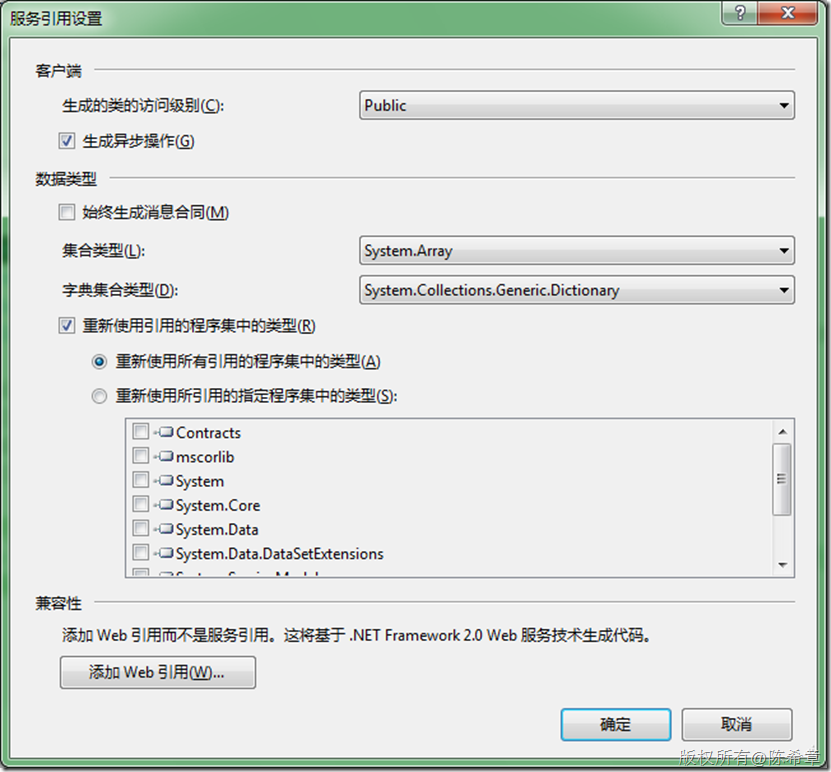
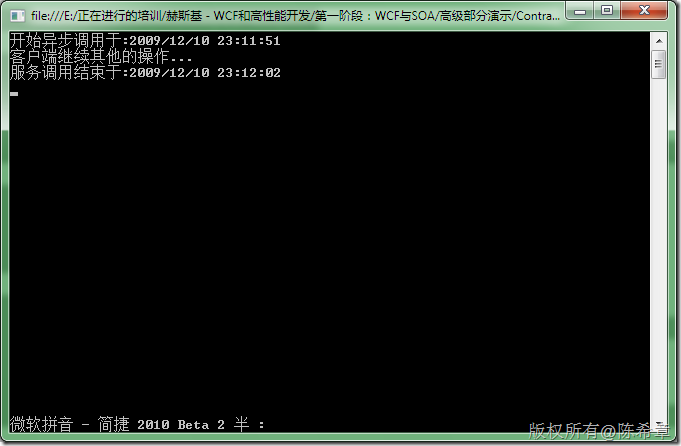
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; namespace Contracts { [ServiceContract] public interface ILongworker { [OperationContract] void Dowork(); } }
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
2. 服务
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Services { public class LongworkService:Contracts.ILongworker { #region ILongworker 成员 public void Dowork() { //这是一个长时间工作的服务方法 System.Threading.Thread.Sleep(10000);//设置休眠10秒钟 Console.WriteLine("服务器执行完了操作"); } #endregion } }
3. 宿主
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; using System.ServiceModel.Description; namespace LongworkServiceHost { class Program { static void Main(string[] args) { #region 长时间工作的服务 using (ServiceHost host = new ServiceHost( typeof(Services.LongworkService), new Uri("http://localhost:8000/LongworkService") )) { host.AddServiceEndpoint( "Contracts.ILongworker", new BasicHttpBinding(), ""); ServiceMetadataBehavior behavior = new ServiceMetadataBehavior(); behavior.HttpGetEnabled = true; host.Description.Behaviors.Add(behavior); host.AddServiceEndpoint( "IMetadataExchange", MetadataExchangeBindings.CreateMexHttpBinding(), "mex"); host.Open(); Console.WriteLine("服务器已经准备好"); Console.Read(); } #endregion } } }
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
4. 客户端 首先如何生成带有异步操作的代理类。可以采用带有/a参数的svcutil工具,也可以在添加引用那个对话框中指定生成异步操作,如下图所示
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
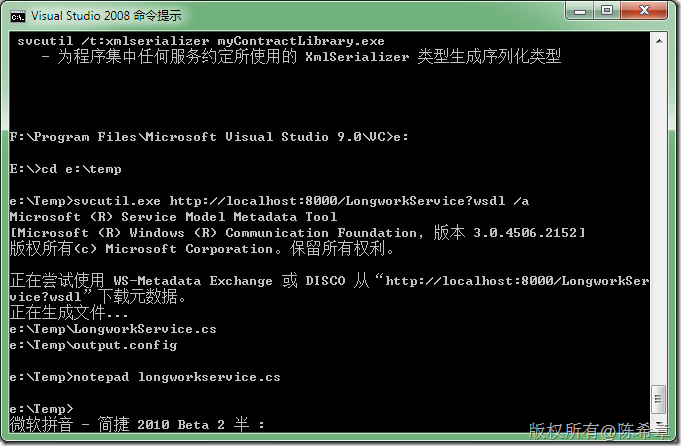
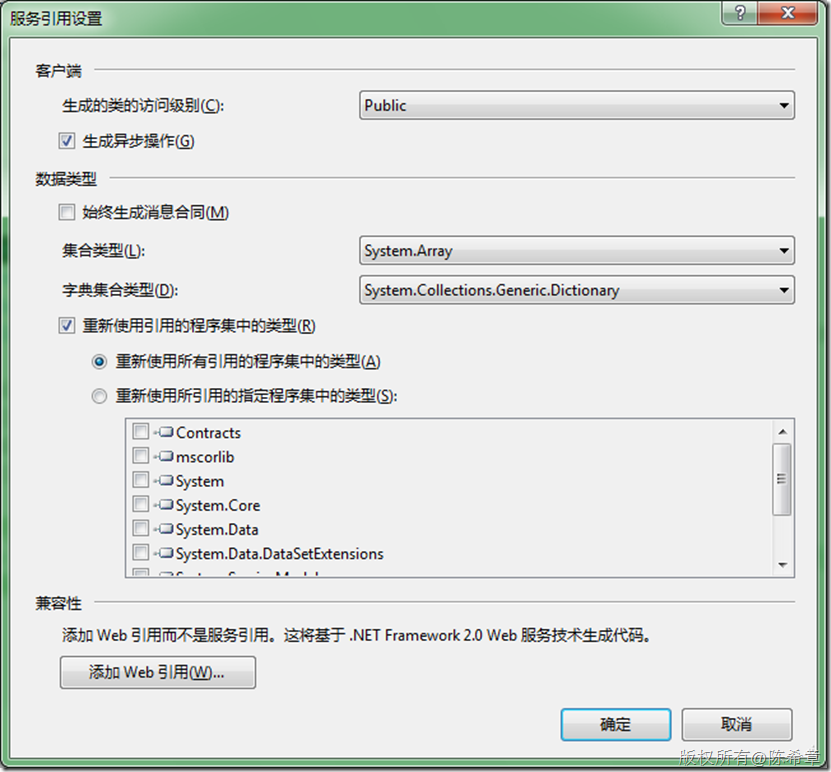
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; namespace LongworkServiceClient { class Program { static void Main(string[] args) { //第一步:默认的调用(同步) //Contracts.ILongworker proxy = // new ChannelFactory( // new BasicHttpBinding(), // new EndpointAddress("http://localhost:8000/LongworkService")).CreateChannel(); //Console.WriteLine("同步调用开始于:{0}", DateTime.Now); //proxy.Dowork(); //Console.WriteLine("同步调用结束于:{0}", DateTime.Now); //Console.Read(); //第二步:采用异步的调用 //svcutil.exe http://localhost:8000/LongworkService?wsdl /a 创建异步委托 //通过添加服务中指定异步选项创建代理类 LongworkerClient client = new LongworkerClient( new BasicHttpBinding(), new EndpointAddress("http://localhost:8000/LongworkService")); Console.WriteLine("开始异步调用于:{0}", DateTime.Now); //client.Dowork();//如果这样调用,则是同步调用 client.BeginDowork(new AsyncCallback(OnCallback), null); Console.WriteLine("客户端继续其他的操作..."); Console.Read(); } static void OnCallback(IAsyncResult ar) { Console.WriteLine("服务调用结束于:{0}", DateTime.Now); } } }
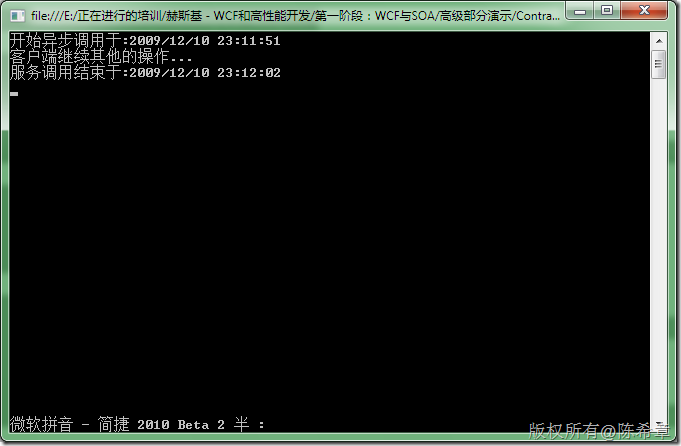
.csharpcode, .csharpcode pre
{
font-size: small;
color: black;
font-family: consolas, "Courier New", courier, monospace;
background-color: #ffffff;
/*white-space: pre;*/
}
.csharpcode pre { margin: 0em; }
.csharpcode .rem { color: #008000; }
.csharpcode .kwrd { color: #0000ff; }
.csharpcode .str { color: #006080; }
.csharpcode .op { color: #0000c0; }
.csharpcode .preproc { color: #cc6633; }
.csharpcode .asp { background-color: #ffff00; }
.csharpcode .html { color: #800000; }
.csharpcode .attr { color: #ff0000; }
.csharpcode .alt
{
background-color: #f4f4f4;
width: 100%;
margin: 0em;
}
.csharpcode .lnum { color: #606060; }
相关文章推荐
- WCF:如何创建带有异步访问委托的代理类
- 用委托在listbox中异步显示信息,解决线程间操作无效,从不是创建控件的线程访问它
- 使用代理类访问WCF应用(不添加web引用、不使用管道模式)以及如何调试WCF
- 谈.Net委托与线程——创建无阻塞的异步调用
- 如何:创建异步 Web 服务方法
- 如何创建一个简单的基于OleTx协议的WCF事务实例
- 如何使用花生壳 发布WCF服务 进行外网访问 z
- 【转载】WCF热门问题编程示例(4):WCF客户端如何异步调用WCF服务?
- FAQ: 如何动态创建并访问网页元素
- Kivy A to Z -- 如何从Python创建一个基于Binder的Service及如何从Java访问Python创建的Service
- Silverlight实用窍门系列:30.Silverlight中创建一个最简单的WCF RIA Services访问数据库实例【实例源码+数据库下载】
- 教你如何解决“线程间操作无效: 从不是创建控件的线程访问它”
- [原创]WCF后续之旅(2): 如何对Channel Layer进行扩展——创建自定义Channel
- Silverlight 客户端如何访问WCF
- asp fso读取文件夹,如何按照(1:按创建时间 2:按访问时间 3:按修改时间 4:按名称)排序(2008-09-18,11:19:18)
- 如何:使用反射提供程序创建数据服务(WCF 数据服务)
- .NET Core/Framework如何创建委托大幅度提高反射调用的性能详解
- 如何创建一个ContentProvider,提供给其他App访问
- CXF学习笔记一:如何创建、发布和访问基于CXF的服务